Can You Index a Dictionary in Python? Exploring the Basics and Beyond
Can You Index A Dictionary In Python?
In the world of programming, dictionaries are one of the most versatile and powerful data structures available, particularly in Python. They allow developers to store data in key-value pairs, making it easy to access and manipulate information efficiently. As you dive deeper into the intricacies of Python, you might find yourself pondering a fundamental question: Can you index a dictionary in Python? This inquiry opens the door to a rich discussion about how dictionaries operate, their unique characteristics, and the various ways you can interact with them.
Unlike lists or tuples, which are indexed by their position, dictionaries are indexed by keys. This distinction is crucial, as it shapes how you retrieve and manage data within this dynamic structure. Understanding the concept of indexing in dictionaries not only enhances your programming skills but also empowers you to leverage the full potential of Python’s capabilities. Throughout this article, we will explore the nuances of dictionary indexing, including how to access values, the implications of key uniqueness, and practical examples that illustrate these principles in action.
As you embark on this journey, prepare to uncover the subtleties of dictionary operations and the creative ways you can utilize them in your coding projects. Whether you’re a beginner looking to solidify your understanding or an experienced programmer seeking to refine your
Understanding Dictionary Indexing in Python
In Python, dictionaries are a versatile data structure that store values in key-value pairs. Unlike lists, which are indexed by their position, dictionaries are indexed by their keys. This unique feature allows for rapid data retrieval without needing to traverse the entire structure.
To access a value in a dictionary, you use its corresponding key within square brackets. For example, if you have a dictionary called `my_dict`, you can retrieve a value associated with the key `key1` using the syntax `my_dict[‘key1’]`. If the key does not exist, a `KeyError` is raised.
Basic Operations on Dictionaries
Here are some fundamental operations you can perform on dictionaries:
- Creating a Dictionary: You can create a dictionary by enclosing key-value pairs in curly braces.
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 25, ‘city’: ‘New York’}
“`
- Accessing Values: Use the key to retrieve values.
“`python
age = my_dict[‘age’] Returns 25
“`
- Adding or Updating a Key-Value Pair: If a key already exists, assigning a new value updates it; if not, it adds a new key-value pair.
“`python
my_dict[‘age’] = 26 Updates age to 26
my_dict[‘country’] = ‘USA’ Adds new key ‘country’
“`
- Deleting a Key-Value Pair: The `del` statement removes a specified key and its associated value.
“`python
del my_dict[‘city’] Removes ‘city’ key
“`
Dictionary Methods
Python dictionaries come with several built-in methods that enhance their functionality. Below is a table summarizing key methods along with their descriptions:
Method | Description |
---|---|
get(key, default) | Returns the value for the specified key if the key is in the dictionary; otherwise, it returns the default value. |
keys() | Returns a view object that displays a list of all the keys in the dictionary. |
values() | Returns a view object that displays a list of all the values in the dictionary. |
items() | Returns a view object that displays a list of dictionary’s key-value tuple pairs. |
pop(key, default) | Removes the specified key and returns its value. If the key is not found, it returns the default value if provided. |
By utilizing these methods, you can efficiently manage and manipulate data stored within dictionaries, making them a powerful tool in Python programming.
Conclusion on Dictionary Indexing
Indexing a dictionary in Python is straightforward and efficient, leveraging the unique key-based access mechanism. Understanding how to effectively interact with dictionaries through indexing and their methods enhances your ability to manage data within your applications efficiently.
Understanding Dictionary Indexing in Python
In Python, dictionaries are implemented as hash tables, which allow for efficient data retrieval. However, the concept of “indexing” in the traditional sense, as seen in lists or tuples, does not apply directly to dictionaries.
Accessing Values by Keys
Instead of using numeric indices, dictionaries use keys to access their values. Each key is unique and serves as a reference point to retrieve the associated value.
- Syntax: `dictionary[key]`
- Example:
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 25}
print(my_dict[‘name’]) Output: Alice
“`
Dictionary Keys and Values
Dictionaries consist of key-value pairs. The keys can be of various immutable types, such as strings, integers, or tuples, while the values can be of any data type.
Key Type | Value Type | Example |
---|---|---|
String | Integer | `{‘age’: 30}` |
Tuple | List | `{‘coordinates’: (10, 20)}` |
Integer | String | `{‘id’: ‘user123’}` |
Retrieving All Keys and Values
To access all keys or values in a dictionary, you can use the following methods:
- Keys: `dictionary.keys()`
- Values: `dictionary.values()`
- Items: `dictionary.items()` (returns key-value pairs)
Example:
“`python
my_dict = {‘name’: ‘Alice’, ‘age’: 25}
print(my_dict.keys()) Output: dict_keys([‘name’, ‘age’])
print(my_dict.values()) Output: dict_values([‘Alice’, 25])
print(my_dict.items()) Output: dict_items([(‘name’, ‘Alice’), (‘age’, 25)])
“`
Checking for Key Existence
Before accessing a value using a key, it is prudent to check if the key exists in the dictionary. This can be accomplished using the `in` keyword.
- Syntax: `key in dictionary`
- Example:
“`python
if ‘name’ in my_dict:
print(my_dict[‘name’])
“`
Modifying Dictionary Values
Values in a dictionary can be updated or modified by assigning a new value to an existing key.
- Example:
“`python
my_dict[‘age’] = 26 Updates the age to 26
“`
Adding New Key-Value Pairs
Adding a new key-value pair is straightforward. Simply assign a value to a new key.
- Example:
“`python
my_dict[‘city’] = ‘New York’ Adds a new key-value pair
“`
Removing Key-Value Pairs
To remove a key-value pair, the `del` statement or the `pop()` method can be used.
- Using del:
“`python
del my_dict[‘age’] Removes the key ‘age’
“`
- Using pop():
“`python
age = my_dict.pop(‘age’, None) Removes ‘age’ and returns its value
“`
Iterating Through a Dictionary
Looping through a dictionary allows you to access keys and values dynamically.
- Example:
“`python
for key, value in my_dict.items():
print(f”{key}: {value}”)
“`
In summary, while dictionaries do not use traditional indexing, they offer robust methods for accessing and manipulating data through keys. This flexibility is one of the many reasons dictionaries are a favored data structure in Python programming.
Understanding Dictionary Indexing in Python: Expert Insights
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, dictionaries are inherently unordered collections of key-value pairs, which means they do not support traditional indexing like lists or arrays. Instead, you can access values using their unique keys, making dictionaries highly efficient for lookups.”
James Liu (Data Scientist, Analytics Hub). “While you cannot index a dictionary in the conventional sense, you can utilize methods such as `.keys()`, `.values()`, and `.items()` to retrieve data in a structured manner. This allows for flexible data manipulation and retrieval based on your specific needs.”
Sarah Thompson (Software Engineer, CodeCraft Solutions). “It’s important to recognize that the lack of indexing in dictionaries is by design, promoting a more semantic approach to data retrieval. This design choice enhances performance and allows developers to work with complex data structures more intuitively.”
Frequently Asked Questions (FAQs)
Can you index a dictionary in Python?
No, dictionaries in Python do not support indexing like lists or tuples. Instead, they use keys to access values.
How do you access a value in a Python dictionary?
You can access a value in a dictionary by using its key inside square brackets, like this: `value = my_dict[key]`.
Can you use integers as keys in a Python dictionary?
Yes, integers can be used as keys in a Python dictionary, along with strings, tuples, and other immutable types.
What happens if you try to access a key that does not exist in a dictionary?
If you attempt to access a key that does not exist, Python raises a `KeyError` exception.
Is it possible to iterate over a dictionary in Python?
Yes, you can iterate over a dictionary using loops. You can iterate over keys, values, or key-value pairs using methods like `.keys()`, `.values()`, or `.items()`.
Can you change the value associated with a key in a Python dictionary?
Yes, you can change the value associated with a key by assigning a new value to that key, like this: `my_dict[key] = new_value`.
In Python, a dictionary is a collection of key-value pairs that allows for efficient data retrieval. Indexing a dictionary is not done in the traditional sense, as dictionaries are inherently unordered collections prior to Python 3.7. However, they can be accessed using their unique keys, which serve as the index for retrieving corresponding values. This key-based access is one of the defining features of dictionaries, making them highly versatile for various programming tasks.
It is important to note that while you cannot index a dictionary using numerical indices like you would with a list or tuple, you can still perform operations that resemble indexing. For example, you can use methods such as `.keys()`, `.values()`, and `.items()` to iterate over the dictionary’s keys, values, or key-value pairs. This functionality allows developers to manipulate and access data efficiently, reinforcing the utility of dictionaries in Python programming.
Overall, understanding how to effectively utilize dictionaries and their key-based access is crucial for any Python programmer. This knowledge not only enhances data handling capabilities but also contributes to writing cleaner and more efficient code. As dictionaries continue to evolve with newer versions of Python, staying informed about their features and best practices will further empower developers in their coding endeavors.
Author Profile
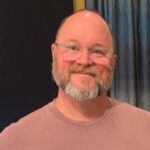
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?