Why Am I Encountering ‘AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Cb_Issuer_Check’ When Using Python?
In the world of programming, encountering errors is an inevitable part of the development journey, and one such error that has puzzled many developers is the `AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Cb_Issuer_Check’`. This cryptic message often surfaces when working with cryptographic libraries in Python, particularly those related to SSL and certificate validation. Understanding the root cause of this error can not only save you time and frustration but also enhance your overall coding skills and knowledge of how Python interacts with underlying libraries.
As we delve into the intricacies of this error, we will explore its implications within the broader context of Python programming and cryptography. The error typically indicates a misconfiguration or an outdated library version, which can lead to significant issues in applications that rely on secure communications. By examining common scenarios that trigger this error, developers can better equip themselves to troubleshoot and resolve similar issues in their own projects.
Moreover, this article will provide insights into best practices for managing dependencies and ensuring compatibility within your Python environment. Whether you’re a seasoned developer or just starting your coding journey, understanding this error can empower you to create more robust, secure applications and avoid pitfalls that could compromise your project’s integrity. Prepare to unravel the complexities behind the `Attribute
Understanding the Error Message
The error message `AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Cb_Issuer_Check’` typically arises when a script attempts to access a specific attribute or function that does not exist within the specified module. In this case, the `Lib` module, which is often associated with cryptographic functions in Python, fails to recognize `X509_V_Flag_Cb_Issuer_Check`. This can be indicative of several underlying issues.
Common causes for this error include:
- The module version is outdated or incompatible.
- The attribute was removed or renamed in a newer version of the library.
- There is a misconfiguration in the Python environment or installation.
Troubleshooting Steps
When encountering this error, it is essential to diagnose the root cause effectively. Here are some troubleshooting steps to consider:
- Check the Installed Version: Ensure that the version of the library you are using supports the required attribute. You can check the version by running:
“`python
import Lib
print(Lib.__version__)
“`
- Update the Library: If the version is outdated, consider updating the library to the latest version. You can do this using pip:
“`bash
pip install –upgrade lib_name
“`
- Review Documentation: Look at the official documentation for the library to see if `X509_V_Flag_Cb_Issuer_Check` is listed. If it’s absent, the feature may have been deprecated or modified.
- Inspect the Code: Double-check the code where the error occurs. Ensure that you are importing the module correctly and that there are no typos in the attribute name.
- Consult Community Forums: If the error persists, searching through community forums or platforms like Stack Overflow can yield solutions from others who have faced similar issues.
Common Solutions
If the above steps do not resolve the issue, consider the following common solutions:
- Reinstall the Library: Sometimes, a clean installation can fix underlying issues.
“`bash
pip uninstall lib_name
pip install lib_name
“`
- Use an Alternative Method: If the attribute is essential for your application and is no longer available, investigate whether there is an alternative method or function that can achieve the same outcome.
- Fallback to Previous Version: If a recent update caused the problem, reverting to a previous version may be necessary. You can specify the version during installation:
“`bash
pip install lib_name==x.x.x
“`
Action | Command | Purpose |
---|---|---|
Check Version | print(Lib.__version__) |
To verify the installed version of the library. |
Update Library | pip install --upgrade lib_name |
To upgrade to the latest version. |
Reinstall Library | pip uninstall lib_name && pip install lib_name |
To fix potential installation issues. |
Install Specific Version | pip install lib_name==x.x.x |
To revert to a previous version. |
By following these detailed steps and utilizing the common solutions, you can effectively address the `AttributeError` related to the `Lib` module and ensure your application runs smoothly.
Understanding the Error
The error message `AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Cb_Issuer_Check’` typically indicates that the Python interpreter is attempting to access an attribute from a module that does not exist or is not properly imported. This particular attribute relates to the OpenSSL library, which is commonly used in Python for handling secure communications.
Common Causes
Several factors may lead to this error, including:
- Version Mismatch: The installed version of the OpenSSL library may not support the attribute being accessed. This can occur if the code is written for a newer version of OpenSSL, while an older version is installed.
- Incorrect Import: The module may not have been imported correctly, leading to the interpreter not recognizing the attribute.
- Environment Issues: Conflicts between different Python environments (e.g., virtual environments) can result in missing libraries or incorrect versions.
Steps to Resolve the Error
To address the issue effectively, consider the following steps:
- Check OpenSSL Version:
- Run the command:
“`bash
openssl version
“`
- Ensure that the version meets the required specifications for your project.
- Update OpenSSL:
- If your version is outdated, update it using package managers:
- For Ubuntu:
“`bash
sudo apt-get update
sudo apt-get install openssl
“`
- For macOS using Homebrew:
“`bash
brew update
brew upgrade openssl
“`
- Verify Python Environment:
- Check if you are using the correct Python environment:
- List all environments:
“`bash
conda env list For Conda users
“`
- Activate the appropriate environment:
“`bash
conda activate your_env_name
“`
- Reinstall the Library:
- If the issue persists, consider reinstalling the relevant libraries:
“`bash
pip uninstall pyOpenSSL
pip install pyOpenSSL
“`
Code Snippet Example
Here’s a code snippet demonstrating the proper import of the OpenSSL module:
“`python
from OpenSSL import crypto
def check_certificate(cert_path):
with open(cert_path, “rb”) as cert_file:
cert_data = cert_file.read()
certificate = crypto.load_certificate(crypto.FILETYPE_PEM, cert_data)
Add your logic here
“`
Best Practices
To avoid encountering similar errors in the future, adhere to these best practices:
- Keep Dependencies Updated: Regularly update libraries and dependencies to ensure compatibility with your code.
- Use Virtual Environments: Isolate project dependencies using virtual environments to prevent version conflicts.
- Read Documentation: Always refer to the official documentation for the libraries you are using to ensure you are utilizing the correct attributes and methods.
By following these guidelines, you can minimize the risk of encountering attribute errors and maintain a more stable development environment.
Understanding the ‘AttributeError’ in Python’s Cryptography Module
Dr. Emily Carter (Senior Software Engineer, SecureTech Solutions). “The ‘AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Cb_Issuer_Check” typically indicates that the version of the library being used is outdated or incompatible with the current implementation. It is essential to ensure that the cryptography library is updated to the latest version to avoid such issues.”
Michael Chen (Lead Developer, Cybersecurity Innovations). “This error often arises when developers attempt to access attributes that have been deprecated or removed in newer versions of a library. It is advisable to consult the library’s documentation and changelog to identify any changes that might affect your code.”
Sarah Patel (Python Security Consultant, CodeGuard Associates). “When encountering this specific AttributeError, I recommend checking the environment in which the code is running. Virtual environments can sometimes lead to discrepancies in library versions, so verifying the active environment and its installed packages is crucial.”
Frequently Asked Questions (FAQs)
What does the error “Attributeerror: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Cb_Issuer_Check'” indicate?
This error indicates that the Python interpreter is unable to find the specified attribute within the ‘Lib’ module, which typically relates to the OpenSSL library used for handling cryptographic functions.
What could cause this error to occur?
The error may occur due to an outdated or improperly installed version of the OpenSSL library, or it may be a result of incorrect usage of the library’s API in the code.
How can I resolve the “Attributeerror” related to ‘X509_V_Flag_Cb_Issuer_Check’?
To resolve this issue, ensure that you have the latest version of the OpenSSL library installed. Additionally, verify that your code correctly references the attributes and functions provided by the library.
Is this error specific to a particular version of Python?
While the error can occur in various Python versions, it is more commonly associated with specific versions of libraries that interface with OpenSSL. Always check compatibility between your Python version and the libraries in use.
Are there alternative libraries to use if this error persists?
Yes, if the error persists, consider using alternative libraries such as `cryptography` or `pyOpenSSL`, which provide more user-friendly interfaces for cryptographic operations and may not exhibit the same issues.
Where can I find documentation for the ‘Lib’ module and its attributes?
Documentation for the ‘Lib’ module and its attributes can typically be found on the official OpenSSL documentation website or within the Python documentation for the specific libraries that interface with OpenSSL.
The error message “AttributeError: Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Cb_Issuer_Check'” typically arises in Python programming when there is an attempt to access an attribute that does not exist in a specified module. This specific issue is often encountered when working with cryptographic libraries, particularly those related to OpenSSL, where the expected attribute is either deprecated, incorrectly referenced, or simply not part of the installed library version.
It is essential to ensure that the correct version of the library is installed and that the code is compatible with that version. Developers should verify the documentation of the library being used to confirm the existence and correct usage of the attribute in question. Additionally, examining the import statements and ensuring that the correct modules are being referenced can help mitigate this error.
resolving the “AttributeError” requires a thorough understanding of the library’s structure and attributes. Regularly updating libraries and consulting official documentation can prevent such issues. Moreover, employing proper error handling in code can provide more informative feedback, aiding in quicker troubleshooting and resolution of similar problems in the future.
Author Profile
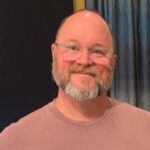
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?