How Can You Troubleshoot and Resolve ‘A Fatal Javascript Error Occurred’ in Your Web Applications?
### Introduction
In the ever-evolving landscape of web development, JavaScript stands as a cornerstone technology, powering dynamic and interactive experiences across the internet. However, with great power comes great responsibility, and developers often find themselves grappling with the complexities of this versatile language. Among the myriad of challenges that can arise, few are as daunting as encountering a message that reads, “A Fatal JavaScript Error Occurred.” This ominous alert can send even seasoned programmers into a spiral of confusion and frustration, as it signifies a critical failure that halts execution and disrupts user experience. In this article, we will delve into the intricacies of this error, exploring its causes, implications, and the best practices for troubleshooting and resolution.
When a fatal JavaScript error occurs, it can stem from a variety of sources, including syntax mistakes, reference errors, or issues with external libraries. Understanding the underlying reasons for these errors is crucial for developers aiming to create robust applications. This article will provide an overview of common triggers that lead to fatal errors, shedding light on how seemingly innocuous lines of code can lead to catastrophic failures in functionality.
Moreover, we will discuss the impact of these errors on both the development process and user experience. A single fatal error can not only halt a web
A Fatal JavaScript Error Occurred
When a fatal JavaScript error occurs, it typically indicates a serious issue that disrupts the execution of a script, preventing it from completing its intended function. These errors can arise from various factors, including syntax errors, type errors, or issues with asynchronous code execution. Understanding the nature of these errors and how to address them is crucial for developers aiming to create robust web applications.
Common causes of fatal JavaScript errors include:
- Syntax Errors: Mistakes in the code structure, such as missing brackets or incorrect function calls.
- Type Errors: Operations performed on incompatible data types, such as trying to call a non-function value.
- Reference Errors: Attempting to access variables that are not defined in the current scope.
- Network Issues: Problems with external resources that fail to load, leading to unhandled exceptions.
To effectively diagnose and resolve these errors, developers can utilize the following strategies:
- Debugging Tools: Use browser developer tools to inspect and debug code. The console can provide error messages that pinpoint the location of the issue.
- Error Handling: Implement try-catch blocks to gracefully handle exceptions and prevent the application from crashing.
- Logging: Utilize logging frameworks to capture error details and stack traces, aiding in the identification of the problem’s root cause.
Common Types of Fatal JavaScript Errors
Understanding the various types of fatal JavaScript errors is essential for effective troubleshooting. Below is a breakdown of the most prevalent error types:
Error Type | Description | Example |
---|---|---|
Syntax Error | Occurs when the code does not conform to the syntax rules of JavaScript. | Missing closing bracket: `if (x > 10 { … } |
Type Error | Happens when a value is not of the expected type. | Calling a method on a non-function: `null.f();` |
Reference Error | Triggered when trying to access a variable that hasn’t been declared. | Accessing an undeclared variable: `console.log(a);` |
Range Error | Occurs when a value is not within the set or expected range. | Invalid array length: `let arr = new Array(-1);` |
Best Practices for Avoiding Fatal Errors
To minimize the occurrence of fatal JavaScript errors, developers should adhere to best practices that enhance code quality and maintainability:
- Use Strict Mode: Enabling strict mode (`’use strict’;`) helps catch common coding issues by throwing errors for potentially problematic syntax.
- Code Reviews: Regular peer reviews can identify potential errors early in the development process.
- Unit Testing: Implement comprehensive unit tests to validate the functionality of code segments and catch errors before deployment.
- Consistent Coding Standards: Follow established coding standards and guidelines to ensure clarity and reduce the likelihood of mistakes.
By applying these strategies and understanding the nature of fatal JavaScript errors, developers can enhance the reliability of their applications and create a smoother user experience.
Understanding the Error Message
The message “A Fatal Javascript Error Occurred” generally indicates a severe problem in the JavaScript execution environment that halts the operation of the script. This could be caused by various issues such as syntax errors, runtime errors, or environment-related problems.
- Syntax Errors: Mistakes in the code that violate JavaScript’s grammatical rules. These errors are typically caught during the parsing phase.
- Runtime Errors: Issues that arise while the script is executing, such as attempting to call a method on an undefined variable.
- Resource Limitations: Errors that occur due to exceeding memory limits or other constraints imposed by the host environment.
Common Causes
Identifying the root cause is essential for resolving the issue effectively. Here are some common triggers:
Cause | Description |
---|---|
Undefined Variables | Attempting to access a variable that hasn’t been defined. |
Incorrect Function Calls | Calling a function with the wrong number or type of arguments. |
Infinite Loops | Scripts that run indefinitely due to loop conditions not terminating. |
External Resource Issues | Problems related to loading scripts or accessing APIs that fail. |
Browser Compatibility | Variability in JavaScript support across different browsers. |
Troubleshooting Steps
To effectively troubleshoot the error, follow these steps:
- Check the Console: Use the browser’s developer tools to inspect console messages. Look for stack traces or additional error information.
- Review Recent Changes: If the error appeared after a recent update, revert the changes to identify the source of the problem.
- Validate Code Syntax: Utilize tools like JSHint or ESLint to analyze the code for syntax errors.
- Isolate the Issue: Comment out sections of code to identify the specific line causing the error.
- Test in Different Browsers: Run the application in various browsers to determine if the issue is browser-specific.
Preventive Measures
Implementing best practices can prevent fatal JavaScript errors:
- Code Reviews: Regularly review code with peers to catch potential issues early.
- Automated Testing: Employ unit tests and integration tests to ensure code functionality before deployment.
- Error Handling: Use try-catch blocks to gracefully handle errors and provide fallback mechanisms.
- Use Modern JavaScript Features: Leverage features like ES6 modules to improve code organization and reduce conflicts.
Resolving the Issue
Once the root cause is identified, apply the following resolution strategies:
- Fix Syntax Errors: Correct any syntax mistakes indicated by tools or the console.
- Handle Undefined Variables: Initialize variables properly before use to avoid undefined behavior.
- Refactor Problematic Code: Simplify complex logic that may lead to runtime errors.
- Optimize Loops: Ensure all loops have a clear termination condition.
Monitoring and Logging
For ongoing maintenance and to prevent future occurrences, consider implementing monitoring solutions:
- Error Tracking Tools: Utilize services like Sentry or Rollbar to automatically log JavaScript errors in production.
- Performance Monitoring: Tools like New Relic or Google Analytics can help identify slow scripts or resource bottlenecks.
By adopting a proactive approach to debugging and monitoring, developers can significantly reduce the frequency and impact of fatal JavaScript errors.
Understanding and Resolving Fatal JavaScript Errors
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “A fatal JavaScript error typically indicates a critical issue that prevents the script from executing. This can stem from syntax errors, reference errors, or issues with asynchronous code execution. Developers must implement robust error handling and debugging techniques to identify and resolve these issues effectively.”
James Liu (Web Development Specialist, CodeCraft Academy). “Encountering a fatal JavaScript error can be daunting, especially for new developers. It is crucial to utilize browser developer tools to trace the error back to its source. Understanding the call stack and utilizing console logs can significantly aid in diagnosing the problem before it escalates.”
Sarah Thompson (JavaScript Framework Consultant, Frontend Experts). “The impact of a fatal JavaScript error extends beyond mere functionality; it can affect user experience and application performance. Adopting best practices, such as modular coding and thorough testing, can mitigate the risk of such errors occurring in production environments.”
Frequently Asked Questions (FAQs)
What does “A Fatal Javascript Error Occurred” mean?
This error message indicates that a critical issue has occurred in the JavaScript code, preventing the script from executing properly. It typically results from syntax errors, type errors, or issues with external libraries.
What are common causes of a Fatal Javascript Error?
Common causes include incorrect syntax, calling functions that do not exist, accessing properties of undefined variables, and issues with asynchronous code handling or promise rejections.
How can I troubleshoot a Fatal Javascript Error?
To troubleshoot, check the browser’s developer console for error messages, review the relevant code for syntax issues, and ensure that all dependencies are correctly loaded. Debugging tools can also help identify the source of the problem.
Does a Fatal Javascript Error affect website functionality?
Yes, a Fatal Javascript Error can significantly impact website functionality, potentially causing features to break or the entire site to become unresponsive, depending on where the error occurs in the code.
Can I prevent Fatal Javascript Errors in my code?
Yes, you can prevent such errors by following best practices, including thorough testing, using linters for code quality, handling exceptions gracefully, and writing modular, maintainable code.
What should I do if I encounter this error in production?
If encountered in production, immediately log the error details for analysis, notify your development team, and consider implementing a temporary fallback or maintenance mode to minimize user impact while resolving the issue.
The phrase “A Fatal Javascript Error Occurred” typically indicates a critical failure in the execution of JavaScript code, which can disrupt the functionality of web applications. Such errors may arise from various issues, including syntax errors, reference errors, or problems with asynchronous code execution. Identifying the root cause of these errors is essential for developers, as they can significantly impact user experience and application performance.
To effectively address fatal JavaScript errors, developers should employ robust debugging techniques. Utilizing browser developer tools can aid in pinpointing the exact location of the error, allowing for quicker resolution. Additionally, implementing error handling mechanisms, such as try-catch blocks, can help manage unexpected failures gracefully, ensuring that the application remains functional even when issues arise.
Moreover, maintaining clean and well-structured code is vital in preventing such errors. Following best practices, such as code reviews and adhering to coding standards, can minimize the likelihood of encountering fatal errors. Regular testing, including unit tests and integration tests, can also help catch potential issues early in the development process, leading to more stable and reliable applications.
understanding and addressing fatal JavaScript errors is crucial for developers aiming to create seamless web experiences. By leveraging debugging tools, implementing error
Author Profile
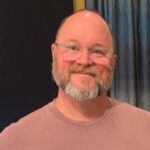
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?