How Can You Easily Convert a String to a JSON Object?
In today’s digital landscape, the ability to seamlessly manipulate data is more critical than ever. As applications increasingly rely on JSON (JavaScript Object Notation) for data interchange, developers often find themselves needing to convert strings into JSON objects. This conversion is not just a technical necessity; it’s a gateway to unlocking the full potential of data-driven applications. Whether you’re building a web service, developing a mobile app, or simply working with APIs, understanding how to convert strings to JSON objects will enhance your coding toolkit and streamline your workflow.
Converting a string to a JSON object may seem straightforward, but it encompasses a variety of techniques and considerations that can significantly impact your application’s performance and reliability. At its core, the process involves parsing a string formatted in JSON syntax and transforming it into a usable object within your programming environment. This transformation allows developers to access and manipulate data structures more efficiently, enabling dynamic interactions and real-time updates in applications.
As we delve deeper into this topic, we will explore the different methods available for converting strings to JSON objects across various programming languages. From built-in functions to third-party libraries, understanding the nuances of each approach will empower you to choose the best solution for your specific needs. Join us as we unravel the intricacies of JSON data handling and equip you with
Understanding JSON Structure
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. A JSON object is a collection of key/value pairs, where keys are strings and values can be strings, numbers, arrays, booleans, or other JSON objects.
The basic structure of a JSON object is as follows:
- Curly braces `{}` denote the beginning and end of the object.
- Key/value pairs are separated by commas.
- A key is always a string enclosed in double quotes.
- A value can be of various data types including:
- Strings (e.g., “value”)
- Numbers (e.g., 123)
- Arrays (e.g., [1, 2, 3])
- Booleans (e.g., true or )
- Null (e.g., null)
For example:
“`json
{
“name”: “John Doe”,
“age”: 30,
“isStudent”: ,
“courses”: [“Math”, “Science”],
“address”: {
“street”: “123 Main St”,
“city”: “Anytown”
}
}
“`
Converting String to JSON Object
To convert a string into a JSON object, you typically use a parsing function provided by the programming language you are working with. In JavaScript, for instance, you can use `JSON.parse()`.
JavaScript Example
“`javascript
const jsonString = ‘{“name”: “John Doe”, “age”: 30}’;
const jsonObject = JSON.parse(jsonString);
console.log(jsonObject.name); // Output: John Doe
“`
Python Example
In Python, the `json` module is used for this purpose. The `loads()` method can convert a string representation of JSON into a Python dictionary.
“`python
import json
json_string = ‘{“name”: “John Doe”, “age”: 30}’
json_object = json.loads(json_string)
print(json_object[‘name’]) Output: John Doe
“`
Common Use Cases
- Data exchange: APIs often return data in JSON format, which needs to be converted into an object for manipulation.
- Configuration files: JSON strings are commonly used in configuration files, requiring conversion for programmatic access.
- Frontend development: JavaScript frameworks frequently use JSON objects for state management.
Best Practices for JSON Conversion
When converting strings to JSON objects, it is essential to follow best practices to avoid errors and improve code quality:
- Validate JSON String: Ensure the string is a valid JSON format before conversion to prevent runtime errors.
- Error Handling: Implement error handling to catch exceptions during conversion.
- Avoid Circular References: Ensure the data structure does not contain circular references, as this will cause conversion failures.
Error Handling Example in JavaScript
“`javascript
try {
const jsonObject = JSON.parse(jsonString);
} catch (error) {
console.error(“Invalid JSON string:”, error);
}
“`
Error Handling Example in Python
“`python
try:
json_object = json.loads(json_string)
except json.JSONDecodeError as e:
print(“Invalid JSON string:”, e)
“`
Summary of JSON Parsing Functions
Below is a comparison of JSON parsing functions in different languages:
Language | Function | Usage Example |
---|---|---|
JavaScript | JSON.parse() | JSON.parse(‘{“key”: “value”}’) |
Python | json.loads() | json.loads(‘{“key”: “value”}’) |
Java | new JSONObject() | new JSONObject(jsonString) |
Understanding JSON Format
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. It uses a text format that is completely language-independent, making it ideal for data exchange between applications. The structure of JSON consists of key/value pairs, which can be nested to create complex data structures.
Key Characteristics of JSON:
- Data Types: Supports strings, numbers, objects, arrays, booleans, and null values.
- Syntax: Keys must be strings enclosed in double quotes, while values can be any valid JSON data type.
- Objects: Defined by curly braces `{}` and consist of key/value pairs.
- Arrays: Defined by square brackets `[]` and can contain multiple values.
JavaScript Method to Convert String to JSON Object
In JavaScript, the conversion from a string to a JSON object can be accomplished using the `JSON.parse()` method. This method parses a JSON string and constructs the JavaScript value or object described by the string.
Example:
“`javascript
const jsonString = ‘{“name”: “John”, “age”: 30, “city”: “New York”}’;
const jsonObject = JSON.parse(jsonString);
console.log(jsonObject);
“`
Key Points:
- Ensure the input string is in valid JSON format.
- Use `try…catch` to handle potential parsing errors.
Common Errors and Troubleshooting
When converting strings to JSON objects, several common issues may arise:
Error Type | Description | Solution |
---|---|---|
SyntaxError | Thrown when the string is not valid JSON. | Validate JSON format using tools. |
Unexpected Token | Occurs when an illegal character is found in the string. | Check for misplaced commas or quotes. |
TypeError | Happens if the input is not a string. | Ensure the data type is string. |
Best Practices for JSON Conversion
To ensure successful string-to-JSON conversion, follow these best practices:
- Validate JSON: Use online JSON validators before parsing.
- Use `try…catch`: Always wrap `JSON.parse()` in a try-catch block to gracefully handle errors.
- Escape Characters: Ensure that special characters in strings are properly escaped.
- Consistent Formatting: Maintain consistency in key/value formatting to avoid syntax issues.
Example Scenarios
Consider the following scenarios for converting strings to JSON objects:
- Valid JSON String:
“`javascript
const validString = ‘{“product”: “Laptop”, “price”: 1200}’;
const validJson = JSON.parse(validString);
“`
- Invalid JSON String:
“`javascript
const invalidString = ‘{“product”: “Laptop”, “price”: 1200’; // Missing closing brace
try {
const invalidJson = JSON.parse(invalidString);
} catch (error) {
console.error(“Parsing Error: “, error.message);
}
“`
- String with Escape Characters:
“`javascript
const escapeString = ‘{“message”: “Hello, it\’s a great day!”}’;
const escapeJson = JSON.parse(escapeString);
“`
By adhering to these practices and understanding the common pitfalls, developers can effectively convert strings to JSON objects in a reliable manner.
Expert Insights on Converting Strings to JSON Objects
Dr. Emily Chen (Software Engineer, Tech Innovations Inc.). “Converting strings to JSON objects is a fundamental skill for developers, especially in web development. It allows for efficient data interchange between clients and servers, making it essential for modern applications.”
Michael Thompson (Data Scientist, Analytics Hub). “Understanding how to convert strings to JSON objects is crucial for data manipulation and analysis. JSON’s lightweight structure facilitates easier data parsing and integration with various programming languages, enhancing workflow efficiency.”
Sarah Patel (Lead Developer, Cloud Solutions Co.). “Mastering the conversion of strings to JSON objects can significantly improve API interactions. It is important to ensure that the string is properly formatted to avoid errors during the parsing process, which can lead to application failures.”
Frequently Asked Questions (FAQs)
What is a JSON object?
A JSON object is a data structure that represents data in a key-value pair format, commonly used for data interchange between a server and a web application.
How can I convert a string to a JSON object in JavaScript?
You can convert a string to a JSON object in JavaScript using the `JSON.parse()` method. This method takes a valid JSON string as an argument and returns the corresponding JavaScript object.
What happens if the string is not a valid JSON format?
If the string is not in a valid JSON format, the `JSON.parse()` method will throw a `SyntaxError`, indicating that the input cannot be parsed into a JSON object.
Can I convert a JSON object back to a string?
Yes, you can convert a JSON object back to a string using the `JSON.stringify()` method. This method serializes the JavaScript object into a JSON string.
Are there any libraries that can assist with JSON conversion?
Yes, various libraries such as `lodash` and `axios` can assist with JSON conversion and manipulation, providing additional features and functionality beyond the native JavaScript methods.
Is there a way to validate a JSON string before converting it to an object?
Yes, you can validate a JSON string using a try-catch block around the `JSON.parse()` method. If the string is valid, it will convert successfully; if not, the catch block will handle the error.
In summary, converting a string to a JSON object is a fundamental operation in programming, particularly in web development and data manipulation. JSON (JavaScript Object Notation) is widely used for data interchange due to its lightweight and easy-to-read format. The process typically involves parsing a string that is formatted in JSON syntax using built-in functions available in various programming languages, such as `JSON.parse()` in JavaScript, or equivalent methods in Python and other languages.
One of the key insights from the discussion is the importance of ensuring that the string is properly formatted as valid JSON. This includes adhering to rules regarding the use of double quotes for keys and string values, as well as the correct placement of commas and brackets. Failure to maintain these standards can result in parsing errors, which can hinder application functionality and lead to debugging challenges.
Additionally, it is crucial to handle exceptions and errors gracefully during the conversion process. Implementing error handling mechanisms can help developers manage unexpected input and provide meaningful feedback to users. This not only enhances the robustness of applications but also improves user experience by preventing crashes or unexpected behavior.
mastering the conversion of strings to JSON objects is essential for developers working with web APIs and data-driven applications.
Author Profile
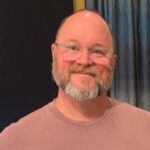
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?