How Can You Override Class Validator Rules Effectively?
In the world of software development, ensuring that data adheres to specific rules and formats is paramount. This is where class validators come into play, acting as the gatekeepers of data integrity. However, there are times when the default validation rules simply don’t align with your unique application requirements. This is where the art of overriding validation rules becomes essential. Whether you’re working with user input, API responses, or database entries, mastering the skill of customizing class validators can elevate your application to new heights of robustness and reliability.
Overriding validation rules allows developers to tailor the behavior of validation frameworks to meet specific needs, enhancing the flexibility of data handling. By understanding how to effectively customize these rules, you can ensure that your application not only meets functional requirements but also adheres to the nuances of your business logic. This process involves a careful balance of extending existing validators and creating new ones, all while maintaining the integrity and readability of your code.
As we delve deeper into the intricacies of overriding class validator rules, we will explore various techniques and best practices that empower developers to implement custom validations seamlessly. From understanding the underlying principles of validation frameworks to practical examples that illustrate the process, this guide will equip you with the knowledge to enhance your application’s data validation capabilities. Get ready to unlock the full potential
Understanding Class Validator Rules
Class Validator is a powerful library used for validating the properties of classes, primarily in TypeScript and JavaScript applications. The library provides a set of built-in rules that can be applied to class properties to ensure they meet specific criteria. However, there are scenarios where the default rules may not suffice, and you may need to override or extend them to cater to your application’s requirements.
Overriding rules allows developers to create custom validation logic tailored to their specific needs. This flexibility is essential for ensuring data integrity and enhancing user experience.
How to Override a Rule
To override a rule in Class Validator, you typically create a custom validation decorator. This involves implementing a function that takes the value being validated and any parameters needed for the validation logic. Below are the steps to create a custom rule:
- Define a Custom Decorator: Use the `registerDecorator` function to create a new validation decorator.
- Implement Validation Logic: Write the logic that determines whether the value is valid or not.
- Attach the Decorator: Apply the custom decorator to the class property that requires validation.
Here is an example of how to create a custom rule that checks if a string contains only alphabetic characters:
“`typescript
import { registerDecorator, ValidationOptions, ValidationArguments } from ‘class-validator’;
function IsAlpha(validationOptions?: ValidationOptions) {
return function (object: Object, propertyName: string) {
registerDecorator({
name: ‘isAlpha’,
target: object.constructor,
propertyName: propertyName,
options: validationOptions,
validator: {
validate(value: any, args: ValidationArguments) {
return typeof value === ‘string’ && /^[A-Za-z]+$/.test(value);
},
defaultMessage(args: ValidationArguments) {
return `${args.property} must contain only alphabetic characters`;
},
},
});
};
}
“`
Applying the Custom Rule
Once the custom rule is defined, it can be applied to any class property. Here’s how you can utilize the `IsAlpha` decorator in a class:
“`typescript
import { IsAlpha } from ‘./validators’;
class User {
@IsAlpha({ message: ‘Username must be alphabetic’ })
username: string;
constructor(username: string) {
this.username = username;
}
}
“`
Testing Your Custom Rule
It’s essential to test your custom validation rule to ensure it works correctly. You can use the Class Validator’s `validate` function to check if an instance of your class meets the validation criteria.
Here’s an example of how to validate the `User` class:
“`typescript
import { validate } from ‘class-validator’;
async function validateUser() {
const user = new User(‘JohnDoe123’);
const errors = await validate(user);
if (errors.length > 0) {
console.log(‘Validation failed. Errors: ‘, errors);
} else {
console.log(‘Validation succeeded!’);
}
}
validateUser();
“`
Common Use Cases for Custom Validation Rules
Custom validation rules can address various scenarios, including:
- Complex Business Logic: When validation involves multiple properties or requires intricate logic.
- Third-Party API Integration: To ensure data conforms to external requirements.
- Localized Validations: Adapting validation messages or logic based on user locale.
Use Case | Example |
---|---|
Email Validation | Check for proper email format and domain validity. |
Age Validation | Ensure age is within a specific range. |
Password Strength | Enforce complexity requirements for passwords. |
By leveraging custom validation rules, developers can ensure that their applications maintain high standards of data integrity and provide a seamless user experience.
Understanding Class Validator
Class Validator is a powerful library in TypeScript and JavaScript that provides decorators to validate objects. It streamlines the validation process by allowing developers to annotate classes with validation rules, which are then enforced at runtime. However, there may be instances where the default rules do not fit your specific requirements. In such cases, overriding rules becomes necessary.
Why Override Validation Rules?
Overriding validation rules allows for:
- Customization: Tailor the validation process to meet specific business logic needs.
- Complex Logic: Implement intricate validation scenarios that go beyond simple constraints.
- Integration: Align validation behavior with other application components or libraries.
How to Override Validation Rules
To effectively override validation rules in Class Validator, you can follow these steps:
- Create a Custom Decorator:
You can create a custom decorator by defining a function that returns a validator function.
“`typescript
import { registerDecorator, ValidationOptions, ValidatorConstraint, ValidatorConstraintInterface } from ‘class-validator’;
@ValidatorConstraint({ async: })
export class IsCustomConstraint implements ValidatorConstraintInterface {
validate(value: any) {
// Custom validation logic
return value === ‘customValue’; // Example condition
}
}
export function IsCustom(validationOptions?: ValidationOptions) {
return function (object: Object, propertyName: string) {
registerDecorator({
name: ‘isCustom’,
target: object.constructor,
propertyName: propertyName,
options: validationOptions,
validator: IsCustomConstraint,
});
};
}
“`
- Apply the Custom Decorator:
Use the newly created custom decorator in your class.
“`typescript
import { IsCustom } from ‘./custom-decorator’;
class MyDto {
@IsCustom({ message: ‘Value must be customValue’ })
myProperty: string;
}
“`
Using Built-in Decorators with Custom Logic
Sometimes, you may want to combine built-in validators with your custom logic. This can be achieved using the `@ValidateIf` decorator, allowing you to conditionally apply validation rules.
“`typescript
import { IsEmail, ValidateIf } from ‘class-validator’;
class User {
@ValidateIf(o => o.isEmailRequired)
@IsEmail({}, { message: ‘Invalid email format’ })
email: string;
isEmailRequired: boolean;
}
“`
Common Scenarios for Overriding Rules
The following table outlines common scenarios where overriding validation rules may be necessary:
Scenario | Default Rule | Custom Solution |
---|---|---|
Conditional validation | N/A | Use `@ValidateIf` decorator |
Complex relationship checks | N/A | Create a custom validator |
Cross-field validation | N/A | Use multiple custom validators |
Custom error messages | Default messages | Provide custom messages in options |
Best Practices for Custom Validators
- Keep Logic Simple: Ensure the logic within custom validators is straightforward to maintain clarity.
- Use Descriptive Names: Name custom decorators meaningfully for better code readability.
- Document Custom Logic: Provide comments or documentation for complex validation rules to aid future developers.
Testing Custom Validation Rules
Testing is crucial for verifying that your custom validation logic works as intended. You can use frameworks like Jest for unit testing your validators:
“`typescript
import { validate } from ‘class-validator’;
describe(‘MyDto Validation’, () => {
it(‘should validate successfully with custom rule’, async () => {
const dto = new MyDto();
dto.myProperty = ‘customValue’;
const errors = await validate(dto);
expect(errors.length).toBe(0);
});
});
“`
Following these practices and utilizing the mechanisms provided by Class Validator will enable you to effectively override rules and implement a robust validation strategy tailored to your application needs.
Expert Insights on Overriding Class Validator Rules
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Overriding rules in Class Validator is essential for tailoring validation logic to specific application needs. By extending existing validators, developers can implement custom validation criteria while maintaining the integrity of the original framework.”
Michael Chen (Lead Developer, CodeCraft Solutions). “To effectively override a rule in Class Validator, one should create a new class that extends the base validator and implement the desired logic in the `validate` method. This approach ensures that the new rule integrates seamlessly with existing validation processes.”
Sarah Thompson (Technical Architect, Future Tech Labs). “When overriding Class Validator rules, it is crucial to consider the implications on performance and maintainability. Leveraging annotations and configuration files can simplify the process and enhance the clarity of custom validation rules.”
Frequently Asked Questions (FAQs)
What is Class Validator?
Class Validator is a powerful library used in TypeScript and JavaScript applications to validate object properties based on defined rules. It provides decorators that can be applied to class properties to enforce validation logic.
How can I override a validation rule in Class Validator?
To override a validation rule in Class Validator, you can create a custom validation decorator. This involves defining a function that implements the desired validation logic and using the `@Validate` decorator to apply it to the class property.
Can I create multiple custom validation rules?
Yes, you can create multiple custom validation rules in Class Validator. Each rule can be defined as a separate function, allowing you to apply different validation logic to various properties within your classes.
How do I use a custom validation decorator?
To use a custom validation decorator, define the decorator function that implements your validation logic. Then, apply the decorator to the desired class property using the `@` symbol, just like with built-in decorators.
Is it possible to combine multiple validation rules on a single property?
Yes, you can combine multiple validation rules on a single property by applying multiple decorators to that property. Class Validator will execute all specified validation rules when validating the object.
What are some common use cases for overriding validation rules?
Common use cases for overriding validation rules include implementing complex validation logic that cannot be achieved with built-in rules, providing custom error messages, and adapting validation to specific business requirements.
overriding rules in a class validator is a crucial skill for developers seeking to customize validation logic in their applications. Class validators provide a framework for ensuring that data adheres to specific criteria, but there are instances where the default rules may not suffice. By understanding how to extend or modify these rules, developers can create more robust and tailored validation processes that meet the unique requirements of their projects.
One of the key takeaways from the discussion is the importance of leveraging inheritance and composition when overriding validation rules. By creating subclasses or utilizing decorators, developers can effectively implement custom validation logic without compromising the integrity of the existing validation structure. This approach not only enhances code maintainability but also promotes reusability across different components of an application.
Additionally, it is essential to thoroughly test any overridden rules to ensure they function as intended and do not introduce new issues. Implementing unit tests can help validate the behavior of custom rules and provide confidence in their reliability. Overall, mastering the art of overriding class validator rules empowers developers to build more flexible and user-friendly applications.
Author Profile
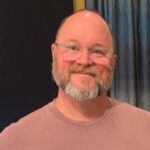
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?