How Can I Handle Async Calls in a Function That Doesn’t Support Concurrency?
In the fast-paced world of software development, the demand for efficient, responsive applications has never been greater. Asynchronous programming has emerged as a powerful paradigm, allowing developers to handle multiple tasks simultaneously without blocking the main thread. However, navigating the complexities of async calls can be challenging, especially when they are invoked within functions that do not inherently support concurrency. This article delves into the intricacies of this common pitfall, equipping you with the knowledge to avoid potential pitfalls and optimize your code for better performance.
When working with asynchronous functions, understanding the underlying mechanics of how they interact with synchronous code is crucial. Functions that are not designed to handle concurrency can lead to unexpected behaviors, such as race conditions or deadlocks, which can severely impact application reliability. By recognizing the limitations of these functions, developers can make informed decisions about structuring their code, ensuring that async calls are made in appropriate contexts.
Moreover, this discussion will explore best practices for managing async calls effectively, including techniques for refactoring existing code and leveraging modern programming constructs. As we unpack the nuances of async calls in non-concurrent functions, you’ll gain insights into how to enhance your application’s responsiveness while maintaining clarity and maintainability in your codebase. Prepare to dive deep into the world of asynchronous programming and emerge with strategies
Understanding Async Calls
When dealing with asynchronous programming, particularly in languages like JavaScript and Python, it is crucial to understand how async calls operate within the context of functions that do not support concurrency. An async call allows for non-blocking operations, enabling programs to handle other tasks while waiting for a promise to resolve. However, if a function is not designed to accommodate asynchronous behavior, invoking an async call can lead to unexpected results or runtime errors.
Challenges of Async Calls in Synchronous Functions
The primary challenge with making async calls within synchronous functions is that the latter does not have the capability to pause execution for asynchronous tasks to complete. This can result in:
- Race Conditions: When the timing of events affects the program’s behavior, leading to inconsistent states.
- Unhandled Promises: If an async function is called without proper error handling, it can lead to unhandled promise rejections.
- Logic Errors: Synchronous functions may not properly account for the asynchronous nature, leading to incorrect assumptions about the order of execution.
To illustrate these challenges, consider the following code example in JavaScript:
“`javascript
function syncFunction() {
const result = asyncFunction(); // This is an async call
console.log(result); // Logs a promise instead of the expected result
}
async function asyncFunction() {
return “Async Result”;
}
“`
In this code, `syncFunction` calls `asyncFunction`, but it does not await the result. Consequently, `result` holds a promise rather than the expected string.
Best Practices for Handling Async Calls
To effectively manage async calls within a synchronous context, adhere to the following best practices:
- Use Async/Await: Transform synchronous functions to async functions when they need to handle asynchronous tasks.
- Error Handling: Implement try-catch blocks around async calls to catch errors gracefully.
- Promise Chaining: If async functions cannot be converted, consider using promise chaining (`.then()`) to handle the resolution of promises.
Here’s an example of how to modify the previous function to support async calls properly:
“`javascript
async function syncFunction() {
try {
const result = await asyncFunction(); // Await the result
console.log(result); // Logs “Async Result”
} catch (error) {
console.error(“Error:”, error);
}
}
“`
Common Error Messages
When async calls are improperly handled, developers may encounter specific error messages. Below is a table summarizing common errors and their implications:
Error Message | Implication |
---|---|
UnhandledPromiseRejectionWarning | Indicates a promise was rejected without a corresponding catch handler. |
TypeError: Cannot read property ‘then’ of | Suggests an attempt to call `.then()` on an object, often due to not returning a promise. |
ReferenceError: variable is not defined | Occurs when a variable referenced within an async context is not properly scoped or initialized. |
By understanding these challenges and employing best practices, developers can effectively manage async calls in their applications, ensuring smoother and more predictable behavior across their codebases.
Understanding Async Calls in Non-Concurrent Functions
When integrating asynchronous calls in functions that do not support concurrency, it is essential to grasp the implications and potential pitfalls. Non-concurrent functions typically execute in a single-threaded manner, making them unsuitable for direct async operations. The following sections highlight the critical considerations and strategies for managing such scenarios effectively.
Challenges of Async Calls
Using asynchronous calls in non-concurrent functions presents several challenges, including:
- Blocking Behavior: The non-concurrent function may block the event loop, negating the benefits of asynchronous processing.
- Error Handling: Asynchronous operations often rely on promises, which can complicate error handling within non-concurrent contexts.
- State Management: Maintaining the function’s state becomes more complex when async calls are interleaved with synchronous logic.
Workarounds for Async Integration
To effectively incorporate asynchronous calls into non-concurrent functions, consider the following strategies:
– **Callback Functions**: Use callbacks to handle the results of async operations. This allows the function to remain non-blocking while still processing async results.
“`javascript
function nonConcurrentFunction(callback) {
asyncOperation(result => {
callback(result);
});
}
“`
– **Promises**: Leverage promises to manage the flow of asynchronous operations. While the main function remains synchronous, it can return a promise to indicate completion.
“`javascript
function nonConcurrentFunction() {
return new Promise((resolve, reject) => {
asyncOperation(result => {
resolve(result);
});
});
}
“`
– **IIFE (Immediately Invoked Function Expression)**: Encapsulate the async call within an IIFE to maintain the non-concurrent nature of the outer function.
“`javascript
function nonConcurrentFunction() {
(async () => {
const result = await asyncOperation();
console.log(result);
})();
}
“`
Best Practices for Handling Async in Non-Concurrent Contexts
To avoid common pitfalls when working with async operations in a non-concurrent environment, adhere to these best practices:
- Keep Async Logic Separate: Maintain clear separation between synchronous and asynchronous logic to simplify debugging and enhance readability.
- Error Propagation: Ensure that errors from async calls are propagated correctly through callbacks or promises.
- Avoid Deep Nesting: Minimize nested async calls to prevent callback hell, which can make code difficult to follow.
Performance Considerations
When implementing async calls in non-concurrent functions, consider the following performance implications:
Factor | Impact on Performance |
---|---|
Event Loop Blocking | Reduced responsiveness, longer wait times for users |
Resource Utilization | Increased memory usage due to promise overhead |
Context Switching | Potential overhead if using multiple async operations |
By being mindful of these factors, developers can optimize their applications while effectively managing asynchronous calls within non-concurrent functions.
Understanding the Challenges of Async Calls in Non-Concurrent Functions
Dr. Emily Chen (Software Architect, Tech Innovations Inc.). “Using async calls in functions that do not support concurrency can lead to unexpected behavior and performance bottlenecks. It is essential to ensure that the underlying function can handle asynchronous execution; otherwise, you may encounter race conditions or deadlocks that compromise application stability.”
Mark Thompson (Lead Developer, CodeCraft Solutions). “When integrating async calls into synchronous functions, developers must be cautious. The lack of concurrency support means that the async operations may not execute as intended, potentially leading to unhandled promises and degraded user experience. It is advisable to refactor the function to support asynchronous processing properly.”
Linda Patel (Principal Engineer, FutureTech Labs). “The integration of async calls into non-concurrent functions can complicate debugging and error handling. Developers should consider using patterns such as callbacks or Promises to manage the flow of execution effectively, ensuring that all paths are accounted for and that the application behaves predictably.”
Frequently Asked Questions (FAQs)
What does it mean when a function does not support concurrency?
A function that does not support concurrency is designed to execute operations sequentially, meaning it cannot handle multiple tasks or requests simultaneously. This limitation can lead to performance bottlenecks in applications requiring asynchronous processing.
Can I use an async call inside a non-concurrent function?
Yes, you can initiate an async call within a non-concurrent function; however, the function itself will not wait for the async operation to complete before continuing execution. This can lead to unhandled promises or unexpected behavior if not managed properly.
What are the risks of making async calls in non-concurrent functions?
Making async calls in non-concurrent functions can result in race conditions, unhandled exceptions, and difficulty in managing the flow of execution. It may also lead to resource leaks if the async operation is not properly awaited or handled.
How can I handle async calls in a function that does not support concurrency?
To handle async calls in such functions, you can use callbacks, promises, or event listeners to manage the flow of execution. Additionally, consider refactoring the function to support concurrency if possible, or using a separate asynchronous function to manage the async operation.
What is the best practice for using async calls in synchronous code?
The best practice is to refactor the synchronous code to be asynchronous if it needs to handle async calls. If refactoring is not feasible, ensure that async calls are properly managed using promises or callbacks to avoid blocking the main execution thread.
Are there tools or libraries that can help with async calls in non-concurrent functions?
Yes, libraries such as `async.js` or `Promise.all` can help manage async calls effectively. These tools provide mechanisms for handling asynchronous operations, allowing better control over execution flow and error handling in non-concurrent contexts.
In programming, particularly in languages that support asynchronous operations, developers often encounter scenarios where they need to call an asynchronous function within a context that does not support concurrency. This situation can lead to complications, as traditional synchronous functions are not designed to handle the non-blocking nature of async calls. Understanding how to navigate these challenges is crucial for maintaining efficient and error-free code.
One of the main points to consider is the importance of recognizing the limitations of synchronous functions. When an async function is invoked in a synchronous context, it may not execute as intended, potentially leading to unexpected behavior or runtime errors. Developers must be aware of these limitations and consider refactoring their code to accommodate asynchronous programming paradigms, such as using promises or async/await syntax where applicable.
Additionally, it is essential to explore alternative strategies for handling async calls in synchronous functions. One approach is to encapsulate the async call within a separate function that can manage the async workflow, allowing for better control over execution flow. Another strategy is to utilize callback functions or event-driven programming techniques to ensure that the asynchronous operations are executed properly without blocking the main thread.
effectively managing async calls in functions that do not support concurrency requires a solid understanding of both
Author Profile
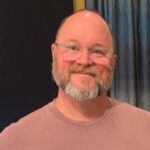
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?