How Can You Retrieve the Index Value of a Map in Java?
In the world of Java programming, maps serve as one of the most versatile and powerful data structures, enabling developers to store and manipulate key-value pairs with ease. Whether you’re building a complex application or simply organizing data, understanding how to effectively retrieve and manipulate values within a map is crucial. One common question that arises among Java developers is how to get the index value of a map. While maps are inherently unordered collections, grasping the concept of indexing in the context of maps can unlock new possibilities for data handling and retrieval.
To navigate the intricacies of maps in Java, it’s essential to first understand the fundamental differences between various map implementations, such as HashMap, TreeMap, and LinkedHashMap. Each of these collections offers unique characteristics that can affect how you access and index their elements. While traditional arrays and lists allow for straightforward indexing, maps require a different approach due to their key-based structure. This distinction can lead to confusion, especially for those new to Java or coming from a background in other programming languages.
In this article, we will explore the methods and techniques for obtaining index-like behavior from maps in Java. We’ll delve into the underlying principles of map operations, the importance of keys, and how to leverage Java’s powerful collections framework to achieve your indexing goals
Understanding Maps in Java
In Java, a Map is a collection that maps keys to values. It is an interface that provides a way to store data in key-value pairs. Unlike lists or arrays, Maps do not maintain the order of elements based on their indices but rather allow for efficient retrieval of values based on their corresponding keys.
The most commonly used implementations of the Map interface include:
- HashMap
- LinkedHashMap
- TreeMap
Each of these implementations has its own characteristics regarding ordering and performance.
Accessing Values in a Map
To access a value in a Map, you typically use the key associated with that value. For example:
“`java
Map
map.put(“One”, 1);
map.put(“Two”, 2);
int value = map.get(“One”); // value will be 1
“`
However, if you want to retrieve the index of a value in a Map, it is important to note that Maps do not have a concept of indices like Lists do. Instead, you can achieve this by iterating over the entry set or using a list of keys or values.
Finding the Index of a Value in a Map
To find the index value of a specific key in a Map, you can convert the keys or values of the Map into a List and then find the index of the desired element. Here’s how you can do it:
- Convert the keys or values of the Map into a List.
- Use the `indexOf()` method to find the index of the desired key or value.
Here is an example demonstrating how to find the index of a key in a HashMap:
“`java
Map
map.put(“One”, 1);
map.put(“Two”, 2);
map.put(“Three”, 3);
// Convert keys to a List
List
int index = keys.indexOf(“Two”); // index will be 1
“`
Example Code: Finding Index Values
Below is an example code snippet that demonstrates both finding the index of a key and a value in a Map:
“`java
import java.util.*;
public class MapIndexExample {
public static void main(String[] args) {
Map
map.put(“One”, 1);
map.put(“Two”, 2);
map.put(“Three”, 3);
// Finding index of a key
List
int keyIndex = keys.indexOf(“Two”); // Output: 1
// Finding index of a value
List
int valueIndex = values.indexOf(3); // Output: 2
System.out.println(“Index of ‘Two’: ” + keyIndex);
System.out.println(“Index of value ‘3’: ” + valueIndex);
}
}
“`
Table: Map Implementations Comparison
Type | Ordering | Performance | Null Keys/Values |
---|---|---|---|
HashMap | No | O(1) average | One null key, multiple null values |
LinkedHashMap | Insertion order | O(1) average | One null key, multiple null values |
TreeMap | Sorted order | O(log n) | No null keys, multiple null values |
Using the above methods, you can effectively find the index of keys or values in a Map, enabling you to work with data collections in a more flexible manner.
Accessing Index Values in a Java Map
In Java, the `Map` interface does not support indexing like lists or arrays, as it is designed to store key-value pairs rather than ordered elements. However, you can achieve a similar effect by using methods that allow you to access the entries in a specific order.
Using List to Maintain Order
To simulate index-based access, one common approach is to convert the keys or entries of the map into a list. This allows you to access the elements by their index. Here’s how you can do it:
“`java
import java.util.*;
public class MapIndexExample {
public static void main(String[] args) {
Map
map.put(“A”, 1);
map.put(“B”, 2);
map.put(“C”, 3);
List
// Access the index value
int index = 1; // Example index
String keyAtIndex = keys.get(index);
Integer valueAtIndex = map.get(keyAtIndex);
System.out.println(“Key at index ” + index + “: ” + keyAtIndex);
System.out.println(“Value at index ” + index + “: ” + valueAtIndex);
}
}
“`
Iterating Over Map Entries
Another way to access elements is by iterating through the map’s entry set. This method does not provide direct index access but allows you to perform operations based on the iteration count:
“`java
for (Map.Entry
// This can be combined with a counter to simulate index access
// Example to retrieve the second entry
if (counter == 1) {
System.out.println(“Key: ” + entry.getKey() + “, Value: ” + entry.getValue());
}
counter++;
}
“`
Using Java Streams for Index-Based Access
Java Streams provide a modern approach to access elements in a map. You can leverage the `IntStream` to generate indices and map them to entries:
“`java
import java.util.stream.IntStream;
IntStream.range(0, keys.size())
.forEach(i -> {
String key = keys.get(i);
Integer value = map.get(key);
System.out.println(“Index ” + i + “: Key = ” + key + “, Value = ” + value);
});
“`
Understanding Map Implementations
Different implementations of the `Map` interface can affect the order of elements:
Implementation | Order Preservation | Use Case |
---|---|---|
`HashMap` | No | Fast lookups, unordered |
`LinkedHashMap` | Yes | Order of insertion maintained |
`TreeMap` | Yes (sorted) | Sorted order based on keys |
Choosing the right map implementation based on your requirement is crucial for efficient data access and manipulation.
Accessing index values in a Java `Map` requires workarounds, as direct indexing is not supported. By converting keys to a list, iterating through entries, or using Java Streams, developers can effectively simulate index-based access to map elements.
Expert Insights on Retrieving Index Values from Maps in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In Java, maps do not maintain an order of elements like lists do, which means they do not have index values in the traditional sense. Instead, if you need to retrieve a value based on a specific key, you should utilize the `get()` method. For scenarios where you need to maintain an order, consider using a `LinkedHashMap`.”
James Lee (Java Developer Advocate, CodeCraft). “Understanding that a `Map` in Java is fundamentally a key-value pair structure is crucial. If you need to access elements by index, you might want to convert the keys or values into a list. This way, you can utilize list indexing to achieve your goal, but remember that this approach can introduce additional overhead.”
Linda Martinez (Java Programming Instructor, DevAcademy). “When working with maps in Java, it is essential to recognize that they are not designed for index-based access. If you require index-like behavior, consider using an `ArrayList` or a combination of a `List` and a `Map`. This allows you to maintain the benefits of both data structures while achieving the desired functionality.”
Frequently Asked Questions (FAQs)
How can I retrieve the index value of a specific entry in a Java Map?
To retrieve the index value of a specific entry in a Java Map, you must convert the Map’s entry set to a List. Use the `ArrayList` constructor to create a list from the entry set, and then use the `indexOf` method to find the index of the desired entry.
Is it possible to get the index of a key in a HashMap?
No, HashMap does not maintain the order of its entries, so it does not support index-based access. If you need index access, consider using a LinkedHashMap or converting the entries to a List.
What is the difference between HashMap and LinkedHashMap regarding index access?
HashMap does not maintain any order, while LinkedHashMap maintains the insertion order. This means you can retrieve the index of an entry in a LinkedHashMap by converting its entry set to a List.
Can I use Streams to get the index of an entry in a Map?
Yes, you can use Java Streams to achieve this. Convert the entry set to a Stream, and then use the `map` method to create a list of indices based on the entry’s position.
How do I find the index of a value in a TreeMap?
Similar to HashMap, TreeMap does not support direct index access. You can convert its entry set to a List and then use the `indexOf` method to find the index of a specific value.
Are there any performance considerations when retrieving index values from a Map?
Yes, converting a Map to a List incurs additional overhead, especially for large Maps. Consider the performance implications and whether you truly need index access before proceeding with this approach.
In Java, the concept of an index value in the context of a Map is somewhat different from that of a traditional array or list. Maps in Java, such as HashMap or TreeMap, do not maintain an inherent order or index for their elements. Instead, they store key-value pairs, where each key is unique and is used to retrieve the corresponding value. Therefore, the notion of obtaining an index value directly from a Map does not apply in the conventional sense.
To access elements in a Map, developers typically use the key associated with the value they wish to retrieve. If the requirement is to iterate over the entries in a Map and obtain an index-like behavior, one approach is to convert the entry set of the Map into a list. This allows for indexed access, but it is essential to remember that the order of elements may vary depending on the type of Map used. For instance, a LinkedHashMap maintains insertion order, while a HashMap does not guarantee any specific order.
In summary, while Maps in Java do not provide an index value in the traditional sense, developers can work around this limitation by converting the Map entries to a list format. This method allows for indexed access, but it is crucial to be aware
Author Profile
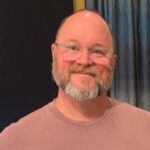
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?