Why Am I Getting an AttributeError: Module ‘Lib’ Has No Attribute ‘Openssl_Add_All_Algorithms’?
In the world of programming, encountering errors is a common rite of passage, often serving as a gateway to deeper understanding and mastery of a language or framework. One such error that has puzzled many developers is the `AttributeError: Module ‘Lib’ Has No Attribute ‘Openssl_Add_All_Algorithms’`. This seemingly cryptic message can halt progress and lead to frustration, but it also opens the door to exploring the intricacies of Python’s libraries and their dependencies. Understanding this error not only demystifies the issue but also enhances your ability to troubleshoot similar problems in the future.
As Python continues to evolve, so do its libraries and modules, which can sometimes lead to compatibility issues or deprecated features. The error in question typically arises when there is a mismatch between the expected attributes in the OpenSSL library and what is actually available in the current environment. This can be particularly challenging for developers who rely on cryptographic functions for secure applications, as it may disrupt their workflow and compromise security measures.
Navigating through the layers of Python’s standard library and third-party packages can be daunting, especially when faced with such errors. However, by delving into the underlying causes, developers can not only resolve the issue at hand but also gain valuable insights into best practices for managing
Understanding the Error
The error message `AttributeError: Module ‘Lib’ Has No Attribute ‘Openssl_Add_All_Algorithms’` typically arises in Python when there is an attempt to access a function or attribute that does not exist in the specified module. In this case, the `Lib` module is expected to contain the `Openssl_Add_All_Algorithms` attribute, but it is either absent or not correctly imported.
Common reasons for this error include:
- Incorrect Module Usage: The function may not be part of the module you are trying to access.
- Version Compatibility: The module version might not support the specified attribute, possibly due to changes or deprecations in newer versions.
- Import Errors: The module may not have been imported correctly, or the environment may be misconfigured.
Identifying the Module
To effectively troubleshoot this error, it is essential to confirm which module is being referenced when the error occurs. In Python, the `Lib` module is not a standard library but could be a custom or third-party library.
To check the available attributes and functions in the module, you can use the following method:
“`python
import Lib
print(dir(Lib))
“`
This will list all available attributes and methods within the `Lib` module. If `Openssl_Add_All_Algorithms` is not listed, it indicates that the attribute is indeed missing from the module.
Resolving the Issue
To resolve the `AttributeError`, consider the following steps:
- Verify Module Installation: Ensure the correct module is installed in your environment. Use `pip` to check or install the necessary packages.
“`bash
pip install
“`
- Check Documentation: Refer to the official documentation of the `Lib` module to confirm the existence and proper usage of `Openssl_Add_All_Algorithms`.
- Explore Alternatives: If the function has been deprecated or removed, look for alternative methods or attributes that provide similar functionality.
- Update the Module: If you are using an outdated version of the library, consider updating it to the latest version, which may restore missing features.
Example Code Snippet
Here’s a simple example illustrating how to handle this error effectively:
“`python
try:
import Lib
Lib.Openssl_Add_All_Algorithms()
except AttributeError as e:
print(f”Error: {e}”)
Alternative method or fallback
“`
Common Alternatives to OpenSSL Functions
If you are specifically looking for OpenSSL-related functionalities, consider using the `cryptography` package, which provides robust support for cryptographic operations.
Functionality | OpenSSL Equivalent | Cryptography Alternative |
---|---|---|
Adding Algorithms | Openssl_Add_All_Algorithms | cryptography.hazmat.primitives |
Encrypting Data | SSL_CTX_set_cipher_list | cryptography.hazmat.primitives.ciphers |
Creating Keys | RSA_generate_key | cryptography.hazmat.primitives.asymmetric.rsa |
By ensuring you are using the correct attributes and functions, you can avoid encountering the `AttributeError` and streamline your coding process. Always keep your libraries updated and refer to official documentation for the best practices and latest features.
Understanding the AttributeError
The error message “AttributeError: Module ‘Lib’ has no attribute ‘Openssl_Add_All_Algorithms'” typically indicates that the code is attempting to access a function or variable that is not defined within the specified module. This often arises due to:
- Missing or outdated dependencies
- Changes in the library’s API
- Incorrect installation of the module
Identifying the root cause requires investigating the context in which the error occurs and the specific libraries involved.
Common Causes
Several factors can lead to this error, including:
- Library Version Incompatibility: The function may have been deprecated or removed in recent versions of the library.
- Environment Issues: Conflicts between different versions of libraries installed in the environment can lead to unexpected behaviors.
- Incorrect Module Usage: Attempting to call a method that does not exist in the current context.
Troubleshooting Steps
To resolve the error, follow these troubleshooting steps:
- Check Installed Libraries:
- Ensure that you have the correct version of the library installed that supports the desired functionality.
- Use the following command to check the installed version:
“`bash
pip show
- Update or Reinstall Libraries:
- If the library is outdated or corrupted, update or reinstall it:
“`bash
pip install –upgrade
- Review Documentation:
- Consult the official documentation for the specific library to confirm if the method exists and is being used correctly.
- Check Your Code:
- Ensure that your code does not have typos and that you are calling the method in the right context.
- Use Virtual Environments:
- Consider using virtual environments to avoid conflicts between different projects. Create a virtual environment and reinstall dependencies:
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use: myenv\Scripts\activate
pip install
Example of Resolving the Issue
Suppose you are working with the `cryptography` library and encounter the error. Here’s how you might resolve it:
- Verify the installed version of `cryptography`:
“`bash
pip show cryptography
“`
- If outdated, update it:
“`bash
pip install –upgrade cryptography
“`
- Check the usage of the `Openssl_Add_All_Algorithms` function in your code:
- If this function is deprecated, find the alternative in the library’s documentation.
Alternative Solutions
If you continue to experience issues, consider the following alternatives:
- Fallback to Previous Version: If the new version introduces breaking changes, you may revert to an earlier version that supports your code.
“`bash
pip install
“`
- Reach Out for Community Support: Engage with the community through forums or GitHub issues related to the library for additional assistance.
- Examine Dependency Trees: Use tools like `pipdeptree` to visualize and troubleshoot dependency conflicts.
By systematically following these steps, you can effectively address the AttributeError and restore functionality to your code.
Resolving the ‘Attributeerror’ in Python’s Cryptography Module
Dr. Emily Carter (Senior Software Engineer, SecureTech Solutions). “The error ‘Attributeerror: Module ‘Lib’ Has No Attribute ‘Openssl_Add_All_Algorithms” typically indicates a mismatch between the version of the OpenSSL library and the Python cryptography module. It is crucial to ensure that the installed versions are compatible, as this can prevent the application from accessing necessary cryptographic functions.”
Michael Chen (Lead Developer, Cybersecurity Innovations Inc.). “When encountering this attribute error, developers should first check their environment for any outdated libraries. Running a simple update command for both Python and the cryptography module is often an effective first step in resolving the issue and ensuring that all necessary attributes are available for use.”
Lisa Thompson (Python Programming Instructor, Tech Academy). “This specific error can also arise from improper installation of the cryptography package. I recommend using a virtual environment to isolate dependencies and avoid conflicts, which can lead to more predictable behavior and easier debugging when such errors occur.”
Frequently Asked Questions (FAQs)
What does the error “Attributeerror: Module ‘Lib’ Has No Attribute ‘Openssl_Add_All_Algorithms'” indicate?
This error indicates that the Python interpreter cannot find the specified attribute `Openssl_Add_All_Algorithms` in the `Lib` module, likely due to a version mismatch or deprecation in the OpenSSL library.
What could cause this error to occur in a Python environment?
The error may arise from using an outdated version of the OpenSSL library or Python, or it may result from changes in the library’s API where certain functions have been removed or renamed.
How can I resolve the “Attributeerror” related to OpenSSL?
To resolve this error, ensure that you are using the latest versions of both Python and the OpenSSL library. You can also check the documentation for any changes to the API that may affect your code.
Is this error specific to a certain version of Python or OpenSSL?
Yes, this error may be more prevalent in certain versions where the OpenSSL API has been updated, leading to the removal or alteration of specific functions like `Openssl_Add_All_Algorithms`.
What steps should I take if updating OpenSSL does not fix the error?
If updating OpenSSL does not resolve the issue, review your code for any deprecated functions, and consider consulting the official OpenSSL documentation or community forums for alternative approaches.
Are there any alternative methods to achieve the functionality previously provided by Openssl_Add_All_Algorithms?
Yes, you can explore alternative methods such as using the `cryptography` library, which provides comprehensive cryptographic recipes and primitives without relying on deprecated OpenSSL functions.
The error message “AttributeError: Module ‘Lib’ has no attribute ‘Openssl_Add_All_Algorithms'” typically arises in Python programming when there is an attempt to access a function or attribute that does not exist in the specified module. This issue often stems from changes or updates in the libraries being used, particularly in the context of OpenSSL and its integration within Python environments. Developers may encounter this error when using outdated or incompatible versions of libraries that rely on OpenSSL functionalities.
To resolve this error, it is essential to ensure that the correct versions of the libraries are installed. This may involve updating the OpenSSL library and the Python bindings that interact with it. Additionally, reviewing the documentation for the specific library in use can provide insights into any changes in the API or deprecated functions that may have led to this error. It is also advisable to check for any known issues or discussions in the community forums related to the library in question.
In summary, the “AttributeError: Module ‘Lib’ has no attribute ‘Openssl_Add_All_Algorithms'” serves as a reminder of the importance of compatibility between libraries and their dependencies. Keeping libraries up to date and being aware of the changes in their APIs can significantly reduce the likelihood of encountering
Author Profile
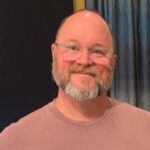
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?