How Can I Resolve the ‘AttributeError: ‘DataFrame’ Object Has No Attribute ‘Iteritems” in Python?
In the world of data manipulation and analysis, Python’s Pandas library stands as a cornerstone for data scientists and analysts alike. However, as with any powerful tool, users can encounter a variety of errors that can halt their progress and lead to frustration. One such common error is the dreaded `AttributeError: ‘DataFrame’ object has no attribute ‘iteritems’`. This error can leave even seasoned developers scratching their heads, wondering what went wrong in their code. Understanding the nuances of this error is crucial for anyone looking to harness the full potential of Pandas and streamline their data workflows.
At its core, this error arises when a user attempts to access a method or attribute that is not available on a DataFrame object. The `iteritems()` method, which is often used to iterate over the columns of a DataFrame, has its own specific context and usage. When this method is called incorrectly or on an incompatible object, it triggers an AttributeError, signaling that something is amiss. This situation not only disrupts the flow of data processing but also serves as a learning opportunity for developers to deepen their understanding of Pandas’ structure and functionality.
Navigating the intricacies of DataFrame methods and attributes is essential for effective data handling. By demystifying the `Attribute
Understanding the Error
The error `AttributeError: ‘DataFrame’ object has no attribute ‘iteritems’` typically occurs when a user attempts to call the `iteritems()` method on a pandas DataFrame. This method is intended to be used with pandas Series, not DataFrames. Understanding the structure of pandas objects is crucial for effective data manipulation.
- DataFrame: A two-dimensional labeled data structure with columns potentially of different types.
- Series: A one-dimensional labeled array capable of holding any data type.
The confusion often arises from the fact that both Series and DataFrames are part of the pandas library and share many similar functionalities. However, methods applicable to Series do not necessarily extend to DataFrames.
Correct Usage of Iteration Methods
To iterate over items in a DataFrame, you can use the following methods:
- items(): Iterates over the columns of the DataFrame, returning pairs of column labels and Series.
- iterrows(): Iterates over the rows of the DataFrame, returning index and row data as Series.
- itertuples(): Iterates over the rows of the DataFrame, returning named tuples.
For example, to iterate through columns using `items()`, you would do:
“`python
import pandas as pd
df = pd.DataFrame({
‘A’: [1, 2],
‘B’: [3, 4]
})
for column_label, column_data in df.items():
print(column_label, column_data)
“`
Common Workarounds for the Error
If you encounter the `AttributeError`, consider the following alternatives:
- Use `items()` instead of `iteritems()`:
“`python
for col, data in df.items():
print(col, data)
“`
- Iterate through rows using `iterrows()`:
“`python
for index, row in df.iterrows():
print(index, row[‘A’], row[‘B’])
“`
- Utilize `itertuples()` for better performance:
“`python
for row in df.itertuples(index=True, name=’Pandas’):
print(row.Index, row.A, row.B)
“`
Best Practices for DataFrame Iteration
When working with DataFrames, consider these best practices to maintain efficiency and clarity:
- Avoid Iteration: Whenever possible, use vectorized operations instead of looping through DataFrames. This approach is typically faster and more efficient.
- Use Built-in Functions: Functions like `apply()` can often replace the need for explicit loops.
- Profile Your Code: If iteration is necessary, use profiling tools to understand performance impacts.
Example of DataFrame Iteration
Here is a simple example of iterating over a DataFrame using `items()`:
“`python
import pandas as pd
data = {
‘Name’: [‘Alice’, ‘Bob’],
‘Age’: [25, 30]
}
df = pd.DataFrame(data)
for column, series in df.items():
print(f”Column: {column}”)
print(series)
“`
This will output the column names alongside their respective data, demonstrating the correct usage of the `items()` method.
Method | Description |
---|---|
items() | Iterates over DataFrame columns |
iterrows() | Iterates over DataFrame rows as (index, Series) pairs |
itertuples() | Iterates over DataFrame rows as named tuples |
Understanding the Error
The error message `AttributeError: ‘Dataframe’ object has no attribute ‘iteritems’` typically arises when attempting to use the `iteritems()` method on a Pandas DataFrame. This method is not available for DataFrames, as it is primarily meant for Series objects.
Common Causes
Several scenarios can lead to this error:
- Incorrect Object Type: Attempting to call `iteritems()` on a DataFrame instead of a Series.
- Typographical Errors: Misspelling the DataFrame’s method or calling a method that does not exist.
- Library Version Issues: Using an outdated or incompatible version of Pandas that does not support certain methods.
Alternative Methods
When you need to iterate over the columns or rows of a DataFrame, consider using the following alternatives:
- `items()` Method: For iterating over columns.
“`python
for column_name, column_data in dataframe.items():
print(column_name, column_data)
“`
- `iterrows()` Method: For iterating over rows as (index, Series) pairs.
“`python
for index, row in dataframe.iterrows():
print(index, row)
“`
- `itertuples()` Method: For iterating over rows as named tuples, which can be more efficient than `iterrows()`.
“`python
for row in dataframe.itertuples(index=True):
print(row.Index, row.ColumnName)
“`
Debugging Steps
To resolve the error, follow these steps:
- Check Object Type: Ensure that you are indeed working with a DataFrame.
“`python
print(type(dataframe)) Should return
“`
- Review Method Usage: Confirm that you are using the correct method for the object type.
- Update Pandas: If you suspect a version issue, update Pandas to the latest version using:
“`bash
pip install –upgrade pandas
“`
- Consult Documentation: Refer to the official Pandas documentation for the methods available for DataFrames and Series.
Example Scenarios
Here are examples illustrating the error and how to correctly iterate through a DataFrame:
Scenario | Code Snippet | Expected Outcome |
---|---|---|
Incorrect method on DataFrame | `dataframe.iteritems()` | Raises `AttributeError` |
Correct method on DataFrame | `dataframe.items()` | Iterates through columns without error |
Iterating through rows | `for index, row in dataframe.iterrows(): …` | Access each row without error |
Identifying the correct methods for iterating through a DataFrame is crucial to prevent the `AttributeError`. By using alternatives such as `items()`, `iterrows()`, or `itertuples()`, you can effectively navigate your data structures in Pandas without encountering this specific error.
Understanding the ‘AttributeError’ in DataFrame Operations
Dr. Emily Chen (Data Science Consultant, Analytics Insights). “The ‘AttributeError: ‘Dataframe’ Object Has No Attribute ‘Iteritems” typically arises when attempting to use a method that is not available for the DataFrame object in the current version of the library. It is essential to ensure that the correct method is being called and that the DataFrame is properly initialized.”
Michael Turner (Senior Software Engineer, DataTech Solutions). “This error often indicates a misunderstanding of the DataFrame’s capabilities. Users should refer to the official Pandas documentation to verify available methods and attributes, as newer versions may introduce changes that affect existing code.”
Sarah Patel (Lead Data Analyst, Insightful Analytics). “When encountering this error, it is crucial to check for typos or incorrect method calls. Additionally, ensuring that the DataFrame is not mistakenly overwritten or modified in a way that removes expected attributes can prevent such issues.”
Frequently Asked Questions (FAQs)
What does the error ‘Attributeerror: ‘Dataframe’ Object Has No Attribute ‘Iteritems” mean?
This error indicates that the DataFrame object you are working with does not have the ‘iteritems’ method available. This typically occurs when the object is not a pandas DataFrame or if there is a typo in the method name.
How can I resolve the ‘Attributeerror: ‘Dataframe’ Object Has No Attribute ‘Iteritems” error?
To resolve this error, ensure that you are using the correct method for the DataFrame. If you intended to iterate over columns, consider using ‘items()’ instead of ‘iteritems()’ as ‘iteritems()’ is deprecated in recent versions of pandas.
What is the difference between ‘iteritems()’ and ‘items()’ in pandas?
In pandas, ‘iteritems()’ was used to iterate over DataFrame columns, but it has been deprecated in favor of ‘items()’, which serves the same purpose but is more consistent with Python’s built-in dictionary methods.
Can I still use ‘iteritems()’ in older versions of pandas?
Yes, ‘iteritems()’ is still available in older versions of pandas. However, it is recommended to update your code to use ‘items()’ for future compatibility and to avoid potential issues with deprecated methods.
What should I check if I encounter this error while using a DataFrame?
Check the version of pandas you are using, verify that the object is indeed a DataFrame, and ensure that you are using the correct method for your intended operation. Additionally, review your code for any potential typos.
Is there a way to avoid this error in the future?
To avoid this error, regularly update your pandas library and familiarize yourself with the latest documentation. Always use the recommended methods for iterating over DataFrames, such as ‘items()’ or ‘iterrows()’ for row-wise iteration.
The error message “AttributeError: ‘DataFrame’ object has no attribute ‘iteritems'” typically arises in Python when using the Pandas library. This error indicates that the code is attempting to call the ‘iteritems’ method on a DataFrame object, which does not exist. Instead, the correct method to iterate over DataFrame columns is ‘items’, which is a common source of confusion among users transitioning from older versions of Pandas or from other programming languages.
Understanding the differences between the methods available in Pandas is crucial for effective data manipulation. While ‘iteritems’ was a method used in earlier versions of Pandas, it has since been deprecated in favor of ‘items’. This shift emphasizes the importance of keeping up-to-date with library documentation and understanding the evolution of the tools being used. Users should familiarize themselves with the current methods available for DataFrame objects to avoid such errors.
the ‘AttributeError’ regarding ‘iteritems’ serves as a reminder of the importance of thorough knowledge of the libraries in use. Developers should ensure they are utilizing the most current practices and methods as defined in the latest Pandas documentation. By doing so, they can enhance their coding efficiency and reduce the likelihood of encountering similar errors
Author Profile
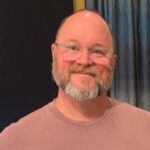
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?