Why Are Vite Tsconfig Vitest Globals Not Working with Webpack?
In the ever-evolving landscape of web development, the choice of tools can significantly impact both the efficiency of your workflow and the performance of your applications. Among the myriad of frameworks and bundlers available, Vite has emerged as a powerful contender, particularly for projects leveraging TypeScript. However, as developers transition from traditional setups like Webpack to Vite, they often encounter challenges—one of the most perplexing being the integration of Vitest and global configurations in their TypeScript setup. If you’ve found yourself wrestling with these issues, you’re not alone.
This article delves into the intricacies of configuring Vite with TypeScript, specifically focusing on the nuances of Vitest and the challenges that arise when trying to replicate the familiar global settings from Webpack. As we explore the common pitfalls and solutions, you’ll gain insights into how to streamline your testing environment and ensure that your global variables are recognized seamlessly. Whether you’re a seasoned developer or just starting your journey with Vite, understanding these configurations is crucial for maximizing productivity and minimizing frustration in your development process.
Join us as we uncover the complexities of Vite’s tsconfig, the role of Vitest in modern testing frameworks, and how to navigate the transition from Webpack to a more efficient Vite setup. By the end of this
Understanding Vite’s Configuration for Vitest
When working with Vite and Vitest, configuring your TypeScript project correctly is essential to ensure that global variables and types are recognized properly. Unlike Webpack, Vite’s approach to handling TypeScript and testing environments can be quite different, leading to potential issues if not set up correctly.
To get started, ensure that your `tsconfig.json` is set up to include the necessary paths and types. Here’s a sample configuration that could help:
“`json
{
“compilerOptions”: {
“target”: “ESNext”,
“module”: “ESNext”,
“moduleResolution”: “Node”,
“strict”: true,
“esModuleInterop”: true,
“skipLibCheck”: true,
“forceConsistentCasingInFileNames”: true,
“types”: [“vitest/globals”]
},
“include”: [“src//*.ts”, “src//*.tsx”, “src//*.vue”, “tests//*.ts”]
}
“`
Key points in this configuration include:
- The `types` array includes `vitest/globals`, which allows Vitest to recognize global variables and types.
- The `include` property specifies the directories and file types that TypeScript should consider for compilation.
Common Issues with Vitest and TypeScript
When integrating Vitest with Vite and TypeScript, developers often encounter specific issues:
- Global Types Not Recognized: If you see errors indicating that global types are not recognized, double-check that the `types` array in `tsconfig.json` includes `vitest/globals`.
- Module Resolution Issues: Ensure that your paths in the `include` section cover all the necessary directories where your test files are located.
- Transpilation Problems: Vite uses ES modules, so make sure your TypeScript configuration aligns with this module system.
Debugging Configuration Problems
If you run into configuration problems, consider the following debugging steps:
- Check for Typos: Ensure there are no typos in your `tsconfig.json` file.
- Validate Configuration: Use TypeScript’s CLI to validate your configuration:
“`bash
tsc –noEmit
“`
- Review Vitest Documentation: The official Vitest documentation can provide insights into the latest features and configuration options.
Comparing Vite with Webpack
While both Vite and Webpack serve the purpose of module bundling, their configurations and behavior differ significantly. Here’s a comparison table highlighting some of the key differences:
Feature | Vite | Webpack |
---|---|---|
Build Time | Fast, using native ES modules | Can be slower, especially for large projects |
Configuration Complexity | Simple, minimal configuration | Can be complex with multiple configurations |
Hot Module Replacement (HMR) | Instant updates | Requires configuration |
Testing | Integrated with Vitest for seamless testing | Requires additional setup with Jest or similar |
By understanding these differences, developers can better appreciate the advantages of using Vite with Vitest, especially when it comes to speed and ease of configuration.
Understanding Vite and Webpack Configurations
Vite and Webpack are two popular build tools used in modern web development, each with unique configurations and optimizations. When transitioning from Webpack to Vite, developers may encounter issues, particularly with TypeScript configurations and test environments.
Vite’s Configuration Approach
- Vite uses `vite.config.js` or `vite.config.ts` for its configuration.
- It relies heavily on ES modules, which allows for faster builds and hot module replacement (HMR).
- Vite handles TypeScript seamlessly by leveraging `tsconfig.json`, but specific configurations might be necessary.
Common Configuration Issues
- Path Aliases: Ensure that path aliases defined in `tsconfig.json` are also reflected in Vite’s configuration.
- Example:
“`json
// tsconfig.json
{
“compilerOptions”: {
“baseUrl”: “.”,
“paths”: {
“@/*”: [“src/*”]
}
}
}
“`
- Corresponding Vite config:
“`javascript
import { defineConfig } from ‘vite’;
import { resolve } from ‘path’;
export default defineConfig({
resolve: {
alias: {
‘@’: resolve(__dirname, ‘src’)
}
}
});
“`
- Testing with Vitest: When using Vitest, ensure that the testing environment is correctly set up to recognize global variables and TypeScript types.
Vitest Global Configuration
- Vitest allows for global setups that can be defined in the configuration file:
“`javascript
// vitest.config.ts
import { defineConfig } from ‘vitest/config’;
export default defineConfig({
test: {
globals: true,
setupFiles: ‘./src/setupTests.ts’
}
});
“`
- Define global types in a `global.d.ts` file:
“`typescript
// src/global.d.ts
declare global {
// Custom global variables or types
const myGlobalVar: string;
}
“`
Troubleshooting Common Issues
Problem | Solution |
---|---|
Globals not recognized in Vitest | Ensure `globals: true` is set in `vitest.config.ts` and that global types are declared. |
TypeScript errors related to modules | Check `tsconfig.json` for correct module resolution settings and ensure paths are correct. |
Tests running but failing to find imports | Verify that import paths in test files match the alias settings in both `tsconfig.json` and Vite config. |
Key Differences Between Vite and Webpack
Feature | Vite | Webpack |
---|---|---|
Development Mode | Instant server start | Bundles all files before serving |
Hot Module Replacement (HMR) | Fast, due to native ESM support | Slower, relies on full re-bundling |
Configuration | Simpler, less boilerplate | More complex, extensive configuration |
TypeScript Integration | Directly uses `tsconfig.json` | Requires additional loaders/plugins |
When transitioning from Webpack to Vite, it’s crucial to re-evaluate your configurations and ensure that TypeScript and testing setups are appropriately aligned. This will minimize friction and enhance development efficiency.
Expert Insights on Vite, Tsconfig, and Vitest Integration Challenges
“Jessica Lin (Senior Software Engineer, Frontend Innovations). As Vite continues to gain traction in the development community, many developers encounter issues when integrating Vitest with their TypeScript configurations. The key challenge often lies in the differences between Vite’s module resolution and Webpack’s. Developers must ensure that their tsconfig.json is correctly set up to recognize the global types introduced by Vitest, which can often be overlooked.”
“Mark Thompson (Technical Lead, Modern Web Solutions). The transition from Webpack to Vite can be daunting, particularly when it comes to testing frameworks like Vitest. A common pitfall is the misconfiguration of the tsconfig.json file, which can lead to globals not being recognized. I recommend double-checking the ‘types’ array in your tsconfig to include ‘vitest’ and ensuring that the ‘moduleResolution’ is set to ‘node’ for better compatibility.”
“Sofia Ramirez (Frontend Architect, CodeCraft). In my experience, integrating Vitest with Vite while using TypeScript requires careful attention to the project’s configuration files. Issues often arise due to the lack of proper type definitions or incorrect paths in the tsconfig.json. It is crucial to align the project’s structure with Vite’s expectations and to utilize the Vitest documentation for guidance on setting up global types effectively.”
Frequently Asked Questions (FAQs)
What is Vite and how does it differ from Webpack?
Vite is a modern build tool that focuses on speed and performance, utilizing native ES modules in the development environment. Unlike Webpack, which bundles files ahead of time, Vite serves files on-demand, resulting in faster startup and hot module replacement.
How do I configure tsconfig.json for a Vite project?
To configure tsconfig.json for a Vite project, ensure that the compiler options align with Vite’s requirements. Common settings include specifying “module”: “ESNext”, “target”: “ESNext”, and including paths for any custom modules or types you may be using.
What are Vitest globals and how do they work?
Vitest globals are predefined global variables provided by Vitest for testing purposes. They include functions like `describe`, `it`, and `expect`, which simplify writing tests by eliminating the need to import them explicitly in every test file.
Why might Vitest globals not work in my Vite project?
Vitest globals may not work if the testing environment is not properly configured. Ensure that Vitest is installed, included in your Vite configuration, and that your test files are recognized by the testing framework.
How can I troubleshoot issues with tsconfig.json when using Vite and Vitest?
To troubleshoot tsconfig.json issues, check for conflicting settings, ensure that the “types” array includes “vitest”, and verify that paths to any custom types are correctly defined. Running `tsc –noEmit` can also help identify type errors.
Can I use Webpack with Vitest, and how does it affect the globals?
While you can use Webpack alongside Vitest, it is not recommended due to potential conflicts in module resolution and global definitions. Vitest is optimized for Vite’s architecture, and using Webpack may lead to issues with the availability of Vitest globals.
In the context of modern web development, the integration of Vite and Vitest with TypeScript presents unique challenges, particularly when transitioning from a Webpack-based setup. Developers often encounter issues with the configuration of the `tsconfig.json` file, which can lead to problems in recognizing global types and modules. This is particularly evident when trying to utilize Vitest for testing, as it may not seamlessly align with the TypeScript settings that were previously established in a Webpack environment.
One of the critical insights is the importance of properly configuring the `tsconfig.json` file to ensure that TypeScript recognizes the global types provided by Vitest. This includes specifying the correct paths and ensuring that the necessary type definitions are included. Additionally, developers should be aware of the differences in how Vite and Webpack handle module resolution and global declarations, which can further complicate the migration process.
Overall, while Vite offers significant advantages in terms of speed and simplicity, the transition from Webpack requires careful attention to configuration details. Developers must take the time to adjust their TypeScript settings and understand the nuances of Vitest to ensure a smooth development and testing experience. By addressing these issues proactively, teams can leverage the benefits of Vite and Vitest
Author Profile
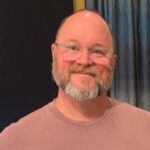
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?