Why Are Control Structures Essential in JavaScript?
In the dynamic world of JavaScript programming, control structures serve as the backbone of logic and decision-making. These essential components allow developers to dictate the flow of their code, enabling it to respond intelligently to varying conditions and inputs. Whether you’re crafting a simple web application or a complex interactive feature, understanding how to effectively utilize control structures is crucial for creating efficient and maintainable code. Join us as we delve into the fascinating realm of control structures in JavaScript, exploring their significance and the powerful capabilities they unlock for developers.
Control structures in JavaScript encompass a variety of tools that manage the execution path of a program. They include conditional statements, loops, and branching mechanisms that guide the code on what to execute based on specific criteria. By leveraging these structures, developers can implement logic that allows their applications to make decisions, repeat tasks, and handle various scenarios seamlessly. This not only enhances the functionality of applications but also contributes to a more organized and readable codebase.
As we explore the different types of control structures, you’ll discover how they empower you to write more dynamic and responsive code. From simple if-else statements that manage conditional logic to sophisticated loops that automate repetitive tasks, each control structure plays a vital role in shaping the overall behavior of your JavaScript applications. Understanding these
Control Structures in JavaScript
Control structures are fundamental components of JavaScript that allow developers to dictate the flow of execution in their programs. By utilizing these structures, programmers can create complex logic that can handle various scenarios based on specific conditions. The primary types of control structures in JavaScript include conditional statements, loops, and exception handling.
Conditional Statements
Conditional statements enable the execution of specific code blocks based on whether a condition evaluates to true or . JavaScript provides several forms of conditional statements, including:
– **if Statement**: Executes a block of code if the specified condition is true.
– **if…else Statement**: Executes one block of code if the condition is true and another block if it is .
– **switch Statement**: Allows the execution of different code blocks based on the value of a variable or expression.
Example of an if-else statement:
“`javascript
let age = 18;
if (age >= 18) {
console.log(“Adult”);
} else {
console.log(“Minor”);
}
“`
Loops
Loops are control structures that allow code to be executed repeatedly based on a condition. JavaScript includes several types of loops:
- for Loop: Executes a block of code a specified number of times.
- while Loop: Continues to execute a block of code as long as the specified condition is true.
- do…while Loop: Similar to the while loop, but it guarantees that the code block will execute at least once.
Example of a for loop:
“`javascript
for (let i = 0; i < 5; i++) {
console.log(i);
}
```
Exception Handling
Exception handling allows developers to manage errors gracefully in their applications. JavaScript provides try…catch statements to handle exceptions that may occur during execution. This enables a program to continue running even if an error is encountered.
The structure of try…catch is as follows:
“`javascript
try {
// Code that may throw an error
} catch (error) {
// Code to handle the error
}
“`
Comparison of Control Structures
Below is a table summarizing the different types of control structures in JavaScript, their purpose, and typical use cases:
Control Structure | Purpose | Typical Use Case |
---|---|---|
if Statement | Execute code based on a condition | Decision making |
for Loop | Repeat code a set number of times | Iterating over arrays |
while Loop | Repeat code while a condition is true | Waiting for user input |
switch Statement | Select among multiple cases | Menu selection |
try…catch | Handle errors | Graceful error recovery |
By mastering these control structures, developers can implement robust and flexible logic in their JavaScript applications, enhancing functionality and user experience.
Purpose of Control Structures in JavaScript
Control structures in JavaScript are fundamental for directing the flow of a program’s execution. They allow developers to create complex behaviors and manage decision-making processes effectively. The primary purposes of control structures include:
- Conditional Execution: Control structures enable the execution of specific blocks of code based on certain conditions. This is vital for implementing logic that changes based on user input or data state.
- Iteration: They facilitate the repetition of code blocks, allowing for efficient processing of collections or repeated tasks without redundancy. This is essential in scenarios such as looping through arrays or executing tasks a predefined number of times.
- Branching: Control structures provide the ability to branch the execution path of a program. This is crucial for building applications that require different responses based on varying conditions or states.
Types of Control Structures
JavaScript primarily utilizes three types of control structures: conditional statements, loops, and exception handling. Each serves a distinct purpose and can be used in combination to create robust applications.
Conditional Statements
Conditional statements allow the execution of code based on specific conditions. The most common forms include:
- if statement: Executes a block of code if a specified condition evaluates to true.
“`javascript
if (condition) {
// Code to execute if condition is true
}
“`
- else statement: Provides an alternative block of code to execute if the associated condition is .
“`javascript
if (condition) {
// Code if condition is true
} else {
// Code if condition is
}
“`
- else if statement: Enables multiple conditions to be checked sequentially.
“`javascript
if (condition1) {
// Code if condition1 is true
} else if (condition2) {
// Code if condition2 is true
} else {
// Code if none are true
}
“`
- switch statement: A more efficient alternative for multiple conditions based on a single variable.
“`javascript
switch (expression) {
case value1:
// Code for value1
break;
case value2:
// Code for value2
break;
default:
// Code if no cases match
}
“`
Loops
Loops allow repeated execution of a block of code while a specific condition holds true. Common loop types include:
- for loop: Executes a block of code a specified number of times.
“`javascript
for (let i = 0; i < 5; i++) {
// Code to repeat
}
```
- while loop: Continues execution as long as the specified condition remains true.
“`javascript
while (condition) {
// Code to repeat
}
“`
- do…while loop: Similar to the while loop, but guarantees at least one execution of the code block.
“`javascript
do {
// Code to execute
} while (condition);
“`
Exception Handling
JavaScript provides mechanisms for handling errors and exceptions using `try`, `catch`, and `finally`. This structure helps maintain program stability and manage unexpected issues.
- try block: Contains code that might throw an error.
“`javascript
try {
// Code that may throw an error
} catch (error) {
// Handle the error
} finally {
// Code that runs regardless of error occurrence
}
“`
Utilizing these control structures effectively allows developers to create dynamic and responsive applications, providing a better user experience and maintaining code clarity.
Understanding the Role of Control Structures in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Control structures are fundamental to JavaScript as they dictate the flow of execution in a program. They allow developers to create dynamic and responsive applications by enabling conditional logic and looping mechanisms, which are essential for handling user interactions and data processing.
Mark Thompson (JavaScript Framework Specialist, CodeMaster Academy). In JavaScript, control structures such as if statements, loops, and switch cases are crucial for managing the complexity of code. They empower developers to write more efficient and readable code by clearly defining how and when certain blocks of code should execute based on specific conditions.
Lisa Chen (Lead JavaScript Developer, Web Solutions Corp.). The use of control structures in JavaScript is vital for implementing algorithms and handling asynchronous operations. They enable developers to control the execution flow, making it possible to manage tasks like API calls and user input validation effectively, which enhances the overall user experience.
Frequently Asked Questions (FAQs)
What are control structures in JavaScript?
Control structures in JavaScript are constructs that dictate the flow of execution in a program. They include conditional statements, loops, and branching mechanisms, allowing developers to control how and when certain blocks of code are executed.
What do we use conditional statements for in JavaScript?
Conditional statements, such as `if`, `else if`, and `switch`, are used to execute specific code blocks based on certain conditions. They enable decision-making in programs, allowing for different outcomes depending on variable values or user input.
How do loops function as control structures in JavaScript?
Loops, including `for`, `while`, and `do…while`, are used to repeatedly execute a block of code as long as a specified condition is true. They are essential for iterating over arrays or performing repetitive tasks efficiently.
What is the purpose of the `break` and `continue` statements in loops?
The `break` statement terminates the loop entirely, while the `continue` statement skips the current iteration and proceeds to the next one. Both are used to control the flow of loops based on specific conditions.
Can you provide an example of using control structures in a real-world scenario?
In a web application, control structures can be used to validate user input. For instance, an `if` statement can check if a user has entered a valid email address, and a `for` loop can iterate through a list of items to display them on the screen.
How do control structures improve code readability and maintainability?
Control structures enhance code readability by organizing logic clearly, making it easier for developers to understand the flow of execution. They also promote maintainability by allowing changes to be made in one location without affecting the overall program structure.
Control structures in JavaScript are fundamental components that dictate the flow of execution within a program. They enable developers to implement decision-making processes, execute repetitive tasks, and manage the overall logic of applications. By utilizing control structures such as conditional statements, loops, and switch cases, programmers can create dynamic and responsive code that reacts to varying conditions and user inputs.
One of the primary uses of control structures is to control the execution path based on specific conditions. Conditional statements like ‘if’, ‘else if’, and ‘else’ allow developers to execute different blocks of code depending on the truthiness of given expressions. This capability is crucial for creating interactive applications that respond appropriately to user actions or data states.
Loops, another essential type of control structure, facilitate the execution of a block of code multiple times. Constructs such as ‘for’, ‘while’, and ‘do…while’ loops are invaluable for performing repetitive tasks efficiently, such as iterating over arrays or processing collections of data. This not only reduces code redundancy but also enhances performance by minimizing the need for manual repetition.
control structures are vital for effective programming in JavaScript, as they empower developers to construct logical, efficient, and interactive applications. Mastery
Author Profile
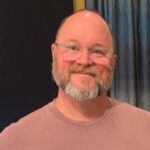
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?