How Can You Efficiently Parse JSON in PostgreSQL Using Stored Procedures?
In the ever-evolving landscape of data management, the ability to efficiently handle and manipulate JSON data has become increasingly vital for developers and database administrators alike. PostgreSQL, a powerful open-source relational database system, has embraced this trend by incorporating robust support for JSON and JSONB data types. As organizations strive to leverage the flexibility of JSON for their applications, the need for effective methods to parse and process this data within stored procedures emerges as a crucial topic. This article delves into the intricacies of using stored procedures in PostgreSQL to parse JSON, offering insights that will empower you to maximize the potential of your data.
Understanding how to work with JSON in PostgreSQL opens up a world of possibilities for enhancing data interactions. Stored procedures, which encapsulate complex logic and operations, serve as a powerful tool for automating tasks and improving performance. By integrating JSON parsing within these procedures, developers can streamline data processing workflows, ensuring that applications can efficiently retrieve and manipulate structured data. This approach not only enhances the readability of code but also promotes reusability, making it easier to maintain and update as requirements evolve.
As we explore the nuances of stored procedures and JSON parsing in PostgreSQL, we will uncover best practices, common pitfalls, and practical examples that illustrate how to harness the full capabilities of
Understanding JSON Data Types in PostgreSQL
PostgreSQL supports two JSON data types: `json` and `jsonb`. The `json` type stores JSON data as a text string, while `jsonb` stores it in a binary format, allowing for more efficient processing and indexing.
- json: This type preserves whitespace and the order of keys, making it suitable for scenarios where exact representation is necessary.
- jsonb: This type strips whitespace, orders keys, and is optimized for performance. It supports indexing, which enhances query speed.
When choosing between the two, consider the following:
Feature | json | jsonb |
---|---|---|
Storage Size | Larger | Smaller |
Performance | Slower | Faster |
Indexing | Not supported | Supported |
Duplicate Keys | Allowed | Not allowed |
Creating a Stored Procedure for JSON Parsing
To effectively parse JSON data, a stored procedure can be employed. This procedure can take a JSON object as input and return structured data. Below is an example of how to create such a stored procedure in PostgreSQL:
“`sql
CREATE OR REPLACE FUNCTION parse_json(json_data jsonb)
RETURNS TABLE(id INT, name TEXT, age INT) AS $$
BEGIN
RETURN QUERY
SELECT
(json_data->>’id’)::INT,
json_data->>’name’,
(json_data->>’age’)::INT
;
END;
$$ LANGUAGE plpgsql;
“`
This function takes a `jsonb` input, extracts values using the `->>` operator, and returns them as a table.
Invoking the Stored Procedure
Once the stored procedure is created, you can invoke it by passing a JSON object. Here’s how to do it:
“`sql
SELECT * FROM parse_json(‘{“id”: 1, “name”: “John Doe”, “age”: 30}’);
“`
The output will be structured as follows:
id | name | age |
---|---|---|
1 | John Doe | 30 |
This structured output allows for easier data manipulation and integration into other database operations.
Handling JSON Arrays
When dealing with JSON arrays, the stored procedure can be adapted to parse multiple entries. Here’s an updated version that handles an array of JSON objects:
“`sql
CREATE OR REPLACE FUNCTION parse_json_array(json_array jsonb)
RETURNS TABLE(id INT, name TEXT, age INT) AS $$
BEGIN
RETURN QUERY
SELECT
(elem->>’id’)::INT,
elem->>’name’,
(elem->>’age’)::INT
FROM jsonb_array_elements(json_array) AS elem;
END;
$$ LANGUAGE plpgsql;
“`
You can call this function with a JSON array like so:
“`sql
SELECT * FROM parse_json_array(‘[{“id”: 1, “name”: “John Doe”, “age”: 30}, {“id”: 2, “name”: “Jane Doe”, “age”: 25}]’);
“`
This would return:
id | name | age |
---|---|---|
1 | John Doe | 30 |
2 | Jane Doe | 25 |
Utilizing these techniques allows for robust handling of JSON data within PostgreSQL, making it a powerful tool for developers and database administrators.
Understanding JSON Data Types in PostgreSQL
PostgreSQL provides robust support for JSON, allowing users to store, query, and manipulate JSON data effectively. The key JSON data types in PostgreSQL are:
- json: Stores JSON data as text, without enforcing any constraints on the structure.
- jsonb: A binary format that stores JSON data in a decomposed binary form, providing efficiency for querying and indexing.
The choice between `json` and `jsonb` depends on the specific use case. `jsonb` is generally preferred for its performance benefits, especially when dealing with large datasets or requiring frequent querying.
Creating a Stored Procedure to Parse JSON
Creating a stored procedure in PostgreSQL to parse JSON data involves defining the procedure, input parameters, and the logic to manipulate the JSON data. Below is an example of such a stored procedure.
“`sql
CREATE OR REPLACE FUNCTION parse_json_data(json_input jsonb)
RETURNS TABLE(id integer, name text, age integer) AS $$
BEGIN
RETURN QUERY
SELECT
(value->>’id’)::integer,
value->>’name’,
(value->>’age’)::integer
FROM jsonb_array_elements(json_input->’people’) AS value;
END;
$$ LANGUAGE plpgsql;
“`
In this example:
- The function `parse_json_data` takes a `jsonb` input.
- It returns a table with columns `id`, `name`, and `age`.
- The `jsonb_array_elements` function is used to iterate through each element in the JSON array under the key `people`.
Calling the Stored Procedure
To call the stored procedure, you can use the following SQL command. Ensure that the JSON structure conforms to the expected format.
“`sql
SELECT * FROM parse_json_data(‘{
“people”: [
{“id”: 1, “name”: “Alice”, “age”: 30},
{“id”: 2, “name”: “Bob”, “age”: 25}
]
}’::jsonb);
“`
This will return a result set structured as follows:
id | name | age |
---|---|---|
1 | Alice | 30 |
2 | Bob | 25 |
Best Practices for JSON Parsing in Stored Procedures
When working with JSON in stored procedures, consider the following best practices:
- Validate Input: Ensure that the JSON structure is validated before parsing to avoid runtime errors.
- Use jsonb: Prefer `jsonb` over `json` for better performance and flexibility in indexing.
- Indexing: Create indexes on frequently accessed JSON fields to improve query performance.
- Error Handling: Implement exception handling to manage unexpected input or parsing errors.
Performance Considerations
Parsing JSON can impact performance, especially with large datasets. To mitigate performance issues:
- Optimize JSON structure to reduce unnecessary nesting.
- Limit the size of JSON objects when possible.
- Use appropriate data types for the fields extracted from JSON to minimize type casting overhead.
Implementing these strategies can lead to more efficient JSON parsing and better overall application performance in PostgreSQL.
Expert Insights on Stored Procedure JSON Parsing in PostgreSQL
Dr. Lisa Chen (Database Architect, Data Solutions Inc.). “The ability to parse JSON within stored procedures in PostgreSQL allows for more dynamic and flexible data manipulation. It significantly enhances the performance of applications that require frequent data transformations, making it a vital skill for modern database developers.”
Mark Thompson (Senior Software Engineer, Tech Innovations). “Utilizing stored procedures for JSON parsing in PostgreSQL not only streamlines data processing but also encapsulates business logic. This encapsulation leads to improved maintainability and security, as the logic is centralized and can be modified without altering the application code.”
Dr. Emily Rodriguez (Data Scientist, Analytics Hub). “Incorporating JSON parsing within stored procedures enhances the ability to handle semi-structured data efficiently. This capability is particularly beneficial for data science applications that rely on diverse data formats, allowing for more comprehensive analysis and insights.”
Frequently Asked Questions (FAQs)
What is a stored procedure in PostgreSQL?
A stored procedure in PostgreSQL is a set of SQL statements that can be stored in the database and executed as a single unit. It allows for encapsulation of complex logic, improving performance and maintainability.
How can I parse JSON data within a stored procedure in PostgreSQL?
To parse JSON data within a stored procedure, you can use PostgreSQL’s built-in JSON functions such as `json_populate_record`, `jsonb_array_elements`, or `jsonb_each`. These functions allow you to extract and manipulate JSON data efficiently.
What are the benefits of using JSON in PostgreSQL?
Using JSON in PostgreSQL provides flexibility in data storage, allowing for semi-structured data representation. It also supports advanced querying capabilities, indexing options, and integration with various programming languages.
Can I return a JSON object from a stored procedure in PostgreSQL?
Yes, you can return a JSON object from a stored procedure by using the `RETURN` statement with `json_build_object` or `json_agg` functions. This allows you to construct and return JSON data directly from your procedure.
What is the difference between JSON and JSONB in PostgreSQL?
JSON stores data as plain text, while JSONB stores it in a binary format. JSONB offers better performance for operations like searching and indexing, as well as support for more complex queries. However, JSON is more human-readable.
How do I handle errors when parsing JSON in a stored procedure?
To handle errors when parsing JSON in a stored procedure, you can use exception handling with the `BEGIN…EXCEPTION` block. This allows you to catch and manage exceptions that may arise during JSON parsing, ensuring robust error management.
Stored procedures in PostgreSQL offer a powerful mechanism for encapsulating complex logic and operations, particularly when dealing with JSON data. The ability to parse JSON within stored procedures allows developers to efficiently manipulate and retrieve data stored in JSON format, which is increasingly common in modern applications. PostgreSQL provides robust support for JSON and JSONB data types, enabling users to perform various operations such as extraction, transformation, and aggregation of JSON data within the context of stored procedures.
One of the key advantages of using stored procedures for JSON parsing is the potential for improved performance. By executing complex queries and data manipulations within the database server, developers can reduce the amount of data transferred between the database and application layers. This not only enhances the speed of data processing but also minimizes network overhead. Furthermore, stored procedures can encapsulate business logic, making the database interactions more maintainable and secure.
Another important consideration is the flexibility that stored procedures provide in handling various JSON structures. With functions like `json_populate_record`, `jsonb_array_elements`, and others, developers can easily navigate and manipulate nested JSON objects and arrays. This capability is essential for applications that rely on dynamic data structures, allowing for more agile and responsive database designs. Overall, leveraging stored procedures for
Author Profile
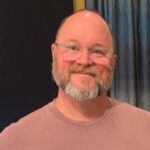
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?