How Can You Add a String to LocalStorage Using PHP?
In the realm of web development, local storage has emerged as a powerful tool for enhancing user experience and optimizing application performance. As developers strive to create seamless, interactive applications, the ability to store data on the client side becomes increasingly valuable. One common task is adding strings to local storage, which allows for the preservation of user preferences, session information, and other vital data without the need for constant server communication. This article will delve into the intricacies of utilizing local storage in PHP, guiding you through the steps to effectively manage string data within your applications.
Understanding how to manipulate local storage can significantly elevate your web projects. Local storage provides a simple key-value store that can be accessed through JavaScript, making it an ideal choice for storing strings that enhance user engagement. By leveraging PHP to generate dynamic content, developers can easily integrate local storage capabilities, ensuring that user interactions are remembered and catered to in future visits.
As we explore the process of adding strings to local storage, we will cover essential concepts such as the interplay between PHP and JavaScript, the syntax for storing data, and best practices for maintaining data integrity. Whether you’re a seasoned developer looking to refine your skills or a newcomer eager to learn, this guide will equip you with the knowledge to harness the full potential of local storage
Add a String to LocalStorage in PHP
LocalStorage is a web storage solution provided by browsers that allows web applications to store data in a key-value format. Although LocalStorage is primarily a JavaScript feature, PHP can interact with it indirectly through JavaScript code. To add a string to LocalStorage using PHP, you typically generate a JavaScript snippet that the browser will execute.
To accomplish this, follow these steps:
- Generate JavaScript with PHP: You can echo out JavaScript code from PHP that will set a value in LocalStorage.
- Use JSON for Complex Data: If you need to store complex data structures, consider using JSON to serialize your data.
Here’s an example of how to set a string in LocalStorage:
“`php
localStorage.setItem(‘myString’, ‘” . addslashes($stringToStore) . “‘);
“;
?>
“`
In this example, `addslashes()` is used to escape any quotes in the string, ensuring that the JavaScript code is valid. The `localStorage.setItem()` method is called to store the string under the key `myString`.
Retrieving Data from LocalStorage
Once a string has been stored in LocalStorage, it can be retrieved using JavaScript. This is done using the `localStorage.getItem()` method. Here is an example:
“`php
var myString = localStorage.getItem(‘myString’);
console.log(myString); // Outputs: Hello, LocalStorage!
“;
?>
“`
This script retrieves the value associated with the key `myString` and logs it to the console.
Managing LocalStorage Data
Managing LocalStorage effectively includes storing, retrieving, and removing data as necessary. Here are some key operations:
- Store Data: Use `localStorage.setItem(key, value);`.
- Retrieve Data: Use `localStorage.getItem(key);`.
- Remove Data: Use `localStorage.removeItem(key);`.
- Clear All Data: Use `localStorage.clear();`.
Operation | JavaScript Code | PHP Integration |
---|---|---|
Store Data | localStorage.setItem(‘key’, ‘value’); | echo ““; |
Retrieve Data | localStorage.getItem(‘key’); | echo ““; |
Remove Data | localStorage.removeItem(‘key’); | echo ““; |
Clear All Data | localStorage.clear(); | echo ““; |
This table summarizes the basic operations for managing LocalStorage, highlighting how to integrate them within PHP scripts. By understanding these concepts, developers can effectively use LocalStorage to enhance user experience on web applications.
Understanding Local Storage in PHP
Local storage is a web storage solution that allows web applications to store data persistently in a user’s browser. However, PHP operates on the server side and does not directly interact with local storage, which is primarily a client-side feature. To utilize local storage in conjunction with PHP, you typically use JavaScript on the client side to handle the storage.
Storing Strings in Local Storage
To add a string to local storage using JavaScript, follow these steps:
- Use the `localStorage.setItem()` method.
- Define a key and a value (string) to store.
Here is a simple example:
“`javascript
localStorage.setItem(‘myKey’, ‘myValue’);
“`
This command will store the string ‘myValue’ under the key ‘myKey’.
Integrating PHP and Local Storage
To effectively store a string in local storage after processing data with PHP, you can use the following approach:
- Generate PHP data:
Use PHP to generate the data you want to store.
“`php
$dataToStore = “Hello, Local Storage!”;
“`
- Echo the data in a script tag:
Embed this data into a JavaScript variable on your web page.
“`php
echo ““;
“`
- Verify storage:
After executing the above, you can check the data in local storage.
“`javascript
console.log(localStorage.getItem(‘greeting’)); // Outputs: Hello, Local Storage!
“`
Retrieving Data from Local Storage
To retrieve data from local storage, use the `localStorage.getItem()` method. This can be done in JavaScript as follows:
“`javascript
let storedValue = localStorage.getItem(‘myKey’);
console.log(storedValue); // Displays the stored value
“`
This method returns the value associated with the specified key or `null` if the key does not exist.
Removing Data from Local Storage
If you need to remove a stored string from local storage, you can utilize the `localStorage.removeItem()` method:
“`javascript
localStorage.removeItem(‘myKey’);
“`
To clear all data from local storage, use:
“`javascript
localStorage.clear();
“`
Using Local Storage with Form Data
You may want to save form input data to local storage. Here’s how to achieve this using JavaScript:
- Capture the input value from a form.
- Store it in local storage when the form is submitted.
Example:
“`html
“`
This approach allows users to retain their input even after refreshing the page.
Considerations for Local Storage
When utilizing local storage, consider the following:
- Data Size: Local storage typically allows around 5-10 MB of data per domain.
- Data Type: All data stored is in string format; objects must be serialized (e.g., using JSON).
- Security: Local storage is accessible through JavaScript, which can expose it to XSS attacks. Always sanitize user inputs.
- Availability: Ensure users’ browsers support local storage; older browsers may not.
By understanding these aspects, you can effectively manage local storage in your PHP applications through client-side JavaScript.
Expert Insights on Adding a String to Local Storage in PHP
Jessica Lin (Web Development Specialist, CodeCraft Solutions). “When working with local storage in PHP, it is crucial to understand that PHP itself cannot directly manipulate the client’s local storage. Instead, you should use JavaScript to handle local storage operations, while PHP can be used to generate the necessary JavaScript code dynamically.”
Michael Thompson (Full Stack Developer, Tech Innovators Inc.). “To effectively add a string to local storage in a PHP environment, developers should consider using AJAX to send data from PHP to JavaScript. This allows for seamless integration of server-side data into the client’s local storage, enhancing user experience and performance.”
Sarah Patel (Software Engineer, Digital Solutions Group). “Utilizing local storage in conjunction with PHP can significantly improve application responsiveness. However, it is essential to ensure that the data being stored is not sensitive, as local storage is accessible via JavaScript and can pose security risks if not handled properly.”
Frequently Asked Questions (FAQs)
How can I add a string to localStorage in PHP?
LocalStorage is a web storage feature that operates on the client side, typically using JavaScript. PHP cannot directly manipulate localStorage, but you can use PHP to generate JavaScript code that adds a string to localStorage when the page loads.
Can I store PHP variables directly in localStorage?
No, you cannot store PHP variables directly in localStorage since localStorage is a client-side feature. However, you can convert PHP variables to JSON format and then use JavaScript to store that JSON string in localStorage.
What is the syntax to set an item in localStorage using JavaScript?
The syntax to set an item in localStorage is `localStorage.setItem(‘key’, ‘value’);`. You can replace `’key’` with your desired identifier and `’value’` with the string you want to store.
How do I retrieve a string from localStorage in JavaScript?
To retrieve a string from localStorage, use the syntax `localStorage.getItem(‘key’);`. This will return the value associated with the specified key.
Is there a way to clear localStorage using PHP?
PHP cannot directly clear localStorage since it operates on the server side. However, you can generate JavaScript code to clear localStorage by using `localStorage.clear();`, which can be executed on the client side when needed.
What are the limitations of localStorage?
LocalStorage has a storage limit of approximately 5-10 MB per origin, depending on the browser. Additionally, data stored in localStorage is not accessible across different domains and is not suitable for sensitive information as it is not encrypted.
In summary, adding a string to local storage in PHP involves understanding the interaction between server-side and client-side technologies. PHP operates on the server, while local storage is a feature of web browsers that allows for the storage of data in the user’s browser. To effectively store a string in local storage, PHP can be used to generate JavaScript code that executes in the client’s browser, thereby bridging the gap between these two environments.
Key takeaways include the importance of using JavaScript to manipulate local storage, as PHP itself does not have direct access to the client’s local storage. By embedding JavaScript within PHP scripts, developers can dynamically create scripts that store data in local storage. Additionally, it is crucial to ensure that the data being stored is properly sanitized and validated to maintain security and integrity.
Moreover, understanding the limitations and capabilities of local storage is essential. Local storage is limited to the same-origin policy, meaning data can only be accessed by pages from the same domain. This reinforces the need for careful planning in web application design, ensuring that data is stored and retrieved efficiently while adhering to security best practices.
Author Profile
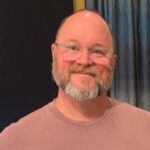
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?