How Can You Print Without a New Line in Python?
Printing output in Python is a fundamental skill that every programmer should master, yet it often comes with nuances that can trip up even seasoned developers. One such nuance is the default behavior of the `print()` function, which automatically adds a new line after each output. While this is convenient for most cases, there are times when you may want to control the formatting of your output more precisely. Whether you’re crafting a user interface, logging information, or simply trying to display data in a more readable format, knowing how to print without a new line can be incredibly useful.
In Python, the ability to customize the output of the `print()` function opens up a world of possibilities. By understanding how to manipulate the end character of the output, you can create dynamic and engaging displays that capture attention and convey information effectively. This technique is particularly valuable when you want to print multiple items on the same line or format your output in a specific way, such as creating progress indicators or displaying results in a tabular format.
As we delve deeper into this topic, we’ll explore the various methods and techniques available in Python to achieve line-less printing. From basic syntax adjustments to more advanced formatting options, you’ll learn how to take control of your output and enhance the interactivity of your programs. Get ready to elevate
Using the `print()` Function with `end` Parameter
In Python, the default behavior of the `print()` function is to end the output with a newline character. To print without adding a new line at the end, you can modify this behavior by using the `end` parameter. The `end` parameter allows you to specify a string that will be appended after the printed value.
For example:
“`python
print(“Hello”, end=’ ‘)
print(“World!”)
“`
In this example, the output will be:
“`
Hello World!
“`
Instead of starting a new line after “Hello”, a space is used as a separator, allowing “World!” to appear on the same line.
Examples of Different `end` Values
You can use various strings with the `end` parameter to customize the output format further. Here are some common examples:
- To use a comma:
“`python
print(“Apple”, end=’, ‘)
print(“Banana”, end=’, ‘)
print(“Cherry”)
“`
Output:
“`
Apple, Banana, Cherry
“`
- To use no space:
“`python
print(“A”, end=”)
print(“B”, end=”)
print(“C”)
“`
Output:
“`
ABC
“`
- To use a custom string:
“`python
print(“First”, end=’ | ‘)
print(“Second”, end=’ | ‘)
print(“Third”)
“`
Output:
“`
First | Second | Third
“`
Using `sys.stdout.write()` for More Control
Another approach to print without a newline is to use the `sys.stdout.write()` method. This method is more low-level than `print()` and allows for finer control over the output.
To utilize `sys.stdout.write()`, you need to import the `sys` module:
“`python
import sys
sys.stdout.write(“Hello “)
sys.stdout.write(“World!\n”)
“`
In this case, you must manually add any necessary newline characters (`\n`), as `sys.stdout.write()` does not automatically append them.
Comparison of `print()` vs `sys.stdout.write()`
Feature | `print()` | `sys.stdout.write()` |
---|---|---|
Automatic newline | Yes | No |
Formatting capabilities | Yes (with `sep`, `end`) | Minimal (only string output) |
Ease of use | High | Moderate |
Performance | Slower due to formatting | Faster for large outputs |
Both methods are useful, and the choice between them depends on your specific needs. For most casual uses, `print()` is more convenient, while `sys.stdout.write()` is preferable for performance-sensitive applications or when more control is necessary.
Conclusion on Printing Without Newlines
By understanding the `end` parameter in the `print()` function and the use of `sys.stdout.write()`, you can effectively manage how output appears in your Python programs, allowing for greater flexibility and control over your printed results.
Printing Without a New Line in Python
In Python, the default behavior of the `print()` function is to terminate with a newline character. However, there are scenarios where you may want to print multiple items on the same line or control the output format. Below are methods to achieve this.
Using the `end` Parameter
The `print()` function includes an `end` parameter that allows you to specify what should be printed at the end of the output instead of the default newline. By setting `end` to an empty string or another character, you can control the output.
“`python
print(“Hello”, end=” “)
print(“World!”)
“`
This code will output:
“`
Hello World!
“`
Common Uses of the `end` Parameter
- Empty String: To continue printing on the same line.
- Space: To separate outputs by a space.
- Comma: To separate outputs by a comma and space.
Using `sys.stdout.write()`
For more control over output, you can use `sys.stdout.write()`. This method does not automatically add a newline, allowing for more precise formatting.
“`python
import sys
sys.stdout.write(“Hello “)
sys.stdout.write(“World!”)
“`
This will produce the same output:
“`
Hello World!
“`
Advantages of `sys.stdout.write()`
- No Automatic Newline: Unlike `print()`, it does not append a newline character.
- Formatted Output: Allows for custom formatting without newline interference.
Formatting Output with `f-strings`
If you need to format your output dynamically while avoiding newlines, you can utilize f-strings introduced in Python 3.6. They can be combined with the `end` parameter for flexible output.
“`python
name = “Alice”
age = 30
print(f”My name is {name}, “, end=””)
print(f”and I am {age} years old.”)
“`
Output:
“`
My name is Alice, and I am 30 years old.
“`
Using `join()` for Concatenation
When you have multiple strings that you wish to print without newlines, the `join()` method can be particularly useful. This method combines strings from an iterable into a single string, which can then be printed in one go.
“`python
words = [“Python”, “is”, “fun”]
print(” “.join(words))
“`
The output will be:
“`
Python is fun
“`
Benefits of Using `join()`
- Efficiency: Combines multiple strings efficiently.
- Custom Separator: Allows for any character or string as a separator.
Example Scenarios
Method | Use Case |
---|---|
`print()` with `end` | Simple outputs on the same line. |
`sys.stdout.write()` | Advanced control of output format. |
f-strings | Dynamic content formatting. |
`join()` | Concatenating multiple strings. |
By utilizing these methods, you can effectively manage how data is printed in Python, ensuring that your output format meets specific requirements without unintended newlines.
Expert Insights on Printing Without New Lines in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “In Python, the `print()` function by default adds a newline after each call. To print without a new line, one can use the `end` parameter, setting it to an empty string or any other desired string. This allows for more control over the output formatting.”
Michael Chen (Lead Python Instructor, Code Academy). “Understanding the `end` parameter in the `print()` function is crucial for effective output management in Python. By default, it is set to `’\n’`, but changing it to `”` enables continuous output on the same line, which is particularly useful in loops or when displaying progress.”
Sarah Johnson (Data Scientist, Tech Innovations). “When dealing with real-time data outputs, such as in data streaming applications, utilizing the `print()` function with the `end` parameter can significantly enhance user experience by presenting information in a more cohesive manner, avoiding unnecessary line breaks.”
Frequently Asked Questions (FAQs)
How can I print multiple items on the same line in Python?
You can print multiple items on the same line by using the `print()` function with the `end` parameter set to an empty string or a space. For example: `print(“Hello”, end=’ ‘)` will print “Hello” without moving to a new line.
What is the default behavior of the print() function in Python?
The default behavior of the `print()` function in Python is to end with a newline character, which means each call to `print()` starts on a new line.
Can I use the print() function to format output without new lines?
Yes, you can format output without new lines by using the `end` parameter to specify a different ending character. For instance, `print(“Item 1”, end=’, ‘)` will print “Item 1,” followed by a space instead of a newline.
Is it possible to print a list without new lines in Python?
Yes, you can print a list without new lines by unpacking the list using the asterisk operator `*` in the `print()` function, along with the `end` parameter. For example: `print(*my_list, end=’ ‘)` will print all elements of `my_list` on the same line.
What are some common use cases for printing without new lines in Python?
Common use cases include displaying progress indicators, formatting output in tables, or creating interactive command-line applications where continuous output is required without line breaks.
How can I achieve a custom separator between printed items in Python?
You can achieve a custom separator by using the `sep` parameter in the `print()` function. For example: `print(“Apple”, “Banana”, “Cherry”, sep=’ | ‘)` will print “Apple | Banana | Cherry” on the same line.
In Python, printing output without automatically adding a new line at the end can be achieved by utilizing the `print()` function’s `end` parameter. By default, the `print()` function appends a newline character (`\n`) after each call, which results in each print statement appearing on a new line. However, by specifying a different value for the `end` parameter, such as an empty string or a space, users can control the formatting of the output more precisely.
For instance, using `print(“Hello”, end=” “)` will output “Hello” followed by a space instead of moving to the next line. This feature is particularly useful when generating output that requires continuous flow or when formatting data in a specific layout. Additionally, it can be employed in loops or when concatenating strings for display purposes.
Overall, understanding how to manipulate the `end` parameter in the `print()` function enhances a programmer’s ability to format output effectively. This capability is essential for creating user-friendly interfaces and for debugging, where clear and concise output is crucial. Mastery of such techniques contributes to more polished and professional Python applications.
Author Profile
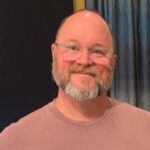
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?