How Can You Create a Basic Makefile for Compiling a Static Library?
In the world of software development, efficiency and organization are paramount, especially when it comes to managing complex projects. One essential tool that developers often rely on is the Makefile, a powerful script that automates the build process. For those venturing into the realm of C or C++ programming, understanding how to create a basic Makefile for compiling a static library can significantly streamline your workflow. Whether you’re a seasoned programmer or a newcomer eager to learn, mastering this skill will not only enhance your productivity but also deepen your understanding of how libraries function within your code.
A static library serves as a collection of object files that can be linked into your programs, providing reusable code without the overhead of dynamic linking. Crafting a Makefile to compile such a library involves defining rules and dependencies, which can seem daunting at first. However, once you grasp the fundamental structure and syntax, you’ll find that it empowers you to manage your builds with precision and ease. This article will guide you through the essential components of a Makefile, illustrating how to specify compilation commands, manage dependencies, and optimize your build process.
As we delve into the intricacies of creating a basic Makefile for a static library, you’ll discover best practices that can help you avoid common pitfalls and enhance your
Understanding Static Libraries
Static libraries are collections of object files that are linked into a program during the linking phase of compilation. Unlike dynamic libraries, static libraries are included directly into the executable, which can lead to larger file sizes but eliminates dependencies on external files at runtime. This approach can enhance performance and simplify deployment, as all necessary code is packaged within the binary.
When compiling static libraries, it’s essential to keep the following in mind:
- Static libraries typically have the `.a` file extension on Unix-like systems.
- The creation process involves compiling source files into object files, followed by archiving these object files into a static library using tools such as `ar` (archiver).
- The linking process is different from dynamic libraries, where the library’s code is not resolved until runtime.
Basic Structure of a Makefile for Static Libraries
A Makefile automates the build process, defining rules for compiling source files and creating the library. The basic structure of a Makefile for a static library includes variables for the compiler, flags, the target library name, and source files. Below is a sample Makefile that illustrates these components:
“`makefile
CC = gcc
AR = ar
CFLAGS = -Wall -g
ARFLAGS = rcs
TARGET = libmystatic.a
SOURCES = file1.c file2.c file3.c
OBJECTS = $(SOURCES:.c=.o)
“`
Explanation of Variables:
- CC: Specifies the compiler to use (in this case, `gcc`).
- AR: Defines the archiver tool to create the static library (`ar`).
- CFLAGS: Compiler flags that enable warnings and debugging.
- ARFLAGS: Flags used with `ar` to create the archive.
- TARGET: Name of the output static library.
- SOURCES: Lists the source files to be compiled.
- OBJECTS: Automatically generates object file names from the source files.
Defining Build Rules
To compile and create the static library, you will need to define build rules in the Makefile. Here is an example of how to do this:
“`makefile
all: $(TARGET)
$(TARGET): $(OBJECTS)
$(AR) $(ARFLAGS) $@ $^
%.o: %.c
$(CC) $(CFLAGS) -c $< -o $@
```
Explanation of Rules:
- all: This is the default target that builds the static library when running `make`.
- $(TARGET): This rule specifies how to create the static library from the object files.
- %.o: %.c: This pattern rule tells `make` how to compile each source file into an object file.
Usage of the Makefile
To use the Makefile, simply navigate to the directory containing the Makefile in the terminal and run:
“`sh
make
“`
This command will execute the rules defined in the Makefile, compiling the source files and creating the static library specified in the `TARGET` variable.
Sample Output
When executed, the Makefile will generate an output similar to the following:
“`
gcc -Wall -g -c file1.c -o file1.o
gcc -Wall -g -c file2.c -o file2.o
gcc -Wall -g -c file3.c -o file3.o
ar rcs libmystatic.a file1.o file2.o file3.o
“`
This output indicates the compilation of each source file into an object file, followed by the creation of the static library.
Command | Description |
---|---|
make | Builds the static library and compiles the source files. |
gcc | Compiles the C source files into object files. |
ar | Creates the static library from the object files. |
This structured approach ensures that you can efficiently manage the compilation of static libraries, leveraging the power of Makefiles for automation and organization.
Understanding the Structure of a Makefile
A Makefile is a special file used by the `make` build automation tool to manage dependencies and compile programs. For a static library, the Makefile typically consists of several key components:
- Variables: Used to define compiler options, source files, and output names.
- Rules: Specify how to build targets from dependencies.
- Comments: Provide explanations within the Makefile, prefixed by “.
Here’s a basic structure for a Makefile used to compile a static library:
“`makefile
Compiler and flags
CC = gcc
CFLAGS = -Wall -g
Library name
LIB_NAME = libmylibrary.a
Source files
SRC = file1.c file2.c file3.c
Object files
OBJ = $(SRC:.c=.o)
Default target
all: $(LIB_NAME)
Rule to create the static library
$(LIB_NAME): $(OBJ)
ar rcs $@ $^
Rule to compile source files into object files
%.o: %.c
$(CC) $(CFLAGS) -c $< -o $@
Clean up build files
clean:
rm -f $(OBJ) $(LIB_NAME)
```
Key Components Explained
- Variables: The use of variables like `CC`, `CFLAGS`, and `LIB_NAME` allows for easy modifications. For instance, changing the compiler or flags only requires updating the variable definition.
- Pattern Rules: The pattern rule `%.o: %.c` streamlines the compilation process by specifying how to create `.o` files from `.c` files. The `$<` represents the first prerequisite, and `$@` refers to the target.
- Library Creation: The `ar` command is used to create the static library. The flags `r`, `c`, and `s` ensure that the library is created if it doesn’t exist, and that the symbols are indexed.
Using the Makefile
To utilize the Makefile, perform the following steps in your terminal:
- Navigate to the directory containing the Makefile.
- Execute the command:
“`bash
make
“`
This command invokes the default target, which compiles the source files and generates the static library `libmylibrary.a`.
Additional Considerations
When creating a Makefile for a static library, consider the following:
- Dependency Management: Although not covered in the basic Makefile, tracking dependencies can prevent unnecessary recompilation. Tools like `makedep` can help automate this process.
- Version Control: To maintain a clean build environment, include versioning in your library name (e.g., `libmylibrary-v1.0.a`).
- Cross-Platform Compatibility: If targeting multiple platforms, consider using conditional variables to adapt the compiler and flags accordingly.
- Debugging: Utilize the `-g` flag in `CFLAGS` for debugging support. This enables the generation of debugging information in the compiled objects, which is crucial during development.
By understanding these components and considerations, you can effectively manage the compilation of static libraries using Makefiles in various projects.
Expert Insights on Creating a Basic Makefile for Compiling Static Libraries
Dr. Emily Chen (Senior Software Engineer, Open Source Projects). “A well-structured Makefile is essential for managing dependencies and ensuring consistent builds. When compiling a static library, it is crucial to define clear targets and utilize variables for compiler options to maintain flexibility and scalability in your project.”
Mark Thompson (Lead Developer, Tech Innovations Inc.). “Using a basic Makefile to compile static libraries can significantly streamline your build process. I recommend including rules for both compilation and archiving, as well as utilizing the ‘clean’ target to remove unnecessary files, which helps in maintaining a clutter-free workspace.”
Linda Garcia (Technical Writer, Software Development Journal). “Documentation within your Makefile is often overlooked but is vital for collaboration. Including comments that explain each target and variable will not only assist others in understanding the build process but also aid in future maintenance and updates to the library.”
Frequently Asked Questions (FAQs)
What is a Makefile?
A Makefile is a special file used by the `make` build automation tool to define how to compile and link a program. It contains a set of rules and dependencies that dictate how source code files are processed.
How do I create a basic Makefile for compiling a static library?
To create a basic Makefile for a static library, define the source files, object files, and the target library name. Use rules to compile the object files from the source files and then archive them into a static library using the `ar` command.
What is a static library?
A static library is a collection of object files that are linked into a program during the linking phase of compilation. Unlike dynamic libraries, static libraries are included in the executable, making them self-contained.
What commands are typically included in a Makefile for a static library?
Typical commands include `CC` for the compiler, `AR` for creating the archive, and `CFLAGS` for compiler flags. You would also include rules for compiling object files and archiving them into a static library.
Can you provide an example of a simple Makefile for a static library?
Certainly. Here is a simple example:
“`
CC = gcc
AR = ar
CFLAGS = -Wall -g
SRC = file1.c file2.c
OBJ = $(SRC:.c=.o)
TARGET = libmylibrary.a
all: $(TARGET)
$(TARGET): $(OBJ)
$(AR) rcs $@ $^
%.o: %.c
$(CC) $(CFLAGS) -c $< -o $@
```
How do I use the Makefile to compile my static library?
To compile your static library using the Makefile, navigate to the directory containing the Makefile in the terminal and run the command `make`. This will execute the rules defined in the Makefile and generate the static library.
In summary, a basic Makefile for compiling a static library serves as an essential tool for automating the build process in C or C++ projects. It streamlines the compilation of object files from source code and facilitates the creation of a static library archive. By defining clear rules and dependencies, a Makefile enhances efficiency and reduces the likelihood of errors during the build process.
Key components of a Makefile include variable definitions for compiler flags, source files, and output libraries. The use of pattern rules allows for flexibility in handling multiple source files, while the inclusion of clean targets ensures that the build environment can be reset easily. These elements collectively contribute to a well-organized and maintainable build system.
Moreover, understanding how to structure a Makefile for static library compilation not only aids in project management but also fosters best practices in software development. It encourages developers to adopt systematic approaches to building and linking libraries, which can significantly enhance collaboration and code reusability across projects.
Author Profile
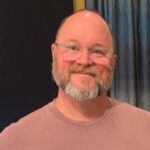
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?