How Can You Use Docker Compose to Mount a File That Doesn’t Exist Yet?
In the world of containerization, Docker has emerged as a game-changer, allowing developers to create, deploy, and manage applications with remarkable ease. One of the standout features of Docker is Docker Compose, a tool that simplifies the orchestration of multi-container applications. However, as with any powerful tool, there are nuances that can trip up even seasoned developers. One such nuance is the ability to mount files that do not yet exist within your Docker containers. This seemingly straightforward task can lead to confusion and frustration, especially for those new to the Docker ecosystem. Understanding how to effectively manage file mounts, even when the files are absent, is crucial for ensuring smooth development workflows and robust application performance.
When working with Docker Compose, you might encounter scenarios where you need to mount a file that hasn’t been created yet. This can happen in various situations, such as when you want to share configuration files, logs, or other data between your host machine and the container. The challenge lies in Docker’s behavior regarding non-existent files during the mounting process. While it may seem logical to expect the system to create the file automatically, Docker has its own set of rules that dictate how mounts are handled.
To navigate this challenge, it’s essential to grasp the underlying principles of how Docker Compose
Understanding File Mounts in Docker Compose
When working with Docker Compose, managing file mounts is crucial for ensuring that your containers have access to the necessary files. A common scenario arises when you attempt to mount a file that does not exist on the host system. Understanding how Docker Compose handles such cases can prevent unexpected behavior in your application.
When you specify a file mount in your `docker-compose.yml` file, Docker expects the file to be present on the host. If the file does not exist, Docker will create an empty file at that path in the container. This behavior can lead to confusion, as you may expect the container to fail or throw an error if the file is missing.
Behavior of Missing Files
The behavior of Docker when a specified file does not exist on the host can be summarized as follows:
- Empty File Creation: If the host file is absent, Docker creates an empty file in the container.
- No Error Thrown: Docker Compose does not throw an error or warning if the specified file is missing.
- Potential Data Loss: If the container writes to this newly created empty file, any expected data may be lost.
This behavior is particularly important in development environments, where you might rely on specific configurations or scripts. A missing configuration file could lead to incorrect application behavior without any immediate indication of the issue.
Best Practices for Managing File Mounts
To effectively manage file mounts in Docker Compose and avoid issues related to missing files, consider the following best practices:
- Ensure File Existence: Always check that the files you intend to mount exist on the host. This can be enforced through scripts or CI/CD processes.
- Use Default Values: In your application, implement default values or error handling for cases where critical configuration files are missing.
- Utilize Volume Mounts: If possible, use volume mounts for directories where files may be created dynamically, rather than relying on specific file mounts.
- Document Dependencies: Clearly document any file dependencies in your project’s README or setup documentation to ensure all team members are aware.
Example Configuration
Here is an example of a `docker-compose.yml` configuration that demonstrates how to handle file mounts effectively:
“`yaml
version: ‘3.8’
services:
app:
image: myapp:latest
volumes:
- ./config/config.yml:/app/config.yml
“`
In this example, the `config.yml` file is expected to be present in the `./config` directory. If it is missing, Docker will create an empty file at `/app/config.yml` in the container.
Managing Errors and Logging
To address potential issues with missing files, you can implement logging within your application. This allows you to capture when a file is not found and handle it gracefully. Here’s a simple approach to logging file existence:
“`python
import os
import logging
logging.basicConfig(level=logging.INFO)
config_path = ‘/app/config.yml’
if not os.path.exists(config_path):
logging.warning(f”Configuration file not found: {config_path}. Using defaults.”)
“`
This snippet checks for the existence of the configuration file and logs a warning if it is missing, allowing the application to continue running with default settings.
By adhering to these practices and understanding Docker’s behavior regarding file mounts, you can enhance the reliability and maintainability of your applications.
Understanding Volume Mounts in Docker Compose
In Docker Compose, volume mounts are essential for sharing files and directories between the host and containers. When specifying a volume mount in your `docker-compose.yml` file, you can point to a file or directory on the host. However, if the specified file does not exist, Docker handles this situation in a specific manner.
Behavior When Mounting Non-Existent Files
When you attempt to mount a file that does not exist on the host:
- Docker Creates the File: If the specified file path does not exist on the host, Docker will create an empty file at that location. This behavior is particularly useful for configuration files or logs that the application expects to exist.
- Container Access: The container will have access to this empty file, but it may not contain any initial data, which could lead to unexpected behavior depending on the application’s requirements.
Example Configuration
Here is a sample `docker-compose.yml` configuration demonstrating how to mount a non-existent file:
“`yaml
version: ‘3.8’
services:
app:
image: my-app-image
volumes:
- ./config/myconfig.conf:/etc/myapp/myconfig.conf
“`
In this example, if `myconfig.conf` does not exist in the `./config` directory on the host, Docker will create it as an empty file when the container starts.
Best Practices for Mounting Non-Existent Files
When working with non-existent file mounts, consider the following best practices:
- Pre-create Required Files: If your application requires specific configurations, it is often better to pre-create these files with default values to avoid runtime issues.
- Use Default Values: Modify your application to handle cases where the configuration file is empty, allowing it to fallback to default settings.
- Error Handling: Implement robust error handling in your application to manage scenarios where expected files are missing or empty.
Common Use Cases
Mounting non-existent files can be beneficial in various scenarios:
- Configuration Files: Applications that rely on configuration files can have these files created at runtime, allowing for flexibility in deployment.
- Logs: When logging to a file, you can specify a log file path that does not exist, letting Docker create it automatically.
- Data Persistence: For databases or applications that require initial data files, setting up a script to create these files if they don’t exist can be part of the container’s entry point.
Testing and Validation
To ensure that your application behaves correctly when mounting non-existent files, follow these steps:
- Local Testing: Run your Docker Compose setup locally to confirm that the expected files are created and that the application functions as intended.
- Error Logs: Monitor application logs for any errors related to missing configurations or files to ensure that the application can handle empty files gracefully.
- Automated Tests: Incorporate automated tests that check the presence and content of mounted files after container startup.
By understanding how Docker Compose handles non-existent file mounts and following best practices, you can create more robust and flexible Docker configurations.
Expert Insights on Mounting Non-Existent Files in Docker Compose
Dr. Emily Carter (DevOps Engineer, Cloud Innovations Inc.). “When working with Docker Compose, attempting to mount a file that does not exist can lead to confusion. It is essential to understand that Docker will create the file at the specified path if it is not present, but this behavior can vary based on the underlying filesystem and permissions. Always ensure the necessary directory structure exists prior to deployment to avoid unexpected issues.”
Michael Chen (Senior Software Architect, Tech Solutions Group). “Mounting a non-existent file in Docker Compose can be a useful feature for initializing configuration files. However, developers should be cautious and implement checks to ensure that the application can handle the absence of the file gracefully. This practice can prevent runtime errors and improve the robustness of the application.”
Sarah Lopez (Containerization Specialist, Open Source Technologies). “In my experience, when a file is not found during a Docker Compose mount, it is crucial to have proper logging and error handling in place. This allows teams to diagnose issues quickly and adapt their deployment strategies accordingly. Understanding how Docker handles mounts can significantly streamline the development process.”
Frequently Asked Questions (FAQs)
Can I mount a file that does not exist in Docker Compose?
Yes, you can specify a file for mounting in Docker Compose even if it does not exist. Docker will create an empty file at the specified path within the container when it starts.
What happens if I mount a non-existent file in Docker Compose?
If the file does not exist on the host, Docker creates an empty file in the container. This allows your application to write to it as needed, but it will not contain any initial data.
How do I specify a non-existent file in my Docker Compose file?
You can specify the file path in the `volumes` section of your Docker Compose file. For example:
“`yaml
volumes:
- ./path/to/nonexistent-file.txt:/path/in/container.txt
“`
Will mounting a non-existent file affect container performance?
Mounting a non-existent file typically does not impact performance. However, the application may need to handle the absence of initial content appropriately.
Can I mount a directory that does not exist in Docker Compose?
Yes, you can mount a non-existent directory. Docker will create the directory on the host when the container starts, allowing for file creation and manipulation within that directory.
How can I verify if a file was created after mounting?
You can check the specified path within the container using the `docker exec` command to access the container’s shell and verify the existence of the file. For example:
“`bash
docker exec -it
“`
In the context of Docker Compose, mounting a file that does not exist can lead to various challenges, particularly when it comes to ensuring that the containerized applications function as intended. Docker Compose allows users to define and run multi-container Docker applications, and the volume mounting feature is integral to this process. When a file specified for mounting is not present on the host system, Docker Compose will create an empty file or directory at the mount point, which may not always align with the application’s requirements. This behavior can lead to unexpected runtime errors or misconfigurations within the application.
One key takeaway is the importance of preemptively managing the files and directories that are required by your containers. Ensuring that all necessary files exist on the host before starting the Docker Compose services can prevent issues related to missing configurations or data. Additionally, utilizing Docker Compose’s built-in features, such as the `volumes` directive, can help streamline the process of managing file mounts, allowing for better organization and clarity in your Docker configurations.
Moreover, understanding the implications of mounting non-existent files highlights the need for thorough testing and validation of Docker Compose setups. Developers should adopt practices such as automated checks or scripts to verify the existence of required files prior to deployment. This
Author Profile
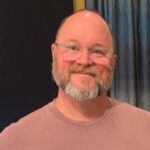
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?