Why Choose A.All Python for Your Next Programming Project?
In the ever-evolving landscape of programming languages, Python stands out as a versatile powerhouse, captivating developers and data enthusiasts alike. With its clean syntax and robust libraries, Python has transcended its initial role as a simple scripting language to become a cornerstone in fields ranging from web development to artificial intelligence. As we delve into the world of “All Python,” we will explore the myriad ways this language empowers innovation, fosters creativity, and shapes the future of technology.
The beauty of Python lies not only in its simplicity but also in its extensive ecosystem. From its foundational principles that prioritize readability and efficiency to its expansive libraries that cater to various domains, Python offers a unique blend of accessibility and depth. Whether you are a novice looking to embark on your programming journey or a seasoned developer seeking to enhance your skill set, understanding the full spectrum of Python’s capabilities is essential in today’s tech-driven world.
As we navigate through the intricacies of Python, we will uncover its applications across different industries, the communities that support its growth, and the tools that make it an essential part of any developer’s toolkit. Join us as we embark on this exploration of “All Python,” where we will illuminate the pathways that lead to mastering this dynamic language and harnessing its potential to drive innovation and
Data Structures in Python
Python provides several built-in data structures that are essential for organizing and managing data efficiently. The primary data structures include lists, tuples, sets, and dictionaries. Each has its unique properties and use cases.
- Lists: Ordered and mutable collections of items. They can contain elements of different types.
- Tuples: Similar to lists but immutable, meaning once created, their contents cannot be altered.
- Sets: Unordered collections of unique items, useful for membership testing and eliminating duplicate entries.
- Dictionaries: Key-value pairs that allow for fast retrieval of data. Keys must be unique and immutable.
Data Structure | Mutable | Ordered | Duplicates |
---|---|---|---|
List | Yes | Yes | Yes |
Tuple | No | Yes | Yes |
Set | Yes | No | No |
Dictionary | Yes | Yes (as of Python 3.7) | No (keys only) |
Control Flow Statements
Control flow statements in Python allow developers to dictate the execution order of code blocks. The primary control flow statements include conditionals, loops, and exception handling.
- Conditionals: `if`, `elif`, and `else` statements enable branching logic based on conditions.
Example:
“`python
if condition:
Execute this block
elif another_condition:
Execute this block
else:
Execute this block
“`
- Loops: `for` and `while` loops facilitate repeated execution of code blocks.
Example:
“`python
for item in iterable:
Execute this block
while condition:
Execute this block
“`
- Exception Handling: Using `try`, `except`, `finally`, and `else`, developers can manage errors gracefully.
Example:
“`python
try:
Code that may cause an exception
except SpecificException:
Handle the exception
else:
Execute if no exception occurs
finally:
Execute regardless of the outcome
“`
Functions and Modules
Functions in Python are blocks of reusable code designed to perform a specific task. They promote modularity and code reusability. Functions can accept parameters and return values.
- Defining Functions: Functions are defined using the `def` keyword.
Example:
“`python
def function_name(parameters):
Function body
return value
“`
- Modules: A module is a file containing Python code that can define functions, classes, or variables. Modules promote organization and reusability of code.
Example of importing a module:
“`python
import module_name
“`
- Built-in Functions: Python provides numerous built-in functions, such as `len()`, `max()`, and `min()`, which can be utilized without needing to define them.
Utilizing functions and modules effectively enhances code clarity and maintainability, making it easier to manage larger projects.
Understanding Python Basics
Python is a versatile programming language that emphasizes readability and simplicity, making it ideal for both beginners and experienced developers. Below are key concepts and components that form the foundation of Python programming.
- Variables and Data Types:
- Variables are used to store information. Python supports several data types including:
- Integers (`int`): Whole numbers.
- Floating-point numbers (`float`): Decimal numbers.
- Strings (`str`): Sequence of characters enclosed in quotes.
- Booleans (`bool`): Represents `True` or “.
- Control Structures:
- Conditional Statements:
- `if`, `elif`, and `else` are used to execute code based on conditions.
- Loops:
- `for` loops iterate over a sequence (like a list or a string).
- `while` loops continue until a specified condition is .
- Functions:
- Functions in Python are defined using the `def` keyword. They encapsulate reusable blocks of code.
- Example:
“`python
def greet(name):
return f”Hello, {name}!”
“`
Advanced Python Features
Python offers various advanced features that enhance its functionality and efficiency.
- List Comprehensions:
- A concise way to create lists. For example:
“`python
squares = [x**2 for x in range(10)]
“`
- Decorators:
- A way to modify the behavior of functions or methods. They are defined using the `@decorator_name` syntax.
- Generators:
- Functions that return an iterable set of items, one at a time, using the `yield` statement. This is useful for handling large datasets efficiently.
- Context Managers:
- Simplify resource management, such as file handling. They are implemented using the `with` statement:
“`python
with open(‘file.txt’) as file:
data = file.read()
“`
Python Libraries and Frameworks
Python’s extensive library ecosystem allows developers to tackle a wide range of tasks efficiently.
Library/Framework | Purpose |
---|---|
NumPy | Numerical computing with powerful array objects. |
Pandas | Data manipulation and analysis. |
Matplotlib | Data visualization through plotting graphs. |
Flask | Lightweight web framework for building web applications. |
Django | High-level web framework that encourages rapid development. |
- Installation:
- Libraries are typically installed using `pip`:
“`bash
pip install library_name
“`
Best Practices in Python Programming
Adhering to best practices enhances code quality and maintainability.
- Code Readability:
- Follow the PEP 8 style guide for Python code formatting.
- Use meaningful variable and function names.
- Documentation:
- Incorporate docstrings to describe functions and modules:
“`python
def example_function(param):
“””Brief description of the function.”””
pass
“`
- Testing:
- Utilize testing frameworks like `unittest` or `pytest` to ensure code reliability.
- Version Control:
- Employ Git for tracking changes and collaborating on projects effectively.
Common Python Applications
Python’s flexibility allows for various applications across different domains.
- Web Development:
- Frameworks like Django and Flask facilitate the creation of robust web applications.
- Data Science and Machine Learning:
- Libraries such as Pandas, NumPy, and scikit-learn support data analysis and modeling.
- Automation and Scripting:
- Python scripts can automate mundane tasks, improving efficiency.
- Game Development:
- Libraries like Pygame enable game creation and development.
- Internet of Things (IoT):
- Used for programming devices and managing data collected from sensors.
By leveraging these features and practices, developers can harness the full potential of Python for diverse applications.
Expert Insights on A.All Python
Dr. Emily Carter (Lead Data Scientist, Tech Innovations Inc.). “A.All Python represents a significant advancement in the way we approach data analysis and machine learning. Its versatility allows for seamless integration of various libraries, making it an essential tool for data scientists today.”
Michael Chen (Senior Software Engineer, CodeCraft Solutions). “The A.All Python framework simplifies complex coding tasks, enabling developers to focus on problem-solving rather than getting bogged down by syntax issues. This efficiency is crucial in fast-paced development environments.”
Laura Patel (Chief Technology Officer, Future Tech Labs). “With A.All Python, organizations can leverage its capabilities to enhance automation processes. This not only increases productivity but also reduces the likelihood of human error in repetitive tasks.”
Frequently Asked Questions (FAQs)
What is Python?
Python is a high-level, interpreted programming language known for its readability and versatility. It supports multiple programming paradigms, including procedural, object-oriented, and functional programming.
What are the main features of Python?
Key features of Python include its simplicity and ease of learning, extensive standard library, dynamic typing, automatic memory management, and strong community support. These features make it suitable for various applications, from web development to data analysis.
How do I install Python on my computer?
To install Python, download the installer from the official Python website (python.org) for your operating system. Run the installer and follow the prompts, ensuring to check the option to add Python to your system PATH.
What are Python libraries, and why are they important?
Python libraries are pre-written code modules that facilitate specific tasks, such as data manipulation, web development, and machine learning. They are important because they save time, promote code reuse, and enhance productivity by providing robust tools and functionalities.
What is the difference between Python 2 and Python 3?
Python 2 and Python 3 differ primarily in syntax and features. Python 3 introduced several improvements, such as better Unicode support, new syntax for print functions, and enhanced standard libraries. Python 2 has reached its end of life, and users are encouraged to transition to Python 3.
Can Python be used for web development?
Yes, Python is widely used for web development. Frameworks such as Django and Flask provide powerful tools for building web applications efficiently. Python’s simplicity and flexibility make it an excellent choice for both backend and frontend development.
In summary, the exploration of “A.All Python” emphasizes the versatility and extensive capabilities of the Python programming language. Python has established itself as a powerful tool across various domains, including web development, data analysis, artificial intelligence, and scientific computing. Its simplicity and readability make it an accessible choice for beginners while also providing advanced features that cater to experienced developers. This duality contributes to Python’s widespread adoption in both educational and professional settings.
Furthermore, the rich ecosystem of libraries and frameworks available in Python significantly enhances its functionality. Libraries such as NumPy and Pandas facilitate data manipulation and analysis, while frameworks like Django and Flask streamline web development. The community support surrounding Python is robust, providing a wealth of resources, tutorials, and forums that foster continuous learning and collaboration among users. This supportive environment is a key factor in Python’s sustained popularity.
Lastly, the future of Python appears bright, with ongoing developments and enhancements that keep pace with evolving technology trends. As industries increasingly rely on data-driven decision-making and automation, Python’s role is likely to expand further. Organizations are encouraged to invest in Python training and development to leverage its full potential, ensuring they remain competitive in an ever-changing technological landscape.
Author Profile
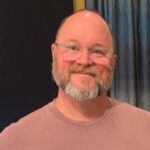
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?