Does Python Have a Switch Statement? Exploring Alternatives and Solutions!
In the world of programming, control flow is a fundamental concept that allows developers to dictate the path their code takes based on varying conditions. Among the many languages available, Python stands out for its simplicity and readability. However, one question often arises among both novice and experienced programmers: Does Python have a switch statement? This seemingly straightforward query opens the door to a deeper exploration of how Python handles conditional logic and whether it offers alternatives to the traditional switch-case structure found in other programming languages.
While many languages, such as C, Java, and JavaScript, incorporate a switch statement to streamline multiple conditional checks, Python takes a different approach. Instead of a dedicated switch construct, Python encourages the use of if-elif-else chains, which provide a clear and expressive way to manage complex decision-making scenarios. This design choice reflects Python’s philosophy of simplicity and explicitness, but it also leads to discussions about the efficiency and readability of alternative methods for handling multiple conditions.
As we delve deeper into this topic, we’ll explore the rationale behind Python’s lack of a switch statement, examine the common patterns used to achieve similar functionality, and consider the implications for code clarity and maintainability. Whether you’re a seasoned Pythonista or just starting your coding journey, understanding these concepts will enhance your ability to write effective
Understanding the Absence of a Switch Statement in Python
Python does not have a built-in switch statement like some other programming languages such as C or Java. This absence can sometimes confuse programmers who are accustomed to using switch statements for cleaner and more organized conditional logic. Instead, Python encourages the use of alternative structures that can achieve similar outcomes.
Alternatives to Switch Statements in Python
While Python lacks a traditional switch statement, there are several methods to replicate its functionality. Here are some common alternatives:
- If-Elif-Else Chains: This is the most straightforward approach. Each condition is checked in sequence until a match is found.
“`python
def switch_example(value):
if value == 1:
return “One”
elif value == 2:
return “Two”
elif value == 3:
return “Three”
else:
return “Invalid”
“`
- Dictionaries: Python’s dictionary structure can be used to simulate switch behavior. You can map keys to functions or values, allowing for more dynamic and concise code.
“`python
def one():
return “One”
def two():
return “Two”
def three():
return “Three”
switch_dict = {
1: one,
2: two,
3: three
}
def switch_using_dict(value):
return switch_dict.get(value, lambda: “Invalid”)()
“`
- Match Statement: Introduced in Python 3.10, the `match` statement brings a form of pattern matching to Python, which can serve as a more powerful alternative to the switch statement.
“`python
def match_example(value):
match value:
case 1:
return “One”
case 2:
return “Two”
case 3:
return “Three”
case _:
return “Invalid”
“`
Comparison of Methods
The following table summarizes the pros and cons of each method for simulating switch-like behavior in Python:
Method | Pros | Cons |
---|---|---|
If-Elif-Else | Simple and easy to understand. | Can become unwieldy with many conditions. |
Dictionaries | Compact and flexible, supports functions as values. | Requires more setup, less intuitive for simple cases. |
Match Statement | Powerful and expressive for complex patterns. | Requires Python 3.10 or later. |
Each method has its own advantages and potential drawbacks, and the choice often depends on the specific requirements of the application being developed. Understanding these alternatives allows Python developers to write clean, efficient, and readable code without the need for a traditional switch statement.
Understanding the Absence of a Switch Statement in Python
Python does not have a built-in switch statement, which is a common feature in many other programming languages. Instead, Python utilizes alternative constructs that provide similar functionality. The absence of a switch statement in Python is often attributed to the language’s design philosophy, which favors readability and simplicity.
Alternatives to Switch Statements
While Python lacks a traditional switch statement, developers can achieve similar results using several methods:
- If-Elif-Else Chains: This is the most straightforward alternative. It allows for multiple conditional checks in a clear and readable manner.
“`python
def switch_example(value):
if value == 1:
return “One”
elif value == 2:
return “Two”
elif value == 3:
return “Three”
else:
return “Invalid value”
“`
- Dictionaries as Switch Alternatives: Dictionaries can be utilized to map keys to functions or values, effectively simulating a switch case.
“`python
def case_one():
return “One”
def case_two():
return “Two”
def case_three():
return “Three”
def switch_dict(value):
switcher = {
1: case_one,
2: case_two,
3: case_three
}
return switcher.get(value, lambda: “Invalid value”)()
“`
- Match Statement (Python 3.10 and later): The of structural pattern matching in Python 3.10 provides a powerful new way to handle multiple conditions.
“`python
def match_example(value):
match value:
case 1:
return “One”
case 2:
return “Two”
case 3:
return “Three”
case _:
return “Invalid value”
“`
Comparison of Alternatives
The following table outlines the strengths and weaknesses of each alternative to the switch statement:
Method | Strengths | Weaknesses |
---|---|---|
If-Elif-Else | Simple and easy to understand | Can become unwieldy with many conditions |
Dictionary Mapping | Clean and efficient for large sets of cases | Requires defining functions or values separately |
Match Statement | Powerful pattern matching; concise syntax | Requires Python 3.10 or later |
Best Practices
When implementing alternatives to the switch statement in Python, consider the following best practices:
- Readability: Choose a method that enhances code readability. If a simple if-elif-else structure is sufficient, it may be the best option.
- Maintainability: Use dictionaries for larger cases where functions are involved. This keeps the codebase cleaner and easier to maintain.
- Utilize Match: If using Python 3.10 or later, leverage the match statement for complex conditional logic, as it provides a more elegant solution than traditional if-elif structures.
By understanding these alternatives and best practices, developers can effectively manage control flow in Python applications without a switch statement.
Expert Insights on Python’s Lack of a Switch Statement
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “While Python does not have a traditional switch statement like many other programming languages, its design philosophy encourages the use of dictionary mappings and if-elif-else constructs, which can achieve similar outcomes with more flexibility.”
Michael Chen (Lead Python Developer, Open Source Solutions). “The absence of a switch statement in Python is intentional, as it promotes readability and simplicity. Developers can utilize function dispatching or pattern matching (introduced in Python 3.10) to handle multiple conditions effectively.”
Sarah Thompson (Python Educator and Author). “Many beginners often miss the switch statement when transitioning to Python, but understanding the alternatives like dictionaries and comprehensions can enhance their coding skills and adaptability in Python’s unique ecosystem.”
Frequently Asked Questions (FAQs)
Does Python have a built-in switch statement?
No, Python does not have a built-in switch statement like some other programming languages. However, similar functionality can be achieved using dictionaries or if-elif-else chains.
What is the alternative to a switch statement in Python?
The most common alternatives to a switch statement in Python are using dictionaries to map cases to functions or values, or employing if-elif-else statements for conditional logic.
How can I implement a switch-like structure in Python?
You can implement a switch-like structure in Python by creating a dictionary where keys represent cases and values are functions or results. Call the function or retrieve the value based on the key.
Are there any libraries that provide switch statement functionality in Python?
While Python does not have a native switch statement, third-party libraries like `PySwitch` can be used to mimic switch-case behavior, though they are not commonly used in standard Python programming.
Is there a performance difference between using if-elif-else and a switch-like dictionary in Python?
In general, using a dictionary for a switch-like structure can offer better performance for a large number of cases, as dictionary lookups are typically faster than evaluating multiple if-elif conditions.
Can I use match-case statements in Python?
Yes, starting from Python 3.10, the `match-case` statement was introduced, which provides a structural pattern matching feature that serves a similar purpose to switch statements in other languages.
In summary, Python does not have a traditional switch statement as found in many other programming languages. Instead, Python developers often utilize alternative constructs such as if-elif-else chains or dictionaries to achieve similar functionality. These methods allow for clear and efficient handling of multiple conditional branches, demonstrating Python’s flexibility in managing control flow.
One of the key insights is that while the absence of a switch statement may seem limiting at first, the use of if-elif-else constructs provides a straightforward and readable approach to branching logic. Additionally, employing dictionaries to map keys to functions or values can create an effective and elegant solution for scenarios that would typically require a switch statement.
Ultimately, understanding these alternatives not only enhances a developer’s ability to write clean and efficient code but also aligns with Python’s design philosophy of simplicity and readability. By leveraging these constructs, Python programmers can implement robust decision-making processes without the need for a dedicated switch statement.
Author Profile
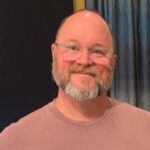
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?