How Can You Effectively Remove Columns from a DataFrame in Python?
In the world of data analysis, Python has emerged as a powerhouse, particularly with the advent of libraries like Pandas that streamline data manipulation tasks. One common challenge that data analysts and scientists face is managing the structure of their datasets. As you delve deeper into your data exploration journey, you may find that certain columns in your DataFrame are no longer relevant or necessary for your analysis. Whether it’s due to redundancy, irrelevance, or simply a need for clarity, knowing how to efficiently remove columns from a DataFrame is an essential skill that can enhance your workflow and improve the quality of your insights.
Removing columns from a DataFrame in Python is a straightforward process, yet it can significantly impact the effectiveness of your analysis. By eliminating extraneous data, you not only streamline your dataset but also make it easier to visualize and interpret the information that truly matters. This task can be accomplished using various methods provided by the Pandas library, each offering flexibility depending on your specific needs. Understanding these methods will empower you to tailor your datasets to better suit your analytical objectives.
As we explore the techniques for removing columns from a DataFrame, you’ll discover practical examples and best practices that can be applied to your own projects. Whether you’re cleaning up a dataset for a machine learning model or simply refining
Removing Columns by Column Name
To remove columns from a DataFrame in Python, you can specify the column names directly. The `drop` method is particularly useful for this purpose. Here’s how you can do it:
“`python
import pandas as pd
Sample DataFrame
data = {
‘A’: [1, 2, 3],
‘B’: [4, 5, 6],
‘C’: [7, 8, 9]
}
df = pd.DataFrame(data)
Dropping a single column by name
df_dropped = df.drop(‘B’, axis=1)
“`
This code snippet will remove column ‘B’ from the DataFrame. The `axis=1` argument specifies that you are dropping columns, while `axis=0` would refer to rows.
For multiple columns, you can pass a list of names:
“`python
Dropping multiple columns
df_dropped_multiple = df.drop([‘A’, ‘C’], axis=1)
“`
Removing Columns by Column Index
In scenarios where you know the index of the columns you want to remove rather than their names, you can also drop columns by index. The `iloc` method can be used to achieve this:
“`python
Dropping a column by index
df_dropped_by_index = df.drop(df.columns[[0, 2]], axis=1) Drops columns at index 0 and 2
“`
This approach is particularly useful when working with DataFrames where the column names may not be known or are dynamic.
Using the `del` Statement
Another approach to remove a column is by using the `del` statement. This method is straightforward and is useful when you want to remove a single column:
“`python
Deleting a column using del
del df[‘B’]
“`
After executing this code, column ‘B’ will be removed from the DataFrame without the need for creating a new DataFrame object.
Using the `pop` Method
The `pop` method allows you to remove a column while also returning it, which can be useful if you need to use the data before discarding it:
“`python
Popping a column
popped_column = df.pop(‘B’)
“`
This will remove column ‘B’ and store its contents in the variable `popped_column`.
Summary of Methods to Remove Columns
Here is a summary table of different methods to remove columns from a DataFrame:
Method | Usage | Returns |
---|---|---|
drop() | df.drop(‘column_name’, axis=1) | New DataFrame without the specified columns |
del | del df[‘column_name’] | None (modifies in place) |
pop() | df.pop(‘column_name’) | Removed column as a Series |
iloc | df.drop(df.columns[[index]], axis=1) | New DataFrame without the specified index columns |
These methods provide flexibility depending on whether you prefer to manipulate the DataFrame in place or create a new instance.
Removing Columns Using the `drop()` Method
The most common way to remove columns from a DataFrame in Python is by using the `drop()` method provided by the pandas library. This method allows for flexible column removal by specifying either column names or index positions.
To use `drop()` effectively:
- Syntax:
“`python
DataFrame.drop(labels, axis=1, inplace=)
“`
- `labels`: Column name(s) to remove.
- `axis=1`: Indicates that columns are being dropped (use `axis=0` for rows).
- `inplace`: If `True`, modifies the DataFrame in place; otherwise, returns a new DataFrame.
- Example:
“`python
import pandas as pd
df = pd.DataFrame({
‘A’: [1, 2, 3],
‘B’: [4, 5, 6],
‘C’: [7, 8, 9]
})
df.drop(‘B’, axis=1, inplace=True)
print(df)
“`
This will result in the following DataFrame:
“`
A C
0 1 7
1 2 8
2 3 9
“`
Removing Multiple Columns
To remove multiple columns simultaneously, simply pass a list of column names to the `drop()` method.
- Example:
“`python
df.drop([‘A’, ‘C’], axis=1, inplace=True)
print(df)
“`
The resulting DataFrame will be empty if both columns are removed.
Using Column Indexing
Another method to remove columns is through column indexing. This approach is particularly useful when you know the index positions of the columns to be removed.
- Example:
“`python
df = pd.DataFrame({
‘A’: [1, 2, 3],
‘B’: [4, 5, 6],
‘C’: [7, 8, 9]
})
df = df[[df.columns[i] for i in range(len(df.columns)) if i != 1]]
print(df)
“`
This will yield the same result as dropping column ‘B’.
Using `del` Statement
The `del` statement provides a straightforward way to remove a column by name.
- Example:
“`python
del df[‘A’]
print(df)
“`
After executing this, if ‘A’ is removed, the DataFrame will show only the remaining columns.
Using `pop()` Method
The `pop()` method can be used to remove a column and return it as a Series.
- Example:
“`python
column_b = df.pop(‘B’)
print(column_b)
print(df)
“`
This not only removes column ‘B’ but also allows you to store it separately for further use.
Conditional Column Removal
Sometimes, you may need to remove columns based on certain conditions, such as column names or data types.
- Example:
“`python
cols_to_remove = [col for col in df.columns if ‘A’ in col]
df.drop(cols_to_remove, axis=1, inplace=True)
“`
This approach provides a flexible method to filter which columns to remove based on specific criteria, enhancing the DataFrame management in data preprocessing tasks.
Expert Insights on Removing Columns from a Dataframe in Python
Dr. Emily Carter (Data Scientist, Analytics Innovations). “When removing columns from a dataframe in Python, it is essential to consider the impact on your dataset’s integrity. Utilizing the `drop()` method is highly effective, but ensure you are aware of the axis parameter to avoid unintentional data loss.”
Michael Chen (Senior Python Developer, Tech Solutions Corp). “I recommend using the `del` statement for a more straightforward approach when you need to remove a single column. This method is not only efficient but also enhances code readability, especially for those new to Python.”
Sarah Thompson (Machine Learning Engineer, Data Insights LLC). “In scenarios where multiple columns need to be removed, leveraging the `drop()` method with a list of column names is the best practice. This approach allows for cleaner code and reduces the likelihood of errors during data manipulation.”
Frequently Asked Questions (FAQs)
How can I remove a single column from a DataFrame in Python?
You can remove a single column from a DataFrame using the `drop()` method. For example, `df.drop(‘column_name’, axis=1, inplace=True)` will remove the specified column from the DataFrame `df`.
What is the difference between `drop()` and `del` for removing columns?
The `drop()` method returns a new DataFrame without the specified column, while `del` modifies the original DataFrame in place. Use `del df[‘column_name’]` to remove a column directly.
Can I remove multiple columns at once from a DataFrame?
Yes, you can remove multiple columns by passing a list of column names to the `drop()` method. For instance, `df.drop([‘col1’, ‘col2’], axis=1, inplace=True)` will remove both specified columns.
What happens if I try to remove a column that does not exist?
If you attempt to remove a non-existent column using `drop()`, it will raise a `KeyError`. To avoid this, you can set the `errors` parameter to `’ignore’`, like `df.drop(‘non_existent_col’, axis=1, errors=’ignore’)`.
Is it possible to remove columns based on a condition?
Yes, you can filter columns based on a condition by using boolean indexing. For example, you can select columns whose names meet certain criteria and then drop them accordingly.
How can I remove columns with missing values?
To remove columns with missing values, use the `dropna()` method with the `axis` parameter set to 1. For instance, `df.dropna(axis=1, inplace=True)` will remove any columns that contain at least one missing value.
In Python, removing columns from a DataFrame is a fundamental operation that can significantly streamline data analysis and manipulation. The primary method to achieve this is by utilizing the `drop()` function from the Pandas library, which allows users to specify the columns they wish to remove. This function can be used in various ways, including by passing column names directly, using a list of names, or even specifying the axis parameter to indicate that columns, rather than rows, are being targeted.
Another important aspect to consider is the impact of the `inplace` parameter. By setting `inplace=True`, changes are applied directly to the original DataFrame without the need to assign the result to a new variable. Alternatively, if `inplace=`, which is the default setting, the operation returns a new DataFrame with the specified columns removed. This flexibility allows users to choose the method that best fits their workflow and data management preferences.
Additionally, it is essential to handle potential errors that may arise when attempting to remove non-existent columns. Utilizing the `errors=’ignore’` parameter can prevent the function from raising an error if a specified column is not found, thus enhancing the robustness of the code. Understanding these nuances not only improves efficiency but also
Author Profile
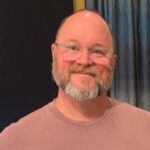
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?