Why Am I Getting ‘AttributeError: ‘Engine’ Object Has No Attribute ‘Cursor’ in My Python Code?
In the world of programming, encountering errors is an inevitable part of the development journey. Among the myriad of error messages that can pop up during coding, the `AttributeError: ‘Engine’ Object Has No Attribute ‘Cursor’` stands out as a particularly perplexing issue for developers working with databases in Python. This error often leaves programmers scratching their heads, questioning both their understanding of the libraries they are using and the underlying mechanics of their code. But fear not! Understanding the roots of this error can not only help you troubleshoot effectively but also deepen your grasp of database interactions in Python.
This article delves into the intricacies of the `AttributeError` in the context of database engines, particularly focusing on the common pitfalls that lead to this specific error. We will explore the role of the Engine object in database connectivity and how misconfigurations or misunderstandings can trigger this frustrating message. By dissecting the underlying causes, we aim to equip you with the knowledge to prevent this error from derailing your projects and to enhance your overall coding experience.
As we navigate through the nuances of this error, we will also touch on best practices for handling database connections in Python, ensuring that you not only resolve the current issue but also build a more robust foundation for your future coding
Understanding the Error
The error message `AttributeError: ‘Engine’ Object Has No Attribute ‘Cursor’` typically arises when attempting to use a cursor object from a database engine in Python, often when utilizing libraries such as SQLAlchemy. This issue indicates that the object you are trying to use does not have a method or attribute named ‘Cursor’.
In SQLAlchemy, the `Engine` object does not directly expose a `Cursor`. Instead, it uses a connection object to interact with the database. It’s essential to obtain a connection from the engine first before performing any SQL operations.
Common Causes
Several factors may lead to this error, including:
- Directly accessing the cursor: Attempting to call `engine.cursor()` instead of using a connection.
- Incorrect library usage: Mixing up libraries or methods from different database interaction libraries, such as using psycopg2’s cursor with SQLAlchemy’s engine.
- Misconfigured database connection: Inadequate setup of the database engine or connection parameters.
Correct Approach
To avoid this error, follow these steps to correctly use the SQLAlchemy `Engine` object:
- Create the Engine: Establish a connection to your database.
- Obtain a Connection: Use the `connect()` method to get a connection.
- Execute SQL Commands: Use the connection to execute your SQL statements.
Here is a sample code snippet illustrating the correct approach:
“`python
from sqlalchemy import create_engine
Step 1: Create the engine
engine = create_engine(‘postgresql://user:password@localhost/dbname’)
Step 2: Obtain a connection
with engine.connect() as connection:
Step 3: Execute SQL commands
result = connection.execute(“SELECT * FROM table_name”)
for row in result:
print(row)
“`
Best Practices
To prevent encountering this error, consider the following best practices:
- Use Context Managers: Always use context managers (with statements) when dealing with connections to ensure proper resource management.
- Refer to Documentation: Familiarize yourself with the SQLAlchemy documentation to understand the latest methods and attributes available.
- Maintain Code Clarity: Keep your code organized and well-commented to help identify where the error may arise.
Quick Reference Table
Action | Method | Notes |
---|---|---|
Create Engine | create_engine() | Initializes the connection to the database. |
Obtain Connection | engine.connect() | Fetches a connection object from the engine. |
Execute SQL | connection.execute() | Runs the SQL command using the connection. |
By following these guidelines, you can effectively manage database interactions in Python using SQLAlchemy without running into the `AttributeError: ‘Engine’ Object Has No Attribute ‘Cursor’`.
Understanding the ‘Engine’ Object in SQLAlchemy
In SQLAlchemy, the `Engine` object serves as the starting point for all interactions with the database. It is responsible for establishing connections to the database and managing the underlying connection pool. However, accessing a cursor directly from the `Engine` object is incorrect, leading to the `AttributeError` mentioned.
Key characteristics of the `Engine` object include:
- Connection Management: Automatically handles connections and connection pools.
- Dialect: Contains information about the specific database backend (e.g., PostgreSQL, MySQL).
- Execution: It does not directly provide cursor methods, which is a common misconception.
Common Causes of the AttributeError
The error typically arises from attempting to call a cursor method on an `Engine` instance, rather than on a connection object obtained from the engine. Understanding these common pitfalls can help prevent the error:
- Misuse of Engine Instance: Attempting to execute SQL commands directly on the `Engine` instead of on a connection.
- Incorrect Code Structure: Failing to obtain a connection before trying to access its cursor.
Correct Usage of Engine and Connection
To avoid the `AttributeError`, one should properly acquire a connection from the `Engine`. Here’s the correct approach:
- Creating an Engine:
“`python
from sqlalchemy import create_engine
engine = create_engine(‘sqlite:///example.db’)
“`
- Obtaining a Connection:
“`python
connection = engine.connect()
“`
- Executing Queries:
After acquiring a connection, you can execute SQL commands. For example:
“`python
result = connection.execute(“SELECT * FROM users”)
“`
- Accessing Cursor-like Behavior:
SQLAlchemy’s `ResultProxy` behaves similarly to a cursor. You can iterate over it or fetch results:
“`python
for row in result:
print(row)
“`
- Closing the Connection:
Always ensure you close the connection when done:
“`python
connection.close()
“`
Best Practices for SQLAlchemy Usage
To enhance your experience with SQLAlchemy and prevent common errors, consider the following best practices:
- Use Context Managers: Leverage Python’s `with` statement to manage connections efficiently.
“`python
with engine.connect() as connection:
result = connection.execute(“SELECT * FROM users”)
for row in result:
print(row)
“`
- Error Handling: Implement robust error handling to catch exceptions and manage database transactions effectively.
- Connection Pooling: Understand and configure connection pooling settings to optimize performance.
- Refer to Documentation: Regularly consult the [SQLAlchemy documentation](https://docs.sqlalchemy.org/) for updates and advanced features.
By adhering to these guidelines, you can minimize errors and enhance the reliability of your database interactions.
Understanding the ‘Engine’ Object AttributeError in Database Management
Dr. Emily Carter (Senior Software Engineer, Data Solutions Inc.). “The error ‘AttributeError: ‘Engine’ Object Has No Attribute ‘Cursor” typically arises when developers attempt to use a cursor method on an SQLAlchemy engine object. It’s crucial to remember that the engine itself does not create cursors directly; instead, it facilitates connections to the database, which can then be used to create a cursor.”
Michael Thompson (Database Administrator, Tech Insights). “When encountering this error, one should verify that they are using the correct method to interact with the database. Instead of calling a cursor on the engine, users should utilize the connection object obtained from the engine to execute SQL commands properly.”
Linda Chen (Python Developer, Code Academy). “This error can often confuse newcomers to SQLAlchemy. It’s essential to familiarize oneself with the framework’s architecture, particularly how the engine, session, and connection objects interact. Understanding this hierarchy will help prevent such attribute errors.”
Frequently Asked Questions (FAQs)
What does the error ‘Attributeerror: ‘Engine’ Object Has No Attribute ‘Cursor” indicate?
This error typically arises when attempting to access a cursor from an SQLAlchemy Engine object, which does not support this method directly. Instead, a connection should be established to obtain a cursor.
How can I resolve the ‘Attributeerror: ‘Engine’ Object Has No Attribute ‘Cursor” error?
To resolve this error, use the `connect()` method of the Engine object to create a connection, and then call the `cursor()` method on that connection object.
What is the correct way to execute a query using SQLAlchemy?
The correct approach involves creating a connection with the Engine, executing the query through the connection, and then closing the connection after the operation is complete.
Can I use raw SQL with SQLAlchemy without encountering this error?
Yes, you can execute raw SQL using SQLAlchemy by first establishing a connection and then using the `execute()` method on the connection object, which allows for raw SQL execution without triggering this error.
Is it necessary to manage connections manually in SQLAlchemy?
While SQLAlchemy provides connection pooling and context management, it is essential to manage connections properly in your application to avoid resource leaks and ensure efficient database interactions.
What are the best practices for handling database connections in SQLAlchemy?
Best practices include using context managers (with statements) for automatic connection handling, closing connections after use, and utilizing session objects for transaction management to ensure data integrity.
The error message “AttributeError: ‘Engine’ object has no attribute ‘Cursor'” typically arises in Python when working with SQLAlchemy, a popular SQL toolkit and Object-Relational Mapping (ORM) library. This error indicates that the code is attempting to call a method or access an attribute that does not exist on the ‘Engine’ object. In SQLAlchemy, the ‘Engine’ serves as the starting point for database interactions, but it does not directly provide a cursor object, which is a common misconception among developers transitioning from other database libraries.
To resolve this error, it is essential to understand the correct usage of SQLAlchemy’s API. Instead of trying to access a cursor directly from the ‘Engine’, users should establish a connection using the ‘connect()’ method or utilize a session object. By doing so, they can then execute SQL statements or interact with the database in a manner consistent with SQLAlchemy’s design principles. This approach not only adheres to best practices but also leverages the full capabilities of the ORM.
In summary, the “AttributeError: ‘Engine’ object has no attribute ‘Cursor'” serves as a reminder of the importance of understanding the underlying architecture of SQLAlchemy. Developers should familiarize themselves with the correct methods
Author Profile
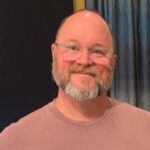
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?