How Can You Change CSS with JavaScript for Dynamic Web Design?
In the dynamic world of web development, the ability to manipulate styles on the fly can significantly enhance user experience and interactivity. Imagine a scenario where your website responds to user actions in real-time, changing colors, layouts, or even animations with just a click or a hover. This is where the power of JavaScript comes into play, allowing developers to seamlessly alter CSS properties and create engaging, responsive designs. Whether you’re looking to add a touch of flair to your site or implement complex functionality, understanding how to change CSS with JavaScript is an essential skill in your toolkit.
Changing CSS with JavaScript opens up a realm of possibilities for web designers and developers alike. By leveraging the Document Object Model (DOM), you can access and modify styles directly, creating dynamic effects that can captivate users and keep them engaged. From simple tasks like changing the background color of a button on hover to more complex operations like animating elements during a page scroll, the integration of JavaScript and CSS can lead to a more vibrant and interactive web experience.
As we delve deeper into this topic, we will explore various methods to manipulate CSS properties using JavaScript, including inline styles, class manipulation, and the use of CSS variables. Whether you’re a seasoned developer or just starting your journey in web
Accessing and Modifying CSS Properties
To change CSS properties using JavaScript, you can access the DOM elements and manipulate their styles directly. The `style` property of an element provides a way to set individual CSS properties inline. Here’s how you can accomplish this:
- Use `document.getElementById()` or other DOM selection methods to obtain a reference to the element you want to modify.
- Access the `style` property of the element and set the CSS properties as needed.
For example:
“`javascript
var element = document.getElementById(“myElement”);
element.style.color = “red”; // Changes text color to red
element.style.backgroundColor = “blue”; // Changes background color to blue
“`
In this case, the properties are set directly using camelCase for CSS properties that normally use hyphens.
Changing Multiple CSS Properties
When you need to change multiple CSS properties at once, you can do so by chaining the assignments or using a loop for better organization. Here’s an example of changing multiple properties:
“`javascript
var element = document.getElementById(“myElement”);
element.style.cssText = “color: red; background-color: blue; font-size: 16px;”; // Multiple properties at once
“`
Alternatively, if you have a set of properties stored in an object, you can loop through them:
“`javascript
var styles = {
color: “red”,
backgroundColor: “blue”,
fontSize: “16px”
};
for (var property in styles) {
element.style[property] = styles[property];
}
“`
Using Classes to Change CSS
Instead of modifying individual CSS properties, you can also add or remove classes from elements, which can be a more efficient way to handle styles defined in a stylesheet. This allows for cleaner JavaScript and better separation of style and behavior.
- To add a class:
“`javascript
element.classList.add(“newClass”);
“`
- To remove a class:
“`javascript
element.classList.remove(“oldClass”);
“`
- To toggle a class:
“`javascript
element.classList.toggle(“toggleClass”);
“`
This method is particularly useful when you want to apply predefined styles without cluttering your JavaScript with numerous style changes.
Example Table of Common CSS Properties and JavaScript Syntax
CSS Property | JavaScript Syntax |
---|---|
Color | element.style.color = “value”; |
Background Color | element.style.backgroundColor = “value”; |
Font Size | element.style.fontSize = “value”; |
Margin | element.style.margin = “value”; |
Display | element.style.display = “value”; |
By utilizing these methods, you can effectively manage CSS styles through JavaScript, enhancing the interactivity and responsiveness of your web applications.
Manipulating Inline Styles
To change CSS directly using JavaScript, one of the simplest methods is manipulating the inline styles of an HTML element. This approach allows you to modify specific styles without affecting the CSS rules defined in stylesheets.
“`javascript
const element = document.getElementById(‘myElement’);
element.style.color = ‘blue’;
element.style.fontSize = ’20px’;
“`
In this example, the color and font size of the element with the ID `myElement` are changed to blue and 20 pixels, respectively.
Changing Class Attributes
Another effective way to alter CSS is by adding or removing class names. This method is beneficial when you want to apply predefined styles from your CSS.
“`javascript
const element = document.getElementById(‘myElement’);
element.classList.add(‘newClass’); // Adds a class
element.classList.remove(‘oldClass’); // Removes a class
“`
Using `classList` provides several useful methods:
- `add(className)` – Adds the specified class.
- `remove(className)` – Removes the specified class.
- `toggle(className)` – Toggles the class on or off.
- `contains(className)` – Checks if the class is present.
Accessing CSS Stylesheets
You can also change styles defined in external CSS stylesheets using JavaScript by accessing the `styleSheets` property of the document. This approach allows for dynamic adjustments at a broader level.
“`javascript
const sheet = document.styleSheets[0]; // Access the first stylesheet
sheet.insertRule(‘.newClass { background-color: yellow; }’, sheet.cssRules.length);
“`
This code snippet adds a new rule to the first stylesheet, setting the background color of elements with the class `newClass` to yellow.
Using CSS Variables
CSS variables, also known as custom properties, can be modified using JavaScript, allowing you to change multiple styles simultaneously.
“`javascript
document.documentElement.style.setProperty(‘–main-color’, ‘green’);
“`
This line sets the `–main-color` variable to green, which can be utilized throughout your CSS.
CSS Variable | Description |
---|---|
`–main-color` | Primary color scheme |
`–font-size` | Base font size |
`–spacing` | General spacing units |
Event-Driven Style Changes
JavaScript can also respond to user actions, adjusting styles based on events such as clicks or hovers.
“`javascript
const button = document.getElementById(‘changeStyleButton’);
button.addEventListener(‘click’, () => {
document.getElementById(‘myElement’).style.backgroundColor = ‘lightblue’;
});
“`
In this example, clicking the button changes the background color of `myElement` to light blue.
Performance Considerations
When dynamically changing styles, consider the following factors to maintain performance:
- Batch DOM Manipulations: Group multiple style changes to minimize reflows.
- Use Classes Over Inline Styles: Utilize class manipulations rather than directly setting styles for better maintainability.
- Limit Access to Stylesheets: Frequent access to stylesheets can degrade performance; cache references when possible.
By employing these strategies, you can effectively manage and modify CSS styles using JavaScript while ensuring optimal performance.
Expert Insights on Changing CSS with JavaScript
Emily Chen (Front-End Developer, Tech Innovations Inc.). “Utilizing JavaScript to modify CSS allows for dynamic styling that enhances user experience. By manipulating the DOM, developers can create responsive designs that adapt to user interactions in real-time.”
James Patel (Web Development Instructor, Code Academy). “One of the most powerful features of JavaScript is its ability to change CSS properties on the fly. This capability not only improves the visual appeal of web applications but also facilitates better performance by reducing the need for page reloads.”
Linda Gomez (UI/UX Designer, Creative Solutions Ltd.). “Incorporating JavaScript to alter CSS can significantly elevate the interactivity of a website. By leveraging event listeners, designers can implement animations and transitions that respond to user actions, creating a more engaging environment.”
Frequently Asked Questions (FAQs)
How can I change the CSS of an element using JavaScript?
You can change the CSS of an element using the `style` property in JavaScript. For example, `document.getElementById(‘myElement’).style.color = ‘red’;` changes the text color of the element with the ID ‘myElement’ to red.
Is it possible to add a new CSS class to an element with JavaScript?
Yes, you can add a new CSS class to an element using the `classList` property. For instance, `document.getElementById(‘myElement’).classList.add(‘newClass’);` adds the ‘newClass’ to the element with the ID ‘myElement’.
Can I remove a CSS class from an element using JavaScript?
Absolutely. You can remove a CSS class using the `classList.remove()` method. For example, `document.getElementById(‘myElement’).classList.remove(‘oldClass’);` removes the ‘oldClass’ from the specified element.
How do I toggle a CSS class on an element with JavaScript?
You can toggle a CSS class using the `classList.toggle()` method. For example, `document.getElementById(‘myElement’).classList.toggle(‘active’);` adds the ‘active’ class if it is not present and removes it if it is.
What is the difference between inline styles and CSS classes when changing styles with JavaScript?
Inline styles are applied directly to an element using the `style` property, which overrides any external or internal styles. CSS classes, on the other hand, allow for more organized and reusable styles, enabling you to apply multiple styles at once.
Can I change multiple CSS properties at once using JavaScript?
Yes, you can change multiple CSS properties at once by assigning a string to the `style.cssText` property. For example, `document.getElementById(‘myElement’).style.cssText = ‘color: blue; background-color: yellow;’;` changes both the text color and background color simultaneously.
changing CSS with JavaScript is a powerful technique that allows developers to create dynamic and responsive web applications. By manipulating the Document Object Model (DOM), JavaScript can be used to alter styles directly or modify class names, providing a flexible approach to styling elements based on user interactions or other conditions. Understanding the various methods available, such as using the `style` property, `classList` API, or frameworks like jQuery, is essential for effective implementation.
One of the key takeaways is the importance of performance considerations when dynamically changing CSS. Frequent style changes can lead to reflows and repaints, which may affect the overall performance of a web page. Therefore, it is advisable to batch DOM manipulations and minimize direct style changes where possible. Utilizing CSS transitions and animations can also enhance user experience while maintaining performance.
Additionally, maintaining a clear separation between JavaScript and CSS is crucial for the long-term maintainability of code. By using classes to define styles and JavaScript to toggle these classes, developers can keep their code organized and easier to manage. This approach not only improves readability but also allows for better collaboration among team members, as the roles of styling and behavior are clearly delineated.
Author Profile
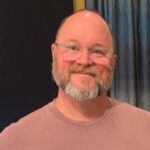
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?