Why Am I Seeing AxiosError: Request Failed With Status Code 500?
In the world of web development, encountering errors is an inevitable part of the journey. One particularly frustrating error that developers often face is the dreaded AxiosError: Request failed with status code 500. This cryptic message can send even the most seasoned programmers into a tailspin, leaving them to sift through layers of code and server responses in search of the root cause. Understanding this error is crucial, not just for troubleshooting but also for enhancing your overall development skills and improving the user experience of your applications.
At its core, a 500 status code signifies an internal server error, indicating that something has gone awry on the server side while processing a request. This error can stem from a variety of issues, ranging from server misconfigurations to unhandled exceptions in the code. For developers using Axios, a popular promise-based HTTP client, the occurrence of this error can be particularly perplexing, as it often disrupts the flow of data between the client and server. As we delve deeper into the intricacies of this issue, we will explore common causes, effective troubleshooting strategies, and best practices to mitigate such errors in future projects.
Navigating the complexities of AxiosError: Request failed with status code 500 requires a blend of analytical thinking and practical problem-solving skills.
Error Explanation
Axios is a popular JavaScript library used for making HTTP requests. When you encounter the error message “AxiosError: Request failed with status code 500,” it indicates that the server encountered an unexpected condition that prevented it from fulfilling the request. A status code of 500 is a generic error response, meaning that there is a problem on the server side rather than with the client’s request.
Common causes of a 500 error may include:
- Server Misconfiguration: Issues in server settings or misconfigured files can lead to errors.
- Faulty Application Logic: Bugs in the application code, such as unhandled exceptions or errors in the logic flow.
- Database Issues: Problems connecting to or querying the database could trigger this error.
- Server Overload: High traffic or resource exhaustion can cause the server to fail to process requests.
Debugging Steps
To effectively troubleshoot a 500 error when using Axios, follow these steps:
- Check Server Logs: Inspect the server logs for detailed error messages that can pinpoint the issue.
- Examine the API Endpoint: Verify that the endpoint you are trying to reach is correct and operational.
- Validate Request Data: Ensure that any data being sent in the request is valid and adheres to the API’s requirements.
- Test with Other Tools: Use tools like Postman or cURL to see if the error persists outside of your application.
- Review Code Changes: If the error started appearing after recent changes, review those changes for potential issues.
Best Practices
When dealing with Axios and handling potential server errors, consider implementing the following best practices:
– **Error Handling**: Always include error handling in your Axios requests to manage unexpected situations gracefully. For example:
“`javascript
axios.get(‘/api/data’)
.then(response => {
// handle success
})
.catch(error => {
if (error.response) {
// The request was made and the server responded with a status code
console.error(“Error Status:”, error.response.status);
} else {
// The request was made but no response was received
console.error(“Error Message:”, error.message);
}
});
“`
- Logging: Implement logging for both client-side and server-side errors to aid in future debugging.
- Retry Mechanism: Consider adding a retry mechanism for transient errors, which can sometimes resolve issues without user intervention.
Common Status Codes
Understanding common HTTP status codes can aid in diagnosing issues quickly. The following table outlines some relevant status codes that may be encountered alongside a 500 error:
Status Code | Description |
---|---|
400 | Bad Request – The server could not understand the request due to invalid syntax. |
401 | Unauthorized – The request requires user authentication. |
403 | Forbidden – The server understood the request, but refuses to authorize it. |
404 | Not Found – The server can’t find the requested resource. |
500 | Internal Server Error – A generic error message when the server fails to fulfill a valid request. |
By thoroughly understanding these aspects of the error, developers can effectively resolve issues and improve the resilience of their applications.
Understanding AxiosError
AxiosError is an error object that is thrown when an Axios request fails. It provides valuable information about the nature of the failure, including the request configuration, the response received, and the error message. When you encounter the error “Request failed with status code 500,” it indicates that the server encountered an internal error while processing the request.
Key components of AxiosError include:
- message: A string that describes the error.
- config: The configuration object used for the request.
- response: The response object returned by the server, which contains:
- status: The HTTP status code (e.g., 500).
- data: The data returned from the server, if any.
- headers: The headers returned from the server.
Common Causes of Status Code 500
A 500 Internal Server Error can arise from several issues on the server side. Some common causes include:
- Application Bugs: Errors in the server-side code that prevent it from processing requests correctly.
- Database Connection Issues: Problems connecting to the database, such as timeouts or misconfigurations.
- Unhandled Exceptions: Exceptions that are not properly caught and handled in the application logic.
- Server Configuration Errors: Issues with the server environment or configuration files that lead to a failure in processing requests.
- Resource Exhaustion: Running out of resources (e.g., memory, CPU) can cause the server to fail.
Troubleshooting Steps
When encountering a 500 error with Axios, follow these troubleshooting steps to diagnose and potentially resolve the issue:
- Check Server Logs: Review server logs to identify error messages and stack traces that provide insight into the root cause.
- Validate API Endpoints: Ensure that the correct endpoint is being called, and that it is functioning as expected.
- Test with Tools: Use tools like Postman or curl to manually test the API endpoints and observe the responses.
- Inspect Request Payload: Validate the request data being sent to the server. Ensure it meets the API requirements.
- Monitor Server Resources: Check server health metrics to ensure adequate resources are available.
Handling AxiosError in Code
Proper error handling in your Axios requests can help manage and respond to errors effectively. Use the following structure to catch and handle errors:
“`javascript
axios.get(‘/api/endpoint’)
.then(response => {
// Handle successful response
})
.catch(error => {
if (error.response) {
// The request was made and the server responded with a status code
console.error(‘Error Status:’, error.response.status);
console.error(‘Error Data:’, error.response.data);
} else if (error.request) {
// The request was made but no response was received
console.error(‘Request Error:’, error.request);
} else {
// Something happened in setting up the request
console.error(‘Error Message:’, error.message);
}
});
“`
This structure allows for granular handling of different error scenarios, enabling you to provide better feedback to users or log issues for further investigation.
Preventing Internal Server Errors
To minimize the occurrence of 500 Internal Server Errors, consider the following best practices:
- Implement Comprehensive Logging: Ensure that all parts of your application log errors and critical events for easier debugging.
- Use Try/Catch Blocks: Surround code with try/catch to manage exceptions effectively.
- API Versioning: Implement version control for your API to manage changes without breaking existing functionality.
- Load Testing: Regularly perform load testing to identify resource limitations and optimize performance before issues arise.
- Monitor Dependencies: Keep track of third-party services and libraries to ensure they are up-to-date and functioning correctly.
By adhering to these practices, you can enhance the stability and reliability of your applications while minimizing the risk of encountering 500 errors.
Understanding Axios Errors: Insights from Software Development Experts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A 500 status code indicates a server-side error, which suggests that the issue lies not with the Axios request itself but rather with the server’s ability to process the request. It’s crucial to analyze server logs to diagnose the root cause of the failure.”
Michael Chen (Lead Backend Developer, Cloud Solutions Group). “When encountering an Axios error with a status code of 500, developers should implement robust error handling in their code. This includes logging the error details and providing fallback mechanisms to enhance user experience during server outages.”
Sarah Thompson (Full Stack Developer, Agile Devs). “To effectively troubleshoot a 500 error in Axios, it’s important to isolate the request parameters and headers. Often, malformed data or unexpected input can trigger server errors, so validating the request payload is essential.”
Frequently Asked Questions (FAQs)
What does “AxiosError: Request failed with status code 500” mean?
This error indicates that the server encountered an unexpected condition that prevented it from fulfilling the request. A status code of 500 signifies an internal server error, which typically points to issues on the server side rather than the client side.
What are common causes of a 500 status code?
Common causes include server misconfigurations, unhandled exceptions in server-side code, database connection failures, or issues with third-party services the server relies on. Debugging server logs can help identify the specific issue.
How can I troubleshoot a 500 status code error?
To troubleshoot, start by checking server logs for error messages. Review recent code changes, ensure all dependencies are functioning correctly, and verify that the server has sufficient resources. Testing with different inputs may also reveal underlying issues.
Is there a way to handle this error in my Axios request?
Yes, you can handle this error using a try-catch block or by using `.catch()` in your Axios request. This allows you to gracefully manage the error and provide user feedback or retry logic as needed.
Can a 500 error be caused by client-side issues?
While a 500 error is primarily a server-side issue, certain client-side issues—such as malformed requests, incorrect headers, or payloads—can inadvertently trigger server errors. Ensuring the request is correctly formatted can help mitigate this.
What should I do if I consistently receive a 500 error from a specific API?
If you consistently receive a 500 error from a specific API, contact the API provider for support. Provide them with details of your request and any error messages. They may be able to offer insights or confirm if there are ongoing issues with their service.
The Axios error indicating a request failure with status code 500 signifies that the server encountered an unexpected condition that prevented it from fulfilling the request. This status code is categorized as a server-side error, implying that the issue lies not with the client or the request itself but rather with the server’s ability to process the request. Understanding the context of this error is crucial for developers and users alike, as it can stem from various underlying issues such as server misconfigurations, application bugs, or resource limitations.
When troubleshooting a 500 error, it is essential to analyze server logs and application code to identify the root cause of the failure. Implementing proper error handling and logging mechanisms can significantly aid in diagnosing these issues. Additionally, developers should ensure that the server environment is correctly configured and that all dependencies are up to date. Testing the server’s response to various requests can also help in pinpointing the specific conditions that trigger the error.
In summary, while a 500 status code indicates a serious problem on the server side, it serves as a critical reminder of the importance of robust server management and error handling practices. By proactively addressing potential issues and maintaining clear communication with users regarding server status, developers can enhance the reliability of their applications and improve user
Author Profile
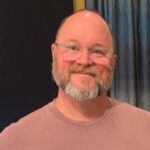
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?