How Can You Develop a Website Using Python?
In today’s digital age, having a robust online presence is essential for individuals and businesses alike. As the demand for dynamic and interactive websites continues to rise, developers are increasingly turning to Python—a versatile and powerful programming language. Whether you’re a seasoned programmer or a curious beginner, learning how to develop a website using Python opens up a world of possibilities. From creating simple static pages to building complex web applications, Python offers a plethora of frameworks and tools that streamline the development process and enhance functionality.
In this article, we will explore the fundamental concepts of web development with Python, highlighting its advantages and the various frameworks that simplify the journey from idea to execution. Python’s readability and efficiency make it an ideal choice for web development, allowing developers to focus on creativity and problem-solving rather than getting bogged down by syntax. As we delve into the essentials of building a website, you will discover how Python can empower you to create engaging user experiences while maintaining clean and maintainable code.
Join us as we navigate the exciting landscape of web development with Python, where we will uncover the steps, best practices, and resources you need to bring your vision to life. Whether you aim to launch a personal blog, an e-commerce platform, or a complex web application, the journey begins here
Choosing the Right Framework
The choice of framework can significantly impact the development process of a Python-based website. A few popular frameworks include Django, Flask, and FastAPI. Each has its strengths and weaknesses, making them suitable for different types of projects.
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It comes with built-in admin functionality, making it ideal for projects with complex data models.
- Flask: A micro-framework that provides the essentials to get an application up and running. It’s lightweight, making it suitable for smaller applications or those that require flexibility.
- FastAPI: Known for its speed and efficiency, FastAPI is perfect for building APIs and applications that require high performance.
When selecting a framework, consider the following factors:
- Project size and complexity
- Development speed versus flexibility
- Community support and documentation
Setting Up Your Development Environment
A well-configured development environment is crucial for any web project. Here’s how to set up your environment:
- Install Python: Ensure you have Python installed on your system. You can download it from the official Python website.
- Create a Virtual Environment: This isolates your project’s dependencies and prevents conflicts with other projects.
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use: myenv\Scripts\activate
“`
- Install Required Packages: Use pip to install the necessary packages based on your selected framework.
“`bash
pip install django For Django
pip install flask For Flask
pip install fastapi For FastAPI
“`
Designing the Database
Database design is a crucial aspect of web development. Depending on your framework, you may have different options for database integration.
Framework | Database Options |
---|---|
Django | SQLite, PostgreSQL, MySQL |
Flask | SQLite, PostgreSQL |
FastAPI | PostgreSQL, MongoDB |
Utilize Object-Relational Mapping (ORM) tools such as Django ORM or SQLAlchemy for Flask and FastAPI to interact with your database more efficiently. This abstraction layer allows you to work with database records as Python objects.
Building the Application Structure
An organized application structure enhances maintainability and scalability. Here’s a typical structure for a Django project:
“`
myproject/
│
├── myapp/
│ ├── migrations/
│ ├── models.py
│ ├── views.py
│ └── urls.py
│
├── myproject/
│ ├── settings.py
│ ├── urls.py
│ └── wsgi.py
│
└── manage.py
“`
For Flask, the structure might look like this:
“`
myflaskapp/
│
├── app/
│ ├── __init__.py
│ ├── routes.py
│ └── models.py
│
└── run.py
“`
Ensure your project is modular, with separate files or directories for views, models, and routes to promote separation of concerns.
Creating Routes and Views
Routing is essential for defining how users interact with your website. Each route corresponds to a specific function in your application.
In Django, you would define a route in `urls.py`:
“`python
from django.urls import path
from . import views
urlpatterns = [
path(”, views.home, name=’home’),
]
“`
For Flask, routing is similarly straightforward:
“`python
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def home():
return “Hello, World!”
“`
Each view function should handle requests and return responses, often rendering HTML templates or returning JSON data.
Implementing Templates
Templates allow you to create dynamic web pages. Use a templating engine such as Jinja2 (default in Flask) or Django’s templating engine.
Here’s a simple example of a template in Django:
“`html
{{ heading }}
“`
In Flask, rendering a template can be done as follows:
“`python
from flask import render_template
@app.route(‘/’)
def home():
return render_template(‘index.html’, title=’Home’, heading=’Welcome!’)
“`
Utilizing templates enhances user experience by allowing dynamic content generation based on the underlying data.
Choosing a Framework
Selecting the right framework is crucial for developing a website using Python. Popular frameworks streamline the development process by providing built-in functionalities. Here are some of the most commonly used frameworks:
- Django: A high-level framework that encourages rapid development and clean, pragmatic design. It includes an ORM, authentication, and an admin panel out of the box.
- Flask: A micro-framework that is lightweight and easy to use. It allows developers to choose their components, making it highly customizable.
- FastAPI: Designed for building APIs, FastAPI is based on standard Python type hints, making it fast and efficient for modern web applications.
- Pyramid: A flexible framework that can be used for both simple and complex applications. It allows developers to start small and scale up as needed.
Framework | Pros | Cons |
---|---|---|
Django | Fully featured, secure, scalable | Can be heavy for small apps |
Flask | Lightweight, flexible, easy to learn | Requires more setup |
FastAPI | Fast, modern, supports async | Less mature ecosystem |
Pyramid | Versatile, great for large apps | Steeper learning curve |
Setting Up Your Development Environment
To effectively develop a website with Python, you need to set up your development environment properly. This includes installing necessary software and libraries.
- Python Installation: Ensure you have Python installed on your machine. Use the latest version for better support.
- Virtual Environment: Create a virtual environment to manage dependencies. This can be done using:
“`bash
python -m venv myenv
“`
Activate the environment:
- On Windows: `myenv\Scripts\activate`
- On macOS/Linux: `source myenv/bin/activate`
- Install Framework: Use pip to install your chosen framework. For example:
“`bash
pip install django
“`
Building the Website Structure
Once the environment is ready, the next step is to build the website structure. This typically involves creating the project and app directories.
- Django:
“`bash
django-admin startproject myproject
cd myproject
python manage.py startapp myapp
“`
- Flask:
Create a file called `app.py` and start defining your application.
- FastAPI:
Create a file called `main.py` and set up the FastAPI instance.
Creating Views and Templates
Views handle the logic of your website, while templates define how the data is presented to the user.
- Django: Define views in `views.py` and create HTML templates in a `templates` directory.
- Flask: Similar to Django, create routes in `app.py` and templates in a `templates` folder.
- FastAPI: Use Jinja2 or similar libraries for rendering templates.
Example of a simple view in Django:
“`python
from django.shortcuts import render
def home(request):
return render(request, ‘home.html’)
“`
Database Integration
Integrating a database is essential for dynamic websites. Most frameworks support various databases.
- Django: Uses Django ORM to interact with databases like PostgreSQL, SQLite, or MySQL.
- Flask: Can use SQLAlchemy for ORM or work directly with SQL.
- FastAPI: Supports SQLAlchemy or Tortoise-ORM for database interactions.
Configure your database settings in your framework’s configuration file, and run migrations to set up the necessary tables.
Testing and Debugging
Testing is a critical part of the development process. Each framework has tools for testing.
- Django: Use `python manage.py test` to run tests.
- Flask: Use `pytest` for testing Flask applications.
- FastAPI: Leverage `pytest` and `httpx` for testing API endpoints.
Debugging tools such as logging and breakpoints are also available in each framework to help diagnose issues efficiently.
Expert Insights on Developing a Website Using Python
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “When developing a website using Python, it’s crucial to choose the right web framework. Django and Flask are two popular options, each with its strengths. Django is great for larger applications requiring a robust structure, while Flask is ideal for smaller, more flexible projects.”
Michael Thompson (Lead Web Developer, CodeCraft Solutions). “Understanding the fundamentals of HTML, CSS, and JavaScript is essential when using Python for web development. These technologies work in tandem with Python frameworks to create a seamless user experience and dynamic web applications.”
Sarah Patel (Digital Solutions Architect, WebTech Experts). “Security should be a top priority when developing a website with Python. Implementing best practices such as input validation, secure session management, and regular security audits can help protect your application from vulnerabilities.”
Frequently Asked Questions (FAQs)
What frameworks can I use to develop a website using Python?
You can use several frameworks, including Django, Flask, Pyramid, and FastAPI. Django is suitable for larger applications with built-in features, while Flask is ideal for smaller projects due to its simplicity and flexibility.
Do I need to know HTML, CSS, and JavaScript to develop a website with Python?
Yes, a fundamental understanding of HTML, CSS, and JavaScript is essential. These technologies are necessary for creating the frontend of your website, while Python typically handles the backend logic.
Can I deploy a Python web application on cloud platforms?
Absolutely. You can deploy Python web applications on various cloud platforms such as Heroku, AWS, Google Cloud, and Microsoft Azure. Each platform provides specific services and tools to facilitate deployment.
Is it possible to integrate a database with a Python web application?
Yes, Python web applications can easily integrate with databases. Popular choices include PostgreSQL, MySQL, and SQLite. You can use Object-Relational Mapping (ORM) tools like SQLAlchemy or Django’s ORM for seamless integration.
What are the best practices for developing a secure website using Python?
Best practices include using HTTPS, validating user input, implementing proper authentication and authorization, keeping dependencies updated, and regularly testing for vulnerabilities. Utilizing security libraries like Flask-Security or Django’s built-in security features is also recommended.
How can I learn more about developing websites with Python?
You can enhance your skills through online courses, tutorials, and documentation specific to the frameworks you choose. Websites like Codecademy, Coursera, and the official documentation for Django and Flask provide valuable resources for learning.
developing a website using Python involves several key steps that leverage the language’s versatility and robust frameworks. Python’s simplicity and readability make it an excellent choice for both beginners and experienced developers. By utilizing frameworks such as Django or Flask, developers can streamline the process of building web applications, as these frameworks provide essential tools and libraries that facilitate rapid development and deployment.
Moreover, understanding the basics of web development, including HTML, CSS, and JavaScript, is crucial for creating a fully functional website. These technologies work in tandem with Python to enhance the user interface and experience. Additionally, incorporating databases, such as PostgreSQL or SQLite, allows for efficient data management and storage, which is vital for dynamic web applications.
Key takeaways from this discussion emphasize the importance of selecting the right framework based on project requirements. Django is ideal for larger applications that require a comprehensive solution, while Flask is better suited for smaller projects or microservices. Furthermore, leveraging Python’s extensive libraries and community support can significantly accelerate the development process, ensuring that developers can focus on building innovative features rather than dealing with repetitive tasks.
Author Profile
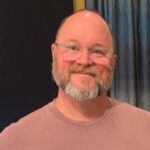
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?