How Can You Effectively Run a Python File in Jenkins?
How To Run Python File In Jenkins: A Comprehensive Guide
In the ever-evolving landscape of software development, automation has become a cornerstone of efficiency and productivity. Jenkins, a powerful open-source automation server, is widely recognized for its ability to streamline the continuous integration and continuous delivery (CI/CD) processes. But what if you want to leverage the versatility of Python within this robust framework? Whether you’re looking to automate testing, deployment, or data processing tasks, knowing how to run a Python file in Jenkins can significantly enhance your workflow.
This article will guide you through the essential steps to seamlessly integrate Python scripts into your Jenkins jobs. From setting up your Jenkins environment to configuring the necessary plugins and executing your scripts, we will cover the foundational aspects that will empower you to harness the full potential of Python in your CI/CD pipelines. By the end, you will have a clear understanding of how to automate your Python processes in Jenkins, paving the way for more efficient development cycles.
As we delve deeper, you will discover the best practices for managing dependencies, handling virtual environments, and troubleshooting common issues that may arise. Whether you are a seasoned developer or just starting your journey with Jenkins and Python, this guide will equip you with the knowledge you need to optimize your automation tasks effectively.
Setting Up Jenkins for Python
To run a Python file in Jenkins, the first step is to ensure that your Jenkins environment is properly configured to support Python. This involves installing the necessary plugins and ensuring that the Python interpreter is accessible from the Jenkins server. Here are the key steps to accomplish this:
– **Install Python**: Ensure Python is installed on the Jenkins server. You can verify this by running `python –version` or `python3 –version` in the command line.
– **Install Required Plugins**: Navigate to the Jenkins dashboard and go to “Manage Jenkins” > “Manage Plugins”. Install the following plugins if they are not already installed:
– **Python Plugin**: This plugin helps integrate Python into Jenkins jobs.
– **ShiningPanda Plugin**: This plugin allows you to create virtual environments and run Python scripts.
– **Configure Python in Jenkins**: Go to “Manage Jenkins” > “Global Tool Configuration”. Under the Python section, add the path to your Python installation.
Creating a Jenkins Job to Run Python Script
Once the environment is set up, you can create a Jenkins job specifically for executing your Python script. Follow these steps:
- Create a New Job: From the Jenkins dashboard, click on “New Item”. Enter a name for your job and select “Freestyle project”.
- Source Code Management: If your Python script is stored in a version control system (like Git), configure the repository settings under the “Source Code Management” section.
- Build Environment: In the “Build Environment” section, you might want to enable the “Use secret text(s) or file(s)” option if your script requires sensitive information.
- Build Steps: Under the “Build” section, add a build step by selecting “Execute shell” or “Execute Python script”. If using “Execute shell”, you can run your Python script with a command such as:
“`bash
python path/to/your_script.py
“`
Or, for Python 3:
“`bash
python3 path/to/your_script.py
“`
Managing Dependencies and Virtual Environments
It is a best practice to manage dependencies using virtual environments. The ShiningPanda plugin allows you to create a virtual environment for your Python project directly within Jenkins. Here’s how to use it:
- Add a Build Step: Select “Virtualenv Builder” under the build steps.
- Configure Virtual Environment: Specify the name and location of the virtual environment. You can also specify dependencies in a requirements.txt file.
By managing dependencies this way, you ensure that your Python script runs in an isolated environment, reducing the risk of conflicts with other projects.
Example Jenkins Pipeline Script
For a more advanced setup, consider using a Jenkins Pipeline script. Below is an example of how to structure a pipeline to run a Python script:
“`groovy
pipeline {
agent any
stages {
stage(‘Clone Repository’) {
steps {
git ‘https://github.com/your-repo.git’
}
}
stage(‘Setup Environment’) {
steps {
sh ‘python3 -m venv venv’
sh ‘./venv/bin/pip install -r requirements.txt’
}
}
stage(‘Run Script’) {
steps {
sh ‘./venv/bin/python your_script.py’
}
}
}
}
“`
This pipeline will:
- Clone the repository.
- Set up a virtual environment and install dependencies.
- Execute the Python script within that environment.
Viewing Results and Logs
After running the job, you can view the output and logs directly in the Jenkins interface. Navigate to the specific job and click on the build number to access the console output. This is essential for debugging and ensuring that your Python script executed correctly.
Stage | Description |
---|---|
Clone Repository | Fetches the latest code from the specified Git repository. |
Setup Environment | Creates a virtual environment and installs the necessary dependencies. |
Run Script | Executes the Python script in the configured environment. |
Prerequisites for Running Python Files in Jenkins
Before running Python files in Jenkins, ensure the following prerequisites are met:
- Jenkins Installed: Ensure you have Jenkins installed on your server or local machine.
- Python Installed: Python must be installed on the machine where Jenkins is running.
- Jenkins Plugins: The following plugins should be installed for efficient execution:
- Pipeline: For defining jobs as code.
- SSH Build Agents: If you’re executing scripts on remote machines.
- Git: To pull code from repositories if needed.
Configuring Jenkins to Run Python Files
To configure Jenkins to run a Python script, follow these steps:
- Create a New Job:
- Open Jenkins Dashboard.
- Click on “New Item”.
- Enter a name for the job and select “Freestyle project” or “Pipeline”.
- Configure Source Code Management (if applicable):
- Select Git or another SCM option.
- Provide the repository URL and credentials.
- Set Up Build Environment:
- In the “Build Environment” section, you can set any environment variables or specify the Python virtual environment if needed.
- Add Build Step:
- Under the “Build” section, choose “Execute Shell” for Linux or “Execute Windows batch command” for Windows.
- Enter the command to run your Python file. For example:
- For Linux:
“`bash
python3 /path/to/your_script.py
“`
- For Windows:
“`cmd
python C:\path\to\your_script.py
“`
Using Jenkins Pipeline to Run Python Scripts
Using a Jenkins pipeline allows for more flexibility and control over the build process. Below is a simple example of a Jenkinsfile that runs a Python script:
“`groovy
pipeline {
agent any
stages {
stage(‘Clone Repository’) {
steps {
git ‘https://github.com/your-repo.git’
}
}
stage(‘Run Python Script’) {
steps {
sh ‘python3 your_script.py’
}
}
}
}
“`
In this example:
- agent any: Specifies that the pipeline can run on any available agent.
- stages: Defines the different stages of the pipeline.
- steps: Contains the commands to execute in each stage.
Handling Dependencies
If your Python script requires external libraries, consider using a virtual environment. Here’s how to set it up in Jenkins:
- Create a Virtual Environment:
- Add a build step to create a virtual environment:
“`bash
python3 -m venv venv
“`
- Activate the Virtual Environment and Install Dependencies:
- Add the following commands in the build step:
“`bash
source venv/bin/activate
pip install -r requirements.txt
“`
- Run Your Python Script:
- Finally, run the script using the activated environment:
“`bash
python your_script.py
“`
Viewing Build Results
After executing your job, Jenkins provides several ways to view the results:
- Console Output: Access the console output of the build to see logs and any error messages by clicking on the build number.
- Email Notifications: Configure Jenkins to send email notifications on build success or failure.
For more advanced logging, consider integrating with tools such as Logstash or using Jenkins’ built-in logging features.
Expert Insights on Running Python Files in Jenkins
Dr. Emily Carter (DevOps Engineer, Tech Innovations Inc.). “To effectively run Python files in Jenkins, it is crucial to configure the Jenkins job correctly. This includes setting up the Python environment, using virtual environments if necessary, and ensuring that all dependencies are installed. Additionally, leveraging Jenkins pipelines can streamline the process and improve maintainability.”
Michael Chen (Senior Software Developer, CodeCraft Solutions). “Integrating Python scripts into Jenkins is straightforward when you utilize the ‘Execute Shell’ build step. It is essential to ensure that the Python interpreter is correctly referenced in the command. Furthermore, consider implementing error handling within your scripts to manage failures gracefully during the CI/CD process.”
Sarah Johnson (Continuous Integration Specialist, AgileTech Labs). “One of the best practices when running Python files in Jenkins is to use Jenkinsfile for defining your pipeline. This approach allows for version control of your CI/CD process. Additionally, incorporating testing frameworks within your Python scripts can help ensure that any changes made during development do not break existing functionality.”
Frequently Asked Questions (FAQs)
How do I set up Jenkins to run a Python file?
To set up Jenkins for running a Python file, first ensure that Jenkins is installed and configured on your system. Then, create a new job in Jenkins, select “Freestyle project,” and in the build section, add a build step to execute a shell command. Use the command `python path/to/your_script.py` to run your Python file.
What plugins are necessary for running Python scripts in Jenkins?
While no specific plugins are required to run Python scripts, the “ShiningPanda” plugin can enhance Python support. It allows you to create and manage Python virtual environments, making it easier to handle dependencies for your scripts.
Can I pass parameters to my Python script when running it in Jenkins?
Yes, you can pass parameters to your Python script in Jenkins. You can define parameters in your Jenkins job configuration and then reference them in the shell command as `python path/to/your_script.py param1 param2`.
How do I handle Python dependencies in Jenkins?
To handle Python dependencies in Jenkins, you can use a virtual environment. Create a virtual environment within your Jenkins job using a shell command, activate it, and then install the required packages using `pip install -r requirements.txt` before running your Python script.
What should I do if my Python script fails to run in Jenkins?
If your Python script fails to run in Jenkins, check the console output for error messages. Common issues include incorrect paths, missing dependencies, or permission errors. Ensure that the Python interpreter is correctly configured in Jenkins and that all necessary libraries are installed.
Is it possible to schedule Python scripts to run at specific times in Jenkins?
Yes, Jenkins allows you to schedule jobs to run at specific times using the “Build Triggers” section. You can set up a cron-like schedule by configuring the “Build periodically” option, allowing you to automate the execution of your Python scripts.
running a Python file in Jenkins is a straightforward process that involves several key steps. First, it is essential to ensure that Jenkins is properly installed and configured on your server. Once Jenkins is set up, you can create a new job or project where you will define the necessary parameters for executing your Python script. This includes specifying the source code repository, build triggers, and build environment settings.
Another crucial aspect is the configuration of the build steps. You can utilize the “Execute Shell” or “Execute Windows batch command” options to run your Python script. It is important to ensure that the Python environment is correctly set up within Jenkins, which may involve installing Python on the Jenkins server and configuring the PATH variable accordingly. Additionally, using virtual environments can help manage dependencies and ensure that your script runs in an isolated environment.
Finally, monitoring the execution of your Python script through Jenkins is vital for troubleshooting and ensuring successful builds. Jenkins provides logs and console output that can help identify any issues that arise during the execution process. By following these steps, you can effectively integrate Python scripts into your CI/CD pipeline, enhancing automation and streamlining your development workflow.
Author Profile
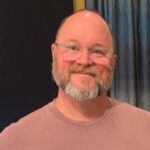
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?