Are Python Dictionaries Mutable? Understanding Their Flexibility and Usage
In the world of programming, understanding data structures is crucial for writing efficient and effective code. Among the myriad of data types available in Python, dictionaries stand out for their versatility and ease of use. But as you delve deeper into the intricacies of Python, one question often arises: Are Python dictionaries mutable? This seemingly simple query opens the door to a broader exploration of how data can be manipulated and stored in Python, providing insights that can enhance your coding skills and deepen your understanding of the language.
Dictionaries in Python are a powerful tool for managing collections of data, allowing you to store key-value pairs with remarkable efficiency. Their ability to provide quick access to values based on unique keys makes them an essential component of many programming tasks. However, the concept of mutability—whether an object can be changed after its creation—plays a pivotal role in how you can interact with dictionaries. Understanding whether dictionaries are mutable not only influences how you manage data but also impacts performance and memory usage in your applications.
As we explore the mutability of Python dictionaries, we will uncover the implications of this characteristic on programming practices. We’ll look at how mutability allows for dynamic data manipulation, the potential pitfalls it may introduce, and the best practices for working with dictionaries in your projects. So
Understanding Mutability in Python
In Python, mutability refers to the ability of an object to change its state or content after it has been created. Mutable objects can be altered in place, while immutable objects cannot be modified once they are created. This distinction is crucial for understanding how Python handles data structures, particularly dictionaries.
Characteristics of Python Dictionaries
Python dictionaries are a built-in data type that stores data in key-value pairs. They are implemented as hash tables, which allows for fast access to values based on their associated keys. The following characteristics highlight the nature of dictionaries:
- Key-Value Pairing: Each entry in a dictionary consists of a key and a corresponding value. Keys must be unique and immutable (e.g., strings, numbers, tuples), while values can be of any data type, including mutable types.
- Dynamic Sizing: Dictionaries can grow and shrink as items are added or removed, which is a hallmark of mutable structures.
- Unordered Collection: The items in a dictionary do not maintain any specific order. However, as of Python 3.7, dictionaries preserve the insertion order of keys.
Mutability of Dictionaries
Python dictionaries are indeed mutable. This mutability allows users to perform a variety of operations that modify the dictionary’s contents without creating a new object. Key operations that demonstrate this mutability include:
- Adding New Key-Value Pairs: New entries can be added using assignment.
- Updating Existing Values: Values associated with existing keys can be modified.
- Removing Entries: Key-value pairs can be deleted using the `del` statement or the `pop()` method.
The following table summarizes the mutability operations on Python dictionaries:
Operation | Description |
---|---|
Add | Insert a new key-value pair: `dict[key] = value` |
Update | Change the value of an existing key: `dict[key] = new_value` |
Delete | Remove a key-value pair: `del dict[key]` or `dict.pop(key)` |
These operations underscore the flexibility and dynamic nature of dictionaries, making them a powerful tool for managing collections of data. Understanding their mutable nature is essential for effectively leveraging dictionaries in various programming scenarios.
Understanding Mutability in Python Dictionaries
Python dictionaries are a fundamental data structure that allows you to store key-value pairs. One of their most important characteristics is their mutability, which means that the contents of a dictionary can be changed after it has been created.
Key Characteristics of Mutability
- Modification of Entries: You can change the value associated with a specific key.
- Addition of New Key-Value Pairs: New entries can be added to the dictionary.
- Deletion of Entries: Existing key-value pairs can be removed.
Examples of Dictionary Mutability
The following examples illustrate how dictionaries can be modified:
“`python
Creating a dictionary
my_dict = {‘name’: ‘Alice’, ‘age’: 30}
Modifying an existing entry
my_dict[‘age’] = 31
Adding a new key-value pair
my_dict[‘city’] = ‘New York’
Deleting a key-value pair
del my_dict[‘name’]
print(my_dict) Output: {‘age’: 31, ‘city’: ‘New York’}
“`
In this example, the dictionary `my_dict` undergoes several modifications, demonstrating its mutable nature.
Comparison with Immutable Data Types
To further clarify the concept of mutability, it is useful to compare dictionaries with immutable data types such as tuples and strings. The following table summarizes their differences:
Feature | Mutable Data Types | Immutable Data Types |
---|---|---|
Modification | Allowed (e.g., lists, dicts) | Not allowed (e.g., tuples, strings) |
Performance Overhead | Higher due to dynamic resizing | Lower, as they have fixed size |
Use Cases | When frequent changes are needed | When data integrity is crucial |
Implications of Mutability
The mutability of dictionaries has several implications for programming:
- Data Integrity: Care must be taken when passing dictionaries to functions, as they can be altered unintentionally.
- Performance: Mutability allows for efficient modifications, but programmers should be aware of the potential overhead associated with resizing and copying.
- Thread Safety: When used in multi-threaded environments, mutable structures like dictionaries require synchronization to avoid data corruption.
Best Practices for Using Mutable Dictionaries
When working with mutable dictionaries, consider the following best practices:
- Use Immutable Keys: Ensure that the keys in the dictionary are of immutable types, such as strings, numbers, or tuples.
- Copy Dictionaries When Needed: Use the `copy()` method or `copy` module to create copies of dictionaries when modifications to the original should be avoided.
- Document Changes: Clearly comment on any changes made to dictionaries within the code to maintain readability and maintainability.
By understanding the mutability of Python dictionaries, developers can harness their flexibility while managing potential pitfalls associated with their use.
Understanding the Mutability of Python Dictionaries
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Python dictionaries are indeed mutable, which means that their contents can be changed after creation. This characteristic allows developers to modify the dictionary by adding, removing, or updating key-value pairs without needing to create a new dictionary.”
James Liu (Data Scientist, Analytics Hub). “The mutability of Python dictionaries is a fundamental aspect that enables efficient data manipulation. It allows for dynamic data structures that can evolve as the program runs, making them an essential tool for data handling in Python.”
Sarah Thompson (Python Instructor, Code Academy). “Understanding that Python dictionaries are mutable is crucial for beginners. It emphasizes the importance of managing state and memory in programming, as changes to a dictionary can affect all references to it in the code.”
Frequently Asked Questions (FAQs)
Are Python dictionaries mutable?
Yes, Python dictionaries are mutable, meaning their contents can be changed after creation. You can add, remove, or modify key-value pairs.
What does it mean for a data structure to be mutable?
A mutable data structure allows for modification of its elements without creating a new instance. This includes changing values, adding new elements, or deleting existing ones.
Can you give an example of modifying a Python dictionary?
Certainly. You can modify a dictionary by using syntax like `my_dict[‘key’] = ‘new_value’` to change a value or `my_dict[‘new_key’] = ‘value’` to add a new key-value pair.
Are there any immutable alternatives to Python dictionaries?
Yes, the `collections.ChainMap` and `types.MappingProxyType` can be used as immutable alternatives to dictionaries. They provide read-only views of dictionaries.
What happens if you try to change an immutable object in Python?
Attempting to change an immutable object will raise a `TypeError`. Immutable objects, such as tuples and strings, do not allow modification of their contents.
How do mutable and immutable types affect performance in Python?
Mutable types, like dictionaries, can be more efficient for frequent updates due to their ability to modify in place. Immutable types may incur overhead when creating new instances for modifications.
In summary, Python dictionaries are indeed mutable data structures. This means that once a dictionary is created, its contents can be modified without the need to create a new dictionary. Users can add, remove, or change key-value pairs at any time, which provides significant flexibility when managing collections of data.
Furthermore, the mutability of dictionaries allows for dynamic data manipulation, making them an essential tool in Python programming. Developers can easily update their data structures to reflect changes in the application state, facilitating efficient data management and retrieval. This characteristic is particularly useful in scenarios where data is frequently updated or modified.
understanding the mutability of Python dictionaries is crucial for effective programming. It empowers developers to leverage dictionaries for various applications, from simple data storage to complex data manipulation tasks. As such, recognizing the implications of using mutable data structures can enhance code performance and maintainability.
Author Profile
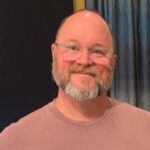
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?