Why Do I Encounter ‘Cannot Create A File When That File Already Exists’ Errors?
In the digital age, where data management and file organization are paramount, encountering the error message “Cannot create a file when that file already exists” can be both frustrating and perplexing. This seemingly straightforward notification can halt your productivity and leave you questioning the integrity of your work. Whether you’re a seasoned programmer, a casual user, or a business professional managing critical documents, understanding the implications of this error is essential for smooth workflows and efficient file handling.
At its core, this error arises when an attempt is made to create a new file with a name that already exists in the designated directory. This situation can occur in various contexts, from software development environments to everyday document management systems. The implications of this error extend beyond mere inconvenience; they can disrupt project timelines, lead to data loss, and complicate collaborative efforts.
As we delve deeper into this topic, we will explore the common scenarios that trigger this error, the underlying principles of file management that contribute to it, and practical strategies for resolving the issue. By gaining a better understanding of how file creation and naming conventions work, you can enhance your ability to navigate these challenges and maintain a more organized digital workspace.
Understanding the Error Message
The error message “Cannot create a file when that file already exists” typically arises in programming and software development contexts. It occurs when an attempt is made to create a file with the same name as an existing one in the specified directory. This error can lead to confusion, especially if the developer is unaware of the current file system state or the existence of the file in question.
When this error is encountered, it is essential to consider the following:
- File Overwrite Protection: Many operating systems and programming environments are designed to prevent accidental overwrites of existing files to safeguard data integrity.
- Permissions: The current user may lack the necessary permissions to modify or delete the existing file, contributing to the inability to create a new file.
- File System Constraints: Some file systems impose specific rules about file naming and existence that can trigger this error.
Common Causes
There are several reasons why this error may occur, including:
- Existing File: The most straightforward cause is that a file with the same name already exists in the target directory.
- Temporary Files: Sometimes, applications create temporary files that are not immediately visible but can still trigger this error.
- File Locks: If another process has the file open or locked, it may prevent the creation of a new file with the same name.
How to Resolve the Error
To address the issue effectively, consider the following steps:
- Check for Existing Files: Before attempting to create a new file, verify whether a file with the same name exists in the target directory.
- Rename the New File: If applicable, modify the name of the file you intend to create to avoid conflicts.
- Delete or Move the Existing File: If the existing file is no longer needed, consider deleting or moving it to a different location.
- Review Permissions: Ensure you have the necessary permissions to create files in the specified directory.
Best Practices
Implementing best practices can minimize the occurrence of this error:
- File Naming Conventions: Utilize clear and systematic naming conventions to avoid duplicates.
- Error Handling: Implement robust error handling in your code to manage exceptions related to file creation.
- Regular Cleanup: Regularly clean up directories to remove unnecessary files, reducing clutter and potential conflicts.
Example Scenario
In a programming context, consider the following pseudocode that illustrates a potential error:
“`python
file_path = “example.txt”
try:
with open(file_path, ‘x’) as file:
file.write(“This is an example.”)
except FileExistsError:
print(“Cannot create a file when that file already exists.”)
“`
In this example, the code attempts to create a new file using the ‘x’ mode, which raises a `FileExistsError` if the file already exists.
Table of File Creation Modes
Mode | Description |
---|---|
‘w’ | Open for writing, truncating the file first. If the file does not exist, it is created. |
‘a’ | Open for writing, appending to the end of the file if it exists. If the file does not exist, it is created. |
‘x’ | Open for exclusive creation. If the file already exists, the operation fails. |
‘r’ | Open for reading. If the file does not exist, an error is raised. |
Understanding the Error Message
The error message “Cannot create a file when that file already exists” typically occurs in various operating systems and applications when an attempt is made to create a file that has the same name as an existing file in the specified directory. This situation can arise under several circumstances:
- File Naming Conflicts: The most common reason is that a file with the same name already exists in the target location.
- Permissions Issues: Sometimes, permissions may prevent overwriting an existing file.
- File System Errors: Corrupted file systems may mistakenly report that a file does not exist when it actually does.
Common Scenarios for the Error
This error can occur in different contexts, such as:
- File Management Operations:
- Copying files to a directory where a file of the same name already exists.
- Attempting to save a document with a name that is currently in use.
- Programming and Scripting:
- Writing scripts that attempt to create files without checking for their existence.
- Using functions or methods that do not allow file overwriting.
- Software Applications:
- Applications like word processors or graphic design tools may trigger this error when saving files.
Resolving the Error
To effectively resolve this error, you can follow these strategies:
- Rename the File: Choose a unique name for the new file to avoid conflicts.
- Delete or Move Existing File: If the existing file is no longer needed, delete it or move it to another location.
- Check Permissions: Ensure you have the necessary permissions to overwrite files in the target directory.
- Use File Management Commands: If you are using a command-line interface, consider employing commands that allow conditional file creation. For instance, in Unix/Linux, you can use:
“`bash
touch filename.txt || echo “File exists”
“`
Preventive Measures
To avoid encountering this error in the future, consider implementing the following practices:
- File Name Validation: Before creating a file, check if it already exists using relevant functions in your programming language.
- Version Control: Implement versioning in file management to keep track of changes without overwriting existing files.
- User Prompts: In applications, prompt users to confirm overwriting an existing file before proceeding.
Key Programming Solutions
Here are some programming solutions to handle this error in common programming languages:
Language | Code Example |
---|---|
Python | “`python if not os.path.exists(“file.txt”): with open(“file.txt”, “w”) as f: f.write(“data”)“` |
JavaScript | “`javascript if (!fs.existsSync(“file.txt”)) { fs.writeFileSync(“file.txt”, “data”); }“` |
Java | “`java File file = new File(“file.txt”); if (!file.exists()) { FileWriter writer = new FileWriter(file); writer.write(“data”); writer.close(); }“` |
Implementing these strategies will help mitigate the occurrence of the “Cannot create a file when that file already exists” error.
Understanding File Management Errors in Computing
Dr. Emily Carter (Software Engineer, Tech Innovations Inc.). “The error message ‘Cannot create a file when that file already exists’ typically arises when a program attempts to generate a file with a name that is already in use. It is essential for developers to implement checks that verify file existence before attempting to create new files, thereby preventing unnecessary errors and enhancing user experience.”
Mark Thompson (IT Support Specialist, Global Tech Solutions). “In many cases, users encounter this error due to misunderstandings about file management systems. Educating users on how to navigate file directories and manage existing files can significantly reduce the frequency of this issue. Additionally, offering clear error messages can guide users toward resolving the problem independently.”
Linda Garcia (Data Management Consultant, DataWise Consulting). “From a data management perspective, the inability to create a file due to its existing counterpart highlights the need for robust file versioning systems. Implementing version control not only prevents conflicts but also allows users to maintain historical data without overwriting existing files, thus improving data integrity and accessibility.”
Frequently Asked Questions (FAQs)
What does the error “Cannot Create A File When That File Already Exists” mean?
This error indicates that an attempt was made to create a file with a name that already exists in the specified directory. The operating system prevents overwriting existing files to avoid data loss.
How can I resolve the “Cannot Create A File When That File Already Exists” error?
To resolve this error, you can either delete or rename the existing file, or choose a different name for the new file you are trying to create.
What should I check if I encounter this error while using an application?
Check the file path and name you are using to ensure it does not conflict with an existing file. Additionally, verify that you have the necessary permissions to modify the directory.
Can this error occur in cloud storage services?
Yes, cloud storage services can also generate this error if you attempt to upload a file with the same name as an existing file in the same location. Renaming or replacing the file may be necessary.
Is there a way to force overwrite the existing file?
Most applications provide an option to overwrite existing files, but this typically requires explicit permission or selection. Review the application’s settings or prompts to enable this feature.
What programming languages commonly encounter this error?
This error can occur in various programming languages, including Python, Java, and C, particularly when file handling functions attempt to create files without checking for existing names.
In summary, the phrase “Cannot create a file when that file already exists” typically indicates an error encountered in various computing environments when attempting to create a file that is already present in the designated directory. This situation can arise in different operating systems and programming contexts, where file management protocols are in place to prevent overwriting existing files inadvertently. Understanding this error is crucial for developers and users alike, as it highlights the importance of proper file handling and management practices.
Key takeaways from this discussion include the necessity of implementing checks before file creation processes. By verifying the existence of a file before attempting to create it, users can avoid unnecessary errors and ensure smoother operation of their applications. Moreover, utilizing error handling techniques, such as try-catch blocks in programming, can provide more robust solutions and enhance user experience by offering informative feedback when such errors occur.
Additionally, it is beneficial to adopt best practices in file naming conventions and directory organization. This approach can minimize the likelihood of encountering conflicts with existing files. Ultimately, being proactive in file management not only prevents errors but also contributes to more efficient workflows and data integrity within systems.
Author Profile
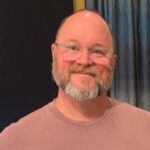
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?