How Can You Effectively Get User Input in Python?
In the realm of programming, the ability to interact with users is a fundamental skill that can elevate your applications from mere code to dynamic experiences. Python, renowned for its simplicity and versatility, provides several straightforward methods for gathering input from users, making it an ideal choice for both beginners and seasoned developers alike. Whether you’re crafting a command-line tool, a data analysis script, or a game, understanding how to effectively capture user input is essential for creating engaging and responsive applications.
When it comes to getting input from users in Python, the language offers a variety of built-in functions and techniques that cater to different needs and scenarios. From simple prompts that request text input to more complex methods that can handle various data types, Python’s capabilities allow developers to tailor user interactions seamlessly. This flexibility not only enhances the user experience but also empowers programmers to build more intuitive and interactive software solutions.
As we delve deeper into the topic, we will explore the various methods available for capturing user input in Python, along with best practices and common pitfalls to avoid. Whether you are looking to gather a single piece of information or create a more intricate dialogue with your users, mastering these input techniques will undoubtedly enhance your programming toolkit and help you create more engaging applications.
Using the input() Function
The primary method to obtain user input in Python is through the `input()` function. This function reads a line from input, typically from the keyboard, and returns it as a string. The `input()` function can also accept a prompt string that is displayed to the user, guiding them on what information is needed.
Here’s a basic example:
“`python
user_input = input(“Please enter your name: “)
print(“Hello, ” + user_input)
“`
In this example, the prompt “Please enter your name: ” is shown to the user, and the entered name is stored in the variable `user_input`.
Type Conversion
Since the `input()` function returns a string, you may need to convert this input into other data types depending on the context of your application. For instance, if you want to get a number from the user, you should convert the string to an integer or a float.
Here are some common conversions:
- Integer: Use `int()` to convert a string to an integer.
- Float: Use `float()` to convert a string to a floating-point number.
Example:
“`python
age = int(input(“Please enter your age: “))
print(“You are ” + str(age) + ” years old.”)
“`
Handling Exceptions
When converting user input, it is essential to handle potential exceptions that may arise, such as a user entering a non-numeric value when a number is expected. Using a try-except block can help manage these errors gracefully.
Example:
“`python
try:
age = int(input(“Please enter your age: “))
print(“You are ” + str(age) + ” years old.”)
except ValueError:
print(“Invalid input! Please enter a numeric value.”)
“`
Advanced Input Techniques
For more complex input scenarios, such as when you need multiple values or specific formats, consider using the following techniques:
- Splitting Input: Use the `.split()` method to allow users to enter multiple values in a single line.
- Using Loops: Create a loop to repeatedly request input until the user provides valid data.
Example of splitting input:
“`python
user_input = input(“Enter your favorite colors separated by commas: “)
colors = user_input.split(“,”)
print(“Your favorite colors are: ” + “, “.join(colors))
“`
Input Validation
Validating user input is crucial for ensuring that the data collected is in the correct format and within expected parameters. You can implement validation by checking conditions before processing the input. Below is a simple table illustrating common validation checks.
Validation Type | Description | Example |
---|---|---|
Numeric Check | Ensure input is a number. | age = int(input(“Enter your age: “)) |
String Length | Check the length of a string input. | if len(name) > 0: … |
Range Check | Check if a numeric input is within a specific range. | if 0 <= age <= 120: ... |
Incorporating these practices in your Python programs will enhance user interaction and ensure that the information collected is reliable and usable.
Using the `input()` Function
The primary method for obtaining user input in Python is through the built-in `input()` function. This function pauses program execution, waiting for the user to enter data via the console. The entered data is returned as a string, which can then be processed as needed.
“`python
user_input = input(“Please enter your name: “)
print(“Hello, ” + user_input + “!”)
“`
This example prompts the user for their name and greets them accordingly.
Data Type Conversion
Since `input()` returns a string, you may need to convert this data to the appropriate type for further processing. Here are common conversions:
- Integer Conversion: Use `int()`
- Float Conversion: Use `float()`
- Boolean Conversion: Use `bool()`
For instance, to capture an integer from the user:
“`python
age = int(input(“Please enter your age: “))
print(“You are ” + str(age) + ” years old.”)
“`
Handling Errors
When converting user input, it’s essential to handle potential errors using `try` and `except` blocks. This prevents the program from crashing due to invalid input.
“`python
try:
age = int(input(“Please enter your age: “))
except ValueError:
print(“That’s not a valid age. Please enter a number.”)
“`
This code snippet ensures that if the user inputs something other than a number, a friendly error message is displayed.
Collecting Multiple Inputs
To collect multiple pieces of input, you can call `input()` multiple times or use a single input prompt and split the result into separate values.
“`python
Multiple calls
name = input(“Enter your name: “)
age = int(input(“Enter your age: “))
Single call
data = input(“Enter your name and age separated by a space: “).split()
name = data[0]
age = int(data[1])
“`
In the single call example, the `split()` method divides the input string into a list based on spaces.
Using Command-Line Arguments
For scenarios where input is provided at runtime rather than interactively, Python offers the `sys.argv` list from the `sys` module. This allows users to pass arguments when running the script.
“`python
import sys
if len(sys.argv) > 1:
print(“Arguments passed:”, sys.argv[1:])
else:
print(“No arguments were passed.”)
“`
Run the script from the command line as follows:
“`bash
python script.py arg1 arg2
“`
This will display the arguments `arg1` and `arg2`.
Input Validation
It is prudent to validate user input to ensure it meets specific criteria. For example, if you require an age that must be a positive integer:
“`python
while True:
try:
age = int(input(“Please enter your age: “))
if age < 0:
raise ValueError("Age cannot be negative.")
break
except ValueError as e:
print(e)
```
This structure ensures that the user continues to be prompted until valid input is received.
By utilizing the `input()` function and implementing data conversion, error handling, and validation techniques, you can effectively gather user input in Python, leading to more robust and user-friendly applications.
Expert Insights on User Input in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “To effectively gather input from users in Python, utilizing the built-in `input()` function is essential. It allows for straightforward interaction, enabling developers to capture user responses dynamically. Ensuring proper validation of this input is equally crucial to maintain the integrity of the program.”
Michael Tran (Lead Software Engineer, CodeCraft Solutions). “When designing user input mechanisms in Python, it is important to consider user experience. Implementing features such as prompts and clear instructions enhances usability. Additionally, using libraries like `argparse` can streamline input handling for command-line applications.”
Sarah Johnson (Python Educator, LearnPythonNow Academy). “In educational contexts, teaching how to get input from users using Python should emphasize the importance of data types. Converting input data to the appropriate type is critical, as user input is inherently a string. Demonstrating this concept through practical examples can significantly aid student understanding.”
Frequently Asked Questions (FAQs)
How do I get input from a user in Python?
You can use the `input()` function to get input from a user in Python. This function reads a line from input, converts it to a string, and returns it.
Can I specify a prompt message when using the input function?
Yes, you can provide a prompt message as an argument to the `input()` function. For example, `input(“Enter your name: “)` will display “Enter your name: ” before waiting for user input.
How do I convert user input to a different data type?
You can convert user input to a different data type by using type conversion functions. For example, to convert input to an integer, use `int(input(“Enter a number: “))`.
What happens if the user enters invalid data?
If the user enters invalid data that cannot be converted to the specified type, Python will raise a `ValueError`. It is advisable to handle this exception using a try-except block to ensure your program runs smoothly.
Is there a way to get multiple inputs from a user in one line?
Yes, you can use the `input()` function to get multiple inputs in one line by prompting the user to separate values with a specific character, such as a comma. You can then split the input string using the `split()` method.
Can I use input from a file instead of user input?
Yes, you can read input from a file using Python’s built-in file handling capabilities. Use the `open()` function to access the file and the `read()` or `readlines()` methods to retrieve the data.
In Python, obtaining user input is a fundamental aspect of creating interactive applications. The primary method for capturing input from the user is through the built-in `input()` function. This function prompts the user for input, which is then returned as a string. It is essential to understand that any data received through `input()` will be in string format, necessitating conversion for numeric or other data types when required.
Another important consideration when handling user input is the implementation of error handling. Utilizing `try` and `except` blocks allows developers to manage exceptions that may arise from invalid input. This practice enhances the robustness of the application, ensuring that it can gracefully handle unexpected user behavior without crashing.
Furthermore, it is beneficial to validate user input to ensure it meets specific criteria before processing. This can be achieved through conditional statements that check the format or value of the input. By implementing such validation, developers can guide users towards providing the correct type of information, thereby improving the overall user experience.
In summary, effectively getting input from users in Python involves using the `input()` function, implementing error handling, and validating the input. Mastering these techniques is crucial for creating user-friendly and resilient applications that can interact with users in
Author Profile
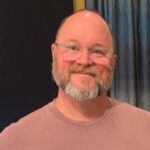
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?