Why Am I Seeing the Error ‘Property [Id] Does Not Exist On The Eloquent Builder Instance’ in Laravel?
In the world of web development, particularly when working with Laravel’s Eloquent ORM, developers often encounter a myriad of challenges that can disrupt their workflow. One such issue that can leave even seasoned programmers scratching their heads is the error message: “Property [Id] Does Not Exist On The Eloquent Builder Instance.” This seemingly cryptic notification can signal a deeper misunderstanding of how Eloquent interacts with database records and the nuances of object-oriented programming. Understanding this error is crucial for anyone looking to harness the full power of Laravel’s elegant syntax and robust database management capabilities.
At its core, this error typically arises when developers attempt to access a property that is not available on the Eloquent Builder instance. This can happen for various reasons, such as misconfigurations in model relationships, incorrect method calls, or simply a misunderstanding of how Eloquent handles data retrieval. By dissecting the underlying causes of this error, developers can not only resolve the immediate issue but also gain a clearer insight into the best practices for using Eloquent effectively.
As we delve deeper into this topic, we will explore the common pitfalls that lead to this error, the fundamental principles of Eloquent’s architecture, and how to properly interact with model instances. Whether you’re a novice developer just starting with Laravel or an
Understanding the Error
The error message “Property [Id] Does Not Exist On The Eloquent Builder Instance” typically indicates that there is an attempt to access a property on an Eloquent query builder object that does not exist. This can occur for several reasons, often related to the misuse of Eloquent methods or misunderstanding the object being worked with.
When using Eloquent in Laravel, it is essential to recognize the distinction between a query builder instance and an Eloquent model instance. A query builder instance is an object that is used to construct and execute database queries, while an Eloquent model instance represents a single record from a database table.
Common Causes
There are several common scenarios that lead to this error:
- Direct Access to Properties: Attempting to access properties directly on the query builder instead of the model instance.
- Improper Method Chaining: When methods are chained incorrectly, the resulting object may not be what is expected.
- Missing Select Statement: If a select statement is not specified, the query may not return the expected attributes.
- Using `get()` Instead of `first()`: Using `get()` returns a collection, while `first()` returns a single model instance. Accessing properties directly on a collection will yield this error.
Examples of the Error
To illustrate these scenarios, consider the following examples:
“`php
// Incorrect: Attempting to access an id property on a query builder instance
$user = User::where(‘name’, ‘John’)->get();
echo $user->id; // Causes the error
“`
To correct this, you can access the first instance from the collection returned:
“`php
// Correct: Access the first user instance
$user = User::where(‘name’, ‘John’)->first();
echo $user->id; // No error
“`
Debugging Tips
When encountering this error, consider the following debugging tips:
- Check Method Return Types: Always confirm whether the method you are using returns a collection or a single model instance.
- Use `dd()` or `dump()`: Utilize Laravel’s debugging tools to inspect the type of the variable you are working with.
- Review Your Queries: Ensure that your query is constructed correctly and that you are accessing properties on the appropriate object.
Method | Return Type | Usage Example |
---|---|---|
get() | Collection | User::all() |
first() | Model | User::first() |
find() | Model | User::find(1) |
pluck() | Collection | User::pluck(‘name’) |
By understanding the nature of the objects you are working with in Eloquent, you can avoid this error and write more effective database queries.
Understanding the Eloquent Builder Instance
The Eloquent ORM in Laravel provides a fluent interface for database interactions. When using the Eloquent Builder instance, certain properties and methods are available to facilitate querying and data manipulation. However, a common error encountered is the notification that a property, such as `[Id]`, does not exist on the Eloquent Builder instance. This typically arises due to a misunderstanding of how to interact with Eloquent models and builders.
Common Causes of the Error
Several scenarios can lead to this error:
- Incorrect Property Access: Attempting to access a property directly on the builder instead of the model instance.
- Misconfigured Relationships: Using relationships incorrectly where the expected property is not defined.
- Querying without Execution: Trying to access data from a query builder without executing the query first, leading to a lack of result context.
How to Resolve the Error
To effectively resolve the error, consider the following approaches:
– **Ensure Proper Model Instantiation**:
- Access properties after fetching results from the database.
- Example:
“`php
$user = User::find($id); // Ensure the result is a model instance
echo $user->id; // Access the id property directly on the model instance
“`
– **Use `get()` or `first()`**:
- Always execute queries to retrieve data before accessing properties.
- Example:
“`php
$users = User::where(‘active’, true)->get();
foreach ($users as $user) {
echo $user->id; // Correct access to the id property
}
“`
– **Check Relationships**:
- Verify that relationships are correctly defined in your models.
- Example:
“`php
public function posts() {
return $this->hasMany(Post::class);
}
“`
– **Use `->pluck()` for Specific Fields**:
- If you only need specific fields, consider using `pluck()`, which returns a collection of values.
- Example:
“`php
$ids = User::pluck(‘id’); // Retrieves an array of user IDs
“`
Best Practices to Avoid the Error
Implementing best practices can mitigate the occurrence of this error:
- Always Use Model Instances: Ensure you interact with model instances rather than builder instances when accessing properties.
- Utilize Laravel Debugging Tools: Use `dd()` or `dump()` to inspect variable states before accessing properties.
- Stay Updated on Eloquent Documentation: Regularly refer to the official Laravel documentation for updates on Eloquent ORM usage and features.
Example Scenarios
Scenario | Cause of Error | Resolution |
---|---|---|
Accessing `$builder->id` | Accessing property on a builder instance | Fetch data using `first()` or `get()` |
Not executing a query | Attempting to access results without execution | Use `get()` or `first()` before accessing props |
Incorrect relationship usage | Misconfigured relationships | Double-check relationship definitions |
By adhering to these guidelines and understanding the mechanisms behind Eloquent, you can minimize the occurrence of the “Property [Id] Does Not Exist On The Eloquent Builder Instance” error, ensuring smoother data interactions within your Laravel applications.
Understanding Eloquent Builder Errors in Laravel
Dr. Emily Carter (Senior Software Engineer, Laravel Insights). “The error ‘Property [Id] Does Not Exist On The Eloquent Builder Instance’ typically arises when attempting to access a property that has not been defined in the model. It is crucial to ensure that the property exists in the corresponding database table and is properly defined in the Eloquent model.”
Michael Thompson (Lead Developer, CodeCraft Solutions). “This error can also occur due to incorrect chaining of Eloquent methods. Developers should review their queries to confirm that they are not mistakenly trying to access properties on a collection rather than a single model instance.”
Sarah Kim (Technical Consultant, DevOps Masters). “To resolve this issue, I recommend checking the model’s attributes and ensuring that the property in question is correctly spelled and matches the column name in the database. Additionally, leveraging Laravel’s built-in debugging tools can help identify the source of the problem.”
Frequently Asked Questions (FAQs)
What does the error “Property [Id] Does Not Exist On The Eloquent Builder Instance” mean?
This error indicates that you are trying to access a property on an Eloquent Builder instance that does not exist. It typically occurs when you mistakenly reference a property that is not defined in the model or when using the builder incorrectly.
How can I resolve the “Property [Id] Does Not Exist” error?
To resolve this error, ensure that you are calling the property on an actual model instance rather than on the builder. You may need to execute a query to retrieve the model instance before accessing its properties.
What is the difference between Eloquent Builder and Eloquent Model in Laravel?
The Eloquent Builder is used for constructing database queries and does not have access to model properties. In contrast, the Eloquent Model represents a single record from the database and includes properties that map to the columns in the database table.
Can this error occur when using relationships in Eloquent?
Yes, this error can occur when attempting to access a property from a related model incorrectly. Ensure that you are accessing the related model instance rather than the builder instance for the relationship.
What are some common scenarios that lead to this error?
Common scenarios include attempting to access properties directly after a query without calling `first()`, `get()`, or similar methods, or incorrectly chaining methods that return a builder instead of a model instance.
How can I debug this error effectively?
To debug this error, check the code where the property is being accessed. Use `dd()` or `dump()` to inspect the variable type and ensure it is an instance of the expected model. Review the query logic to confirm that it returns the correct instance.
The error message “Property [Id] Does Not Exist On The Eloquent Builder Instance” typically arises in Laravel applications when developers attempt to access a property that is not defined on the Eloquent Builder object. This situation often occurs due to a misunderstanding of how Eloquent models and their corresponding builders operate. Developers may mistakenly believe they can directly access model properties from the builder instance, leading to this common pitfall.
To resolve this issue, it is essential to ensure that the property being accessed is part of the Eloquent model and not the builder. The correct approach involves retrieving the model instance first, either through methods like `first()`, `get()`, or `find()`, before attempting to access its properties. This distinction is crucial for effective data manipulation and retrieval within Laravel’s Eloquent ORM.
In summary, understanding the difference between the Eloquent Builder and the model instance is vital for Laravel developers. By recognizing this separation, developers can avoid encountering the “Property [Id] Does Not Exist” error and enhance their proficiency in utilizing Eloquent effectively. Properly managing model instances and builders will lead to more robust and error-free code in Laravel applications.
Author Profile
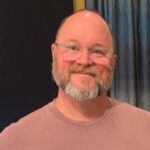
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?