Why Am I Encountering the ‘Error: Jump To Case Label’ in My Code?
In the world of programming, encountering errors is an inevitable part of the development process. Among the myriad of error messages that developers may face, the “Error: Jump To Case Label” stands out as a particularly perplexing one, often leaving even seasoned coders scratching their heads. This error typically arises in languages that utilize switch-case statements, and it can disrupt the flow of execution in a program, leading to confusion and frustration. Understanding the nuances of this error is crucial for any programmer looking to write clean, efficient code.
The “Jump To Case Label” error signifies a violation of the control flow rules within a switch-case structure, where the compiler detects an attempt to jump to a case label inappropriately. This can happen for a variety of reasons, such as improper use of break statements, missing case labels, or even issues with variable scope. As developers delve deeper into the intricacies of their code, recognizing the root cause of this error becomes essential for troubleshooting and debugging.
By exploring the common scenarios that lead to the “Jump To Case Label” error, as well as effective strategies for resolution, programmers can enhance their coding practices and avoid potential pitfalls. This article will guide you through understanding this error, its implications, and how to navigate it with confidence
Error: Jump To Case Label
The `Error: Jump To Case Label` occurs in programming, particularly within languages that utilize the `switch` statement, such as C, C++, and Java. This error indicates that the control flow of the program is trying to jump to a case label that is not valid at the current point in execution. This can happen for several reasons, including improper use of break statements or incorrect structure in the switch-case syntax.
When the control flow reaches a `switch` statement, the program evaluates the expression and jumps to the matching case label. If there are issues with the arrangement of the case labels or if the code attempts to access a case label that is not accessible due to scope or execution flow, the error will be triggered.
Common Causes of the Error
- Lack of Break Statements: Failing to use `break` statements can cause the flow to fall through to subsequent case labels unintentionally.
- Incorrect Case Label Declaration: Declaring case labels that do not conform to the expected types or values can lead to this error.
- Unreachable Code: Code that cannot be executed due to logical errors or improper control flow can result in this error.
- Nested Switch Statements: When switch statements are nested, care must be taken to ensure that each case label is properly scoped.
Example of Incorrect Usage
Consider the following code snippet that leads to the `Jump To Case Label` error:
“`c
int value = 3;
switch (value) {
case 1:
// Do something
break;
case 2:
// Do something
break;
case 3:
// Do something
// No break statement here, leading to unintentional fall-through
case 4:
// Do something for case 4
break;
default:
// Handle unexpected values
}
“`
In this example, if no `break` statement is included after case 3, the execution will fall through to case 4, which may not be intended.
Preventing the Error
To avoid this error, follow these best practices:
- Always use `break` statements after each case to prevent fall-through unless intentional.
- Structure your switch statements clearly, ensuring that each case label is valid and correctly placed.
- Use default cases wisely to handle unexpected values without leading to jumps to incorrect labels.
Troubleshooting Tips
When encountering this error, consider the following troubleshooting steps:
- Review the switch-case structure for any missing `break` statements.
- Check the data types of case labels to ensure they match the switch expression.
- Verify the logical flow of the program to determine if any code is unreachable.
Cause | Solution |
---|---|
Missing Break Statements | Add break statements after each case. |
Invalid Case Labels | Ensure that case labels match the switch expression type. |
Unreachable Code | Refactor the code to remove unreachable segments. |
Nesting Issues | Clarify scope and case labels in nested switches. |
By adhering to these guidelines and understanding the underlying causes of the `Jump To Case Label` error, developers can enhance the reliability of their code and prevent unexpected behavior in their applications.
Error: Jump To Case Label
The “Jump To Case Label” error typically arises in programming languages that utilize switch statements, such as C, C++, and Java. This error indicates that there is a jump in control flow to a case label that is not permitted due to the structure of the code. Understanding the causes and remedies for this error can improve code quality and functionality.
Common Causes
- Improper Use of Case Statements:
- Jumping directly to a case statement without a preceding case or default statement can lead to this error.
- Missing Break Statements:
- If a break statement is omitted, the program may unintentionally “fall through” to the next case, leading to erroneous behavior or compilation errors.
- Invalid Case Labels:
- Using case labels that are not constant expressions or that do not conform to the expected data type can trigger this error.
- Uninitialized Variables:
- Jumping to case labels involving uninitialized variables may lead to unpredictable behavior and errors.
- Control Flow Structures:
- Using control flow structures improperly, such as trying to jump from a loop or if-else structure to a case label, can also result in this error.
Debugging Techniques
- Code Review:
- Conduct a thorough review of the switch-case structure to ensure all case labels are correctly defined.
- Compiler Warnings:
- Pay attention to compiler warnings that may point out potential issues with case statements.
- Add Break Statements:
- Ensure that each case statement ends with a break statement, unless intentional fall-through is needed.
- Use of Default Case:
- Implement a default case to handle unexpected input, which can help catch errors before they escalate.
- Static Analysis Tools:
- Utilize static code analyzers that can identify potential control flow issues within the codebase.
Example Scenario
Consider the following code snippet that demonstrates the “Jump To Case Label” error:
“`c
switch (value) {
case 1:
// Action for case 1
break;
case 2:
// Action for case 2
// Missing break; leads to fall-through error
case 3:
// Action for case 3
break;
default:
// Action for default
}
“`
In this example, the omission of the break statement after case 2 will cause the program to execute case 3 immediately after case 2, potentially leading to unexpected behavior.
Preventive Measures
To prevent the “Jump To Case Label” error from occurring:
- Maintain Consistent Formatting:
- Use consistent indentation and formatting to enhance readability and reduce errors in flow control.
- Document Code:
- Comment on complex switch-case logic to clarify intended flow and behavior to other developers.
- Testing:
- Regularly test the switch-case logic with a variety of inputs to ensure all cases are handled appropriately.
- Refactoring:
- If a switch statement becomes complex, consider refactoring it into separate functions or using a map/dictionary structure for better clarity.
By adhering to these guidelines, developers can effectively manage and reduce the incidence of the “Jump To Case Label” error in their code.
Understanding the ‘Jump To Case Label’ Error in Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘Jump To Case Label’ error typically arises when there is an attempt to jump to a label that is not defined within the current scope. This often occurs in switch-case statements when a developer mistakenly omits a case or fails to properly structure the control flow.”
Mark Thompson (Lead Developer, CodeSecure Solutions). “To effectively resolve the ‘Jump To Case Label’ error, it is crucial to ensure that all case labels are correctly defined and that the control flow adheres to the language’s syntax rules. This error can lead to unpredictable behavior if not addressed promptly.”
Sarah Lee (Programming Instructor, Advanced Coding Academy). “Students often encounter the ‘Jump To Case Label’ error when they are new to programming. It serves as an important lesson in understanding the significance of scope and proper label management in control structures.”
Frequently Asked Questions (FAQs)
What does the error “Jump To Case Label” mean?
The “Jump To Case Label” error typically occurs in programming languages like C or C++ when the control flow attempts to jump to a case label in a switch statement without a proper preceding case or default label.
What causes the “Jump To Case Label” error?
This error is often caused by attempting to use a goto statement to jump into a switch-case structure, which violates the language’s rules regarding control flow and scope.
How can I resolve the “Jump To Case Label” error?
To resolve this error, ensure that any jumps made with goto statements do not target case labels directly. Instead, refactor the code to maintain proper control flow without violating the language’s constraints.
Is the “Jump To Case Label” error specific to certain programming languages?
Yes, this error is primarily associated with languages like C and C++. Other languages may have different mechanisms for handling switch-case statements and may not exhibit this error.
Can this error be avoided through coding best practices?
Yes, adhering to coding best practices, such as avoiding the use of goto statements and maintaining clear control flow, can significantly reduce the likelihood of encountering the “Jump To Case Label” error.
Are there any tools to help identify the “Jump To Case Label” error in my code?
Many integrated development environments (IDEs) and static analysis tools can help identify this error by analyzing the code structure and flow, providing warnings or errors during compilation.
The “Error: Jump To Case Label” is a common issue encountered in programming, particularly in languages that utilize switch-case statements. This error typically arises when the control flow of the program attempts to jump to a case label that is not valid or is unreachable due to the structure of the code. Understanding the underlying causes of this error is crucial for developers to maintain code integrity and functionality.
One of the primary reasons for this error is the improper use of break statements, which are essential for terminating a case in a switch statement. If break statements are omitted, the program may inadvertently fall through to subsequent case labels, leading to unexpected behavior or errors. Additionally, the error can occur if there are mismatched data types or if the case labels are not properly defined, highlighting the importance of careful coding practices.
To avoid the “Jump To Case Label” error, developers should ensure that all case labels are reachable and that the control flow is logically sound. Implementing thorough testing and debugging practices can help identify potential issues before they manifest as errors. Furthermore, adhering to best practices in coding, such as using clear and consistent case labels and properly managing control flow, can significantly reduce the likelihood of encountering this error in future projects.
Author Profile
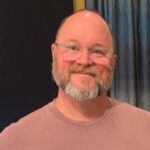
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?