Why Do We Use If Statements in JavaScript: Exploring Their Importance and Functionality
In the world of programming, decision-making is a fundamental aspect that shapes how applications respond to user inputs and environmental conditions. JavaScript, a cornerstone language of web development, employs a powerful tool known as the “if statement” to facilitate this decision-making process. Whether you’re crafting a simple website or developing a complex web application, understanding the role of if statements is crucial for creating dynamic, interactive experiences. But what exactly are if statements, and why are they so integral to JavaScript programming?
At its core, an if statement allows developers to execute specific blocks of code based on whether a certain condition is true or . This capability enables programmers to build logic into their applications, allowing for tailored responses to various scenarios. From validating user input to controlling the flow of data, if statements are the backbone of conditional logic in JavaScript, making them indispensable for any coder looking to enhance their programming prowess.
As we delve deeper into the significance of if statements in JavaScript, we will explore their syntax, functionality, and practical applications. By understanding how to effectively implement these statements, you will unlock the ability to create more responsive and intelligent applications that can adapt to the needs of users and the environment. Join us as we unravel the intricacies of if statements and discover why
Understanding Conditional Logic
If statements in JavaScript are fundamental for implementing conditional logic, allowing developers to execute different blocks of code based on specific conditions. This capability is essential for creating interactive applications and dynamic web pages. The power of if statements lies in their ability to evaluate boolean expressions, which can lead to various outcomes based on the truthiness or falsiness of the conditions provided.
Syntax of If Statements
The basic syntax of an if statement is straightforward and can be expanded with additional conditions using else if and else. The structure is as follows:
“`javascript
if (condition) {
// code to execute if condition is true
} else if (anotherCondition) {
// code to execute if anotherCondition is true
} else {
// code to execute if all conditions are
}
“`
This structure allows for a clear and readable flow of logic. Each condition is evaluated in order, and the first true condition’s corresponding block of code will be executed.
Examples of If Statements
To illustrate the use of if statements, consider the following examples:
“`javascript
let age = 18;
if (age < 18) { console.log("You are a minor."); } else if (age === 18) { console.log("You just turned adult."); } else { console.log("You are an adult."); } ``` In this example, the output will be "You just turned adult." because the condition evaluates to true.
Use Cases for If Statements
If statements can be employed in various scenarios, including but not limited to:
- User Authentication: Validate user credentials and determine access levels.
- Input Validation: Check user inputs in forms and provide feedback.
- Feature Toggles: Enable or disable features based on user preferences or settings.
Logical Operators with If Statements
JavaScript supports several logical operators that can be used in conjunction with if statements to create complex conditions. These include:
- AND (`&&`): Both conditions must be true.
- OR (`||`): At least one condition must be true.
- NOT (`!`): Negates the condition.
Here’s how these operators can be applied:
“`javascript
let isLoggedIn = true;
let hasPermission = ;
if (isLoggedIn && hasPermission) {
console.log(“Access granted.”);
} else {
console.log(“Access denied.”);
}
“`
In this case, “Access denied.” will be logged because the second condition is .
Table of Comparison Operators
Understanding comparison operators is crucial for creating effective if statements. The following table summarizes common comparison operators used in JavaScript:
Operator | Description | Example |
---|---|---|
== | Equal to (loose comparison) | 5 == ‘5’ // true |
=== | Equal to (strict comparison) | 5 === ‘5’ // |
!= | Not equal to (loose comparison) | 5 != ‘6’ // true |
!== | Not equal to (strict comparison) | 5 !== 5 // |
> | Greater than | 5 > 3 // true |
< | Less than | 5 < 7 // true |
Utilizing if statements along with these comparison operators allows developers to create robust, responsive applications that react appropriately to user inputs and other dynamic factors.
Purpose of If Statements in JavaScript
If statements serve a fundamental role in programming, particularly in JavaScript, allowing developers to implement decision-making capabilities within their code. The main purposes of using if statements include:
- Conditional Execution: If statements enable the execution of code blocks based on specific conditions. This allows the program to react differently under varying circumstances.
- Flow Control: They help control the flow of execution in a program, directing the path taken based on boolean evaluations.
- Dynamic Behavior: If statements allow for dynamic behavior in applications, enabling them to adapt based on user input, external data, or other runtime conditions.
Syntax of If Statements
The basic syntax of an if statement in JavaScript is as follows:
“`javascript
if (condition) {
// Code to execute if the condition is true
}
“`
This structure can be expanded with else and else if clauses to handle multiple conditions:
“`javascript
if (condition1) {
// Code to execute if condition1 is true
} else if (condition2) {
// Code to execute if condition2 is true
} else {
// Code to execute if neither condition is true
}
“`
Examples of If Statements
To illustrate the application of if statements, consider the following examples:
- **Basic Condition Check**:
“`javascript
let age = 18;
if (age >= 18) {
console.log(“You are an adult.”);
}
“`
- **Multiple Conditions**:
“`javascript
let score = 85;
if (score >= 90) {
console.log(“Grade: A”);
} else if (score >= 80) {
console.log(“Grade: B”);
} else {
console.log(“Grade: C or lower”);
}
“`
- **Using Logical Operators**:
“`javascript
let temperature = 30;
if (temperature > 20 && temperature < 35) {
console.log("It's a pleasant day.");
}
```
Common Use Cases of If Statements
If statements are widely used in various scenarios, including:
- User Input Validation:
- Checking if input values meet certain criteria (e.g., form validation).
- Game Logic:
- Determining outcomes based on player actions or game states.
- Conditional Rendering in Web Applications:
- Displaying different UI elements based on user permissions or application state.
Best Practices for Using If Statements
To enhance code readability and maintainability, consider the following best practices when using if statements:
Best Practice | Description |
---|---|
Keep Conditions Simple | Avoid complex conditions; break them into smaller checks. |
Use Descriptive Variable Names | Clear variable names improve understanding of conditions. |
Limit Nesting | Avoid deep nesting of if statements for clarity. |
Utilize Ternary Operators | For simple conditions, consider using the ternary operator for brevity. |
The utilization of if statements in JavaScript is crucial for implementing logic and control flow in applications. Their flexibility allows developers to create responsive and adaptable code that enhances the user experience.
Understanding the Importance of If Statements in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). If statements are fundamental in JavaScript as they allow developers to implement conditional logic, enabling the program to make decisions based on varying inputs and states. This capability is essential for creating dynamic and responsive applications that react to user interactions.
Michael Chen (Lead JavaScript Developer, CodeCraft Solutions). The use of if statements in JavaScript is crucial for controlling the flow of execution in a program. They provide a mechanism to execute certain blocks of code only when specific conditions are met, which is vital for error handling and ensuring that applications behave as expected under different scenarios.
Sarah Patel (JavaScript Educator, Web Development Academy). If statements are a cornerstone of programming logic in JavaScript, allowing developers to create complex decision-making processes. Their versatility enables the implementation of features like form validation, user authentication, and interactive user interfaces, making them indispensable in modern web development.
Frequently Asked Questions (FAQs)
Why do we use if statements in JavaScript?
If statements are used in JavaScript to execute a block of code conditionally. They allow developers to control the flow of execution based on whether a specified condition evaluates to true or .
How does an if statement work in JavaScript?
An if statement evaluates a condition within parentheses. If the condition is true, the code block within the curly braces executes. If , the code block is skipped.
Can if statements be nested in JavaScript?
Yes, if statements can be nested within one another. This allows for more complex conditional logic, enabling developers to check multiple conditions in a structured manner.
What are the alternatives to if statements in JavaScript?
Alternatives to if statements include switch statements for multiple conditions and ternary operators for simple conditional expressions. Both provide different ways to handle conditional logic.
What is the difference between if and else if statements?
An if statement checks a condition, while an else if statement allows for additional conditions to be evaluated if the previous if condition is . This enables multiple branches of logic to be handled sequentially.
Can if statements be used with logical operators in JavaScript?
Yes, if statements can utilize logical operators such as AND (&&) and OR (||) to combine multiple conditions. This enhances the flexibility and complexity of the conditions being evaluated.
If statements in JavaScript are fundamental constructs that enable developers to control the flow of execution in their programs. By allowing for conditional logic, if statements facilitate decision-making processes within the code. This capability is essential for creating dynamic applications that respond to user input or other changing conditions. The use of if statements helps to ensure that certain blocks of code are executed only when specific criteria are met, thereby enhancing the functionality and interactivity of web applications.
Moreover, if statements contribute to the readability and maintainability of code. By clearly defining conditions under which certain actions should occur, developers can create more organized and understandable code structures. This clarity is particularly important in larger projects where multiple developers may be collaborating. Well-structured if statements can help prevent errors and make it easier for others to follow the logic of the code.
In summary, the use of if statements in JavaScript is crucial for implementing conditional logic, which is a cornerstone of programming. They empower developers to create responsive and interactive applications while promoting code clarity and maintainability. Understanding how to effectively utilize if statements is an essential skill for any JavaScript developer, as it lays the groundwork for more complex programming concepts and structures.
Author Profile
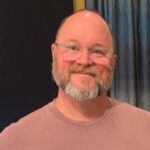
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?