How Can You Use Pandas to Remove Rows Based on Specific Conditions?
In the realm of data analysis, the ability to manipulate and refine datasets is paramount for extracting meaningful insights. One of the most powerful tools in a data analyst’s arsenal is the Pandas library in Python, renowned for its versatility and efficiency in handling structured data. Among the myriad of operations that Pandas facilitates, the ability to remove rows based on specific conditions stands out as a crucial skill. Whether you’re cleaning up a messy dataset or filtering out irrelevant information, mastering this technique can significantly enhance the quality of your analyses and the accuracy of your results.
Removing rows with certain conditions is not just about tidying up your data; it’s about honing in on the information that truly matters. With Pandas, you can easily identify and eliminate entries that do not meet your specified criteria, allowing you to focus on the data that drives your conclusions. This process is essential in various scenarios, such as excluding outliers, filtering based on categorical variables, or removing incomplete records that could skew your findings.
As we delve deeper into this topic, we will explore the various methods and functions that Pandas offers for row removal, providing you with practical examples and best practices. By the end of this article, you will be equipped with the knowledge to effectively cleanse your datasets, ensuring that your analyses are both robust
Using Boolean Indexing to Remove Rows
Boolean indexing is a powerful feature in Pandas that allows you to filter data based on specific conditions. To remove rows from a DataFrame, you can create a boolean mask that represents the rows you want to keep and then apply this mask to the DataFrame.
For example, if you have a DataFrame named `df` and you want to remove rows where the value in the column `A` is less than 5, you can do the following:
“`python
df = df[df[‘A’] >= 5]
“`
This code creates a boolean mask where each element is `True` if the condition is met (i.e., the value in column `A` is 5 or greater) and “ otherwise. The DataFrame is then filtered to only include rows where the mask is `True`.
Using the `drop` Method
The `drop` method in Pandas is another approach to remove rows based on certain conditions. This method is particularly useful when you know the index labels of the rows you wish to remove.
Here’s an example to illustrate this:
“`python
df = df.drop(df[df[‘A’] < 5].index)
```
In this example, the `drop` method is used in conjunction with a boolean condition to identify the indices of the rows that need to be removed. The result is a DataFrame that excludes those rows.
Conditional Removal Based on Multiple Columns
Sometimes, you may need to remove rows based on conditions across multiple columns. In such cases, you can combine conditions using logical operators.
For instance, if you want to remove rows where column `A` is less than 5 and column `B` is equal to ‘X’, you can use:
“`python
df = df[(df[‘A’] >= 5) & (df[‘B’] != ‘X’)]
“`
This will keep rows that do not meet either of the conditions specified.
Example DataFrame
To demonstrate how to remove rows with conditions, consider the following example DataFrame:
“`python
import pandas as pd
data = {
‘A’: [1, 5, 7, 3, 6],
‘B’: [‘X’, ‘Y’, ‘X’, ‘Z’, ‘Y’]
}
df = pd.DataFrame(data)
“`
The DataFrame `df` appears as follows:
A | B |
---|---|
1 | X |
5 | Y |
7 | X |
3 | Z |
6 | Y |
To remove rows where column `A` is less than 5, you can apply:
“`python
df = df[df[‘A’] >= 5]
“`
After executing this command, the updated DataFrame will be:
A | B |
---|---|
5 | Y |
7 | X |
6 | Y |
This method efficiently filters the DataFrame to retain only the desired rows based on the specified conditions.
Pandas Remove Rows With Condition
In Pandas, removing rows based on specific conditions is a common task that can be accomplished using various methods. The most straightforward way to filter data is through boolean indexing, which allows you to create a mask of the rows you want to keep or remove.
Using Boolean Indexing
Boolean indexing involves creating a boolean Series that indicates whether each row meets the specified condition. You can then use this Series to filter the DataFrame.
“`python
import pandas as pd
Sample DataFrame
data = {‘A’: [1, 2, 3, 4, 5],
‘B’: [10, 20, 30, 40, 50]}
df = pd.DataFrame(data)
Remove rows where column A is greater than 3
df_filtered = df[df[‘A’] <= 3]
```
In this example, rows where column 'A' is greater than 3 are removed, resulting in a DataFrame that only includes rows with values 1, 2, and 3 in column 'A'.
Using the `drop` Method
The `drop` method is another effective way to remove rows, especially when you know the indices of the rows you want to eliminate.
“`python
Remove rows with indices 3 and 4
df_dropped = df.drop(index=[3, 4])
“`
This method directly removes the specified rows based on their index labels.
Conditional Filtering with `query` Method
The `query` method offers a more readable syntax for filtering rows based on conditions. It allows you to express the conditions in a string format.
“`python
Remove rows where column B is less than 30
df_filtered_query = df.query(‘B >= 30’)
“`
This method returns a DataFrame that includes only the rows where column ‘B’ has values of 30 or greater.
Using `loc` for Conditional Removal
The `loc` indexer can also be used to filter DataFrames conditionally. This method is particularly useful when you want to update a DataFrame in place.
“`python
Keeping rows where column A is less than or equal to 3
df.loc[df[‘A’] <= 3, :]
```
This command modifies the DataFrame to include only the desired rows.
Removing Rows with `isin` Method
To remove rows based on membership in a list, the `isin` method can be utilized. This is useful when you want to exclude multiple specific values.
“`python
Remove rows where column A is in [1, 2]
df_filtered_isin = df[~df[‘A’].isin([1, 2])]
“`
The tilde `~` operator negates the condition, thereby excluding the specified values from the DataFrame.
Example Table of Methods
Method | Description | Example Code |
---|---|---|
Boolean Indexing | Filter rows based on a condition | `df[df[‘A’] <= 3]` |
`drop` | Remove rows by index | `df.drop(index=[3, 4])` |
`query` | Filter using a query string | `df.query(‘B >= 30’)` |
`loc` | Conditional filtering and updating | `df.loc[df[‘A’] <= 3, :]` |
`isin` | Remove rows based on membership in a list | `df[~df[‘A’].isin([1, 2])]` |
These methods allow for flexible and efficient manipulation of DataFrames in Pandas, enabling users to maintain clean and relevant datasets for analysis.
Expert Insights on Removing Rows with Conditions in Pandas
Dr. Emily Chen (Data Scientist, Analytics Innovations). “When working with large datasets in Pandas, efficiently removing rows based on specific conditions is crucial for data integrity. Utilizing the `DataFrame.drop()` method in combination with boolean indexing allows for precise control over which data points to retain or discard.”
Michael Thompson (Senior Python Developer, CodeCraft Solutions). “In my experience, leveraging the `DataFrame.loc[]` method not only simplifies the process of filtering out unwanted rows but also enhances code readability. This approach is particularly beneficial when dealing with complex conditions that require multiple criteria.”
Sarah Patel (Machine Learning Engineer, DataWise Technologies). “Removing rows with conditions in Pandas is not just about cleaning data; it is about preparing it for analysis. I recommend using the `query()` method for more complex filtering scenarios, as it provides a more intuitive syntax and can significantly improve performance on larger datasets.”
Frequently Asked Questions (FAQs)
What is the method to remove rows with a specific condition in a Pandas DataFrame?
You can use the `DataFrame.drop()` method in combination with boolean indexing to remove rows that meet a specific condition. For example, `df = df[df[‘column_name’] != value]` will keep only the rows where ‘column_name’ is not equal to ‘value’.
How can I remove rows based on multiple conditions in Pandas?
To remove rows based on multiple conditions, you can combine boolean conditions using the bitwise operators `&` (and) or `|` (or). For instance, `df = df[(df[‘col1’] != value1) & (df[‘col2’] != value2)]` will filter out rows where both conditions are met.
Is there a way to remove rows in-place without creating a new DataFrame?
Yes, you can use the `inplace` parameter with the `DataFrame.drop()` method. For example, `df.drop(df[df[‘column_name’] == value].index, inplace=True)` will remove the rows that meet the condition directly in the original DataFrame.
Can I remove rows based on NaN values in a specific column?
Yes, you can remove rows with NaN values in a specific column using the `DataFrame.dropna()` method. For example, `df.dropna(subset=[‘column_name’], inplace=True)` will remove all rows where ‘column_name’ contains NaN values.
What happens to the DataFrame index after removing rows?
When rows are removed, the original index is preserved by default. If you want to reset the index after removing rows, you can use the `DataFrame.reset_index(drop=True)` method to reindex the DataFrame without adding the old index as a column.
Are there any performance considerations when removing rows from large DataFrames?
Yes, removing rows from large DataFrames can be resource-intensive and may lead to performance issues. It is advisable to filter the DataFrame first and then create a new one, or use methods that operate in-place to minimize memory usage.
In the realm of data manipulation using the Pandas library in Python, removing rows based on specific conditions is a fundamental operation. This task is typically accomplished using boolean indexing, the `.drop()` method, or the `.loc[]` accessor. Each of these methods allows users to filter out unwanted data efficiently, ensuring that the resulting DataFrame aligns with the desired analysis criteria. Understanding how to apply these techniques is crucial for maintaining data integrity and optimizing data processing workflows.
One of the most common approaches to removing rows is through boolean indexing, where a condition is applied to create a boolean mask. This mask can then be used to filter the DataFrame, effectively retaining only the rows that meet the specified criteria. Alternatively, the `.drop()` method can be employed to remove rows by their index labels, while the `.loc[]` accessor provides a more flexible way to select and manipulate data based on conditions. Each method has its advantages, and the choice often depends on the specific requirements of the analysis at hand.
Key takeaways from the discussion on removing rows with conditions in Pandas include the importance of understanding the underlying data structure and the implications of removing data. Careful consideration should be given to the conditions used to filter data, as improper
Author Profile
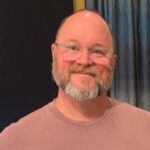
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?