Why Does My Component Say It ‘Cannot Be Used As A JSX Component’?
In the vibrant world of React development, the ability to seamlessly integrate components is crucial for building dynamic user interfaces. However, developers often encounter a perplexing error: “Cannot be used as a JSX component.” This seemingly cryptic message can halt progress and lead to frustration, especially for those new to the framework. Understanding the underlying causes and solutions to this issue is essential for any React developer looking to refine their skills and enhance their applications.
This error typically arises when there is a mismatch between the expected component structure and what is actually being rendered. It can stem from various factors, including incorrect imports, improper component definitions, or even issues with the way props are being passed. As developers navigate through the intricacies of JSX and component-based architecture, recognizing the signs of this error can empower them to troubleshoot effectively and maintain the fluidity of their coding workflow.
By delving into the nuances of this error, readers will gain valuable insights into best practices for component creation and management in React. Whether you’re a seasoned developer or just starting your journey, understanding how to resolve the “Cannot be used as a JSX component” issue will not only enhance your coding proficiency but also boost your confidence in tackling more complex challenges in the realm of front-end development.
Understanding the Error Message
The error message “Cannot be used as a JSX component” typically occurs in React applications when the compiler is unable to recognize a component as a valid JSX element. This can stem from various issues, including improper imports, incorrect component definitions, or issues with TypeScript types.
Common causes include:
- Incorrect Import Statements: Ensure that your component is imported correctly from its file. A typo in the path or file name can lead to this error.
- Component Definition: The component must be defined as a function or class component. An incorrect syntax might result in the component being unrecognized.
- TypeScript Issues: If you’re using TypeScript, the component might be missing type annotations or might not be exported properly.
Diagnosing the Issue
To diagnose the issue effectively, follow these steps:
- Check the import statement for the component.
- Ensure the component is defined correctly, either as a function or a class.
- Review TypeScript definitions, if applicable.
Here’s a simple checklist:
- [ ] Verify the component is exported from its module.
- [ ] Confirm the component is imported with the correct path.
- [ ] Check the syntax of the component definition.
- [ ] Ensure you are using the correct capitalization, as JSX is case-sensitive.
Common Solutions
To resolve the “Cannot be used as a JSX component” error, consider the following solutions:
– **Correct the Import Statement**: Make sure you are importing your component correctly. For example:
“`javascript
import MyComponent from ‘./MyComponent’;
“`
– **Define the Component Properly**: If using a functional component, ensure it is defined as follows:
“`javascript
const MyComponent = () => {
return
;
};
“`
- Ensure TypeScript Compatibility: If using TypeScript, ensure your component type is specified correctly:
“`typescript
interface MyComponentProps {
title: string;
}
const MyComponent: React.FC
return
{title}
;
};
“`
Example of a Component Structure
Here’s an example of a properly structured React component:
“`javascript
// MyComponent.js
import React from ‘react’;
const MyComponent = () => {
return
;
};
export default MyComponent;
“`
In your main application file, ensure the import is done correctly:
“`javascript
// App.js
import React from ‘react’;
import MyComponent from ‘./MyComponent’;
const App = () => {
return (
);
};
export default App;
“`
Comparison of Functional and Class Components
Understanding the differences between functional and class components can also help in identifying the source of the issue. Here’s a comparison:
Aspect | Functional Component | Class Component |
---|---|---|
Syntax | Function declaration or arrow function | Class declaration extending React.Component |
State Management | Hooks (e.g., useState) | this.state and this.setState |
Lifecycle Methods | useEffect Hook | componentDidMount, componentDidUpdate, componentWillUnmount |
Performance | Generally faster due to less overhead | More overhead |
By following these guidelines, you can effectively troubleshoot and resolve the “Cannot be used as a JSX component” error in your React applications.
Understanding the Error Message
The error message “Cannot be used as a JSX component” typically occurs in React applications when the component being referenced is not correctly defined or imported. This can lead to confusion, especially for developers who are new to the framework. Understanding the underlying causes can help in troubleshooting effectively.
Common Causes of the Error
- Incorrect Component Definition: The component might not be defined as a function or class.
- Default vs Named Exports: The component may be exported as a named export but imported incorrectly as a default export, or vice versa.
- Improper Imports: The component file might not be correctly imported, leading to references.
- TypeScript Issues: If using TypeScript, the component may not have the correct type annotations or interfaces defined.
- File Naming Conventions: The casing of filenames can lead to issues, especially on case-sensitive file systems.
Resolving the Error
To fix the “Cannot be used as a JSX component” error, follow these steps:
Check Component Definition
Ensure that the component is defined correctly. A functional component should look like this:
“`javascript
const MyComponent = () => {
return
;
};
“`
For a class component:
“`javascript
class MyComponent extends React.Component {
render() {
return
;
}
}
“`
Verify Import Statements
Make sure your import statements are accurate. For example:
- Named Export:
“`javascript
import { MyComponent } from ‘./MyComponent’;
“`
- Default Export:
“`javascript
import MyComponent from ‘./MyComponent’;
“`
Ensure Correct File Paths
Check that the file path specified in the import statement is correct and that the file exists. Pay attention to:
- Relative paths (e.g., `./` or `../`)
- Correct file extensions (.js, .jsx, .ts, .tsx)
TypeScript Considerations
If using TypeScript, confirm that the component has the appropriate types defined. This can prevent type-related issues that lead to the error message. For example:
“`typescript
interface MyComponentProps {
title: string;
}
const MyComponent: React.FC
return
{title}
;
};
“`
Check for Circular Dependencies
Circular dependencies can also lead to this issue. Inspect your components to ensure that they do not reference each other in a manner that creates a loop.
Debugging Techniques
Here are some techniques to help debug the issue effectively:
- Console Logging: Use `console.log` statements to check if the component is defined correctly before it is rendered.
- React DevTools: Utilize React DevTools to inspect component hierarchies and see if the component appears as expected.
- Linting Tools: Use ESLint or other linting tools to identify import/export issues and other syntax errors.
- Check Compiler Output: Review any compiler output or warnings that may provide insight into the error.
Example Scenarios
Scenario | Description |
---|---|
Incorrect Import | Importing a named export as a default export will cause this error. |
Misnamed Component | If the component is defined as `MyComponent` but imported as `myComponent`, the error will occur. |
TypeScript Mismatch | Using a prop in JSX that is not defined in the component’s props interface may trigger the error. |
Circular Reference | Components referencing each other may lead to components during rendering. |
By understanding the error message and applying the above resolutions, developers can effectively troubleshoot and resolve the “Cannot be used as a JSX component” issue in their React applications.
Understanding the “Cannot Be Used As A JSX Component” Error
Jessica Tran (Senior Frontend Developer, CodeCraft Solutions). “The ‘Cannot Be Used As A JSX Component’ error typically arises when a component is not defined correctly or when there is a mismatch in the expected props. It is crucial to ensure that the component is a valid function or class component and that it adheres to the expected structure of JSX.”
Michael Chen (React Specialist, DevInsights). “This error can also occur if you attempt to use a primitive type or a non-component function in a JSX context. Always verify that the component is imported correctly and that it is not being shadowed by another variable with the same name.”
Laura Patel (Senior Software Engineer, Tech Innovators). “In many cases, this issue can be resolved by reviewing the component’s export statement. If a component is exported as a default export, ensure it is imported without curly braces. Misunderstandings around default and named exports are common sources of this error.”
Frequently Asked Questions (FAQs)
What does “Cannot Be Used As A JSX Component” mean?
This error indicates that a function or class is not recognized as a valid JSX component. It typically occurs when the component is not properly defined or exported.
What are common reasons for encountering this error?
Common reasons include incorrect import statements, missing or incorrect component definitions, or attempting to use a non-component function as a JSX element.
How can I resolve the “Cannot Be Used As A JSX Component” error?
To resolve this error, ensure that the component is correctly defined, exported, and imported. Verify that the component name starts with an uppercase letter and check for any syntax errors.
Does this error occur with functional components only?
No, this error can occur with both functional and class components. The key factor is whether the component is properly defined and recognized by React.
Can this error be caused by TypeScript issues?
Yes, TypeScript can contribute to this error if the component type is not correctly defined or if the props do not match the expected types. Ensure that the component’s props are properly typed.
What should I check if the error persists after making changes?
If the error persists, check for circular dependencies, ensure that the component file is saved, and verify that your development environment is correctly set up to recognize JSX syntax.
The phrase “Cannot be used as a JSX component” typically arises in React development when there is an issue with how a component is defined or imported. This error can occur for various reasons, including incorrect component naming, improper export methods, or issues with the component’s return type. Understanding the underlying causes of this error is essential for developers to effectively troubleshoot and resolve issues in their React applications.
One of the primary reasons for this error is the failure to properly export a component. In React, components must be exported correctly for them to be imported and used in other files. Developers should ensure they are using default or named exports appropriately. Additionally, the component must return valid JSX, as returning or null can also lead to this error. Ensuring that the component is a functional or class component is crucial for its proper usage.
Another important takeaway is the significance of component naming conventions. JSX components must start with an uppercase letter; otherwise, React interprets them as HTML elements. This distinction is vital to avoid confusion and ensure that React treats the component correctly. Furthermore, developers should check for any typos or incorrect imports that could lead to this error, as even minor mistakes can disrupt the functioning of the application.
In
Author Profile
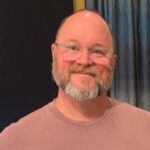
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?