Is Close Python the Right Tool for Your Next Project?
In the ever-evolving landscape of programming languages, Python stands out as a versatile and user-friendly option that has captured the hearts of developers worldwide. Among its many features, the concept of “closing” in Python plays a crucial role in managing resources and ensuring that applications run smoothly. Whether you’re a seasoned programmer or just starting your coding journey, understanding how to effectively close files, connections, and other resources in Python is essential for building robust applications. This article delves into the intricacies of closing mechanisms in Python, shedding light on best practices and common pitfalls.
When working with Python, managing resources efficiently is paramount. The language provides various constructs to handle the opening and closing of files, sockets, and other resources, ensuring that they are released properly after use. This not only prevents memory leaks but also enhances the overall performance of your applications. Python’s built-in functions and context managers, such as the `with` statement, simplify the process of resource management, allowing developers to focus on writing clean and effective code.
Moreover, understanding the implications of not closing resources can save you from potential headaches down the line. Improper handling can lead to data corruption, security vulnerabilities, and application crashes. By exploring the nuances of closing in Python, you’ll gain valuable insights that
Understanding the Close Function in Python
The `close()` function in Python is typically associated with file handling, specifically when you want to terminate the use of a file object. It is essential to properly close files to ensure that all resources are freed and that any changes made to the file are saved correctly. Failing to close a file can lead to memory leaks and potential data corruption.
When you open a file, Python allocates resources for that file. The `close()` method is used to release these resources. Here is a simple example of how to use the `close()` method:
“`python
file = open(‘example.txt’, ‘w’)
file.write(‘Hello, World!’)
file.close()
“`
In this snippet, the file is opened in write mode, some text is written to it, and then it is closed to free up system resources.
Using the with Statement for File Handling
A more efficient and safer way to manage file operations in Python is by using the `with` statement. This approach automatically handles closing the file, even if an exception occurs during file operations. The `with` statement simplifies the code and enhances readability. Here’s how it works:
“`python
with open(‘example.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
“`
In this example, the file will be automatically closed once the block of code under the `with` statement is exited, regardless of whether an error occurred.
Best Practices for File Closure
When working with files in Python, adhere to the following best practices to ensure proper resource management:
- Always close files after completing operations using `close()` or the `with` statement.
- Check if a file is open before attempting to close it.
- Handle exceptions to avoid leaving files open unintentionally.
Common Errors Related to File Closure
When using the `close()` method, there are several common pitfalls that developers may encounter:
Error Type | Description |
---|---|
Attempting to close a closed file | Raises a `ValueError` indicating that the file is already closed. |
Not closing a file | Can lead to memory leaks and data loss. |
Improper error handling | Failing to manage exceptions can leave files open. |
To avoid these errors, consider implementing error handling using try-except blocks:
“`python
try:
file = open(‘example.txt’, ‘w’)
file.write(‘Hello, World!’)
except Exception as e:
print(f’An error occurred: {e}’)
finally:
file.close()
“`
This pattern ensures that the file is closed properly, even if an error occurs during the write operation.
Conclusion on File Closure
Understanding the importance of the `close()` function and adopting best practices in file handling are crucial for efficient programming in Python. By utilizing the `with` statement and being mindful of potential errors, you can ensure that your file operations are both effective and safe.
Understanding the Close Function in Python
In Python, the `close()` function is integral for managing resources, particularly when dealing with file operations or network connections. It ensures that resources are released properly, preventing memory leaks or resource exhaustion.
File Handling with Close
When working with files in Python, it is crucial to close the file after its operations are complete. This is done using the `close()` method of the file object.
- Syntax: `file_object.close()`
- Importance:
- Frees up system resources.
- Ensures that data is written to the disk.
- Prevents data corruption.
Example of File Handling
Here is a practical example demonstrating the use of the `close()` function in file handling:
“`python
Open a file for writing
file = open(‘example.txt’, ‘w’)
file.write(‘Hello, World!’)
file.close() Closing the file
“`
Alternatively, using the `with` statement automatically handles closing:
“`python
with open(‘example.txt’, ‘w’) as file:
file.write(‘Hello, World!’)
No need to explicitly call close()
“`
Networking and Close Functionality
In network programming, closing connections is equally essential. For instance, when using sockets, the `close()` method ensures that the socket is properly closed.
- Syntax: `socket.close()`
- Consequences of Not Closing:
- Potential memory leaks.
- Unreleased ports leading to “address already in use” errors.
Socket Example
Consider the following example of a socket connection:
“`python
import socket
Create a socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((‘localhost’, 8080))
Perform network operations…
s.close() Closing the socket connection
“`
Best Practices for Using Close
To ensure efficient resource management, adhere to the following best practices:
- Always use the `close()` method after file or network operations.
- Prefer using context managers (`with` statement) for automatic resource management.
- Implement exception handling to ensure `close()` is called even in error scenarios:
“`python
try:
file = open(‘example.txt’, ‘r’)
Read operations…
finally:
file.close() This ensures the file is closed
“`
Table: Common Scenarios for Using Close
Scenario | Resource Type | Method Call |
---|---|---|
File Operations | File | `file.close()` |
Network Connections | Socket | `socket.close()` |
Database Connections | Database Cursor | `cursor.close()` |
Threads | Thread | `thread.join()` |
Utilizing the `close()` method appropriately is key to maintaining optimal performance and resource efficiency in Python applications.
Expert Insights on the Use of Close in Python Programming
Dr. Emily Chen (Senior Python Developer, Tech Innovations Inc.). “The ‘close’ function in Python is essential for resource management, particularly when working with file I/O operations. It ensures that files are properly closed after their use, preventing memory leaks and potential data corruption.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “Understanding the importance of the ‘close’ method in Python is crucial for developers. Neglecting to close resources can lead to performance issues, especially in applications that handle multiple files or network connections.”
Sarah Patel (Python Programming Instructor, LearnCode Academy). “In my experience teaching Python, I emphasize the use of ‘close’ in file handling. It is a fundamental practice that not only enhances code reliability but also instills good habits in new programmers.”
Frequently Asked Questions (FAQs)
Is Close Python a programming language?
Close Python is not a standalone programming language. It refers to the concept of closing resources or connections in Python programming, ensuring efficient memory management and resource utilization.
How do you close a file in Python?
You can close a file in Python using the `close()` method on a file object. For example, `file_object.close()` releases the file resource and ensures that all data is written to disk.
What is the purpose of using ‘with’ statement in Python?
The ‘with’ statement in Python is used for resource management and exception handling. It automatically closes resources, such as files, when the block of code is exited, even if an error occurs.
Can you close a database connection in Python?
Yes, you can close a database connection in Python using the `close()` method on the connection object. This action is crucial for freeing up database resources and preventing memory leaks.
Is there a way to ensure all resources are closed in Python?
Yes, using context managers (the ‘with’ statement) ensures that all resources are properly closed after their use. This approach minimizes the risk of resource leaks in your application.
What happens if you forget to close a file in Python?
Forgetting to close a file in Python can lead to memory leaks and data corruption. It may also prevent other processes from accessing the file until the program terminates or the operating system closes it automatically.
the term “Is Close Python” likely refers to discussions surrounding the Python programming language and its capabilities in various domains, including data analysis, machine learning, web development, and automation. Python’s syntax and versatility make it an attractive option for both beginners and experienced developers. Its extensive libraries and frameworks further enhance its functionality, allowing for rapid development and deployment of applications.
Moreover, Python’s community support and continuous evolution contribute significantly to its popularity. The language’s ability to integrate with other technologies and platforms ensures that it remains relevant in an ever-changing tech landscape. As businesses increasingly adopt data-driven strategies, Python’s role as a primary tool for data manipulation and analysis becomes even more critical.
Key takeaways from the discussion include the importance of Python’s simplicity and readability, which facilitate learning and collaboration among developers. Additionally, the language’s adaptability to various fields, from web services to scientific computing, underscores its significance in modern programming. Overall, Python’s robust ecosystem and ongoing development position it as a leading choice for a wide array of programming tasks.
Author Profile
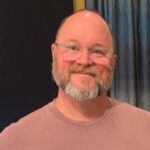
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?