How Can You Effectively Use a WHERE Clause with a CASE Statement in SQL?
In the world of SQL, the ability to filter and manipulate data is paramount for effective database management and insightful analysis. Among the powerful tools at a developer’s disposal is the combination of the WHERE clause and the CASE statement. This dynamic duo allows for nuanced queries that can adapt to various conditions, enabling users to extract precisely the information they need from complex datasets. Whether you are a seasoned SQL veteran or a newcomer eager to enhance your data querying skills, understanding how to leverage these constructs can significantly elevate your data manipulation capabilities.
The WHERE clause serves as the backbone of SQL queries, allowing users to specify criteria that must be met for records to be included in the result set. When paired with the CASE statement, which facilitates conditional logic within queries, the potential for data analysis expands exponentially. This combination empowers developers to create more sophisticated filters that can handle multiple scenarios, making it easier to derive insights from diverse data points.
As we delve deeper into the intricacies of using a WHERE clause with a CASE statement, we will explore practical applications, syntax variations, and best practices that can help streamline your SQL queries. By mastering this powerful technique, you will not only enhance your querying prowess but also gain a deeper understanding of how to manipulate and analyze data effectively in any relational database environment.
Using the CASE Statement in the WHERE Clause
The `CASE` statement can be employed within the `WHERE` clause to create conditional logic that filters results based on specific criteria. This allows for greater flexibility and precision when querying data. The `CASE` statement evaluates conditions and returns values accordingly, which can then be used to determine which records to include in the results.
Syntax of CASE in WHERE Clause
The basic syntax for using a `CASE` statement in a `WHERE` clause is as follows:
“`sql
SELECT column1, column2
FROM table_name
WHERE
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
ELSE default_result
END = some_value;
“`
In this syntax:
- `condition1`, `condition2`, etc. are the conditions being evaluated.
- `result1`, `result2`, and `default_result` are the values returned based on the conditions.
- `some_value` is the value you want to compare against the result of the `CASE` statement.
Example of CASE in WHERE Clause
Consider a scenario where you have an `employees` table and you want to filter employees based on their performance ratings, converting those ratings into more readable formats. Here’s how you could do it:
“`sql
SELECT employee_id, employee_name, performance_rating
FROM employees
WHERE
CASE
WHEN performance_rating >= 90 THEN ‘Excellent’
WHEN performance_rating >= 75 THEN ‘Good’
WHEN performance_rating >= 50 THEN ‘Average’
ELSE ‘Poor’
END = ‘Good’;
“`
In this example, only employees with a performance rating classified as ‘Good’ will be returned.
Benefits of Using CASE in WHERE Clause
- Enhanced Readability: Makes complex filtering conditions easier to understand.
- Dynamic Filtering: Allows for more dynamic queries that can adjust based on varying conditions.
- Reduced Complexity: Simplifies queries that would otherwise require multiple `OR` conditions.
Considerations When Using CASE in WHERE Clause
- Performance: Using `CASE` in the `WHERE` clause may impact performance if not used judiciously, particularly with large datasets.
- SQL Standards: Ensure that the SQL dialect being used supports the `CASE` statement in the `WHERE` clause, as implementations may vary.
Practical Applications
The `CASE` statement in the `WHERE` clause is particularly useful in scenarios such as:
- Dynamic Reporting: When generating reports that need to filter data based on user input or other dynamic criteria.
- Data Transformation: When transforming data values into more meaningful categorizations for analysis.
Performance Rating | Description |
---|---|
90 and above | Excellent |
75 to 89 | Good |
50 to 74 | Average |
Below 50 | Poor |
In summary, leveraging the `CASE` statement within the `WHERE` clause provides a powerful tool for executing complex queries that can adapt based on various conditions, enhancing the querying capabilities in SQL.
Understanding the CASE Statement
The CASE statement in SQL provides conditional logic, allowing you to return different values based on specified conditions. It operates like an IF-THEN-ELSE structure found in programming languages. The general syntax of the CASE statement is as follows:
“`sql
CASE
WHEN condition1 THEN result1
WHEN condition2 THEN result2
…
ELSE default_result
END
“`
Types of CASE Statements
- Simple CASE Statement: Compares an expression against a set of values.
- Searched CASE Statement: Evaluates a set of Boolean expressions to determine the result.
Example of a Simple CASE Statement
“`sql
SELECT
product_name,
CASE category_id
WHEN 1 THEN ‘Electronics’
WHEN 2 THEN ‘Clothing’
ELSE ‘Other’
END AS category_name
FROM products;
“`
Using CASE in the WHERE Clause
The WHERE clause can benefit from the CASE statement to create dynamic filtering criteria. This allows for more complex queries based on varying conditions.
Example of a CASE Statement in the WHERE Clause
“`sql
SELECT *
FROM orders
WHERE
order_status =
CASE
WHEN order_date < '2022-01-01' THEN 'Archived'
WHEN order_date >= ‘2022-01-01’ AND order_date < '2023-01-01' THEN 'Active'
ELSE 'Future'
END;
```
Important Considerations
- Ensure that the CASE statement returns a consistent data type across all branches.
- The CASE statement can simplify complex conditions but should be used judiciously to maintain query readability.
Practical Use Cases
Using the CASE statement within the WHERE clause can enhance data retrieval based on varying conditions. Here are some practical scenarios:
- Filtering by Status: Dynamically change the filtering criteria based on the current date.
- Conditional Aggregation: Adjusting filters based on user roles or permissions.
Example Use Case: Filtering by User Role
“`sql
SELECT user_id, user_name
FROM users
WHERE
user_role =
CASE
WHEN is_active = 1 THEN ‘Active User’
WHEN is_active = 0 AND last_login < DATEADD(year, -1, GETDATE()) THEN 'Inactive User'
ELSE 'New User'
END;
```
Performance Considerations
While using the CASE statement can simplify SQL queries, it is essential to consider its impact on performance. Here are some factors to keep in mind:
- Index Usage: CASE statements may prevent the use of indexes, leading to slower query performance.
- Complexity: Overly complex CASE statements can make queries difficult to read and maintain.
- Execution Plan: Always analyze the execution plan to understand how the SQL engine processes the query.
Recommendations
- Use CASE statements sparingly in the WHERE clause to maintain clarity.
- Optimize queries by testing performance with and without CASE statements.
- Leverage indexing on columns involved in the filtering conditions when possible.
The integration of the CASE statement within the WHERE clause allows for sophisticated data manipulation and retrieval strategies. By understanding its structure, applications, and performance implications, SQL developers can write more effective and efficient queries.
Expert Insights on Using Where Clause with Case Statement in SQL
Dr. Emily Chen (Senior Data Analyst, Tech Innovations Inc.). “Incorporating a CASE statement within a WHERE clause can significantly enhance the flexibility of your SQL queries. It allows for conditional filtering based on complex business logic, enabling more dynamic data retrieval that aligns with specific user requirements.”
Michael Thompson (Database Architect, Global Data Solutions). “Using a CASE statement in the WHERE clause can be a powerful tool for scenarios where multiple conditions need to be evaluated. However, it is crucial to ensure that performance is not compromised, as complex conditions can lead to slower query execution times.”
Sara Patel (SQL Consultant, Data Mastery Group). “When applying a CASE statement within a WHERE clause, clarity is essential. It is advisable to document the logic behind the conditions to maintain readability for other developers. This practice not only aids in collaboration but also helps in future maintenance of the SQL code.”
Frequently Asked Questions (FAQs)
What is a WHERE clause in SQL?
The WHERE clause in SQL is used to filter records that meet specific criteria, allowing users to retrieve only the data that is relevant to their queries.
What is a CASE statement in SQL?
The CASE statement in SQL is a conditional expression that allows users to perform IF-THEN-ELSE logic within queries, enabling dynamic value assignment based on specified conditions.
How can I use a CASE statement within a WHERE clause?
A CASE statement can be used within a WHERE clause to evaluate conditions and return a value that can be used for filtering. This allows for complex filtering based on multiple criteria.
Can you provide an example of a WHERE clause with a CASE statement?
Certainly. An example would be:
“`sql
SELECT * FROM Employees
WHERE Department = CASE
WHEN JobTitle = ‘Manager’ THEN ‘Management’
WHEN JobTitle = ‘Developer’ THEN ‘IT’
ELSE ‘Other’
END;
“`
Are there performance considerations when using CASE statements in WHERE clauses?
Yes, using CASE statements can impact query performance, especially with large datasets. It is advisable to analyze execution plans and optimize queries accordingly to maintain efficiency.
Is it possible to use multiple CASE statements in a single WHERE clause?
Yes, multiple CASE statements can be used in a single WHERE clause. However, it is essential to ensure that the logic remains clear and maintainable to avoid confusion in the query structure.
The use of a WHERE clause with a CASE statement in SQL is a powerful technique that allows for conditional filtering of query results. By integrating a CASE statement within the WHERE clause, users can create complex conditions that evaluate multiple criteria, enabling more dynamic and tailored data retrieval. This approach enhances the flexibility of SQL queries, making it possible to apply different filtering logic based on specific conditions directly within the same query context.
One of the key advantages of utilizing a CASE statement in the WHERE clause is the ability to streamline queries that would otherwise require multiple conditional statements or even subqueries. This not only simplifies the SQL syntax but also improves readability and maintainability of the code. Additionally, it can lead to performance optimizations by reducing the number of logical evaluations that the database engine must perform during query execution.
In practice, implementing a WHERE clause with a CASE statement can significantly enhance data analysis capabilities. It allows analysts and developers to derive insights from data by applying nuanced logic that reflects business rules or specific analytical needs. This technique is particularly useful in scenarios where data must be filtered based on varying criteria, such as user roles, transaction types, or time periods, ultimately leading to more informed decision-making.
Author Profile
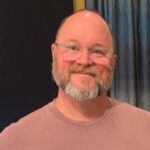
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?