Are Python Lists Mutable? Exploring Their Flexibility and Functionality
In the world of programming, understanding data structures is fundamental to mastering any language, and Python is no exception. Among its many features, lists stand out as one of the most versatile and widely used data types. But what makes Python lists particularly interesting is their mutability. This characteristic not only influences how we manipulate data but also shapes the way we approach problem-solving in our code. If you’ve ever wondered how lists can be modified after creation or how this flexibility can be harnessed in your projects, you’re in the right place. Join us as we delve into the fascinating realm of Python lists and explore their mutable nature.
Python lists are collections that can hold a variety of data types, ranging from integers and strings to even other lists. Their ability to change dynamically—adding, removing, or altering elements—sets them apart from other data structures like tuples, which remain fixed once created. This mutability allows developers to create more adaptable and efficient programs, enabling real-time data manipulation and enhancing overall functionality.
However, with great power comes great responsibility. Understanding the implications of mutability is crucial for effective programming. It can lead to unexpected behaviors, especially when passing lists between functions or when dealing with shared references. As we navigate through the intricacies of Python lists, we
Understanding the Mutability of Python Lists
Python lists are fundamentally mutable, meaning that their contents can be changed without changing the identity of the list itself. This characteristic allows for various operations that modify the list in place, such as adding, removing, or altering elements.
To elaborate on the mutability of lists, consider the following operations that can be performed on them:
- Appending elements: You can add new elements to the end of a list using the `append()` method.
- Inserting elements: The `insert()` method allows you to add an element at a specific index.
- Modifying elements: Individual elements can be changed by accessing them via their index.
- Removing elements: Elements can be removed using the `remove()` method or the `pop()` method, which also returns the removed element.
The mutability of lists makes them particularly useful for scenarios where a dynamic collection of items is required. However, this also means that caution should be exercised when using lists in concurrent programming environments, as changes to a list can lead to unintended side effects if not managed properly.
Examples of List Mutability
Here are a few examples that illustrate how lists can be modified:
“`python
Initial list
my_list = [1, 2, 3]
print(my_list) Output: [1, 2, 3]
Appending an element
my_list.append(4)
print(my_list) Output: [1, 2, 3, 4]
Inserting an element
my_list.insert(1, 5)
print(my_list) Output: [1, 5, 2, 3, 4]
Modifying an element
my_list[2] = 6
print(my_list) Output: [1, 5, 6, 3, 4]
Removing an element
my_list.remove(5)
print(my_list) Output: [1, 6, 3, 4]
“`
Comparison with Immutable Data Types
In contrast to lists, Python also has immutable data types, such as tuples and strings. Once these data types are created, their contents cannot be altered. This immutability can be beneficial in certain scenarios, particularly when data integrity is paramount.
Here is a comparison of mutable and immutable types:
Feature | Mutable (e.g., List) | Immutable (e.g., Tuple) |
---|---|---|
Modification | Can be changed | Cannot be changed |
Memory Usage | More efficient for dynamic operations | More efficient for fixed data |
Use Cases | Dynamic collections, queues, stacks | Fixed collections, function arguments |
Understanding the mutability of Python lists allows developers to leverage their capabilities effectively while being aware of the potential implications of mutable data structures in their applications.
Understanding Mutability in Python Lists
Python lists are inherently mutable, meaning that the contents of a list can be changed after it has been created. This characteristic allows for flexibility in data manipulation and is one of the reasons why lists are a widely used data structure in Python programming.
Implications of Mutability
The mutability of lists enables various operations that modify their content without the need for recreating the list. Some key implications include:
- In-place modification: Lists can be altered without generating a new list, which can lead to performance benefits.
- Dynamic sizing: Lists can grow or shrink as elements are added or removed, accommodating varying amounts of data seamlessly.
- Multiple references: Multiple variables can refer to the same list object, leading to potential side effects if one variable modifies the list.
Common List Operations Demonstrating Mutability
Several operations illustrate the mutability of lists:
- Appending elements: Using the `append()` method, elements can be added to the end of the list.
“`python
my_list = [1, 2, 3]
my_list.append(4) my_list is now [1, 2, 3, 4]
“`
- Inserting elements: The `insert()` method allows adding an element at a specific index.
“`python
my_list.insert(1, ‘a’) my_list is now [1, ‘a’, 2, 3, 4]
“`
- Modifying elements: Accessing an index allows direct modification of an element.
“`python
my_list[2] = ‘b’ my_list is now [1, ‘a’, ‘b’, 3, 4]
“`
- Removing elements: The `remove()` method can delete a specific element by value.
“`python
my_list.remove(‘a’) my_list is now [1, ‘b’, 3, 4]
“`
- Clearing the list: The `clear()` method removes all elements.
“`python
my_list.clear() my_list is now []
“`
Comparison with Immutable Data Structures
To highlight the mutability of lists, it is helpful to compare them with immutable data structures, such as tuples. Below is a comparison table:
Feature | Lists | Tuples |
---|---|---|
Mutability | Mutable | Immutable |
Syntax | `[]` | `()` |
Methods for modification | Yes (append, remove) | No (cannot change) |
Iteration | Yes | Yes |
Use case | Frequently changing data | Fixed collections |
Practical Considerations
When using mutable lists, developers should be aware of certain considerations:
- Shared references: When passing lists to functions, they are passed by reference. Changes made within the function will affect the original list.
“`python
def modify_list(lst):
lst.append(5)
original_list = [1, 2, 3]
modify_list(original_list) original_list is now [1, 2, 3, 5]
“`
- Thread safety: In a multi-threaded environment, modifications to lists may lead to race conditions unless properly managed.
By understanding these characteristics and implications of Python lists’ mutability, programmers can effectively leverage this data structure to achieve efficient and dynamic data handling in their applications.
Understanding the Mutability of Python Lists
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Python lists are indeed mutable, which means that their contents can be changed after the list has been created. This characteristic allows for flexibility in programming, enabling developers to modify data structures dynamically as needed.”
Michael Chen (Lead Data Scientist, Data Solutions Group). “The mutability of Python lists is a fundamental aspect that distinguishes them from tuples. Being mutable allows lists to support operations such as appending, removing, and altering elements, which is crucial for data manipulation in various applications.”
Sarah Thompson (Python Instructor, Code Academy). “Understanding that Python lists are mutable is essential for beginners. It enables them to grasp the concept of reference vs. value, as changes to a list will reflect in all references to that list, which can lead to unintended side effects if not managed properly.”
Frequently Asked Questions (FAQs)
Are Python lists mutable?
Yes, Python lists are mutable, meaning their contents can be changed after they are created. You can add, remove, or modify elements within a list.
What does it mean for a list to be mutable?
Mutability refers to the ability of an object to be modified in place. For lists, this includes changing elements, appending new items, or removing existing ones without creating a new list.
How can I modify a Python list?
You can modify a Python list using methods such as `append()`, `extend()`, `insert()`, `remove()`, and `pop()`, or by directly accessing elements via their index.
Can I change the type of elements in a Python list?
Yes, you can change the type of elements in a Python list. Lists can hold mixed data types, and you can replace an element with a different type at any time.
Are there any limitations to mutability in Python lists?
While lists themselves are mutable, the objects contained within them may not be. For instance, if a list contains immutable objects like tuples or strings, those objects cannot be changed directly.
How does mutability affect performance in Python?
Mutability can lead to performance benefits when modifying data structures, as lists can be altered without the overhead of creating new objects. However, excessive modifications can lead to fragmentation and increased memory usage.
In summary, Python lists are indeed mutable, which means that their contents can be changed after the list has been created. This mutability allows for a range of operations, such as adding, removing, or altering elements within the list without needing to create a new list. This characteristic is fundamental to the functionality of lists in Python, making them a versatile data structure for various programming tasks.
The ability to modify lists in place provides significant advantages in terms of performance and memory usage. Since lists can be altered without creating new copies, they allow for efficient manipulation of data, especially in scenarios involving large datasets. This mutability also facilitates dynamic programming techniques, where the size and contents of the list may need to change frequently during execution.
However, it is essential to understand the implications of mutability, particularly in the context of shared references. When multiple variables reference the same list, changes made through one variable will reflect in all references. This behavior can lead to unintended side effects if not carefully managed. Therefore, developers should be mindful of how they handle list references in their code.
the mutability of Python lists is a powerful feature that enhances their usability in programming. Understanding this property, along with its implications,
Author Profile
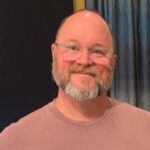
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?