Why Am I Getting ‘Object Of Type Set Is Not JSON Serializable’ Error in Python?
In the world of programming, few things are as frustrating as encountering an error message that halts your progress. One such notorious message that developers often face is the dreaded “Object of Type Set Is Not JSON Serializable.” This cryptic notification can leave even seasoned programmers scratching their heads, as it signals a fundamental issue with how data is being handled in their applications. Understanding this error is crucial for anyone working with JSON, particularly in Python, where the set data type is commonly used.
At its core, JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write, and easy for machines to parse and generate. However, not all Python data types are compatible with JSON serialization. The set type, for instance, is one such data structure that JSON does not natively support. When developers attempt to convert a set into JSON format, they may encounter this error, which serves as a reminder of the importance of understanding data types and their compatibility with serialization processes.
Navigating the intricacies of JSON serialization requires a solid grasp of both the data structures in use and the methods available for converting them. This article will delve into the reasons behind the “Object of Type Set Is Not JSON Serializable” error, explore common scenarios that lead
Understanding JSON Serialization
When working with JSON (JavaScript Object Notation), it is essential to recognize that not all Python objects can be directly converted into JSON format. JSON is primarily designed to support a limited set of data types, which include:
- Strings
- Numbers
- Objects (dictionaries in Python)
- Arrays (lists in Python)
- Booleans
- Null
However, data structures such as sets do not have a direct equivalent in JSON. This limitation leads to the error message: “Object of type set is not JSON serializable.”
Common Causes of the Error
The error typically arises in the following scenarios:
- Direct Serialization: Attempting to serialize a set directly using `json.dumps()`.
- Nested Structures: Sets being part of a larger data structure, such as a list or dictionary, which is being serialized.
- Data Retrieval: Fetching data from a database or API that returns sets, which are then passed to a JSON serialization function.
How to Resolve the Issue
To handle the “Object of type set is not JSON serializable” error, you can convert the set to a JSON-compatible format before serialization. Here are some common strategies:
- Convert to List: The simplest approach is to convert the set to a list. This can be done using the `list()` constructor.
“`python
import json
my_set = {1, 2, 3}
my_list = list(my_set)
json_data = json.dumps(my_list)
“`
- Custom Serialization: If you need to serialize more complex structures that include sets, consider creating a custom serialization function.
“`python
def serialize(obj):
if isinstance(obj, set):
return list(obj)
raise TypeError(f”Type {type(obj)} not serializable”)
my_data = {‘my_set’: {1, 2, 3}}
json_data = json.dumps(my_data, default=serialize)
“`
- Using a Custom Encoder: You can also define a custom JSON encoder that extends `json.JSONEncoder`.
“`python
import json
class CustomEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, set):
return list(obj)
return super().default(obj)
my_set = {1, 2, 3}
json_data = json.dumps(my_set, cls=CustomEncoder)
“`
Comparison of Serialization Techniques
The following table outlines the advantages and disadvantages of different approaches to handle serialization of sets:
Method | Advantages | Disadvantages |
---|---|---|
Convert to List | Simplicity; straightforward usage | May lose the properties of a set (e.g., uniqueness) |
Custom Serialization Function | Flexible; can handle various types | Requires additional code and maintenance |
Custom JSON Encoder | Reusable; integrates well with the `json` module | More complex; may require additional understanding of JSON encoding |
By understanding the limitations of JSON serialization with respect to sets and applying these solutions, developers can effectively manage data serialization without encountering errors.
Understanding the Error
The error message “Object of type set is not JSON serializable” typically arises when attempting to convert a Python set object to a JSON format using the `json` module. JSON (JavaScript Object Notation) does not support sets natively, as it is designed to handle data structures that are common in JavaScript, such as arrays and objects.
Common Scenarios Leading to the Error
This error can occur in various situations, including:
- Direct Serialization: Trying to serialize a set directly.
- Data Structures: Using sets as elements within lists or dictionaries.
- Function Returns: Functions returning sets when JSON serialization is expected.
Example of the Error
Consider the following Python code snippet that leads to the error:
“`python
import json
data = {‘numbers’: {1, 2, 3, 4}} ‘numbers’ contains a set
json_data = json.dumps(data) This will raise a TypeError
“`
In this example, attempting to serialize `data` will result in a `TypeError`.
How to Resolve the Error
To resolve the error, you can convert the set to a list or another JSON-serializable type before serialization. Here are some approaches:
- Convert to List: Change the set to a list.
“`python
data = {‘numbers’: list({1, 2, 3, 4})}
json_data = json.dumps(data)
“`
- Use a Custom Encoder: Implement a custom JSON encoder that can handle sets.
“`python
class SetEncoder(json.JSONEncoder):
def default(self, obj):
if isinstance(obj, set):
return list(obj) Convert set to list
return super().default(obj)
data = {‘numbers’: {1, 2, 3, 4}}
json_data = json.dumps(data, cls=SetEncoder)
“`
Best Practices for JSON Serialization
To avoid serialization issues, consider the following best practices:
- Use Lists or Dictionaries: Prefer lists or dictionaries over sets when dealing with JSON data.
- Data Validation: Validate data structures before serialization to ensure compatibility.
- Custom Serialization Logic: Implement custom serialization methods for complex data types.
Alternative Libraries
In addition to the built-in `json` module, other libraries can handle more complex data types, including sets:
Library | Features |
---|---|
`simplejson` | A fast JSON encoder/decoder that can handle additional types. |
`ujson` | Ultra-fast JSON parsing and serialization. |
`orjson` | High-performance JSON library that supports additional types. |
Utilizing these libraries can help streamline the serialization process and reduce errors related to data types.
Understanding the Challenges of JSON Serialization in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘Object Of Type Set Is Not Json Serializable’ arises because the JSON module in Python does not support the direct serialization of set objects. To resolve this, developers should convert sets to lists or another serializable format before attempting to serialize them.”
Mark Thompson (Lead Data Scientist, Data Solutions Group). “When working with JSON in Python, it’s crucial to understand the data types that can be serialized. Sets, being unordered collections, pose a challenge. I recommend using a custom encoder or a simple conversion to a list to ensure compatibility with JSON serialization.”
Linda Hayes (Technical Writer, Python Programming Journal). “The ‘Object Of Type Set Is Not Json Serializable’ error serves as a reminder for developers to be mindful of data structures. Utilizing built-in functions like list() for conversion can streamline the serialization process and prevent runtime errors.”
Frequently Asked Questions (FAQs)
What does “Object Of Type Set Is Not Json Serializable” mean?
This error indicates that a Python `set` data structure cannot be directly converted to JSON format because JSON does not support the `set` type. JSON serialization requires data types such as lists, dictionaries, strings, numbers, and booleans.
How can I convert a set to a JSON serializable format?
To convert a set to a JSON serializable format, you can transform it into a list using the `list()` function. For example, `json.dumps(list(my_set))` will serialize the set correctly.
What are the common data types that are JSON serializable?
Common JSON serializable data types include strings, numbers (integers and floats), booleans, lists, and dictionaries. Custom objects must be converted to one of these types to be serialized.
Is there a way to serialize a set without converting it to a list?
While the standard `json` module does not support direct serialization of sets, you can create a custom encoder by subclassing `json.JSONEncoder` and defining how to handle sets. However, converting to a list is the simplest method.
What libraries can I use for JSON serialization that support sets?
Libraries such as `simplejson` or `orjson` offer extended support for various data types, including sets. These libraries can handle serialization more flexibly than the standard `json` module.
Can I serialize a set within a dictionary in JSON?
No, you cannot directly serialize a dictionary that contains a set as a value. You must convert the set to a list before serialization. For example, use `json.dumps({ “my_set”: list(my_set) })` to serialize the dictionary correctly.
The error message “Object of type set is not JSON serializable” typically arises when attempting to convert a Python set into a JSON format using the `json` module. JSON, or JavaScript Object Notation, is a widely used data interchange format that only supports specific data types, including dictionaries, lists, strings, numbers, and booleans. Since sets are not part of the JSON specification, they cannot be directly serialized, leading to this error. Understanding this limitation is crucial for developers working with data serialization in Python.
To resolve this issue, developers can convert sets to a compatible data type before serialization. Commonly, this involves transforming the set into a list using the `list()` function. This conversion allows the data to be properly serialized into JSON format. Additionally, it is important to ensure that all elements contained within the set are also JSON serializable. This practice not only prevents errors but also maintains the integrity of the data being processed.
In summary, the “Object of type set is not JSON serializable” error serves as a reminder of the importance of understanding data types in programming. By being aware of the limitations of JSON serialization and implementing appropriate data type conversions, developers can effectively manage data serialization tasks. This knowledge
Author Profile
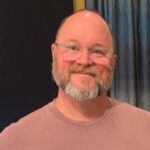
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?