How Can You Call a PowerShell Script From Another PowerShell Script?
In the ever-evolving landscape of IT automation and scripting, PowerShell stands out as a powerful tool for system administrators and developers alike. Its versatility allows users to streamline tasks, manage configurations, and automate workflows with ease. However, as scripts grow in complexity, the need to modularize code becomes apparent. One effective way to achieve this is by calling a PowerShell script from another PowerShell script. This practice not only enhances code organization but also promotes reusability, making it a fundamental skill for anyone looking to optimize their scripting capabilities.
When you call a PowerShell script from another script, you unlock a world of possibilities. This method allows you to break down large scripts into smaller, more manageable components, each handling specific tasks. By leveraging this approach, you can maintain cleaner code, reduce redundancy, and simplify troubleshooting. Additionally, it facilitates collaboration among team members, as different scripts can be developed independently and integrated seamlessly.
As you delve deeper into the intricacies of invoking scripts within PowerShell, you’ll discover various techniques and best practices that can enhance your scripting efficiency. From passing parameters to handling return values, understanding how to effectively call scripts will empower you to create more dynamic and responsive automation solutions. Get ready to elevate your PowerShell skills and streamline your scripting processes like never before
Methods to Call a PowerShell Script
Calling a PowerShell script from another PowerShell script can be accomplished through various methods. Each method has its own use cases and implications depending on the scripting context, parameters needed, and the environment.
Using the & Operator
The `&` operator (known as the call operator) is a straightforward method to invoke a script. This allows you to run a script file directly, passing any necessary parameters.
Example:
powershell
& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2
This method is ideal for executing scripts in the same context as the caller and can handle parameters seamlessly.
Using the . (Dot-Sourcing) Operator
Dot-sourcing allows you to run a script in the current scope, which means any variables or functions defined in the called script will remain available after execution.
Example:
powershell
. “C:\Path\To\YourScript.ps1”
This method is beneficial when you want to preserve the state or variables defined in the called script for further use.
Using the Start-Process Cmdlet
The `Start-Process` cmdlet enables you to start a new process, which can be useful when you want to run a script independently of the calling script’s session. This method is particularly useful when dealing with scripts that may require elevated permissions or need to run in a separate window.
Example:
powershell
Start-Process “powershell.exe” -ArgumentList “-File C:\Path\To\YourScript.ps1”
This approach is advantageous when you want to run scripts asynchronously or in a different environment.
Passing Parameters to Scripts
When calling scripts, you can pass parameters that the called script can utilize. This is done by defining parameters in the called script and specifying them in the call.
Example of parameter definition in a script:
powershell
param(
[string]$Param1,
[int]$Param2
)
When calling the script, you can pass these parameters as shown earlier.
Best Practices
To ensure clarity and maintainability in your scripts, consider the following best practices:
- Always use full paths to script files to avoid ambiguity.
- Employ meaningful parameter names that convey their purpose.
- Include comments in your scripts to document their purpose and usage.
- Handle errors gracefully using try-catch blocks.
Common Pitfalls
While calling scripts, be aware of common pitfalls:
Issue | Description | Solution |
---|---|---|
Script not found | The path to the script is incorrect. | Verify and use absolute paths. |
Scope issues | Variables/functions not accessible. | Use dot-sourcing if necessary. |
Permission issues | Insufficient permissions to execute. | Run PowerShell as an administrator. |
By adhering to these practices and being aware of potential issues, you can effectively manage script calls and enhance your PowerShell scripting experience.
Methods to Call a PowerShell Script from Another PowerShell Script
There are several methods to invoke a PowerShell script from another script. Each method has its own use cases and advantages, depending on the requirements of the task at hand.
Using the Call Operator
The call operator (`&`) allows you to run a script within the context of the current session. This method is straightforward and provides access to variables and functions defined in the calling script.
powershell
& “C:\Path\To\YourScript.ps1”
- Pros:
- Retains the current session context.
- Can pass parameters directly.
- Cons:
- Limited to scripts that reside within the same environment.
Using the Start-Process Cmdlet
`Start-Process` can be used to initiate a new PowerShell process to execute another script. This is beneficial when you want to run the script in a separate session.
powershell
Start-Process powershell.exe -ArgumentList “-File C:\Path\To\YourScript.ps1”
- Pros:
- Runs independently of the calling script’s context.
- Can be used to run scripts with different user permissions.
- Cons:
- Does not allow access to variables or functions from the calling script.
Dot Sourcing a Script
Dot sourcing a script allows you to run a script in the current scope, making its functions and variables available in the calling script.
powershell
. “C:\Path\To\YourScript.ps1”
- Pros:
- Makes functions and variables available for immediate use.
- Simple and effective for utility scripts.
- Cons:
- Can lead to variable conflicts if not managed properly.
Passing Parameters to Scripts
When calling a script, parameters can be passed to customize its behavior. Here’s how to do that with both the call operator and dot sourcing.
Using Call Operator:
powershell
& “C:\Path\To\YourScript.ps1” -Param1 “Value1” -Param2 “Value2”
Using Dot Sourcing:
powershell
. “C:\Path\To\YourScript.ps1” -Param1 “Value1” -Param2 “Value2”
- Ensure that the called script is designed to accept these parameters.
Handling Return Values
To capture the output from the script being called, you can assign it to a variable:
powershell
$result = & “C:\Path\To\YourScript.ps1” -Param “Value”
This allows further manipulation of the returned data within the calling script.
Method | Session Context | Can Pass Parameters | Returns Output |
---|---|---|---|
Call Operator (`&`) | Yes | Yes | Yes |
Start-Process | No | Yes | No |
Dot Sourcing | Yes | Yes | Yes |
Best Practices
- Use meaningful script names: Helps in identifying the purpose of scripts.
- Modularize scripts: Break down complex processes into smaller scripts that can be reused.
- Document parameters and expected output: Ensures clarity for future reference and team collaboration.
By employing these methods and practices, you can efficiently manage script execution in PowerShell, enhancing the maintainability and functionality of your automation tasks.
Expert Insights on Calling a PowerShell Script from Another PowerShell Script
Jessica Lane (Senior Systems Administrator, Tech Solutions Inc.). “When calling a PowerShell script from another script, it is crucial to ensure that the execution policy allows for script execution. Using the `&` operator is a straightforward method to invoke the script, but understanding the context and scope of variables between scripts can prevent unexpected behavior.”
Michael Chen (DevOps Engineer, Cloud Innovations). “Incorporating modular scripts can significantly enhance code reusability and maintainability. When you call a script from another, consider using parameters to pass data effectively. This practice not only streamlines operations but also minimizes hard-coded values that could lead to errors.”
Sarah Thompson (PowerShell Automation Specialist, IT Pro Magazine). “It is essential to handle errors gracefully when executing one script from another. Utilizing try-catch blocks can help manage exceptions and provide meaningful feedback, ensuring that the calling script can respond appropriately to any issues that arise during execution.”
Frequently Asked Questions (FAQs)
How can I call a PowerShell script from another PowerShell script?
You can call a PowerShell script from another script by using the `&` (call operator) followed by the path to the script. For example: `& “C:\Path\To\YourScript.ps1″`.
What happens if the called script has parameters?
If the called script requires parameters, you can pass them after the script path. For example: `& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2`.
Can I use relative paths when calling a script?
Yes, you can use relative paths. The path is relative to the current working directory of the script that is executing the call.
Is there a way to capture the output of the called script?
Yes, you can capture the output by assigning it to a variable. For example: `$output = & “C:\Path\To\YourScript.ps1″`.
What are the implications of using `.` (dot sourcing) instead of calling a script?
Dot sourcing allows the called script to run in the same scope as the calling script, meaning any variables or functions defined in the called script will be accessible in the calling script. Use `.` followed by the script path, like `. “C:\Path\To\YourScript.ps1″`.
Can I call a script located on a remote machine?
Yes, you can call a script on a remote machine using PowerShell remoting. Use the `Invoke-Command` cmdlet with the `-ScriptBlock` parameter to execute the script remotely.
In summary, calling a PowerShell script from another PowerShell script is a straightforward yet powerful technique that enhances modularity and reusability in scripting. This practice allows developers to break down complex tasks into smaller, manageable scripts, which can be executed independently or as part of a larger workflow. By leveraging the `&` operator or the `Invoke-Expression` cmdlet, users can efficiently invoke scripts, passing parameters as needed to customize their execution.
Moreover, understanding the scope and context in which scripts are called is crucial. When invoking a script, it is important to consider the execution policy settings and the environment in which the scripts operate. This ensures that scripts run smoothly without encountering permission issues. Additionally, error handling and output management should be implemented to capture any issues that arise during execution, thereby improving the robustness of the scripts.
Key takeaways from this discussion include the importance of structuring PowerShell scripts for clarity and maintainability. By organizing scripts into functions or modules, users can create a library of reusable components that simplify the development process. Furthermore, adopting best practices for parameter passing and output handling will lead to more efficient and effective scripts, ultimately enhancing productivity in automation tasks.
Author Profile
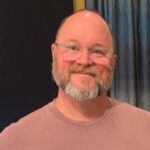
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?