Why Are Control Structures Essential in Python Programming?
In the world of programming, control structures are the backbone of logic and decision-making, guiding the flow of execution in a program. Python, known for its simplicity and readability, offers a variety of control structures that empower developers to create dynamic and responsive applications. Whether you’re building a simple script or a complex software system, understanding how to effectively utilize these structures is essential for crafting efficient and maintainable code. In this article, we will explore the fundamental role of control structures in Python, unlocking the potential to make your programs not only functional but also intelligent.
Control structures in Python serve as the essential tools that dictate how a program responds to different conditions and inputs. They enable developers to implement decision-making processes, iterate over data, and manage the flow of execution based on specific criteria. From the ubiquitous if-else statements that allow for branching logic to loops that facilitate repetitive tasks, these structures form the core of algorithmic thinking in programming. By mastering control structures, programmers can write code that is not only more powerful but also easier to understand and maintain.
As we delve deeper into the various types of control structures available in Python, you’ll discover how they can be leveraged to solve complex problems, enhance code readability, and improve overall program efficiency. Whether you’re a novice looking to grasp
Understanding Control Structures
Control structures are fundamental components in Python programming that dictate the flow of execution of code blocks. They enable developers to create dynamic and responsive applications by allowing conditional execution and iteration.
Types of Control Structures
In Python, control structures can be categorized into three primary types:
- Sequential: The default mode of execution where statements are executed line by line.
- Selection: Enables the execution of specific blocks of code based on conditions. Common selection structures include `if`, `elif`, and `else`.
- Repetition: Facilitates the execution of code blocks multiple times. This is achieved through loops such as `for` and `while`.
Selection Control Structures
Selection control structures allow a program to make decisions. The most common forms include:
- if statement: Executes a block of code if the specified condition is true.
- elif statement: A continuation of an `if` statement that checks additional conditions if the previous ones were .
- else statement: Executes a block of code if none of the preceding conditions were true.
Example:
“`python
age = 18
if age < 18:
print("You are a minor.")
elif age == 18:
print("You are an adult.")
else:
print("You are a senior.")
```
Repetition Control Structures
Repetition control structures, or loops, are used to execute a block of code multiple times until a specified condition is met. The primary types are:
- for loop: Iterates over a sequence (like a list, tuple, or string).
- while loop: Continues to execute as long as a specified condition remains true.
Example of a `for` loop:
“`python
for i in range(5):
print(i)
“`
Example of a `while` loop:
“`python
count = 0
while count < 5:
print(count)
count += 1
```
Control Flow with Break and Continue
In loops, the `break` and `continue` statements provide additional control over loop execution.
- break: Terminates the loop and moves to the next statement outside the loop.
- continue: Skips the current iteration and jumps to the next iteration of the loop.
Example:
“`python
for i in range(10):
if i == 5:
break
print(i)
“`
This will output numbers from 0 to 4 and then terminate.
Using Control Structures Effectively
When employing control structures, it is crucial to ensure code readability and maintainability. Below is a table summarizing best practices:
Practice | Description |
---|---|
Indentation | Use consistent indentation to define code blocks clearly. |
Logical Conditions | Keep conditions simple and logical to improve readability. |
Commenting | Include comments to explain complex control flows. |
Avoid Deep Nesting | Minimize the nesting of control structures to enhance clarity. |
By following these practices, developers can leverage control structures to create efficient, understandable, and maintainable Python code.
Purpose of Control Structures in Python
Control structures in Python are essential for directing the flow of execution in a program. They allow developers to dictate how statements are executed based on certain conditions or to repeat actions.
Types of Control Structures
Control structures can be categorized into three main types: selection statements, iteration statements, and jump statements.
Selection Statements
Selection statements enable the program to choose different paths of execution based on the evaluation of boolean expressions. The primary selection statements in Python are:
– **if statement**: Executes a block of code if a condition evaluates to true.
– **if-else statement**: Provides an alternative block of code that executes when the condition is .
– **if-elif-else statement**: Allows for multiple conditions to be checked sequentially.
Example:
“`python
if score >= 90:
print(“Grade: A”)
elif score >= 80:
print(“Grade: B”)
else:
print(“Grade: C”)
“`
Iteration Statements
Iteration statements facilitate the execution of a block of code multiple times. The primary iteration statements in Python are:
- for loop: Iterates over a sequence (such as a list or string).
- while loop: Repeats a block of code as long as a specified condition is true.
Example of a for loop:
“`python
for number in range(5):
print(number)
“`
Example of a while loop:
“`python
count = 0
while count < 5:
print(count)
count += 1
```
Jump Statements
Jump statements alter the flow of control by skipping or jumping to a different part of the code. The main jump statements in Python include:
- break: Terminates the nearest enclosing loop.
- continue: Skips the current iteration and proceeds to the next one.
- return: Exits a function and optionally returns a value.
Example:
“`python
for number in range(10):
if number == 5:
break
print(number)
“`
Importance of Control Structures
Control structures enhance the functionality and flexibility of Python programs. They allow for:
- Decision Making: Execute code based on specific conditions.
- Repetitive Tasks: Automate repetitive actions without redundancy.
- Structured Programming: Promote clear and maintainable code through organized flow.
Common Use Cases
Control structures find applications in various programming scenarios:
Use Case | Control Structure Used |
---|---|
Validating user input | if statement |
Iterating over data | for loop |
Running conditional tasks | if-elif-else statement |
Implementing timers | while loop |
Exiting loops | break statement |
By using control structures effectively, developers can create efficient, readable, and maintainable code in Python.
The Importance of Control Structures in Python Programming
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Control structures are fundamental in Python as they dictate the flow of execution in a program. They enable developers to implement decision-making processes, allowing for dynamic responses based on varying conditions. This capability is essential for creating efficient algorithms and enhancing code readability.
Michael Chen (Lead Python Developer, CodeCraft Solutions). In Python, control structures such as loops and conditionals are vital for managing repetitive tasks and branching logic. They provide a framework for developers to write concise and maintainable code, which is crucial in collaborative environments where clarity and efficiency are paramount.
Sarah Thompson (Educational Technology Specialist, LearnPython.org). Understanding control structures is a key part of mastering Python programming. These structures not only facilitate logical reasoning in coding but also empower learners to tackle complex problems by breaking them down into manageable parts, ultimately fostering better problem-solving skills.
Frequently Asked Questions (FAQs)
What are control structures in Python?
Control structures in Python are constructs that dictate the flow of execution of the program. They include conditional statements, loops, and control flow statements that allow for decision-making and repetition in code.
What do we use if statements for in Python?
If statements are used to execute a block of code conditionally, based on whether a specified condition evaluates to true. This allows for branching logic in programs.
How do loops function as control structures in Python?
Loops, such as for and while loops, are used to repeat a block of code multiple times. They enable iteration over sequences or repeated execution until a certain condition is met.
What is the purpose of the break and continue statements?
The break statement is used to exit a loop prematurely when a specific condition is met, while the continue statement skips the current iteration and proceeds to the next one, allowing for more control over loop execution.
Can you explain the use of the try and except blocks in control structures?
Try and except blocks are used for exception handling in Python. They allow the programmer to test a block of code for errors and handle those errors gracefully without crashing the program.
How do control structures enhance code readability and maintainability?
Control structures improve code readability by providing clear logic flow and making it easier to understand the decision-making process. They enhance maintainability by allowing changes to be made in a structured manner without altering the entire codebase.
Control structures in Python are fundamental components that allow developers to dictate the flow of execution within a program. They enable the implementation of decision-making processes, iteration, and branching, which are essential for creating dynamic and responsive applications. By utilizing control structures such as conditional statements (if, elif, else) and loops (for, while), programmers can manage how and when specific blocks of code are executed based on varying conditions and inputs.
One of the key takeaways is that control structures enhance the readability and maintainability of code. By clearly defining the paths that the program can take, developers can create more understandable and organized code. This clarity is crucial, especially in larger projects where multiple developers may be involved. Additionally, effective use of control structures can lead to more efficient algorithms, as they allow for the implementation of logic that can minimize unnecessary computations.
Moreover, control structures are not just limited to basic programming tasks; they are integral to complex programming paradigms such as event-driven programming and state machines. Understanding how to utilize these structures effectively can significantly improve a programmer’s ability to solve problems and create robust applications. Ultimately, mastering control structures is essential for anyone looking to excel in Python programming and software development as a whole.
Author Profile
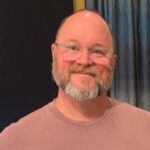
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?