How Can You Effectively Send Messages to a Network Interface?
In our increasingly interconnected world, the ability to communicate effectively with network interfaces is essential for developers, network engineers, and tech enthusiasts alike. Whether you’re troubleshooting network issues, developing applications that rely on real-time data transmission, or simply exploring the vast landscape of networking, knowing how to send messages to a network interface can unlock a wealth of possibilities. This fundamental skill not only enhances your technical toolkit but also empowers you to harness the full potential of your networked devices.
At its core, sending messages to a network interface involves understanding the protocols and methods that facilitate communication between devices. This process can range from simple commands executed in a command-line interface to more complex interactions involving programming languages and networking libraries. By mastering these techniques, you can ensure that your messages reach their intended destinations, whether you’re working with local networks or traversing the complexities of the internet.
As we delve deeper into this topic, we will explore various approaches to sending messages to network interfaces, including the underlying principles of networking, the tools and technologies available, and practical examples that illustrate these concepts in action. Whether you’re a seasoned professional or a curious beginner, this guide will equip you with the knowledge and skills necessary to navigate the intricate world of network communication with confidence.
Understanding Network Interfaces
To effectively send messages to a network interface, it is crucial to comprehend the role of network interfaces in computing. A network interface is a point of interconnection between a computer and a network. It could be physical, such as an Ethernet port, or virtual, such as a software-defined interface used in virtual machines.
Network interfaces operate using protocols that allow data packets to be sent and received. The most common protocols include:
- TCP/IP: Transmission Control Protocol/Internet Protocol, foundational for internet communication.
- UDP: User Datagram Protocol, suitable for applications requiring fast, efficient communication without error correction.
- ICMP: Internet Control Message Protocol, used for diagnostics and network management.
Sending Messages to a Network Interface
To send messages to a network interface, specific tools and methods can be utilized based on the operating system and programming environment. Here are some commonly used methods:
- Using Command-Line Tools: Tools like `ping`, `curl`, and `telnet` can be employed to send messages or packets to a network interface.
- Programming Libraries: Libraries in various programming languages, such as Python’s `socket` library or Java’s networking classes, provide functionalities to send data to a network interface programmatically.
The following table summarizes some common tools and their purposes:
Tool | Purpose | Command Example |
---|---|---|
ping | Test connectivity to a network interface | ping 192.168.1.1 |
curl | Transfer data to or from a server | curl http://example.com |
telnet | Connect to remote systems via command line | telnet example.com 80 |
Example: Sending Data Using Sockets in Python
Python’s `socket` library is a powerful tool for sending messages to a network interface. Below is a basic example demonstrating how to create a socket and send a simple message:
“`python
import socket
Create a socket object
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Define the target host and port
target_host = “127.0.0.1”
target_port = 8080
Connect to the server
sock.connect((target_host, target_port))
Send some data
message = “Hello, Network Interface!”
sock.sendall(message.encode())
Close the socket
sock.close()
“`
This code establishes a connection to a server at the specified IP address and port, sends a message, and then closes the connection. It is essential to handle exceptions and errors for a robust implementation.
Security Considerations
When sending messages to network interfaces, security should always be a paramount concern. Consider the following practices:
- Use Encryption: Always encrypt sensitive data using protocols like SSL/TLS to prevent interception.
- Firewall Configuration: Ensure that firewalls are configured to allow only necessary traffic to network interfaces.
- Regular Updates: Keep network interface drivers and software updated to mitigate vulnerabilities.
By understanding the tools and methods available for sending messages to network interfaces, along with the associated security considerations, users can effectively communicate over networks while maintaining security and efficiency.
Understanding Network Interfaces
Network interfaces serve as the critical points of communication between devices and networks. Each interface can be associated with different protocols, such as Ethernet or Wi-Fi, and can support various types of data transmission. Understanding how to interact with these interfaces is essential for effective network management and troubleshooting.
Identifying the Network Interface
Before sending messages to a network interface, it’s important to identify the specific interface you wish to target. This can usually be accomplished via command-line tools or network configuration utilities. Common methods include:
- Linux/Unix:
- Use the command `ifconfig` or `ip addr` to list all network interfaces.
- Windows:
- Use the command `ipconfig` to display all active interfaces.
Command | Description |
---|---|
`ifconfig` | Displays configuration of network interfaces in Linux. |
`ip addr` | Shows IP addresses associated with network interfaces. |
`ipconfig` | Displays network configuration in Windows. |
Sending Messages to a Network Interface
Messages can be sent to a network interface using various protocols and tools, depending on the operating system and the intended application. Here are common methods:
- Using Command-Line Tools:
- Ping: This tool can send ICMP echo requests to test connectivity.
- Example: `ping
` - Netcat (nc): A versatile networking utility that can read and write data across network connections.
- Example: `echo “Hello” | nc
`
- Scripting and Programming:
- Python: Utilizing libraries like `socket` for TCP/UDP communication.
“`python
import socket
sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
sock.sendto(b’Hello, World’, (‘
“`
- PowerShell: For Windows environments, you can use cmdlets to send messages.
“`powershell
Send-MailMessage -To “
“`
Monitoring Network Messages
Monitoring messages sent to and from network interfaces is crucial for debugging and ensuring proper communication. Various tools are available:
- Wireshark: A powerful network protocol analyzer that captures and displays packet data.
- tcpdump: A command-line packet analyzer that allows you to capture network traffic.
- Example: `tcpdump -i
`
Tool | Description |
---|---|
Wireshark | GUI-based tool for analyzing network traffic. |
tcpdump | CLI tool for capturing and analyzing network packets. |
Best Practices
When sending messages to a network interface, consider the following best practices:
- Use Secure Protocols: Whenever possible, utilize secure communication protocols (e.g., HTTPS, SSH) to safeguard data in transit.
- Error Handling: Implement error handling in scripts to manage potential issues effectively.
- Logging: Maintain logs of sent messages for future reference and troubleshooting.
- Performance Monitoring: Regularly assess the performance of network interfaces to identify bottlenecks or failures.
By adhering to these guidelines, you can ensure more reliable and secure communication within your network environment.
Expert Insights on Sending Messages to a Network Interface
Dr. Emily Carter (Network Protocol Specialist, Tech Innovations Inc.). “Effectively sending messages to a network interface requires a thorough understanding of the underlying protocols, such as TCP/IP. By leveraging socket programming, developers can establish a reliable communication channel that ensures data integrity and timely delivery.”
Michael Chen (Senior Systems Engineer, CyberSecure Solutions). “When sending messages to a network interface, it is crucial to consider the security implications. Implementing encryption protocols like TLS can safeguard the data being transmitted, protecting it from potential interception during transit.”
Laura Patel (Chief Technology Officer, NetComm Technologies). “Utilizing APIs for network communication can significantly simplify the process of sending messages to a network interface. By abstracting the complexities of direct socket management, developers can focus on building robust applications that enhance user experience.”
Frequently Asked Questions (FAQs)
What is a network interface?
A network interface is a hardware or software component that connects a computer to a network, enabling communication with other devices. It can be a physical device like a network card or a virtual interface in software.
How can I send messages to a network interface?
To send messages to a network interface, you can use programming languages like Python or C with socket programming. This involves creating a socket, binding it to the interface, and using appropriate protocols (TCP/UDP) to send data.
What protocols can be used to send messages to a network interface?
Common protocols for sending messages include Transmission Control Protocol (TCP) for reliable communication and User Datagram Protocol (UDP) for faster, connectionless communication. The choice depends on the application requirements.
Can I send messages to a network interface using command-line tools?
Yes, command-line tools like `ping`, `netcat`, or `telnet` can be used to send messages or test connectivity to a network interface. These tools are useful for troubleshooting and testing network connections.
What are the common issues when sending messages to a network interface?
Common issues include network congestion, incorrect IP addressing, firewall restrictions, and protocol mismatches. These can lead to packet loss or failure to establish a connection.
Is it possible to send messages to a virtual network interface?
Yes, messages can be sent to virtual network interfaces just like physical ones. Virtual interfaces are often used in virtualized environments and can be addressed using their assigned IP addresses.
In summary, sending messages to a network interface involves understanding the fundamental concepts of networking and the specific protocols that govern data transmission. It requires familiarity with the tools and programming languages that facilitate communication with network interfaces, such as sockets in Python or C. By leveraging these tools, users can send and receive messages effectively, ensuring proper formatting and adherence to protocol specifications.
Additionally, it is crucial to consider the configuration of the network interface and the underlying operating system. Proper permissions and settings must be in place to allow for successful message transmission. Understanding the role of different layers in the OSI model can also aid in troubleshooting any issues that may arise during the process.
Key takeaways include the importance of selecting the right protocol for the task at hand, whether it be TCP for reliable communication or UDP for faster, connectionless messaging. Moreover, testing and validation of the message transmission process are essential to ensure that messages are sent and received accurately. Overall, mastering these techniques can greatly enhance one’s ability to interact with network interfaces effectively.
Author Profile
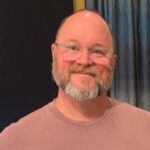
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?