How Can I Change the Center Position of a Rectangle in My Design?
Introduction
In the world of graphic design and digital art, precision is key, especially when it comes to manipulating shapes and objects within a canvas. One fundamental aspect that often goes overlooked is the center position of rectangles, or “rects.” Whether you’re creating a stunning visual layout, developing a user interface, or working on intricate animations, understanding how to change the center position of a rect can significantly enhance your design process. This article delves into the importance of rect positioning, the techniques to adjust it effectively, and the impact it can have on your overall composition.
When working with rectangles in graphic design software or programming environments, the center position serves as a crucial reference point for alignment, rotation, and scaling. By mastering the methods to change this position, designers can achieve greater control over their compositions, ensuring that elements are not only visually appealing but also functionally sound. From simple adjustments to more complex transformations, the ability to manipulate a rect’s center can lead to innovative design solutions and improved user experiences.
Moreover, understanding how to adjust the center position of a rect is not just about aesthetics; it also plays a vital role in the technical aspects of design. For instance, in animation, the center point can determine how an object rotates or scales, affecting the fluidity and
Understanding the Center Position of a Rectangle
In graphical applications, the center position of a rectangle is crucial for various operations such as transformations, animations, and layouts. This center position is typically calculated based on the rectangle’s width and height. The coordinates of the center point can be determined using the following formulas:
- For a rectangle defined by its top-left corner (x, y), width (w), and height (h):
- Center X = x + (w / 2)
- Center Y = y + (h / 2)
These calculations ensure that the center point reflects the geometric center of the rectangle, facilitating accurate positioning and manipulation.
Changing the Center Position Programmatically
To change the center position of a rectangle, adjustments need to be made to its coordinates based on the desired new center point. The following steps outline this process:
- Determine the current center position using the formulas provided.
- Calculate the offset required to move the center to the new position.
- Update the rectangle’s position by adjusting the x and y coordinates accordingly.
For example, if you wish to move the center of a rectangle from its current position (cx, cy) to a new center position (newCx, newCy), the following calculations are employed:
- Offset X = newCx – cx
- Offset Y = newCy – cy
Then, update the rectangle’s top-left corner as follows:
- New X = x + Offset X
- New Y = y + Offset Y
Implementation Example
The implementation can be represented in a table format, showcasing the steps with example values:
Step | Description | Example Values |
---|---|---|
1 | Calculate current center | x = 10, y = 20, w = 40, h = 30 Center X = 10 + (40 / 2) = 30 Center Y = 20 + (30 / 2) = 35 |
2 | Determine new center | newCx = 50, newCy = 50 |
3 | Calculate offsets | Offset X = 50 – 30 = 20 Offset Y = 50 – 35 = 15 |
4 | Update rectangle position | New X = 10 + 20 = 30 New Y = 20 + 15 = 35 |
By following these steps, one can effectively change the center position of a rectangle in any graphical context, ensuring that it remains centered around the desired point.
Practical Considerations
When modifying the center position of a rectangle, consider the following:
- Coordinate System: Ensure you are aware of the coordinate system being used (top-left origin vs. bottom-left origin).
- Aspect Ratio: Maintaining the aspect ratio when resizing may also require adjustments to the center position.
- Performance: Frequent recalculations can impact performance in real-time applications, so consider optimizing by caching values where appropriate.
These considerations will aid in achieving accurate and efficient manipulation of rectangle positions in your graphical projects.
Understanding the Change Center Position of a Rectangle
Changing the center position of a rectangle involves recalculating its coordinates based on the desired center point. The rectangle’s center is typically defined by its width and height, allowing for precise positioning within a coordinate system.
To effectively alter the rectangle’s center position, consider the following parameters:
- Current Center Coordinates: (Cx, Cy)
- New Center Coordinates: (Nx, Ny)
- Width: W
- Height: H
The rectangle’s corner coordinates can be derived using these values. The top-left corner (X1, Y1) and the bottom-right corner (X2, Y2) can be calculated as follows:
- \( X1 = Nx – \frac{W}{2} \)
- \( Y1 = Ny – \frac{H}{2} \)
- \( X2 = Nx + \frac{W}{2} \)
- \( Y2 = Ny + \frac{H}{2} \)
Implementation in Graphics Programming
In graphics programming, the method to change the center position will depend on the specific framework or library in use. Below are examples in several popular frameworks:
- HTML5 Canvas
javascript
function changeCenterPosition(rect, newCenterX, newCenterY) {
rect.x = newCenterX – (rect.width / 2);
rect.y = newCenterY – (rect.height / 2);
}
- Unity (C#)
csharp
void ChangeCenterPosition(RectTransform rectTransform, Vector2 newCenter) {
rectTransform.anchoredPosition = newCenter;
}
- Java Swing
java
void changeCenterPosition(JComponent rect, int newCenterX, int newCenterY) {
int width = rect.getWidth();
int height = rect.getHeight();
rect.setLocation(newCenterX – width / 2, newCenterY – height / 2);
}
Considerations for Center Position Changes
When changing the center position of a rectangle, several factors should be taken into account:
- Coordinate System: Ensure that the coordinate system is understood, as different systems may have varying origins (top-left vs. center-based).
- Aspect Ratio: Maintain the aspect ratio if the rectangle is intended to remain proportional after repositioning.
- Collision Detection: Update any collision detection mechanisms that may rely on the rectangle’s position.
Performance Implications
While changing the center position of a rectangle is generally efficient, consider the following performance implications:
Factor | Impact |
---|---|
Rendering Frequency | Frequent changes may necessitate optimization. |
Object Pooling | Reuse of rectangle objects can enhance performance. |
Batch Processing | Group updates to reduce draw calls. |
Implementing these strategies ensures that the center position change does not adversely affect application performance.
Expert Insights on Changing the Center Position of Rectangles
Dr. Emily Carter (Senior Graphic Designer, Design Innovations Inc.). “Adjusting the center position of a rectangle is crucial in graphic design as it directly influences the visual balance of a layout. By repositioning the center, designers can create more dynamic compositions that draw the viewer’s eye effectively.”
Michael Tran (Software Engineer, UI/UX Solutions). “In programming, particularly in UI design, changing the center position of a rectangle can enhance user interaction. It allows for better alignment with other elements, ensuring a cohesive user experience that is both functional and aesthetically pleasing.”
Sarah Lee (Architectural Designer, Urban Space Architects). “From an architectural perspective, the center position of a rectangle plays a vital role in spatial organization. Adjusting this position can significantly impact the flow of movement within a space, leading to more efficient and engaging environments.”
Frequently Asked Questions (FAQs)
What does it mean to change the center position of a rectangle?
Changing the center position of a rectangle involves adjusting its coordinates so that its center point is relocated to a new specified location on a coordinate plane.
How can I calculate the new center position of a rectangle?
To calculate the new center position, determine the rectangle’s width and height, then add half of the width to the x-coordinate and half of the height to the y-coordinate of the desired center point.
What are common methods for changing the center position of a rectangle in programming?
Common methods include using transformation functions such as translation, adjusting the rectangle’s coordinates directly, or applying a matrix transformation depending on the graphics library or framework in use.
Are there specific tools or libraries that facilitate changing the center position of a rectangle?
Yes, many graphic libraries such as HTML5 Canvas, SVG, and frameworks like p5.js or Processing provide built-in functions to easily manipulate shapes, including changing their center positions.
What considerations should I keep in mind when changing the center position of a rectangle?
Consider the rectangle’s original position, the effect on surrounding elements, and whether the change maintains the intended visual layout or design integrity.
Can changing the center position of a rectangle affect its dimensions?
No, changing the center position does not affect the rectangle’s dimensions; it only alters its location on the coordinate plane while maintaining its width and height.
In summary, changing the center position of a rectangle involves understanding the geometric principles that govern the shape’s coordinates. The center of a rectangle is typically defined as the midpoint of its diagonal, which can be calculated using the coordinates of its vertices. By manipulating these coordinates, one can effectively reposition the rectangle within a given space, ensuring that its dimensions remain unchanged while altering its location.
Moreover, the process of adjusting the center position can be applied in various fields, such as computer graphics, architectural design, and mathematical modeling. It is essential to consider the implications of such changes, particularly how they affect the rectangle’s alignment with other elements in a design or layout. Understanding the mathematical relationships involved allows for precise control over the rectangle’s placement.
Key takeaways include the importance of accurately calculating the new center position and the potential applications of this knowledge in practical scenarios. Whether for aesthetic purposes in design or for functional requirements in programming, the ability to change the center position of a rectangle is a fundamental skill that enhances spatial awareness and geometric manipulation.
Author Profile
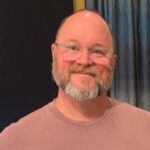
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?