Why Does My Anaconda Encounter a ‘Bytes’ Object Has No Attribute ‘Get’ Error?
In the world of Python programming, especially when working with data science libraries like Anaconda, encountering errors can be a common yet frustrating experience. One such error that often leaves developers scratching their heads is the infamous “`Bytes’ object has no attribute ‘get’`”. This seemingly cryptic message can halt your workflow, leaving you to ponder the intricacies of data types and object attributes. Understanding the root cause of this error is crucial for any programmer aiming to streamline their coding experience and enhance their problem-solving skills.
As you delve into the nuances of this error, you’ll discover that it often arises from a misunderstanding of how different data types interact within Python. The `bytes` object, a fundamental type in Python, is designed for handling binary data, but it doesn’t possess the same methods as more complex data structures like dictionaries. This discrepancy can lead to confusion, particularly for those transitioning from high-level data manipulation to lower-level data handling. By unraveling the specifics of this error, you’ll gain insights that not only help you resolve the issue at hand but also deepen your understanding of Python’s type system.
In this article, we will explore the common scenarios that lead to the “`Bytes’ object has no attribute ‘get’`” error, providing clarity on how to
Understanding the Error
The error message `’Bytes’ object has no attribute ‘get’` typically arises in Python when attempting to call the `get()` method on a bytes object. In Python, the `get()` method is associated with dictionary objects and is not applicable to bytes. This misunderstanding often occurs when handling data, especially in the context of libraries that deal with binary data or network communications.
When you receive this error, it’s essential to identify the object type you are working with. The following scenarios can lead to this error:
- Attempting to access data from a byte stream as if it were a dictionary.
- Mismanaging the data types when processing HTTP responses, where the response body may be in bytes.
To troubleshoot effectively, check the type of the object using the `type()` function. If it is indeed a bytes object, you may need to decode it into a string or convert it into a dictionary format if it represents serialized data.
Common Causes and Solutions
Several common programming practices can lead to this error. Below are a few situations and their corresponding solutions:
- Receiving HTTP Responses: When working with libraries like `requests` in Anaconda, the response content is often in bytes. To convert it to a string, use the `.text` attribute instead of calling `get()`.
“`python
import requests
response = requests.get(‘https://api.example.com/data’)
data = response.content This is in bytes
json_data = response.json() Directly parse JSON without `get()`
“`
- Improper Data Serialization: If you’re serializing data into bytes and then trying to access it like a dictionary, make sure to deserialize it correctly.
“`python
import json
Serializing
data_dict = {‘key’: ‘value’}
byte_data = json.dumps(data_dict).encode(‘utf-8’)
Deserializing
retrieved_dict = json.loads(byte_data.decode(‘utf-8’)) Decode before loading
“`
- Using Libraries: Some libraries may handle data in unexpected formats. Always refer to the library documentation to understand the data types being returned.
Best Practices to Avoid the Error
To prevent encountering the `’Bytes’ object has no attribute ‘get’` error, consider the following best practices:
- Type Checking: Always check the data type before attempting to manipulate it. Use `isinstance()` to confirm the object type.
“`python
if isinstance(data, bytes):
data = data.decode(‘utf-8’) Convert to string
“`
- Proper Response Handling: Use the appropriate attributes for accessing response data, such as `.json()` for JSON responses.
- Debugging: Utilize logging or print statements to output the type and value of variables at different points in your code. This will help identify where the issue may arise.
Error Type | Description | Solution |
---|---|---|
Bytes vs. Dictionary Access | Attempting to use `get()` on a bytes object | Decode bytes or convert to a dictionary |
Improper Serialization | Serialized data accessed incorrectly | Ensure correct encoding/decoding |
Library Misunderstanding | Using library functions without understanding their return types | Consult library documentation |
By adhering to these practices and understanding the nature of bytes objects, you can effectively avoid the pitfalls associated with this error in your Anaconda projects.
Understanding the Error
The error message `Bytes’ object has no attribute ‘Get’` typically arises in Python when attempting to invoke the `Get` method on a bytes object, which does not possess this attribute. This situation is common in data handling, particularly when working with libraries that manage binary data.
Common Scenarios
- Incorrect Data Type: The bytes object is being used where a different type (like a string or a dictionary) is expected.
- Misconfigured API Calls: An API may return data in bytes, requiring conversion before further manipulation.
- Library Functions: Some libraries may return bytes when you expect a more complex object.
Identifying the Source
To resolve this error, consider the following steps:
- Trace the Error Location: Identify where the error occurs in your code. The traceback will provide the exact line number.
- Check Object Types: Use the `type()` function to confirm the data type of the object you are trying to manipulate.
- Review Function Documentation: Ensure that the functions you’re using return the expected types, particularly when interacting with APIs or libraries.
Solutions to Resolve the Error
Here are some strategies to rectify the issue:
Convert Bytes to String
If the intention is to work with a string, decode the bytes object:
“`python
bytes_object = b’example’
string_object = bytes_object.decode(‘utf-8′)
“`
Use Appropriate Methods
Instead of using `Get`, utilize methods that apply to bytes objects. For instance, if you’re trying to extract information from a bytes object, you might use slicing or built-in functions instead.
Example Code
“`python
data = b’example data’
Check if data is bytes
if isinstance(data, bytes):
Convert to string
data_str = data.decode(‘utf-8’)
print(data_str) Now you can use string methods
“`
Debugging Best Practices
When debugging this kind of error, adopt these practices:
- Logging: Implement logging to track the flow and types of data through your functions.
- Unit Tests: Create tests that validate the types of objects being passed around, ensuring they meet expectations.
- Interactive Debugging: Use an interactive debugger to step through the code and inspect the types of objects at runtime.
Common Libraries and Their Behaviors
Understanding the behavior of libraries you frequently use can help mitigate this issue.
Library | Expected Return Type | Notes |
---|---|---|
Requests | Bytes or JSON | Check if response is in bytes; decode if necessary |
NumPy | Arrays | Ensure data types are consistent |
Pandas | DataFrame | When reading files, ensure correct format |
By following these guidelines and understanding the context of the error, you can effectively troubleshoot and resolve the `Bytes’ object has no attribute ‘Get’` error in your Anaconda environment.
Understanding the ‘Bytes’ Object Error in Anaconda
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). The error message ‘Bytes’ object has no attribute ‘get’ typically arises when attempting to access methods that are not applicable to byte objects. In Python, bytes are immutable sequences of bytes, and they do not support dictionary-like methods such as ‘get’. Developers should ensure that they are working with the correct data type, potentially converting bytes to a string or dictionary before attempting to use such methods.
James Liu (Python Developer and Educator, CodeMaster Academy). This error often indicates a misunderstanding of data structures in Python. When handling data in Anaconda, it is crucial to verify the type of the object being manipulated. If you encounter this error, consider using the ‘type()’ function to debug and ensure that you are indeed working with the expected object type, which can prevent similar issues in the future.
Dr. Sarah Patel (Software Engineer, Data Solutions Corp.). Encountering the ‘Bytes’ object has no attribute ‘get’ error in Anaconda can be frustrating, especially for those new to Python. It is essential to remember that bytes are often used for binary data, and when you need to access key-value pairs, you should utilize a dictionary instead. A common solution is to decode the bytes into a string format and then convert it into a dictionary if necessary.
Frequently Asked Questions (FAQs)
What does the error ‘Bytes’ object has no attribute ‘get’ mean?
The error indicates that you are attempting to call the ‘get’ method on a bytes object, which does not support this method. The ‘get’ method is typically associated with dictionaries or similar data structures, not bytes.
How can I resolve the ‘Bytes’ object has no attribute ‘get’ error in Anaconda?
To resolve this error, ensure that you are working with the correct data type. If you need to access data from a bytes object, consider decoding it to a string or converting it to a dictionary if it represents serialized data.
What data types can I use the ‘get’ method on in Python?
In Python, the ‘get’ method is primarily used with dictionaries. It retrieves the value for a specified key, returning a default value if the key is not found. Other data structures, such as pandas DataFrames, also have similar methods for accessing data.
How can I convert a bytes object to a string in Python?
You can convert a bytes object to a string using the ‘decode’ method. For example, `my_bytes.decode(‘utf-8’)` will convert the bytes object to a UTF-8 encoded string.
What are common scenarios that lead to the ‘Bytes’ object has no attribute ‘get’ error?
Common scenarios include mistakenly treating a bytes object as a dictionary, such as when reading data from a file or network response without proper decoding. Always verify the type of the object before using methods specific to other data types.
Can I use the ‘get’ method on a JSON object in Python?
Yes, if you parse a JSON string into a Python dictionary using the `json.loads()` method, you can then use the ‘get’ method to access values by their keys. However, ensure that the original data is in string format before parsing.
The error message “Bytes’ object has no attribute ‘get'” typically arises in Python programming when attempting to call the ‘get’ method on a bytes object. This situation often occurs when developers mistakenly treat a bytes object as a dictionary or similar data structure that supports the ‘get’ method. Understanding the distinction between bytes and other data types is crucial for effective debugging and code development.
In the context of using Anaconda, this error may surface when working with libraries that handle data in various formats, such as JSON or binary files. Developers should ensure that they are manipulating the correct data types and using appropriate methods for the specific data structures they are working with. This highlights the importance of thorough data type checks and understanding the methods available for each type.
Key takeaways from this discussion include the necessity of familiarizing oneself with Python’s data types and their respective attributes and methods. Additionally, employing proper error handling can mitigate the impact of such issues, allowing for more robust and maintainable code. By being vigilant about data types, developers can avoid common pitfalls associated with type mismatches and improve their overall coding practices.
Author Profile
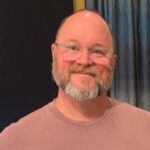
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?