How Can You Use PowerShell to Delete a File If It Exists?
In the world of system administration and automation, PowerShell stands out as a powerful tool that empowers users to streamline their workflows and manage files with precision. One common task that often arises is the need to delete files, particularly when dealing with temporary files, logs, or outdated data. However, before executing a delete command, it’s crucial to ensure that the file in question exists to avoid unnecessary errors. In this article, we’ll explore how to effectively use PowerShell to delete files only if they exist, ensuring your scripts run smoothly and efficiently.
Understanding how to manipulate files in PowerShell is essential for anyone looking to enhance their scripting skills. The ability to check for a file’s existence before attempting to delete it not only prevents errors but also adds a layer of safety to your scripts. This practice is particularly beneficial in environments where file management is critical, such as server maintenance, data processing, or automated backups. By leveraging PowerShell’s built-in cmdlets, users can create robust scripts that handle file deletions gracefully.
As we delve deeper into this topic, we’ll cover the fundamental commands and techniques that allow you to check for file existence and perform deletions seamlessly. Whether you’re a seasoned PowerShell user or just starting out, mastering these skills will enhance your ability to manage files
Using PowerShell to Delete a File If It Exists
PowerShell provides a straightforward way to delete files conditionally, ensuring that you do not encounter errors when a file is absent. The `Test-Path` cmdlet is an essential tool in this process, allowing you to verify the existence of a file before attempting to delete it. This approach helps maintain the flow of your script without interruption from exception errors.
To delete a file if it exists, you can utilize the following syntax:
“`powershell
$filePath = “C:\path\to\your\file.txt”
if (Test-Path $filePath) {
Remove-Item $filePath -Force
Write-Host “File deleted successfully.”
} else {
Write-Host “File does not exist.”
}
“`
In this example:
- `$filePath` is the variable holding the file path you want to check.
- `Test-Path` checks if the file exists.
- `Remove-Item` is used to delete the file, with the `-Force` parameter ensuring that the deletion occurs without prompting for confirmation.
- `Write-Host` provides feedback in the console.
Alternative Method Using Try-Catch
Another effective way to manage file deletion is through the `Try-Catch` mechanism. This method can be beneficial when you want to handle exceptions that may arise during the deletion process, such as permissions issues.
Here’s an example of this approach:
“`powershell
$filePath = “C:\path\to\your\file.txt”
try {
Remove-Item $filePath -ErrorAction Stop
Write-Host “File deleted successfully.”
} catch {
Write-Host “An error occurred: $_”
}
“`
In this script:
- `Remove-Item` is executed within a `try` block.
- The `-ErrorAction Stop` parameter ensures that any error triggers the `catch` block.
- The `catch` block captures and displays the error message.
Summary of Cmdlets and Parameters
To facilitate understanding, the following table summarizes the cmdlets and parameters used for deleting files in PowerShell.
Cmdlet | Description |
---|---|
Test-Path | Checks if the specified path exists. |
Remove-Item | Deletes the specified item (file or folder). |
-Force | Suppresses confirmation prompts and forces deletion. |
-ErrorAction | Specifies how PowerShell responds to non-terminating errors. |
Utilizing these cmdlets effectively allows for efficient file management within PowerShell, enhancing your automation scripts by preventing unnecessary errors and providing clear feedback.
Powershell Command to Delete a File if It Exists
To delete a file using PowerShell only if it exists, you can utilize the `Test-Path` cmdlet along with `Remove-Item`. This method ensures that you avoid errors that occur when attempting to delete a file that is not present.
“`powershell
$filePath = “C:\path\to\your\file.txt”
if (Test-Path $filePath) {
Remove-Item $filePath -Force
Write-Output “File deleted successfully.”
} else {
Write-Output “File does not exist.”
}
“`
In this script:
- $filePath: This variable holds the path to the file you wish to delete.
- Test-Path: This cmdlet checks if the specified file exists.
- Remove-Item: This command deletes the file, with the `-Force` parameter ensuring that it removes read-only files without prompting.
- Write-Output: This provides feedback on the operation’s outcome.
Using a Function for Reusability
For enhanced usability, you can encapsulate the deletion logic within a function. This allows for easy reuse throughout your scripts.
“`powershell
function Delete-FileIfExists {
param (
[string]$filePath
)
if (Test-Path $filePath) {
Remove-Item $filePath -Force
Write-Output “File deleted successfully: $filePath”
} else {
Write-Output “File does not exist: $filePath”
}
}
Example usage
Delete-FileIfExists “C:\path\to\your\file.txt”
“`
This function takes a file path as an argument and performs the same checks and deletion process.
Handling Multiple Files
To delete multiple files based on a pattern, you can use wildcard characters with `Get-ChildItem` in conjunction with the `Remove-Item` command.
“`powershell
$directoryPath = “C:\path\to\your\directory”
$filePattern = “*.txt”
Get-ChildItem -Path $directoryPath -Filter $filePattern | ForEach-Object {
Remove-Item $_.FullName -Force
Write-Output “Deleted file: $_.FullName”
}
“`
In this example:
- Get-ChildItem: This cmdlet retrieves files matching the specified pattern.
- ForEach-Object: This processes each file object returned by `Get-ChildItem`, allowing for deletion.
Error Handling
Incorporating error handling into your script can provide more robust operation and feedback during execution.
“`powershell
try {
if (Test-Path $filePath) {
Remove-Item $filePath -Force -ErrorAction Stop
Write-Output “File deleted successfully: $filePath”
} else {
Write-Output “File does not exist: $filePath”
}
} catch {
Write-Output “An error occurred: $_.Exception.Message”
}
“`
Using `try` and `catch` blocks helps manage any exceptions that may arise, such as permissions issues or file locks.
Scheduled Deletion with Task Scheduler
If you require periodic deletion of files, consider utilizing Windows Task Scheduler to run your PowerShell script at specified intervals.
- Create a `.ps1` script containing your deletion logic.
- Open Task Scheduler and select Create Basic Task.
- Follow the prompts to set the task name, trigger, and action:
- Action: Start a program.
- Program/script: Enter `powershell.exe`.
- Add arguments: Use `-File “C:\path\to\your\script.ps1″`.
This approach allows for automated file management, ensuring files are deleted as needed without manual intervention.
Expert Insights on Using PowerShell to Delete Files if They Exist
Dr. Emily Carter (Senior Systems Administrator, Tech Solutions Inc.). “Utilizing PowerShell to delete files conditionally is a powerful technique that can streamline processes. By using the ‘Test-Path’ cmdlet, administrators can check for file existence before executing the ‘Remove-Item’ command, ensuring that scripts run efficiently without unnecessary errors.”
Michael Chen (DevOps Engineer, Cloud Innovations). “Incorporating error handling when deleting files with PowerShell is essential. I recommend wrapping the delete command in a try-catch block to manage exceptions gracefully. This approach not only improves script reliability but also provides clear feedback on the operation’s success or failure.”
Sarah Thompson (IT Security Consultant, SecureTech Advisors). “When automating file deletion with PowerShell, it is crucial to consider security implications. Ensure that the script runs with the least privilege necessary and implement logging to track deletions. This practice helps maintain accountability and can prevent accidental data loss.”
Frequently Asked Questions (FAQs)
How can I delete a file in PowerShell if it exists?
You can use the `Remove-Item` cmdlet along with a conditional check. For example:
“`powershell
if (Test-Path “C:\path\to\your\file.txt”) { Remove-Item “C:\path\to\your\file.txt” }
“`
What does the `Test-Path` cmdlet do in PowerShell?
The `Test-Path` cmdlet checks for the existence of a file or directory. It returns `True` if the specified path exists and “ if it does not.
Can I delete multiple files at once using PowerShell?
Yes, you can delete multiple files by using wildcards or an array of file paths. For example:
“`powershell
Get-ChildItem “C:\path\to\your\*.txt” | Remove-Item
“`
What happens if I try to delete a file that does not exist?
If you attempt to delete a file that does not exist, PowerShell will return an error message indicating that the file could not be found, unless you use the `-ErrorAction SilentlyContinue` parameter to suppress the error.
Is there a way to confirm the deletion of a file in PowerShell?
Yes, you can prompt for confirmation by using the `-Confirm` parameter with the `Remove-Item` cmdlet. For example:
“`powershell
Remove-Item “C:\path\to\your\file.txt” -Confirm
“`
Can I use PowerShell to delete files based on specific criteria?
Yes, you can filter files based on criteria such as file size, date modified, or file type using `Where-Object`. For example:
“`powershell
Get-ChildItem “C:\path\to\your\*” | Where-Object { $_.Length -gt 1MB } | Remove-Item
“`
In summary, utilizing PowerShell to delete a file if it exists is a straightforward yet powerful capability for system administrators and users alike. The primary command used for this purpose is the `Remove-Item` cmdlet, which can be combined with conditional statements to ensure that the deletion occurs only when the specified file is present. This approach not only enhances efficiency but also minimizes the risk of errors that could arise from attempting to delete non-existent files.
Moreover, incorporating checks such as `Test-Path` allows users to verify the existence of a file before attempting deletion. This practice is crucial in scripting and automation, as it adds an additional layer of safety and helps prevent unnecessary error messages. By leveraging these commands, users can create robust scripts that manage file systems effectively.
Key takeaways from this discussion include the importance of understanding the syntax and options available in PowerShell for file management tasks. Familiarity with these commands can significantly streamline workflows and improve productivity. Additionally, users should always consider implementing error handling and logging to track actions taken by scripts, further enhancing their reliability and maintainability.
Author Profile
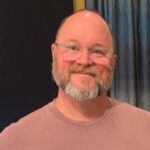
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?