Can We Leverage Partial Classes for XML Serialization in C#?
In the realm of Cprogramming, the concept of partial classes often sparks curiosity among developers looking to streamline their code organization and enhance maintainability. But what happens when you pair this powerful feature with XML serialization? Can we leverage partial classes to improve the way we serialize and deserialize objects in XML format? This article delves into the intriguing intersection of partial classes and XML serialization, exploring their compatibility and the potential benefits they offer for developers seeking efficient solutions in their applications.
Partial classes allow developers to split the definition of a class across multiple files, which can be particularly useful in large projects or when working with auto-generated code. This flexibility enables teams to collaborate more effectively and manage their codebase with greater ease. However, when it comes to XML serialization, understanding how partial classes interact with the serialization process is crucial. As we examine this relationship, we’ll uncover how partial classes can be structured to facilitate seamless XML serialization, making it easier to handle complex data structures.
Moreover, the use of partial classes in XML serialization not only promotes cleaner code but also enhances the ability to extend functionality without modifying existing structures. By strategically organizing properties and methods across different files, developers can maintain clarity in their code while ensuring that the serialization process remains robust and efficient. Join us as we explore the
Understanding Partial Classes in XML Serialization
Partial classes in Callow for the definition of a class across multiple files. This feature is particularly useful for organizing code and separating auto-generated code from custom business logic. When it comes to XML serialization, partial classes can play a significant role in customizing the serialization process without altering the auto-generated code.
XML serialization in Cis typically used to convert an object into an XML format, which can then be easily stored or transmitted. Utilizing partial classes for this purpose can enhance flexibility and maintainability of the code.
Benefits of Using Partial Classes for XML Serialization
The advantages of employing partial classes in XML serialization include:
- Separation of Concerns: Different aspects of the class can be managed separately, making it easier to maintain and update.
- Custom Attributes: You can add custom serialization attributes in a separate file, allowing for clean integration with the auto-generated class code.
- Easier Testing: By isolating custom logic in a partial class, testing becomes more straightforward.
Implementing Partial Classes with XML Serialization
To implement XML serialization using partial classes, follow these steps:
- Define the Main Class: This is usually auto-generated by tools like Visual Studio or serialization frameworks.
- Create a Partial Class: In a separate file, define the same class as partial, where you can add your custom methods or properties.
Here’s a simple example:
“`csharp
// Auto-generated part
public partial class Person
{
public string Name { get; set; }
public int Age { get; set; }
}
// Custom part
public partial class Person
{
public string Address { get; set; }
public void SerializeToXml(string filePath)
{
XmlSerializer serializer = new XmlSerializer(typeof(Person));
using (TextWriter writer = new StreamWriter(filePath))
{
serializer.Serialize(writer, this);
}
}
}
“`
In this example, the `Person` class is split into two files, allowing for the addition of the `Address` property and a custom serialization method without modifying the auto-generated code.
Common Attributes Used in XML Serialization
When working with XML serialization, several attributes can control the serialization behavior:
Attribute | Description |
---|---|
`[XmlRoot]` | Specifies the XML root element. |
`[XmlElement]` | Defines an XML element for a public property. |
`[XmlIgnore]` | Excludes a property from being serialized. |
`[XmlAttribute]` | Indicates that a property should be serialized as an XML attribute. |
For example:
“`csharp
[XmlRoot(“PersonInfo”)]
public partial class Person
{
[XmlElement(“FullName”)]
public string Name { get; set; }
[XmlIgnore]
public int Age { get; set; }
[XmlAttribute(“ResidentialAddress”)]
public string Address { get; set; }
}
“`
This customization allows for precise control over how the object is represented in XML, enhancing interoperability with other systems.
Conclusion on Partial Classes and XML Serialization
Utilizing partial classes in XML serialization not only organizes code effectively but also streamlines the serialization process, making it easier to work with complex data structures. The combination of separation of concerns and the ability to control serialization attributes provides a robust framework for managing XML data in C.
Understanding Partial Classes in C
Partial classes in Callow a single class definition to be split across multiple files. This feature facilitates code organization, particularly in large projects, where different developers can work on different aspects of a class simultaneously. The compiler combines these partial class definitions into a single class at compile time.
Key Benefits of Partial Classes:
- Separation of Concerns: Different functionalities can be isolated, making maintenance easier.
- Collaboration: Multiple developers can work on the same class without causing merge conflicts.
- Auto-Generated Code: Useful in scenarios where code is automatically generated, such as with designer files in WinForms or ASP.NET.
XML Serialization in C
XML serialization in Cconverts an object into an XML format, making it suitable for storage or transmission. The `System.Xml.Serialization` namespace provides classes that facilitate this process, including `XmlSerializer`.
Common Attributes Used in XML Serialization:
- `[XmlRoot]`: Specifies the root element for the XML output.
- `[XmlElement]`: Defines how properties are represented in XML.
- `[XmlAttribute]`: Indicates that a property should be serialized as an XML attribute.
- `[XmlIgnore]`: Prevents a property from being serialized.
Using Partial Classes with XML Serialization
Partial classes can be effectively used with XML serialization, allowing you to organize your code while maintaining the ability to serialize and deserialize objects. When implementing XML serialization with partial classes, ensure that all relevant members across the partial class files are properly decorated with the appropriate serialization attributes.
Considerations for Using Partial Classes with XML Serialization:
- All parts of the partial class should be in the same namespace.
- Serialization attributes can be applied in any of the partial class files.
- Ensure that the class is marked as `public` if it needs to be accessible for serialization.
Example of Partial Class with XML Serialization
Below is an example demonstrating the implementation of partial classes with XML serialization:
“`csharp
// File: Person.Part1.cs
using System.Xml.Serialization;
[XmlRoot(“Person”)]
public partial class Person
{
[XmlElement(“FirstName”)]
public string FirstName { get; set; }
[XmlElement(“LastName”)]
public string LastName { get; set; }
}
// File: Person.Part2.cs
using System.Xml.Serialization;
public partial class Person
{
[XmlElement(“Age”)]
public int Age { get; set; }
[XmlIgnore]
public string SensitiveData { get; set; }
}
“`
In this example:
- The `Person` class is split into two files.
- Serialization attributes are used to specify how properties are serialized.
- The `SensitiveData` property is ignored during serialization, preventing it from being included in the XML output.
Best Practices for Implementing Partial Classes with XML Serialization
When using partial classes in conjunction with XML serialization, adhere to the following best practices:
- Consistent Naming: Ensure that properties across partial class files are consistently named to avoid confusion.
- Documentation: Comment on the purpose of each partial file, especially when dealing with auto-generated code.
- Testing: Thoroughly test serialization and deserialization processes to confirm that all data is correctly handled.
- Version Control: Use version control systems to manage changes in partial classes effectively, ensuring collaboration does not lead to broken functionality.
Conclusion on Partial Classes and XML Serialization
Integrating partial classes with XML serialization in Cprovides a robust framework for managing complex data structures while promoting code organization and maintainability. By adhering to best practices and understanding the nuances of both features, developers can enhance their applications’ readability and functionality.
Exploring the Viability of Partial Classes in XML Serialization
Dr. Emily Carter (Software Architect, Tech Innovations Inc.). “Partial classes can indeed be utilized for XML serialization in C. This approach allows developers to separate the serialization logic from the business logic, enhancing code maintainability and readability. However, it is crucial to ensure that all parts of the partial class are properly synchronized to avoid inconsistencies during serialization.”
Michael Thompson (Lead Developer, CodeCraft Solutions). “Using partial classes for XML serialization offers significant advantages, particularly in large applications where modularity is essential. By splitting the class definition, developers can manage serialization attributes more effectively without cluttering the main class. Nonetheless, careful design is necessary to prevent serialization issues related to missing properties.”
Sarah Nguyen (Technical Consultant, DevOps Strategies). “The use of partial classes in XML serialization is a powerful technique that promotes cleaner code architecture. It allows for the extension of class functionality without modifying the original class file. However, developers must be vigilant about the order of property definitions and ensure that all relevant data is included in the serialization process to avoid runtime errors.”
Frequently Asked Questions (FAQs)
Can we use partial classes for XML serialization in C?
Yes, partial classes can be used for XML serialization in C. When you define a class as partial, the XML serializer can serialize and deserialize properties defined across multiple files seamlessly.
What are the benefits of using partial classes with XML serialization?
Using partial classes allows for better organization of code, especially in large projects. It enables separation of auto-generated code from custom logic, making it easier to maintain and modify without affecting the serialization process.
Do all members of a partial class get serialized?
Not all members of a partial class are serialized by default. Only public properties and fields are serialized unless specific attributes are applied to control the serialization behavior.
How do you control XML serialization in a partial class?
You can control XML serialization in a partial class by using attributes such as [XmlElement], [XmlAttribute], and [XmlIgnore] on the properties or fields. These attributes dictate how the serializer handles each member.
Are there any limitations to using partial classes with XML serialization?
While partial classes provide flexibility, they can introduce complexity if not managed properly. Developers must ensure that all parts of the partial class are consistent and that they do not inadvertently create conflicting members.
Can you serialize private members of a partial class in C?
By default, XML serialization does not include private members. However, you can use the [XmlElement] attribute on private fields or properties to include them in the serialization process if required.
In the context of XML serialization in C, partial classes can indeed be utilized effectively. Partial classes allow developers to split the definition of a class across multiple files, which can be particularly advantageous when working with auto-generated code, such as that produced by tools like Visual Studio’s designer. This feature enables developers to extend the functionality of a class without modifying the generated code directly, thereby preserving the integrity of the auto-generated sections.
When employing partial classes for XML serialization, it is crucial to ensure that all parts of the class are consistent and that the XML serialization attributes are appropriately applied. This means that any properties intended for serialization must be defined in one of the partial class files. Additionally, developers should be mindful of the serialization process, as it relies on the public accessibility of properties and fields, which must be maintained across all partial definitions.
Overall, leveraging partial classes in XML serialization can enhance code organization and maintainability. It allows for a clean separation of concerns, especially in larger projects where auto-generated code and custom logic coexist. By adhering to best practices in structuring partial classes, developers can create robust applications that effectively utilize XML serialization while minimizing potential issues related to code management.
Author Profile
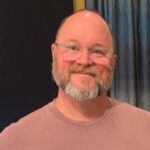
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?