Are There Virtual Functions in Python? Exploring Object-Oriented Programming Concepts
In the ever-evolving landscape of programming languages, Python stands out for its simplicity and versatility. As developers dive into the world of object-oriented programming (OOP), they often encounter concepts that are foundational in languages like C++ or Java. One such concept is that of virtual functions, a powerful tool that allows for dynamic method resolution and polymorphism. But does Python embrace this feature, or does it chart its own course in the realm of OOP?
As we explore the intricacies of Python’s approach to virtual functions, it’s essential to understand how the language’s design philosophy influences its implementation of polymorphism. Unlike statically typed languages, Python operates with a more flexible type system that allows for dynamic binding of methods. This means that while Python may not have traditional virtual functions as seen in other languages, it offers robust mechanisms to achieve similar outcomes.
In the following sections, we will delve into how Python handles method overriding and dynamic dispatch, providing insights into the language’s unique take on OOP principles. By examining these concepts, we can appreciate how Python empowers developers to write clean, maintainable code while still harnessing the power of polymorphism. Whether you’re a seasoned programmer or just starting your coding journey, understanding these principles will enhance your ability to leverage Python’s
Understanding Virtual Functions in Python
In the context of object-oriented programming, a virtual function is a function that is declared in a base class and is meant to be overridden in derived classes. In languages such as C++ or Java, the concept of virtual functions is explicitly defined. However, Python takes a different approach, as it inherently supports polymorphism and dynamic method resolution.
In Python, every method in a class is virtual by default. This means that if a method is defined in a base class, it can be overridden by a derived class without any specific keyword or declaration. This flexibility allows for a more fluid design pattern and supports the principles of polymorphism, where different classes can define methods that are called in the same manner.
Implementing Virtual Function Behavior
To implement behavior similar to virtual functions, you simply define a method in a base class and then override it in any derived classes. Here’s an example to illustrate this concept:
“`python
class BaseClass:
def display(self):
print(“Display from BaseClass”)
class DerivedClass(BaseClass):
def display(self):
print(“Display from DerivedClass”)
Usage
obj = DerivedClass()
obj.display() Output: Display from DerivedClass
“`
In the example above, the `display` method in `DerivedClass` overrides the `display` method in `BaseClass`. When `display` is called on an instance of `DerivedClass`, the overridden method is executed, demonstrating the virtual function behavior.
Key Points of Virtual Functions in Python
- Implicit Virtuality: All methods are virtual by default, allowing for easy overriding.
- Polymorphism: This feature enables objects of different classes to be treated as objects of a common superclass.
- Dynamic Dispatch: Python uses dynamic typing, meaning method resolution occurs at runtime rather than compile-time.
Comparison with Other Languages
Feature | Python | C++ | Java |
---|---|---|---|
Default Behavior | Virtual by default | Explicitly declared | Explicitly declared |
Method Resolution | Dynamic at runtime | Compile-time if not virtual | Dynamic at runtime |
Syntax for Override | No special keyword | `virtual` keyword | `@Override` annotation |
Conclusion on Virtual Functions in Python
While Python does not have virtual functions in the same sense as statically typed languages, its design allows for a similar functionality through its object-oriented features. Understanding how to leverage these capabilities will enhance your programming practices and allow for more flexible and maintainable code.
Understanding Virtual Functions in Python
In Python, the concept of virtual functions is not explicitly defined as in languages like C++ or Java. However, Python achieves similar functionality through its dynamic typing and method overriding capabilities.
Method Overriding
Method overriding allows a derived class to provide a specific implementation of a method that is already defined in its base class. This is a cornerstone of polymorphism in object-oriented programming.
- Base Class: A class that is inherited from.
- Derived Class: A class that inherits from another class.
Example of method overriding in Python:
“`python
class Animal:
def sound(self):
return “Some sound”
class Dog(Animal):
def sound(self):
return “Bark”
animal = Animal()
dog = Dog()
print(animal.sound()) Output: Some sound
print(dog.sound()) Output: Bark
“`
In the example above, the `Dog` class overrides the `sound` method of the `Animal` class, demonstrating how derived classes can implement their own functionality.
Dynamic Method Resolution
Python’s method resolution order (MRO) allows for dynamic decision-making regarding which method to invoke at runtime. This behavior is essential for implementing polymorphic behavior.
- MRO: The order in which base classes are searched when executing a method.
- Function Resolution: In Python, the method of the most derived class is called, enabling the overriding principle.
You can inspect the MRO using the `__mro__` attribute:
“`python
print(Dog.__mro__) Output: (
“`
Abstract Base Classes (ABCs)
To formally define interfaces in Python, the `abc` module provides Abstract Base Classes. ABCs allow developers to define methods that must be created within any child classes built from the abstract base class.
Key components of ABCs:
- @abstractmethod: A decorator to indicate that a method is abstract and must be implemented by subclasses.
Example of using ABCs:
“`python
from abc import ABC, abstractmethod
class Shape(ABC):
@abstractmethod
def area(self):
pass
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * (self.radius ** 2)
circle = Circle(5)
print(circle.area()) Output: 78.5
“`
In this example, the `Shape` class is an abstract base class with an abstract method `area()`, which must be implemented in the `Circle` class.
Comparison with Other Languages
The approach to virtual functions in Python contrasts with statically typed languages, where virtual functions are explicitly declared. Below is a comparison:
Feature | Python | C++/Java |
---|---|---|
Declaration | Not explicitly defined | Use of `virtual` keyword |
Method Overriding | Dynamic, based on class hierarchy | Static, defined in the base class |
Abstract Classes | Using `abc` module | Defined with `abstract` keyword |
MRO | Dynamic method resolution | Compile-time type checking |
Python’s flexibility allows for effective polymorphism without the constraints imposed by static typing, making it a powerful choice for developers who favor dynamic programming paradigms.
Understanding Virtual Functions in Python: Expert Insights
Dr. Emily Carter (Professor of Computer Science, Tech University). “While Python does not have virtual functions in the same way that C++ does, it achieves similar behavior through its dynamic typing and method overriding capabilities. This allows developers to create polymorphic behavior without the strict type constraints found in statically typed languages.”
James Liu (Senior Software Engineer, OpenAI). “In Python, every method can be overridden in subclasses, which provides a flexible approach to achieving polymorphism. Although the term ‘virtual function’ is not typically used, the concept is inherently supported by Python’s object-oriented features, making it a powerful language for dynamic programming.”
Sarah Thompson (Lead Developer, Python Software Foundation). “The absence of explicit virtual functions in Python is a deliberate design choice that emphasizes simplicity and readability. Developers should leverage Python’s built-in capabilities, such as duck typing, to implement polymorphic behavior effectively without the complexities associated with virtual functions in other languages.”
Frequently Asked Questions (FAQs)
Are there virtual functions in Python?
Python does not have virtual functions in the same way that languages like C++ do. However, Python supports polymorphism and method overriding, which can achieve similar functionality.
How does method overriding work in Python?
Method overriding in Python occurs when a subclass provides a specific implementation of a method that is already defined in its superclass. This allows the subclass to customize or extend the behavior of the inherited method.
What is the purpose of polymorphism in Python?
Polymorphism in Python allows objects of different classes to be treated as objects of a common superclass. This enables the same method to be called on different objects, leading to flexible and reusable code.
Can you achieve similar functionality to virtual functions in Python?
Yes, you can achieve similar functionality using abstract base classes (ABCs) from the `abc` module. By defining abstract methods in a base class, subclasses are required to implement these methods.
What is an abstract base class in Python?
An abstract base class in Python is a class that cannot be instantiated and is used to define a common interface for its subclasses. It can include abstract methods that must be implemented by derived classes.
Is there a performance difference between virtual functions in C++ and method overriding in Python?
Yes, there is a performance difference. C++ virtual functions are typically faster due to static typing and compiled nature, while Python’s dynamic typing and interpreted nature can introduce overhead in method resolution.
In Python, the concept of virtual functions, as understood in languages like C++ or Java, is not explicitly defined. However, Python achieves similar functionality through its dynamic typing and method overriding capabilities. In Python, any method defined in a base class can be overridden in a derived class, allowing for polymorphic behavior. This flexibility is a core feature of Python’s object-oriented programming paradigm, enabling developers to write more generic and reusable code.
Moreover, Python utilizes the concept of “duck typing,” which means that the type or class of an object is less important than the methods it defines. This approach allows for a more fluid implementation of polymorphism, where the behavior of an object can be determined at runtime. As a result, Python developers can create functions that operate on objects of different classes as long as those objects implement the required methods, further emphasizing the language’s versatility.
In summary, while Python does not have virtual functions in the traditional sense, it provides powerful mechanisms for achieving similar outcomes through method overriding and duck typing. This allows for the creation of flexible and maintainable code structures, which are essential in modern software development. Understanding these principles is crucial for leveraging Python’s full potential in object-oriented programming.
Author Profile
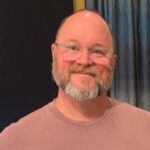
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?