Why Can’t I Make a Static Reference to a Non-Static Method?
In the world of programming, particularly in Java, encountering errors can be a common yet frustrating experience for developers at all levels. One such error that often leaves both novice and seasoned programmers scratching their heads is the infamous “Cannot make a static reference to the non-static method.” This error message serves as a crucial reminder of the distinctions between static and non-static contexts within object-oriented programming. Understanding this concept is not only essential for writing efficient code but also for mastering the nuances of Java itself.
When you delve into the intricacies of Java, you’ll find that the language’s design revolves around the principles of object-oriented programming, where the distinction between static and non-static elements plays a pivotal role. Static methods and variables belong to the class itself, while non-static members are tied to specific instances of the class. This fundamental difference can lead to confusion, especially when developers attempt to access non-static methods from a static context, resulting in the error message that has become a rite of passage for many.
Navigating this error requires a deeper understanding of how Java manages memory and object lifecycles. By exploring the underlying principles of static and non-static members, developers can not only troubleshoot their code more effectively but also enhance their overall programming skills. As we unpack the details of this error
Understanding Static and Non-Static Methods
In Java, the distinction between static and non-static methods is fundamental to how classes and objects operate. Static methods belong to the class itself, whereas non-static methods belong to instances of the class (objects). This distinction leads to the common error: “Cannot make a static reference to the non-static method.”
- Static Methods:
- Declared with the `static` keyword.
- Can be called without creating an instance of the class.
- Typically used for utility or helper methods that do not require any object state.
- Non-Static Methods:
- Do not have the `static` keyword.
- Require an instance of the class to be called.
- Often manipulate instance variables or rely on the object’s state.
This difference is crucial when trying to access a non-static method from a static context. If you attempt to call a non-static method directly from a static method (or a static context), the Java compiler will throw the “Cannot make a static reference to the non-static method” error.
Common Scenarios Leading to the Error
Several common scenarios can lead to this error:
- Calling Non-Static Method in Static Context:
When a static method attempts to call a non-static method directly without an object reference.
- Static Blocks:
Similar to static methods, static blocks cannot access non-static members directly.
- Static Classes:
In nested static classes, accessing non-static members of the outer class will also result in this error.
Solutions to the Error
To resolve the “Cannot make a static reference to the non-static method” error, consider the following approaches:
- Create an Instance: Instantiate the class to call the non-static method.
“`java
public class Example {
public void nonStaticMethod() {
System.out.println(“Non-static method called.”);
}
public static void staticMethod() {
Example example = new Example(); // Create instance
example.nonStaticMethod(); // Call non-static method
}
}
“`
- Change Method to Static: If the method does not rely on instance variables, change it to static.
“`java
public class Example {
public static void staticMethod() {
System.out.println(“Static method called.”);
}
}
“`
- Use an Object Reference: When calling a non-static method from a static method, always use an object reference.
Example Code
Here is an example demonstrating both the error and its resolution:
“`java
public class Demo {
public void displayMessage() {
System.out.println(“Hello from non-static method!”);
}
public static void main(String[] args) {
// Error: Cannot make a static reference to the non-static method displayMessage() from the type Demo
// displayMessage();
// Correct way
Demo demo = new Demo();
demo.displayMessage();
}
}
“`
Best Practices
To avoid such errors, adhere to the following best practices:
- Always be aware of the context (static vs. non-static) in which methods are called.
- Use static methods for operations that do not require object state.
- Encapsulate related functionality within instance methods when it relates to object data.
Method Type | Access Modifier | Instance Required |
---|---|---|
Static Method | Can be public, private, protected | No |
Non-Static Method | Can be public, private, protected | Yes |
Understanding the Error
The error message “Cannot make a static reference to the non-static method” typically occurs in Java when attempting to call an instance method from a static context. To clarify:
- Static Methods: Belong to the class rather than any specific instance. They can be called without creating an object of the class.
- Non-Static Methods: Require an instance of the class to be called. They can access instance variables and other instance methods directly.
This distinction leads to the error when trying to reference a non-static method directly from a static method or context.
Common Scenarios Leading to the Error
Several scenarios frequently lead to this error:
- Calling Non-Static Method from Static Method: Attempting to invoke an instance method within a static method without an object reference.
“`java
public class Example {
void instanceMethod() {
System.out.println(“Instance method called”);
}
static void staticMethod() {
instanceMethod(); // Error: Cannot make a static reference to the non-static method
}
}
“`
- Accessing Non-Static Method from Static Context: Trying to access a non-static method from a static block or initialization.
“`java
public class Example {
void instanceMethod() {
// Some logic
}
static {
instanceMethod(); // Error: Cannot make a static reference
}
}
“`
How to Resolve the Error
To resolve this error, consider the following approaches:
- Create an Instance: Instantiate the class containing the non-static method before calling it.
“`java
public class Example {
void instanceMethod() {
System.out.println(“Instance method called”);
}
static void staticMethod() {
Example example = new Example();
example.instanceMethod(); // Correct usage
}
}
“`
- Change Method to Static: If the method does not use any instance variables, consider making it static.
“`java
public class Example {
static void instanceMethod() {
System.out.println(“Static method called”);
}
static void staticMethod() {
instanceMethod(); // Now valid
}
}
“`
- Use Class Name: If the method belongs to another class, use the class name to call the static method.
“`java
public class AnotherClass {
static void staticMethod() {
System.out.println(“Static method in another class”);
}
}
public class Example {
static void staticMethod() {
AnotherClass.staticMethod(); // Valid call
}
}
“`
Best Practices to Avoid This Error
To prevent encountering this error in the future, consider the following best practices:
- Understand Context: Clearly differentiate between static and non-static contexts while designing your classes.
- Use Object-Oriented Principles: Utilize encapsulation and object-oriented principles to structure code effectively, reducing the need for static calls.
- Code Review: Regularly review your code for static and instance method calls to ensure proper context usage.
Example Code Review
Here’s an example illustrating both the error and its resolution:
“`java
public class Example {
void nonStaticMethod() {
System.out.println(“Non-static method called”);
}
static void staticMethod() {
// Error: Cannot make a static reference to the non-static method
nonStaticMethod();
}
public static void main(String[] args) {
Example example = new Example();
example.nonStaticMethod(); // Correct usage
staticMethod(); // This needs to be corrected
}
}
“`
In the above code, the call to `nonStaticMethod()` within `staticMethod()` generates the error. The solution involves creating an instance of `Example` to properly call the non-static method.
Understanding the Static Reference Error in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘Cannot make a static reference to the non-static method’ typically arises when developers attempt to call instance methods from a static context. It is essential to understand that static methods belong to the class itself, while non-static methods require an instance of the class to be invoked.”
Michael Chen (Java Development Specialist, CodeCraft Solutions). “To resolve this issue, developers should either create an instance of the class containing the non-static method or change the method to static if it does not rely on instance variables. This distinction is crucial for effective object-oriented programming.”
Lisa Patel (Lead Instructor, Advanced Java Programming Course). “Understanding the difference between static and non-static contexts is fundamental for Java programmers. This error not only highlights a common mistake but also serves as a reminder of the principles of encapsulation and object-oriented design.”
Frequently Asked Questions (FAQs)
What does “Cannot make a static reference to the non-static method” mean?
This error occurs in Java when you attempt to call a non-static method from a static context, such as a static method or a static block. Non-static methods require an instance of the class to be invoked.
How can I resolve the “Cannot make a static reference to the non-static method” error?
To resolve this error, create an instance of the class containing the non-static method and use that instance to call the method. Alternatively, you can change the method to static if it does not rely on instance variables.
Can I call a non-static method from a static method without creating an instance?
No, you cannot call a non-static method from a static method without an instance of the class. Static methods do not have access to instance-specific data or methods.
What is the difference between static and non-static methods in Java?
Static methods belong to the class itself and can be called without creating an object, while non-static methods belong to instances of the class and require an object to be accessed.
Are there any scenarios where calling a non-static method from a static context is acceptable?
Generally, it is not acceptable to call non-static methods from static contexts without an instance. However, you can use design patterns or static factory methods to manage instances effectively.
What are the implications of changing a non-static method to static?
Changing a non-static method to static may improve performance by avoiding object instantiation, but it restricts access to instance variables and methods, potentially altering the intended functionality of the code.
The error message “Cannot make a static reference to the non-static method” typically arises in object-oriented programming, particularly in Java. This error indicates that a static method is attempting to call a non-static method without an instance of the class. Static methods belong to the class itself, while non-static methods are associated with instances of the class. As a result, static methods cannot directly access instance methods or instance variables unless they create an instance of the class or have a reference to an existing instance.
To resolve this issue, developers must understand the distinction between static and non-static contexts. One common solution is to create an instance of the class containing the non-static method and then call the method on that instance. Alternatively, if the method does not rely on instance variables or other non-static methods, it may be beneficial to refactor the non-static method into a static method. This approach allows for greater flexibility in how methods are accessed and utilized throughout the codebase.
In summary, the “Cannot make a static reference to the non-static method” error serves as a reminder of the fundamental principles of object-oriented programming. Understanding the relationship between static and non-static methods is crucial for effective coding practices. By adhering to these principles, developers can avoid
Author Profile
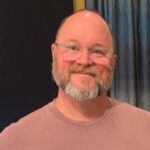
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?