Can a Tuple Serve as a Key in a Python Dictionary?
In the world of Python programming, dictionaries stand out as one of the most versatile and powerful data structures. They allow developers to store and retrieve data efficiently using key-value pairs. While most programmers are familiar with using strings or integers as keys, the question often arises: can a tuple be a key in a dictionary? This inquiry opens the door to a deeper understanding of how Python handles data types and the unique properties that make tuples particularly suitable for this role.
Tuples, being immutable sequences, possess characteristics that align perfectly with the requirements of dictionary keys. Unlike lists, which can be modified and thus cannot serve as keys, tuples maintain their integrity once created. This immutability ensures that the hash value of a tuple remains constant, making it a reliable candidate for use as a key in a dictionary. As we delve into this topic, we will explore the nuances of tuples, their behavior within dictionaries, and the implications of using them as keys in various programming scenarios.
Understanding the mechanics behind using tuples as dictionary keys not only enhances your coding skills but also equips you with the knowledge to leverage Python’s data structures more effectively. As we navigate through examples and best practices, you will discover the flexibility and power of tuples in creating complex data models that can simplify your programming tasks. Whether
Understanding Tuple Immutability
Tuples in Python are immutable sequences, meaning that once they are created, their contents cannot be changed. This immutability is one of the key characteristics that allows tuples to be used as keys in dictionaries. Unlike lists, which are mutable and thus cannot be hashed, tuples can be hashed and are therefore a valid option for dictionary keys.
Using Tuples as Dictionary Keys
In Python, a dictionary is a collection of key-value pairs, where each key is unique. Tuples can serve as keys in a dictionary, providing a way to group related data. The tuple’s immutability ensures that the key remains constant, which is necessary for the integrity of the dictionary.
For example, you can use a tuple containing a combination of values as a key:
“`python
Example of using a tuple as a key
my_dict = {}
my_dict[(1, 2)] = “Value for (1, 2)”
my_dict[(3, 4)] = “Value for (3, 4)”
“`
In this case, the tuple `(1, 2)` and `(3, 4)` serve as unique keys in `my_dict`, each associated with a respective value.
When to Use Tuples as Keys
Using tuples as dictionary keys can be advantageous in various scenarios:
- Grouping Related Data: Tuples can represent composite keys, allowing you to group related data points.
- Maintaining Order: The order of elements in a tuple matters, which is useful when the sequence is significant.
- Fixed Data Points: When you have a fixed number of elements that represent a specific entity.
Limitations of Using Tuples as Keys
While tuples can be used as dictionary keys, there are some limitations to consider:
- All Elements Must Be Hashable: If a tuple contains mutable elements (like lists or dictionaries), it cannot be used as a key.
- Complexity: Using complex tuples as keys might make your code harder to read and maintain.
Limitation | Description |
---|---|
Non-hashable Elements | Tuples containing lists or dictionaries can’t be keys. |
Readability | Complex tuples can lead to less readable code. |
Example Scenarios
Here are a few scenarios where tuples can effectively serve as keys in a dictionary:
- Geographical Coordinates: Storing data points based on latitude and longitude.
“`python
locations = {}
locations[(34.0522, -118.2437)] = “Los Angeles”
locations[(40.7128, -74.0060)] = “New York”
“`
- Date and Event Pairing: Associating a date with a specific event.
“`python
events = {}
events[(“2023-10-01”, “Meeting”)] = “Project Kickoff”
events[(“2023-10-15”, “Deadline”)] = “Submit Report”
“`
tuples provide a powerful way to use composite keys in dictionaries, enhancing the capability to model complex data relationships while ensuring the integrity and immutability of the keys.
Using Tuples as Dictionary Keys
In Python, tuples can indeed be used as keys in a dictionary. This capability arises from the fact that tuples are immutable and hashable, which are essential properties for dictionary keys.
Why Tuples Can Be Dictionary Keys
- Immutability: Tuples cannot be modified after their creation. This ensures that the hash value remains constant, which is crucial for dictionary operations.
- Hashability: A key must be hashable, meaning it must have a fixed hash value during its lifetime. Since tuples are immutable, their hash value remains stable.
Examples of Tuple Keys
Here are some examples demonstrating how tuples can be used as keys in dictionaries:
“`python
Creating a dictionary with tuple keys
coordinates = {
(0, 0): “Origin”,
(1, 1): “Point A”,
(2, 3): “Point B”
}
Accessing a value using a tuple key
print(coordinates[(1, 1)]) Output: Point A
“`
Key Considerations
While tuples are valid keys, there are some important considerations to keep in mind:
- Nested Mutability: If a tuple contains mutable objects (like lists or dictionaries), it cannot be used as a dictionary key because the mutable objects can change, affecting the hash value.
- Performance: Lookup times for dictionaries are generally O(1), but if you are using complex tuples as keys, be mindful of their size and structure, as this may impact performance.
Comparison of Tuple Keys with Other Types
Type | Mutable/Immutable | Hashable | Can be Used as Key |
---|---|---|---|
Tuple | Immutable | Yes | Yes |
List | Mutable | No | No |
Dictionary | Mutable | No | No |
String | Immutable | Yes | Yes |
Practical Use Cases
Using tuples as dictionary keys is particularly useful in scenarios such as:
- Graph Representation: Storing edges with (node1, node2) as keys.
- Multi-dimensional Data: Representing coordinates in multi-dimensional space.
- Caching: Using function arguments as keys for memoization.
Example of using tuples for graph representation:
“`python
graph_edges = {
(1, 2): “Edge from 1 to 2”,
(2, 3): “Edge from 2 to 3”,
(3, 1): “Edge from 3 to 1”
}
Accessing edge information
print(graph_edges[(2, 3)]) Output: Edge from 2 to 3
“`
Tuples provide a robust way to manage complex key structures in dictionaries, enabling efficient data retrieval and manipulation.
Understanding Tuple Keys in Python Dictionaries
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, tuples are immutable and hashable, making them suitable as keys in dictionaries. This property allows developers to use tuples to create complex keys that can represent multiple dimensions of data.”
Michael Chen (Data Scientist, Analytics Solutions Group). “Using tuples as dictionary keys can enhance data organization, especially when dealing with multi-dimensional data. However, developers must ensure that the tuple elements themselves are also hashable to avoid runtime errors.”
Sarah Johnson (Software Engineer, CodeCraft Labs). “While tuples can serve as dictionary keys, one should be cautious about their usage. It is essential to maintain clarity in code, as overly complex tuple keys can lead to confusion and maintenance challenges in larger projects.”
Frequently Asked Questions (FAQs)
Can a tuple be used as a key in a dictionary in Python?
Yes, a tuple can be used as a key in a dictionary in Python because tuples are immutable and hashable.
What types of objects can be used as keys in a Python dictionary?
Keys in a Python dictionary must be immutable and hashable types, such as strings, numbers, and tuples (containing only hashable elements).
Are there any restrictions on the contents of a tuple used as a dictionary key?
Yes, the contents of the tuple must also be hashable. This means it cannot contain mutable types like lists or dictionaries.
Can a dictionary contain tuples as both keys and values?
Yes, a dictionary can contain tuples as keys and also as values, as long as the keys are immutable and hashable.
How do you create a dictionary with tuples as keys?
You can create a dictionary with tuples as keys by defining the tuples in parentheses and assigning them to values, for example: `my_dict = { (1, 2): “value1”, (3, 4): “value2” }`.
What happens if you try to use a mutable object as a key in a dictionary?
If you try to use a mutable object, such as a list, as a key in a dictionary, Python will raise a `TypeError` indicating that the object is unhashable.
In Python, tuples can indeed be used as keys in dictionaries. This is primarily due to the fact that tuples are immutable, which means their contents cannot be altered after creation. This immutability is a crucial characteristic that allows tuples to maintain a consistent hash value, making them suitable for use as dictionary keys. In contrast, mutable data types, such as lists, cannot be used as dictionary keys because their contents can change, leading to unpredictable behavior in key-value mappings.
Another important aspect to consider is that the elements contained within the tuple must also be hashable. This means that any element of the tuple should either be an immutable type, such as strings, numbers, or other tuples, or a user-defined object that implements the hashing protocol. If any element within a tuple is mutable, the tuple itself cannot be used as a dictionary key, which is a key limitation to keep in mind when designing data structures in Python.
In summary, using tuples as dictionary keys can be a powerful feature in Python programming, allowing for complex data structures and efficient data retrieval. Developers should ensure that the tuples are composed of hashable elements to fully leverage this capability. Understanding these principles can enhance the effectiveness of data handling and manipulation in Python applications.
Author Profile
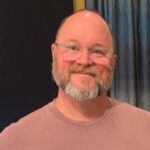
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?