How Can You Call a PowerShell Script from a Batch File?
In the world of Windows automation, the ability to seamlessly integrate different scripting languages can significantly enhance productivity and streamline workflows. One powerful combination is using batch files and PowerShell scripts together. While batch files have been a staple for command-line operations for decades, PowerShell brings a modern, robust scripting environment that can handle complex tasks with ease. If you’ve ever found yourself needing to leverage the strengths of both, you’re in the right place. This article will guide you through the process of calling a PowerShell script from a batch file, unlocking a new level of efficiency in your scripting endeavors.
Understanding how to call a PowerShell script from a batch file opens up a world of possibilities for automation. Batch files are excellent for executing a series of commands quickly, but they often fall short when it comes to more advanced functionalities. PowerShell, on the other hand, is designed for system administration and automation, offering a rich set of features that can be harnessed within a simple batch file. This synergy allows users to create scripts that are not only powerful but also easy to manage and execute.
As we delve deeper into this topic, we’ll explore the syntax and methods for invoking PowerShell scripts from batch files, ensuring you can leverage the full capabilities of both scripting environments. Whether you’re an
Using the Call Command
To execute a PowerShell script from a batch file, one of the most straightforward methods is to use the `call` command. This command allows the batch file to call another batch file or script and return to the current script upon completion. In the case of PowerShell, it can be utilized as follows:
“`batch
call powershell.exe -ExecutionPolicy Bypass -File “C:\Path\To\Your\Script.ps1”
“`
In this command:
- `powershell.exe` is the executable that launches PowerShell.
- `-ExecutionPolicy Bypass` allows the script to run without being blocked by execution policies set in PowerShell.
- `-File` specifies the path to the PowerShell script.
It’s important to ensure that the path to the PowerShell script is correct to avoid errors during execution.
Using the Start Command
Another method to call a PowerShell script is by using the `start` command. This command opens a new command window and runs the script independently. The syntax is:
“`batch
start powershell.exe -ExecutionPolicy Bypass -File “C:\Path\To\Your\Script.ps1”
“`
Using `start` has its benefits, such as allowing the batch file to continue executing without waiting for the PowerShell script to complete. However, this means that any output from the PowerShell script will not be captured back in the batch file.
Passing Arguments to PowerShell Scripts
If your PowerShell script requires parameters, you can easily pass them from the batch file. Here’s an example of how to do that:
“`batch
call powershell.exe -ExecutionPolicy Bypass -File “C:\Path\To\Your\Script.ps1” -Param1 “Value1” -Param2 “Value2”
“`
In this example, `-Param1` and `-Param2` are parameters that the PowerShell script can accept. It’s crucial to ensure that the parameters are defined within the script to handle the incoming values correctly.
Example Batch File
Here is a complete example of a batch file that calls a PowerShell script with parameters:
“`batch
@echo off
echo Starting PowerShell Script…
call powershell.exe -ExecutionPolicy Bypass -File “C:\Path\To\Your\Script.ps1” -Param1 “Value1” -Param2 “Value2”
echo PowerShell Script Completed.
“`
This batch file will execute the PowerShell script and display messages indicating the start and completion of the process.
Common Issues and Troubleshooting
When calling PowerShell scripts from a batch file, users may encounter several common issues. Below is a table summarizing these issues and their solutions:
Issue | Solution |
---|---|
Script not found | Verify the script path is correct. |
Execution policy prevents running scripts | Use `-ExecutionPolicy Bypass` to override the policy. |
Parameters not recognized | Ensure parameters are correctly defined in the script. |
PowerShell window closes immediately | Add a pause command at the end of the script to keep the window open. |
By following the outlined methods and troubleshooting tips, users can effectively call PowerShell scripts from batch files, enhancing automation and script execution capabilities.
Calling a PowerShell Script from a Batch File
To execute a PowerShell script from a batch file, you can utilize the `powershell.exe` command. This allows seamless integration between batch scripts and PowerShell, providing access to advanced scripting capabilities.
Basic Syntax
The general syntax for calling a PowerShell script from a batch file is as follows:
“`batch
powershell.exe -ExecutionPolicy Bypass -File “Path\To\YourScript.ps1”
“`
- ExecutionPolicy Bypass: This flag allows the script to run without being blocked by the execution policy settings.
- -File: This parameter specifies the path to the PowerShell script you wish to execute.
Example Batch File
Here’s an example of a simple batch file that calls a PowerShell script:
“`batch
@echo off
echo Starting PowerShell script…
powershell.exe -ExecutionPolicy Bypass -File “C:\Scripts\MyScript.ps1”
echo PowerShell script completed.
pause
“`
- @echo off: Prevents the commands from being displayed in the command prompt.
- pause: Keeps the command prompt open until a key is pressed, allowing the user to see output messages.
Passing Parameters to PowerShell Scripts
You can also pass parameters from the batch file to the PowerShell script. Here is the modified command:
“`batch
powershell.exe -ExecutionPolicy Bypass -File “C:\Scripts\MyScript.ps1” -Param1 Value1 -Param2 Value2
“`
In your PowerShell script, you would define the parameters at the beginning:
“`powershell
param (
[string]$Param1,
[string]$Param2
)
“`
Error Handling
When calling PowerShell scripts, it’s crucial to handle potential errors gracefully. Use the following approach in your batch file:
“`batch
@echo off
echo Starting PowerShell script…
powershell.exe -ExecutionPolicy Bypass -File “C:\Scripts\MyScript.ps1”
if %ERRORLEVEL% neq 0 (
echo An error occurred while executing the PowerShell script.
) else (
echo PowerShell script completed successfully.
)
pause
“`
- %ERRORLEVEL%: This variable captures the exit code of the last executed command. A non-zero value typically indicates an error.
Using PowerShell Integrated Scripting Environment (ISE)
If you prefer to test your script interactively, consider using PowerShell ISE. To call a PowerShell script from within ISE, you can execute the following command in the console:
“`powershell
& “C:\Scripts\MyScript.ps1”
“`
This allows for direct execution and debugging of your script before incorporating it into a batch file.
By integrating PowerShell scripts into batch files, you can enhance automation workflows, leverage advanced scripting features, and maintain cleaner code. Ensure proper syntax and error handling for a seamless experience.
Expert Insights on Integrating PowerShell Scripts with Batch Files
Jessica Lin (Senior Systems Administrator, Tech Solutions Inc.). “Integrating PowerShell scripts within batch files allows for enhanced automation and flexibility in Windows environments. It is crucial to ensure that the PowerShell execution policy is set correctly to avoid permission issues when invoking scripts.”
Michael Chen (DevOps Engineer, Cloud Innovations Group). “Using a batch file to call a PowerShell script can streamline processes and reduce manual intervention. I recommend using the ‘Start-Process’ cmdlet for better control over script execution and to capture any output or errors effectively.”
Rachel Adams (IT Consultant, Future Tech Advisors). “When calling PowerShell scripts from batch files, it is essential to handle paths correctly, especially when dealing with spaces. Enclosing paths in quotes and using the ‘Call’ command can prevent unexpected errors during execution.”
Frequently Asked Questions (FAQs)
How can I call a PowerShell script from a batch file?
You can call a PowerShell script from a batch file by using the `powershell.exe` command followed by the `-File` parameter. For example:
“`batch
powershell.exe -File “C:\path\to\your\script.ps1”
“`
What if my PowerShell script requires parameters?
You can pass parameters to your PowerShell script by including them after the script path in the batch file. For example:
“`batch
powershell.exe -File “C:\path\to\your\script.ps1” -Param1 Value1 -Param2 Value2
“`
Do I need to set the execution policy to run a PowerShell script from a batch file?
Yes, if the execution policy is set to restrict script execution, you may need to adjust it. You can temporarily bypass the policy by adding `-ExecutionPolicy Bypass` to your command:
“`batch
powershell.exe -ExecutionPolicy Bypass -File “C:\path\to\your\script.ps1”
“`
Can I run a PowerShell script in the background from a batch file?
Yes, you can run a PowerShell script in the background by using the `Start-Process` cmdlet within the PowerShell command. For example:
“`batch
powershell.exe -Command “Start-Process powershell.exe -ArgumentList ‘-File C:\path\to\your\script.ps1’ -NoNewWindow”
“`
What should I do if the PowerShell script does not execute properly from the batch file?
Check for errors in your script by running it directly in PowerShell. Ensure the file path is correct and that any required permissions are granted. You may also want to check the batch file for syntax errors.
Is it possible to capture the output of a PowerShell script when called from a batch file?
Yes, you can capture the output by redirecting it to a text file using the `>` operator. For example:
“`batch
powershell.exe -File “C:\path\to\your\script.ps1” > output.txt
“`
In summary, calling a PowerShell script from a batch file is a straightforward process that can significantly enhance the functionality of your scripts. By leveraging the capabilities of both batch files and PowerShell, users can create more complex automation tasks that utilize the strengths of each scripting language. The integration of PowerShell within batch files allows for advanced operations, such as handling objects and utilizing .NET framework features, which are not natively available in traditional batch scripting.
One of the key insights is the importance of understanding the syntax and parameters required to execute PowerShell scripts from a batch file. The command `powershell -ExecutionPolicy Bypass -File “path\to\script.ps1″` is a common method that ensures the script runs without being blocked by execution policies. Additionally, users should be aware of the potential need to adjust execution policies on their systems to allow scripts to run smoothly, which can be done temporarily or permanently based on security requirements.
Furthermore, error handling and output management are critical when combining these two scripting environments. Users should implement mechanisms to capture any errors that may arise during the execution of the PowerShell script and handle them appropriately within the batch file. This ensures that the batch process remains robust and can provide feedback or logs for
Author Profile
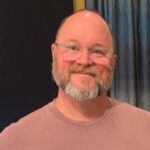
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?