How Can You Effectively Get Input in Python?
In the world of programming, the ability to gather input from users is a fundamental skill that can transform a simple script into an interactive application. Python, known for its simplicity and readability, offers various methods to capture input, making it an ideal choice for both beginners and seasoned developers alike. Whether you’re creating a command-line tool, a game, or a data processing script, understanding how to effectively get input from users is essential for enhancing functionality and user experience. In this article, we will explore the different techniques available in Python for obtaining user input, equipping you with the tools to make your programs more dynamic and engaging.
To start, it’s important to recognize that Python provides built-in functions that allow developers to easily prompt users for information. The most commonly used method is the `input()` function, which captures text input from the console. However, the way input is handled can vary significantly depending on the type of data you need, whether it’s a simple string, a number, or even a more complex structure. Understanding these nuances will help you tailor your approach to meet the specific needs of your application.
Moreover, as you delve deeper into the topic, you’ll discover various strategies for validating and processing user input. Ensuring that the data collected is accurate and in the
Using the `input()` Function
The primary method for obtaining user input in Python is through the built-in `input()` function. This function allows you to prompt the user for information, which is then returned as a string. The usage is straightforward:
“`python
user_input = input(“Please enter your name: “)
“`
In this example, the string “Please enter your name: ” is displayed to the user, and whatever they type in response is stored in the variable `user_input`. It is important to note that the data collected from `input()` is always in string format, necessitating further conversion if numerical data is required.
Type Conversion
When numeric input is needed, conversion of the string input to the appropriate type must be performed. Python provides several built-in functions for this purpose, including `int()`, `float()`, and `str()`. The following illustrates this concept:
“`python
age_input = input(“Please enter your age: “)
age = int(age_input) Convert the string input to an integer
“`
Here are common type conversions:
- Integer Conversion: `int()`
- Float Conversion: `float()`
- String Conversion: `str()`
It is crucial to handle potential errors when converting types, as users may enter unexpected values.
Error Handling
To manage erroneous input and ensure the program runs smoothly, implementing error handling is essential. Utilizing a `try-except` block can help capture exceptions that arise from invalid input.
“`python
try:
age = int(input(“Please enter your age: “))
except ValueError:
print(“That’s not a valid age!”)
“`
This code will catch a `ValueError` if the input cannot be converted to an integer, allowing for a more graceful user experience.
Reading Multiple Inputs
In scenarios where multiple inputs are required, you can call the `input()` function multiple times or utilize list comprehension. For instance, to gather multiple names:
“`python
names = input(“Enter names separated by commas: “).split(‘,’)
“`
This code snippet will take a single line of input, split it by commas, and store the resulting list in the variable `names`.
Table of Input Types
The following table summarizes how to handle different types of inputs in Python:
Input Type | Method | Example Code |
---|---|---|
String | input() | name = input(“Enter your name: “) |
Integer | int(input()) | age = int(input(“Enter your age: “)) |
Float | float(input()) | height = float(input(“Enter your height: “)) |
List | split() | items = input(“Enter items: “).split(‘,’) |
This table serves as a quick reference for various input types and their corresponding methods in Python, aiding developers in efficiently gathering user data.
Using the Input Function
In Python, the primary method to receive user input is through the `input()` function. This function reads a line from input, converts it into a string, and returns it. Here’s how to effectively use it:
“`python
user_input = input(“Please enter your name: “)
print(“Hello, ” + user_input + “!”)
“`
Key points to remember:
- The string passed to `input()` is a prompt displayed to the user.
- The input is always returned as a string, regardless of the data type entered.
Handling Different Data Types
To handle inputs of various data types, explicit conversion is necessary. Here are common conversions:
- Integer: Use `int()`
- Float: Use `float()`
- String: No conversion needed, as `input()` returns a string
Example of converting input to different types:
“`python
age = int(input(“Enter your age: “))
height = float(input(“Enter your height in meters: “))
“`
Validating User Input
Input validation is crucial for ensuring the data received meets expected formats and constraints. Here are methods to validate input:
- Try-Except Blocks: Catch exceptions for invalid conversions.
- While Loops: Re-prompt the user until valid input is received.
Example of input validation:
“`python
while True:
try:
age = int(input(“Enter your age: “))
if age < 0:
raise ValueError("Age cannot be negative.")
break
except ValueError as e:
print("Invalid input. Please enter a valid age.")
```
Using Regular Expressions for Complex Validation
For complex input patterns, the `re` module can be utilized. This allows for sophisticated validation techniques.
Example of validating an email address:
“`python
import re
email = input(“Enter your email: “)
pattern = r’^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$’
if re.match(pattern, email):
print(“Valid email address.”)
else:
print(“Invalid email address.”)
“`
Reading Multiple Inputs
To read multiple inputs in one line, you can use the `split()` method. This allows users to input values separated by spaces.
Example of reading multiple integers:
“`python
numbers = input(“Enter numbers separated by spaces: “).split()
numbers = [int(num) for num in numbers]
“`
Advanced Input Handling
For advanced scenarios, consider using libraries like `argparse` for command-line arguments or `getpass` for password inputs.
- Argparse: This library allows you to create user-friendly command-line interfaces.
Example using `argparse`:
“`python
import argparse
parser = argparse.ArgumentParser(description=’Process some integers.’)
parser.add_argument(‘integers’, metavar=’N’, type=int, nargs=’+’, help=’an integer for the accumulator’)
args = parser.parse_args()
print(args.integers)
“`
- Getpass: This is useful for securely capturing passwords without echoing them.
Example using `getpass`:
“`python
import getpass
password = getpass.getpass(“Enter your password: “)
“`
By employing these techniques, you can robustly manage user input in your Python applications, ensuring both functionality and security.
Expert Insights on Obtaining User Input in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively get user input in Python, utilizing the built-in `input()` function is essential. This function allows for seamless interaction with users, enabling the retrieval of data in a straightforward manner. It is crucial to ensure that the input is validated and sanitized to prevent potential security vulnerabilities.”
Mark Thompson (Python Developer Advocate, CodeCraft). “When working with user input in Python, it is important to consider the context of the application. For command-line interfaces, the `input()` function suffices, but for GUI applications, libraries like Tkinter or PyQt provide more robust options for user interaction. Always aim for a user-friendly experience.”
Lisa Chen (Data Scientist, Analytics Hub). “In data-driven applications, gathering user input can significantly influence the outcomes of analyses. Using `input()` to collect parameters is a good start, but incorporating libraries such as `argparse` for command-line arguments can enhance flexibility and usability in scripts designed for data processing.”
Frequently Asked Questions (FAQs)
How do I get user input in Python?
You can use the built-in `input()` function in Python to get user input. For example, `user_input = input(“Enter something: “)` will prompt the user to enter data.
What type of data does the input() function return?
The `input()` function always returns data as a string. If you need a different data type, you must convert it explicitly using functions like `int()`, `float()`, or `bool()`.
Can I get multiple inputs in a single line?
Yes, you can use the `input()` function to get multiple inputs in a single line by prompting the user to separate values with a specific character, such as a comma. You can then split the string using the `split()` method.
How can I handle exceptions when getting input?
You can handle exceptions using a `try` and `except` block. This allows you to catch errors, such as invalid data types, and prompt the user to enter the input again.
Is there a way to set a default value for user input?
The `input()` function does not support default values directly. However, you can implement this by checking if the user input is empty and assigning a default value if it is.
How can I validate user input in Python?
You can validate user input by using conditional statements to check if the input meets specific criteria. If the input is invalid, you can prompt the user to enter the data again until it is valid.
In Python, obtaining user input is a fundamental aspect of programming that enhances interactivity and functionality. The primary method for capturing input from users is through the built-in `input()` function, which allows developers to prompt users for data during program execution. This function reads a line from input, converts it to a string, and returns it, thereby enabling the incorporation of user responses into the program’s logic. Understanding how to effectively use this function is crucial for creating dynamic and responsive applications.
Moreover, Python’s input handling can be further enhanced by implementing data type conversions, such as using `int()` or `float()` to convert string inputs into numerical values. This capability is essential for applications that require arithmetic operations or numerical analysis. Additionally, error handling techniques, such as try-except blocks, can be employed to manage invalid inputs gracefully, ensuring that the program remains robust and user-friendly.
In summary, mastering how to get input in Python is not only about using the `input()` function but also about understanding data types, conversions, and error management. These skills contribute significantly to a programmer’s ability to create effective and user-centric applications. By leveraging these techniques, developers can enhance the interactivity of their programs, leading to a more engaging
Author Profile
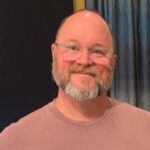
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?