How Can You Effectively Display ViewData[Title] in HTML?
In the ever-evolving world of web development, displaying dynamic content effectively is crucial for creating engaging user experiences. One common challenge developers face is how to present data seamlessly within HTML views, particularly when using frameworks like ASP.NET MVC. Among the various methods available, utilizing `ViewData` to pass information from controllers to views stands out as a powerful and flexible solution. This article delves into the intricacies of displaying `ViewData[Title]` in HTML, offering insights that will enhance your web applications and streamline your coding process.
To successfully display `ViewData[Title]` in HTML, it’s essential to understand the foundational concepts behind `ViewData` itself. This feature allows developers to store and retrieve data in a dictionary-like structure, making it easy to pass information from the server-side to the client-side. By leveraging `ViewData`, you can ensure that your web pages are not only informative but also tailored to the needs of your users. Whether you are displaying page titles, headings, or other critical data, mastering this technique can significantly elevate your web development skills.
As we explore the practical steps to implement `ViewData[Title]` in your HTML views, we will uncover best practices and common pitfalls to avoid. This knowledge will empower you to
Using ViewData to Display Title in HTML
When working with ASP.NET MVC, `ViewData` is a powerful mechanism that allows you to pass data from your controller to your view. Displaying a title using `ViewData` can enhance the user interface by ensuring that the relevant information is dynamically rendered based on the context. Here’s how to effectively display `ViewData[Title]` in your HTML.
To access `ViewData` in your view, you can reference it directly using the key associated with the data. In this case, you would use `ViewData[“Title”]`. Here’s how you can achieve this in your Razor view:
“`html
@ViewData[“Title”]
“`
This line of code will render the title in an `
` HTML element, making it prominent on the page. It’s essential to ensure that the title is set in the controller before the view is returned.
Setting ViewData in the Controller
In your controller, you can set the `ViewData` key like this:
“`csharp
public ActionResult Index()
{
ViewData[“Title”] = “Welcome to My Website”;
return View();
}
“`
This action method sets the title to “Welcome to My Website” before returning the view.
Best Practices for Using ViewData
Using `ViewData` can be beneficial, but it is essential to follow best practices to maintain code readability and maintainability. Consider the following guidelines:
- Use `ViewData` for small amounts of data or temporary data that doesn’t require strong typing.
- Prefer strongly typed models for larger datasets or when the data structure is complex.
- Keep the keys consistent to avoid confusion and errors in retrieving data.
- Document the purpose of each `ViewData` entry to aid future developers.
Example Implementation
Here’s a complete example that demonstrates how to use `ViewData` effectively to display a title:
Controller Code:
“`csharp
public class HomeController : Controller
{
public ActionResult Index()
{
ViewData[“Title”] = “Welcome to My Website”;
return View();
}
}
“`
View Code:
“`html
@{
Layout = null;
}
@ViewData[“Title”]
This is the homepage of our website.
“`
Comparison of ViewData and Strongly Typed Models
While `ViewData` serves a purpose, it’s beneficial to compare it with strongly typed models for clarity:
Feature | ViewData | Strongly Typed Model |
---|---|---|
Type Safety | No | Yes |
Ease of Use | Moderate | High |
Performance | Lower | Higher |
Complexity | Lower | Higher |
In summary, while `ViewData` is a flexible option for passing data to views, using strongly typed models is generally recommended for larger or more complex data structures. This approach enhances type safety and reduces potential runtime errors.
Displaying ViewData in HTML
To display `ViewData` in an HTML view in ASP.NET MVC, you can access the data stored in the `ViewData` dictionary using the key associated with the value. The `ViewData` object allows you to pass data from the controller to the view dynamically.
Accessing ViewData
You can retrieve values from `ViewData` in your Razor view using the following syntax:
“`html
@ViewData[“Title”]
“`
This code snippet will render the value associated with the key “Title” from the `ViewData` dictionary. It is essential to ensure that the key exists to avoid runtime errors.
Example Usage
Suppose you have a controller that sets the `ViewData` as follows:
“`csharp
public ActionResult Index()
{
ViewData[“Title”] = “Welcome to My Website”;
return View();
}
“`
In your corresponding Razor view, you can display the title with:
“`html
@ViewData[“Title”]
“`
This will render:
“`html
Welcome to My Website
“`
Handling Null Values
It is crucial to handle potential null values when accessing `ViewData`. You can use the null-coalescing operator to provide a default value:
“`html
@ViewData[“Title”] ?? “Default Title”
“`
This ensures that if “Title” is not set, “Default Title” will be displayed instead.
Using ViewData with Other HTML Elements
You can incorporate `ViewData` values into various HTML elements. Here are some examples:
- Paragraphs:
“`html
@ViewData[“Description”]
“`
- Links:
“`html
@ViewData[“LinkText”]
“`
- Attributes:
“`html
“`
Best Practices
When using `ViewData`, consider the following best practices:
- Type Safety: Prefer using strongly typed models over `ViewData` for better maintainability and type safety.
- Clear Keys: Use descriptive keys to make it easier to understand the purpose of each piece of data.
- Limit Usage: Use `ViewData` sparingly; excessive use can lead to confusion and harder-to-maintain code.
Comparison with ViewBag and TempData
Feature | ViewData | ViewBag | TempData |
---|---|---|---|
Lifetime | Request | Request | Session |
Type Safety | Non-typed | Dynamic | Non-typed |
Usage | Pass data to views | Pass data to views | Pass data between requests |
Using `ViewBag` offers a dynamic alternative to `ViewData`, while `TempData` is useful for passing data between requests. Choose the appropriate method based on your specific requirements.
By following these guidelines and examples, you can effectively display `ViewData` in your HTML views, ensuring a smooth data-passing experience within your ASP.NET MVC applications.
Expert Insights on Displaying ViewData in HTML
Jessica Lin (Senior Web Developer, Tech Innovations Inc.). “Displaying ViewData in HTML requires a clear understanding of the MVC framework. It is essential to ensure that the data is properly bound to the view, which allows for dynamic content rendering that enhances user experience.”
Mark Thompson (Lead Software Engineer, CodeCraft Solutions). “Utilizing Razor syntax effectively is crucial when displaying ViewData in HTML. By leveraging the power of Razor, developers can seamlessly integrate server-side data into their client-side views, ensuring a fluid and interactive application.”
Emily Carter (Web Application Architect, Digital Dynamics). “When displaying ViewData in HTML, it is vital to implement proper data validation and sanitation techniques. This practice not only enhances security but also improves the overall integrity of the data being presented to the user.”
Frequently Asked Questions (FAQs)
How can I display ViewData in an HTML page?
You can display ViewData in an HTML page by using Razor syntax. For example, use `@ViewData[“Title”]` to render the value stored in the ViewData dictionary.
What is ViewData in ASP.NET MVC?
ViewData is a dictionary object that allows you to pass data from a controller to a view in ASP.NET MVC. It is a dynamic way to share data between the two components.
Can I use ViewData to pass complex objects?
Yes, you can pass complex objects using ViewData, but it is generally recommended to use strongly typed models for better type safety and maintainability.
What is the difference between ViewData and ViewBag?
ViewData is a dictionary of objects that requires type casting, while ViewBag is a dynamic wrapper around ViewData that allows you to access properties without casting.
Is ViewData suitable for large amounts of data?
ViewData is not ideal for large amounts of data due to its dictionary nature and potential performance implications. For larger datasets, consider using models or view models.
How do I check if a ViewData entry exists before displaying it?
You can check if a ViewData entry exists by using the `ViewData.ContainsKey(“key”)` method. If it returns true, you can safely access and display the value.
displaying data in HTML using ViewData is a fundamental technique in ASP.NET MVC applications. ViewData is a dictionary object that allows developers to pass data from the controller to the view. By utilizing ViewData, developers can easily render dynamic content on web pages, enhancing user experience and interactivity. Understanding how to effectively use ViewData to display titles and other data types is crucial for building robust web applications.
One key takeaway is the simplicity and flexibility that ViewData offers. It allows for quick data retrieval in views without the need for strongly typed models. This can be particularly useful for small projects or scenarios where a full model is not necessary. However, it is essential to note that relying heavily on ViewData can lead to less maintainable code, as it lacks compile-time checking and can introduce runtime errors if keys are mistyped.
Another important insight is the best practices associated with using ViewData. While it is a powerful tool, developers should consider using strongly typed views or ViewModels for larger applications to improve code clarity and maintainability. This approach not only enhances type safety but also makes the code more self-documenting, which is beneficial for future development and collaboration.
Author Profile
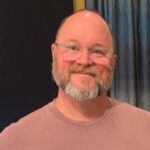
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?