How Can You Add a Class When Scrolling to an Element?
In the ever-evolving landscape of web design, creating a dynamic and engaging user experience is paramount. One effective technique that has gained popularity among developers is the ability to add classes to elements when the user scrolls to them. This approach not only enhances visual appeal but also improves functionality, making websites feel more interactive and responsive. Whether you’re looking to highlight specific sections, trigger animations, or change styles based on user behavior, understanding how to implement this feature can elevate your web projects to new heights.
As users navigate through a webpage, their attention can easily wane. By strategically adding classes to elements upon scrolling, you can draw their focus back to key content areas, creating a more immersive experience. This technique leverages the power of JavaScript and CSS, allowing for seamless transitions and transformations that react to user actions. From subtle changes in color and size to more complex animations, the possibilities are endless.
Moreover, adding classes on scroll can significantly enhance accessibility and usability. By signaling to users which parts of the page are currently relevant or important, you can guide their journey through your content more effectively. This article will delve into the mechanics of implementing this feature, offering insights and practical examples that will empower you to harness the full potential of scroll-triggered class additions. Prepare
Add Class When Scroll To Element
To effectively add a class when scrolling to a specific element on a webpage, you can leverage JavaScript or jQuery for better performance and responsiveness. This technique is particularly useful for enhancing user experience by highlighting sections or triggering animations as users navigate through your content.
One common approach is to use the `Intersection Observer` API, which allows you to asynchronously observe changes in the intersection of a target element with an ancestor element or the viewport. This method is more efficient than traditional scroll event listeners, as it does not cause performance issues when many elements are being monitored.
Implementing the Intersection Observer
Here’s a step-by-step guide to implementing this technique:
- **Select the Target Element**: Identify the element that will receive the new class when it comes into view.
- **Create the Observer**: Instantiate an `IntersectionObserver` with a callback function that will execute whenever the target enters or exits the viewport.
- **Define the Options**: You can specify options like `root`, `rootMargin`, and `threshold` to customize the observer’s behavior.
- **Observe the Element**: Use the `observe` method to start observing the target element.
Example Code
“`javascript
// Select the target element
const targetElement = document.querySelector(‘.target’);
// Create an intersection observer
const observer = new IntersectionObserver((entries) => {
entries.forEach(entry => {
if (entry.isIntersecting) {
targetElement.classList.add(‘active’); // Add class when in view
} else {
targetElement.classList.remove(‘active’); // Remove class when out of view
}
});
}, {
threshold: 0.1 // Trigger when 10% of the element is visible
});
// Start observing the target element
observer.observe(targetElement);
“`
jQuery Alternative
If your project uses jQuery, you can achieve a similar effect using the following approach. This utilizes the `scroll` event to check the position of the target element relative to the viewport.
Example Code
“`javascript
$(window).on(‘scroll’, function() {
var scrollPos = $(window).scrollTop();
var targetPos = $(‘.target’).offset().top;
if (scrollPos + $(window).height() > targetPos) {
$(‘.target’).addClass(‘active’); // Add class when scrolled to
} else {
$(‘.target’).removeClass(‘active’); // Remove class when scrolled past
}
});
“`
Performance Considerations
While using the `scroll` event in jQuery is straightforward, it can lead to performance issues on pages with many scroll events. The Intersection Observer API is recommended for improved performance, especially on pages with multiple elements to track.
Key Advantages of Intersection Observer
- Better Performance: Reduces the number of calculations required during scroll events.
- Versatile: Can observe multiple elements without significant performance degradation.
- Asynchronous: Works independently of the main thread, enhancing responsiveness.
Feature | Intersection Observer | jQuery Scroll Event |
---|---|---|
Performance | High | Moderate |
Number of Observed Elements | Many | Limited |
Ease of Use | Moderate | Easy |
Compatibility | Modern Browsers | Widely Supported |
By implementing these methods, you can enhance the visual dynamics of your site as users scroll, creating a more engaging and interactive experience.
Understanding the Scroll Event
The scroll event is a critical aspect of web development, allowing developers to trigger actions based on the user’s scrolling behavior. In this context, detecting when a user scrolls to a specific element can enhance user experience significantly.
- Event Listener: The scroll event can be monitored by attaching an event listener to the window object.
- Performance Considerations: It is advisable to debounce or throttle the scroll event to improve performance, as firing too many events can lead to lag.
Implementing the Scroll Detection
To add a class when the user scrolls to a specific element, one can use JavaScript or jQuery. Below is a straightforward implementation using vanilla JavaScript.
“`javascript
window.addEventListener(‘scroll’, function() {
const targetElement = document.getElementById(‘target’);
const rect = targetElement.getBoundingClientRect();
if (rect.top < window.innerHeight && rect.bottom >= 0) {
targetElement.classList.add(‘active’);
} else {
targetElement.classList.remove(‘active’);
}
});
“`
- getBoundingClientRect(): This method retrieves the size of the element and its position relative to the viewport.
- Condition Check: The conditions check if the element is within the viewport.
Using jQuery for Scroll Detection
For those who prefer jQuery, the implementation can be simplified with its built-in methods.
“`javascript
$(window).on(‘scroll’, function() {
var targetElement = $(‘target’);
var elementTop = targetElement.offset().top;
var elementHeight = targetElement.outerHeight();
if ($(window).scrollTop() + $(window).height() > elementTop &&
$(window).scrollTop() < elementTop + elementHeight) {
targetElement.addClass('active');
} else {
targetElement.removeClass('active');
}
});
```
- offset(): Retrieves the current coordinates of the first element in the set of matched elements.
- outerHeight(): Gets the outer height of an element, including padding and border.
Styling the Active Class
Once the class is added, it is essential to style it accordingly to create a noticeable effect. Below is an example of CSS that can be applied to the active class.
“`css
target.active {
transition: background-color 0.3s ease;
background-color: yellow;
}
“`
- Transition: Smooths the color change, enhancing visual feedback.
- Background Color: Provides a clear indication that the element is active.
Performance Optimization Techniques
To ensure that the scroll detection remains performant, consider the following techniques:
– **Debouncing**: Limits the rate at which the function is executed. This is helpful for heavy computations.
– **Throttling**: Ensures that the function is called at most once every specified time interval.
Example of a debounce function:
“`javascript
function debounce(func, delay) {
let timeout;
return function(…args) {
clearTimeout(timeout);
timeout = setTimeout(() => func.apply(this, args), delay);
};
}
window.addEventListener(‘scroll’, debounce(function() {
// Scroll detection logic
}, 200));
“`
- Optimize Selectors: Cache selectors that are used multiple times in the scroll event.
Cross-Browser Compatibility
When implementing scroll detection, ensure that your code is compatible across different browsers. Utilize feature detection and consider potential polyfills for older browsers.
Feature | Supported Browsers |
---|---|
`getBoundingClientRect` | All modern browsers |
`classList` | IE10+ |
jQuery methods | jQuery 1.9+ |
Testing in various environments is crucial to avoid unexpected behavior.
Expert Insights on Adding Classes When Scrolling to Elements
Jessica Lin (Front-End Developer, CodeCraft). “Implementing a class addition upon scrolling to an element enhances user experience by providing visual feedback. It allows developers to create dynamic interfaces that engage users and highlight important content as they navigate.”
Michael Chen (UI/UX Designer, Digital Trends). “The strategic use of classes when an element comes into view can significantly improve the aesthetic appeal of a website. It allows for animations and transitions that can draw attention to key areas, making the interaction more intuitive.”
Sarah Patel (Web Performance Specialist, Speedy Sites). “From a performance perspective, adding classes on scroll can be optimized to reduce reflows and repaints. It is crucial to ensure that the implementation is efficient to avoid compromising the overall performance of the website.”
Frequently Asked Questions (FAQs)
What is the purpose of adding a class when scrolling to an element?
Adding a class when scrolling to an element allows developers to trigger specific styles or behaviors, enhancing user interaction and visual feedback as users navigate a webpage.
How can I implement the functionality to add a class on scroll?
You can implement this functionality using JavaScript or jQuery. By listening for the scroll event, you can check the position of the element relative to the viewport and add a class when it comes into view.
Is it possible to remove the class when the element is out of view?
Yes, it is possible to remove the class when the element is out of view. You can achieve this by checking the element’s position during the scroll event and toggling the class based on its visibility.
What are some common use cases for adding classes on scroll?
Common use cases include triggering animations, changing styles for header navigation, highlighting sections, or implementing lazy loading effects as users scroll through content.
Are there any performance concerns when using scroll events?
Yes, performance can be a concern with scroll events, especially if the event listener executes heavy computations. It is advisable to debounce or throttle the scroll event to improve performance and user experience.
Can this functionality be achieved using CSS alone?
No, this functionality cannot be achieved using CSS alone. CSS does not have the capability to detect scroll events or manipulate classes dynamically; JavaScript is required for this interaction.
In summary, the technique of adding a class when scrolling to an element is a powerful tool in web development that enhances user experience and engagement. This method typically involves utilizing JavaScript or jQuery to detect the scroll position of the webpage and applying a specific class to an element when it enters the viewport. This functionality can be particularly useful for triggering animations, changing styles, or highlighting content as users navigate through a page.
Key takeaways from this discussion include the importance of understanding the intersection of the viewport and the targeted element. Developers can leverage the Intersection Observer API for efficient performance, as it provides a more optimized way to monitor the visibility of elements compared to traditional scroll event listeners. Moreover, implementing this feature can significantly improve the visual dynamics of a website, making it more interactive and visually appealing.
Additionally, it is crucial to consider accessibility and performance when implementing such features. Ensuring that the added classes do not hinder the user experience on various devices and maintaining fast load times are essential factors. By focusing on these aspects, developers can create a seamless and engaging experience for users while effectively utilizing the technique of adding classes during scroll events.
Author Profile
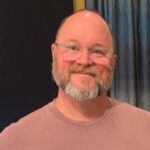
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?